From Idea to Reality: Building Concept-Ecomm with Next.js and Tailwind CSS
Project Genesis
Cultivating a Vision: My Journey into Plant E-Commerce with Apple Vision Pro
From Idea to Implementation
Journey from Concept to Code: Apple Vision Pro Plant E-commerce Web Application
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Conclusion
Under the Hood
Technical Deep-Dive: Apple Vision Pro Plant E-commerce Web Application
1. Architecture Decisions
Key Architectural Choices:
- Next.js: Provides a robust framework for building React applications with built-in routing, API routes, and optimized performance.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development without the need for writing custom CSS for every component.
- TypeScript: Adds type safety to JavaScript, reducing runtime errors and improving code quality through static type checking.
2. Key Technologies Used
Next.js
- File-based Routing: Automatically creates routes based on the file structure in the
pages
directory. - API Routes: Allows for backend functionality within the same codebase, making it easier to handle data fetching and server-side logic.
Tailwind CSS
<button className="bg-green-500 hover:bg-green-700 text-white font-bold py-2 px-4 rounded">
Add to Cart
</button>
TypeScript
interface Product {
id: number;
name: string;
price: number;
imageUrl: string;
}
3. Interesting Implementation Details
State Management
import React, { createContext, useContext, useReducer } from 'react';
const CartContext = createContext();
const cartReducer = (state, action) => {
switch (action.type) {
case 'ADD_TO_CART':
return [...state, action.payload];
case 'REMOVE_FROM_CART':
return state.filter(item => item.id !== action.payload.id);
default:
return state;
}
};
export const CartProvider = ({ children }) => {
const [state, dispatch] = useReducer(cartReducer, []);
return (
<CartContext.Provider value={{ state, dispatch }}>
{children}
</CartContext.Provider>
);
};
export const useCart = () => useContext(CartContext);
Responsive Design
md:w-1/2
and lg:w-1/3
can be used to adjust the layout based on the screen size.<div className="max-w-sm rounded overflow-hidden shadow-lg">
<img className="w-full" src={product.imageUrl} alt={product.name} />
<div className="px-6 py-4">
<div className="font-bold text-xl mb-2">{product.name}</div>
<p className="text-gray-700 text-base">${product.price}</p>
</div>
</div>
4. Technical Challenges Overcome
Handling Asynchronous Data Fetching
getServerSideProps
, we were able to fetch data on the server side, ensuring that the page is pre-populated with data before it reaches the client.export async function getServerSideProps() {
const res = await fetch('https://api.example.com/products');
const products = await res.json();
return { props: { products } };
}
Managing Environment Variables
.env.local
file allows for local development without exposing sensitive data in the codebase.Performance Optimization
next/image
component allows for automatic resizing and lazy loading of images, which significantly improves load times.import Image from 'next/image';
<Image src={product.imageUrl} alt={product.name} width={500} height={500} />
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
State Management: Implementing a robust state management solution (like React Context or Zustand) is crucial for managing the cart and product listings effectively. This helps in maintaining a consistent state across components and improves the user experience.
-
Type Safety with TypeScript: Utilizing TypeScript helped catch errors during development, especially when dealing with props and state. Defining interfaces for product data and cart items improved code readability and maintainability.
-
Responsive Design: Tailwind CSS made it easier to implement responsive design principles. Learning how to use utility classes effectively allowed for rapid prototyping and adjustments to the layout for different screen sizes.
-
Environment Variables: Setting up environment variables correctly is essential for managing API keys and other sensitive information. Understanding how to use
.env.local
files in Next.js was a valuable lesson.
What Worked Well
-
Development Speed: The combination of Next.js and Tailwind CSS significantly accelerated the development process. Next.js’s file-based routing and Tailwind’s utility-first approach allowed for quick iterations.
-
User Experience: The application’s design, inspired by Apple Vision Pro, provided a modern and clean user interface that enhanced the overall shopping experience. Users found it easy to navigate and manage their carts.
-
Performance: Next.js’s built-in optimizations, such as server-side rendering and static site generation, contributed to fast load times and improved SEO, which is crucial for e-commerce applications.
What You’d Do Differently
-
Testing: Implementing a more comprehensive testing strategy (unit tests, integration tests, and end-to-end tests) would have been beneficial. Using tools like Jest and React Testing Library could help ensure the reliability of components and features.
-
Accessibility: While the design was visually appealing, more focus on accessibility (a11y) would improve the application for users with disabilities. Implementing ARIA roles and ensuring keyboard navigation would be a priority in future iterations.
-
API Integration: If the application were to scale, a more structured approach to API integration (using a service layer or hooks) would help manage data fetching and error handling more effectively.
Advice for Others
-
Plan Your Architecture: Before diving into coding, take the time to plan the architecture of your application. Consider how components will interact, how state will be managed, and how data will flow through the app.
-
Leverage Documentation: Make use of the extensive documentation available for Next.js, Tailwind CSS, and TypeScript. Understanding the best practices and features of these tools can save time and prevent common pitfalls.
-
Iterate Based on Feedback: After launching the initial version, gather user feedback and iterate on the design and functionality. Continuous improvement based on real user experiences is key to building a successful application.
-
Stay Updated: The web development landscape is constantly evolving. Stay updated with the latest features and best practices in the frameworks and libraries you use to ensure your application remains modern and efficient.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
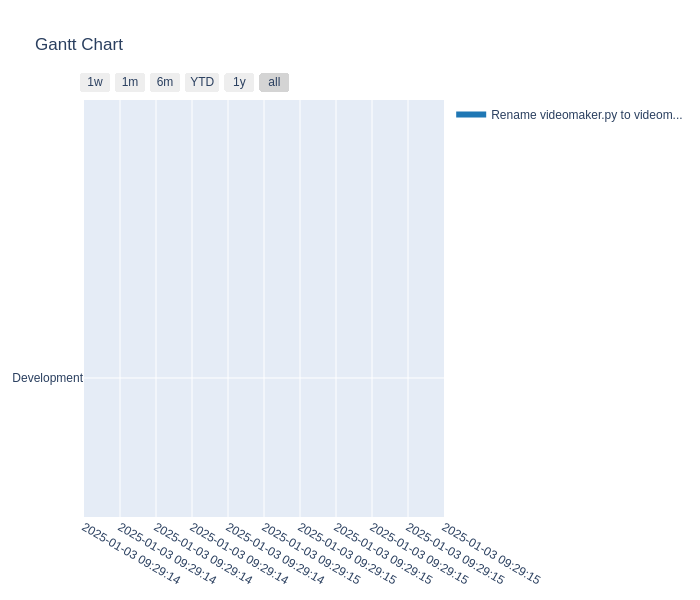
Commit Activity Heatmap
Contributor Network
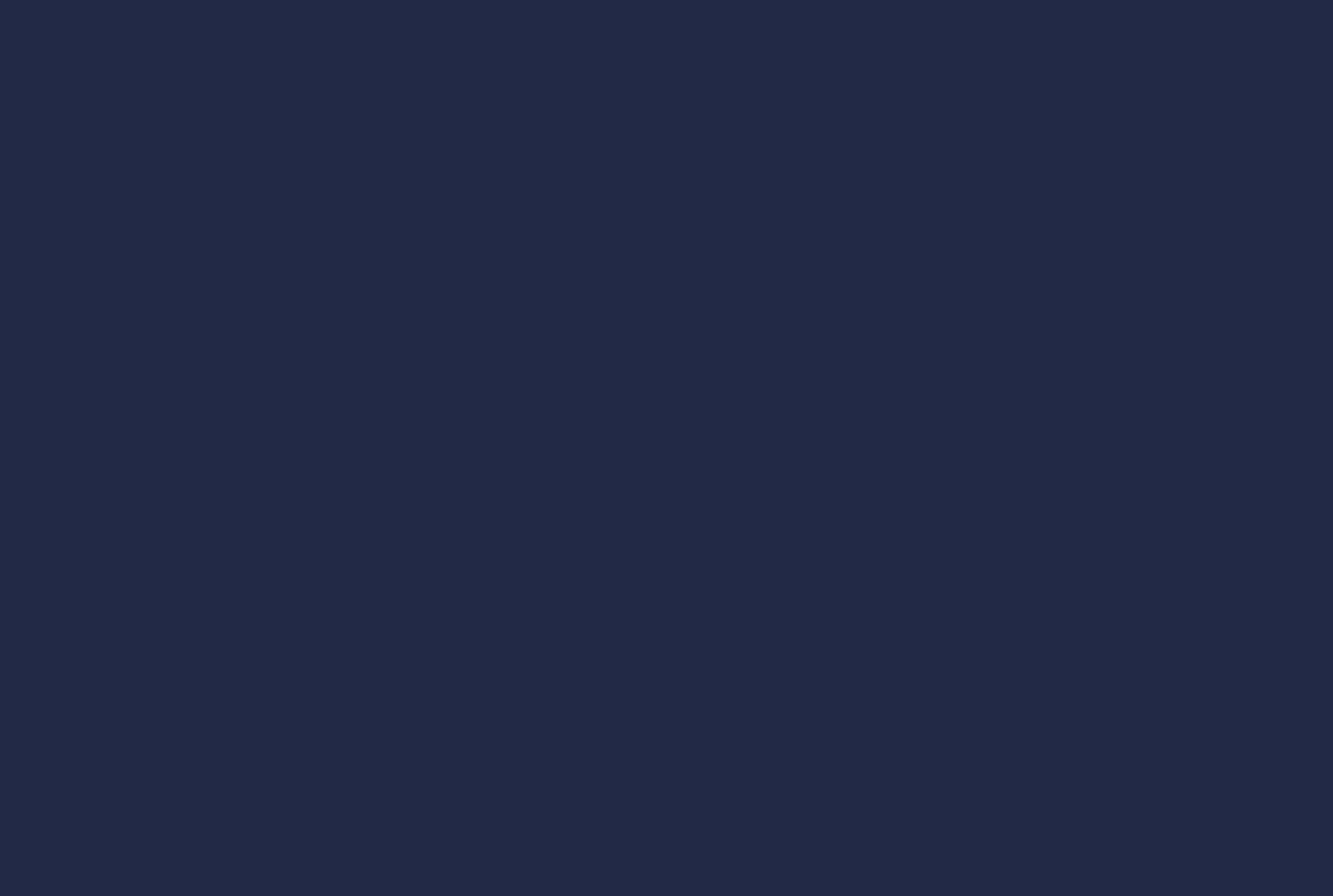
Commit Activity Patterns
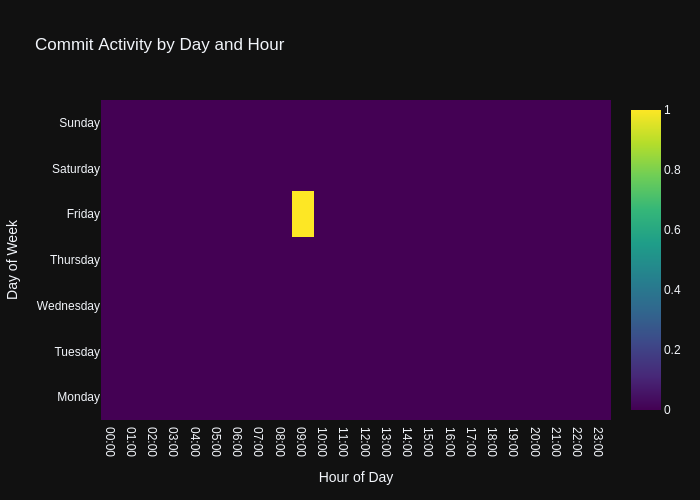
Code Frequency
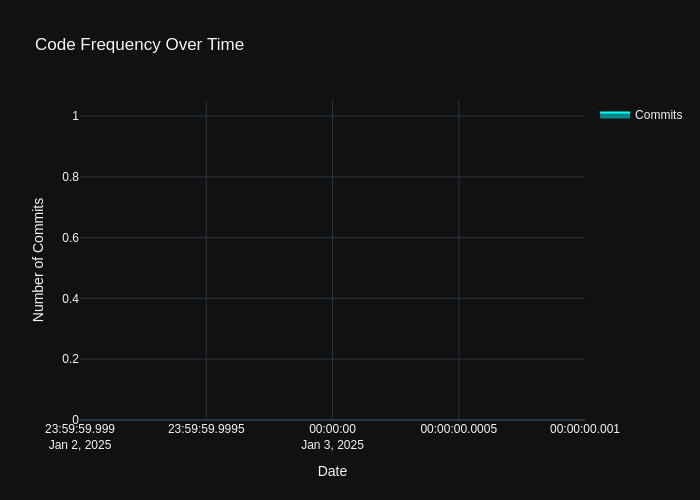
- Repository URL: https://github.com/wanghaisheng/concept-ecomm
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日