Building Color-Palette-Hunter: Unveiling UI Trends from Top 100 Apps
Project Genesis
Discovering the Art of Color: My Journey with Color-Palette-Hunter
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Data Acquisition Method: After evaluating various sources, it was decided to use a combination of APIs and web scraping. APIs from the App Store and Play Store were prioritized for their accuracy, while web scraping was considered a fallback option for additional data. This dual approach ensured a robust data collection strategy.
-
Screenshot Capture: The choice to use emulators (Android Studio and Xcode) was driven by the need for control over the screenshot process. Emulators allow for consistent environments, reducing variability caused by different devices. This decision also facilitated automation through command-line tools, streamlining the workflow.
-
Color Palette Extraction: The selection of libraries like
ColorThief
andPillow
was based on their ease of use and effectiveness in color analysis. K-means clustering was chosen for its ability to identify dominant colors, which is crucial for generating a representative color palette. -
Markdown Generation: Utilizing
jinja2
for templating was a strategic choice to maintain flexibility in generating markdown files. This library allows for dynamic content generation, making it easier to adapt the output format as needed.
3. Alternative Approaches Considered
-
Manual Data Collection: Initially, there was a thought to manually compile a list of top apps from tech blogs. However, this approach was quickly dismissed due to the time-consuming nature and the potential for outdated information. Automating data acquisition through APIs and scraping was deemed more efficient.
-
Third-Party Screenshot Services: While services like BrowserStack and LambdaTest were considered for capturing screenshots, the associated costs and potential limitations in customization led to the decision to rely on emulators. This choice allowed for greater control over the screenshot process and reduced ongoing expenses.
-
Different Color Extraction Algorithms: Other algorithms, such as median cut or octree quantization, were evaluated for color extraction. However, k-means clustering was favored for its balance of performance and simplicity, making it easier to implement and understand.
4. Key Insights That Shaped the Project
-
Importance of Color in User Experience: Research highlighted the psychological impact of color on user behavior and perception. Understanding this relationship reinforced the project’s goal of analyzing color palettes, emphasizing the need for a thoughtful approach to color selection in app design.
-
Dynamic Nature of App UIs: The realization that app interfaces are often dynamic and can change based on user interactions underscored the challenges of capturing accurate screenshots. This insight led to the decision to use emulators, which provide a more stable environment for consistent results.
-
Scalability Considerations: Early on, it became clear that the project needed to be scalable. This influenced decisions around data storage, automation, and the choice of technologies for website publishing. Ensuring that the project could grow and adapt to include more apps and features was a priority.
-
Legal and Ethical Considerations: The importance of adhering to app store guidelines and copyright laws became apparent during the research phase. This awareness shaped the project’s approach to data collection and the use of screenshots, ensuring that the project remained ethical and compliant with industry standards.
Conclusion
Under the Hood
Technical Deep-Dive: Learn UI Color Palette from Master
1. Architecture Decisions
-
Data Acquisition Module: Responsible for fetching data about the top 100 apps from various sources. This module can be easily extended to include new data sources or methods of acquisition.
-
Screenshot Capture Module: This module handles the capturing of app screenshots using emulators or third-party services. It abstracts the complexity of different platforms (iOS and Android) and screen sizes.
-
Color Palette Extraction Module: This module processes the captured screenshots to extract dominant colors using image processing techniques.
-
Markdown Generation Module: This module generates Markdown files that document the app’s name, screenshot, color palette, and additional analysis.
-
Website Publishing Module: This module is responsible for rendering the generated Markdown files into a user-friendly website.
2. Key Technologies Used
-
Python: The primary programming language used for data acquisition, screenshot capture, color extraction, and Markdown generation. Python’s rich ecosystem of libraries makes it ideal for this project.
-
Libraries:
requests
: For making HTTP requests to fetch app data.BeautifulSoup
: For parsing HTML and scraping app data from web pages.Pillow (PIL)
: For image manipulation and processing.ColorThief
: For extracting dominant colors from images.jinja2
: For templating and generating Markdown files.
-
Emulators: Android Studio and Xcode are used for capturing screenshots of mobile applications, providing a controlled environment for testing.
-
Static Site Generators: Technologies like Jekyll, Hugo, or Next.js can be used for publishing the generated Markdown files as a website.
3. Interesting Implementation Details
Data Acquisition
def get_top_apps():
url = "https://example-app-ranking-site.com/top-apps" # replace with the right site
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
app_names = [item.text for item in soup.find_all(".app-name-class")] # Replace with the right class
app_ids = [item.get('data-app-id') for item in soup.find_all(".app-item-class")] # Replace with the right class
return dict(zip(app_ids, app_names))
Screenshot Capture
def take_screenshot(app_id, app_name):
# Placeholder
print(f"Capturing Screenshot for {app_name}")
image = Image.new('RGB', (200, 400), color='black')
image.save(f"screenshots/{app_id}_{app_name.replace(' ', '_')}.png")
return f"screenshots/{app_id}_{app_name.replace(' ', '_')}.png"
Color Palette Extraction
ColorThief
library to identify dominant colors from the captured screenshots. The following code snippet demonstrates this process:def extract_color_palette(image_path):
color_thief = ColorThief(image_path)
palette = color_thief.get_palette(color_count=5)
return [f"#{r:02x}{g:02x}{b:02x}" for r, g, b in palette]
Markdown Generation
jinja2
templating engine to create structured documentation for each app. The following code snippet shows how to generate a Markdown file:def generate_markdown(app_name, screenshot_path, color_palette):
template_str = """
# {{ app_name }}

## Color Style Analysis Procedure
The color palette for this app was extracted by analyzing the provided app screenshot. A color clustering algorithm was used to extract the 5 most dominant colors.
## Color Report
The primary colors in this app are {{color_palette}}
## Color Style Tailwind Code
```js
module.exports = {
theme: {
extend: {
colors: {
{%- for color in color_palette %}
{{ 'color'+loop.index }}: '{{color}}',
{%- endfor %}
}
}
}
}
```
## Color Style Other Code
```css
:root {
{%- for color in color_palette %}
--
## Lessons from the Trenches
### Key Technical Lessons Learned
1. **API vs. Scraping**: Choosing between using APIs and web scraping was crucial. APIs provide structured data and are generally more reliable, but they often require authentication and have usage limits. Scraping can be more flexible but is prone to breaking if the website structure changes. Understanding the trade-offs helped in making informed decisions.
2. **Image Processing**: Extracting color palettes from images using libraries like `colorthief` was straightforward, but understanding the nuances of color representation (e.g., RGB vs. HEX) and the impact of image quality on results was essential. Experimenting with different algorithms (like k-means clustering) provided better insights into color extraction.
3. **Markdown Generation**: Using templating engines like Jinja2 simplified the process of generating structured Markdown files. It allowed for dynamic content insertion and made the code cleaner and more maintainable.
4. **Error Handling**: Implementing robust error handling was critical, especially when dealing with network requests and file operations. This ensured that the program could gracefully handle issues like timeouts or missing files without crashing.
5. **Automation**: Automating the screenshot capture process using emulators was a game-changer. It saved time and ensured consistency across screenshots. However, setting up the environment for emulators required careful configuration and testing.
### What Worked Well
1. **Modular Code Structure**: Breaking down the project into distinct functions (data acquisition, screenshot capture, color extraction, Markdown generation) made the code easier to manage and debug. Each function had a clear responsibility, which facilitated testing and future enhancements.
2. **Use of Libraries**: Leveraging existing libraries (like `requests`, `BeautifulSoup`, `Pillow`, and `colorthief`) significantly accelerated development. These libraries provided robust solutions for common tasks, allowing for a focus on higher-level logic.
3. **Markdown Output**: The Markdown generation process produced well-structured and visually appealing outputs. This made it easy to present the data in a user-friendly format, which is essential for the final website.
4. **Initial Testing with a Small Dataset**: Starting with a small set of apps allowed for quick iterations and testing of the entire workflow. This approach helped identify potential issues early on without overwhelming complexity.
### What You'd Do Differently
1. **Data Source Selection**: I would invest more time in evaluating and selecting the best data source for app rankings. A reliable and comprehensive source would streamline the data acquisition process and reduce the need for frequent updates.
2. **Screenshot Automation**: I would explore more advanced automation tools or services for screenshot capture earlier in the process. This could include using headless browsers or dedicated screenshot APIs to improve efficiency and reduce manual intervention.
3. **User Interface Design**: While the focus was on backend functionality, I would prioritize designing a more user-friendly interface for the final website. A well-designed UI can significantly enhance user engagement and experience.
4. **Documentation and Comments**: I would ensure that the code is better documented with comments explaining the purpose of each function and key steps. This would aid future developers (or myself) in understanding the codebase more quickly.
### Advice for Others
1. **Start Small**: Begin with a limited scope and gradually expand. This approach allows for manageable testing and debugging, making it easier to identify and fix issues.
2. **Prioritize Automation**: Invest time in automating repetitive tasks early in the project. This will save time in the long run and reduce the potential for human error.
3. **Stay Updated on Legal Guidelines**: Always be aware of the legal implications of using app data and screenshots. Familiarize yourself with the terms of service of the platforms you are working with to avoid potential issues.
4. **Iterate and Improve**: Be open to iterating on your design and functionality based on feedback and testing. Continuous improvement is key to developing a successful project.
5. **Engage with the Community**: Don’t hesitate to seek help or advice from developer communities. Platforms like Stack Overflow or GitHub can provide valuable insights and solutions to common problems.
By following these lessons and advice, others can navigate similar projects more effectively and efficiently.
## What's Next?
## Conclusion
As we wrap up this phase of the Color Palette Hunter project, we are excited to share our current status and future development plans. We have successfully outlined the core functionality, including app data acquisition, screenshot capture, color palette extraction, markdown generation, and website publishing. Our initial focus has been on gathering data from the top 100 apps, and we are currently in the process of implementing the data acquisition methods, with plans to automate screenshot capturing and color extraction soon.
Looking ahead, our development plans include refining our automation processes, enhancing the accuracy of our color palette extraction, and expanding our app database. We aim to implement a robust search functionality on our website, allowing users to explore color palettes by app names, colors, or tags. Additionally, we envision creating a community-driven platform where contributors can share their insights and suggestions, further enriching the project.
We invite all contributors—designers, developers, and color enthusiasts—to join us on this journey. Your expertise and creativity can help us enhance the project, whether through code contributions, sharing app suggestions, or providing feedback on our color analysis. Together, we can build a comprehensive resource that not only showcases color palettes but also inspires innovative design choices.
Reflecting on this side project journey, we are grateful for the learning experiences and the collaborative spirit that has emerged. Color Palette Hunter is not just about extracting colors; it’s about understanding the impact of color in user interfaces and fostering a community of like-minded individuals passionate about design. We look forward to the next steps and hope you will join us in making Color Palette Hunter a valuable tool for designers everywhere. Let’s create something beautiful together!
## Project Development Analytics
### timeline gant
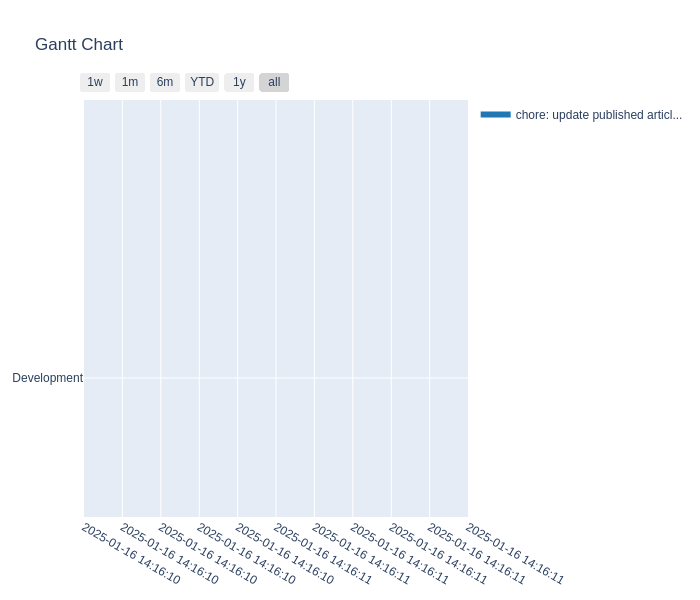
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
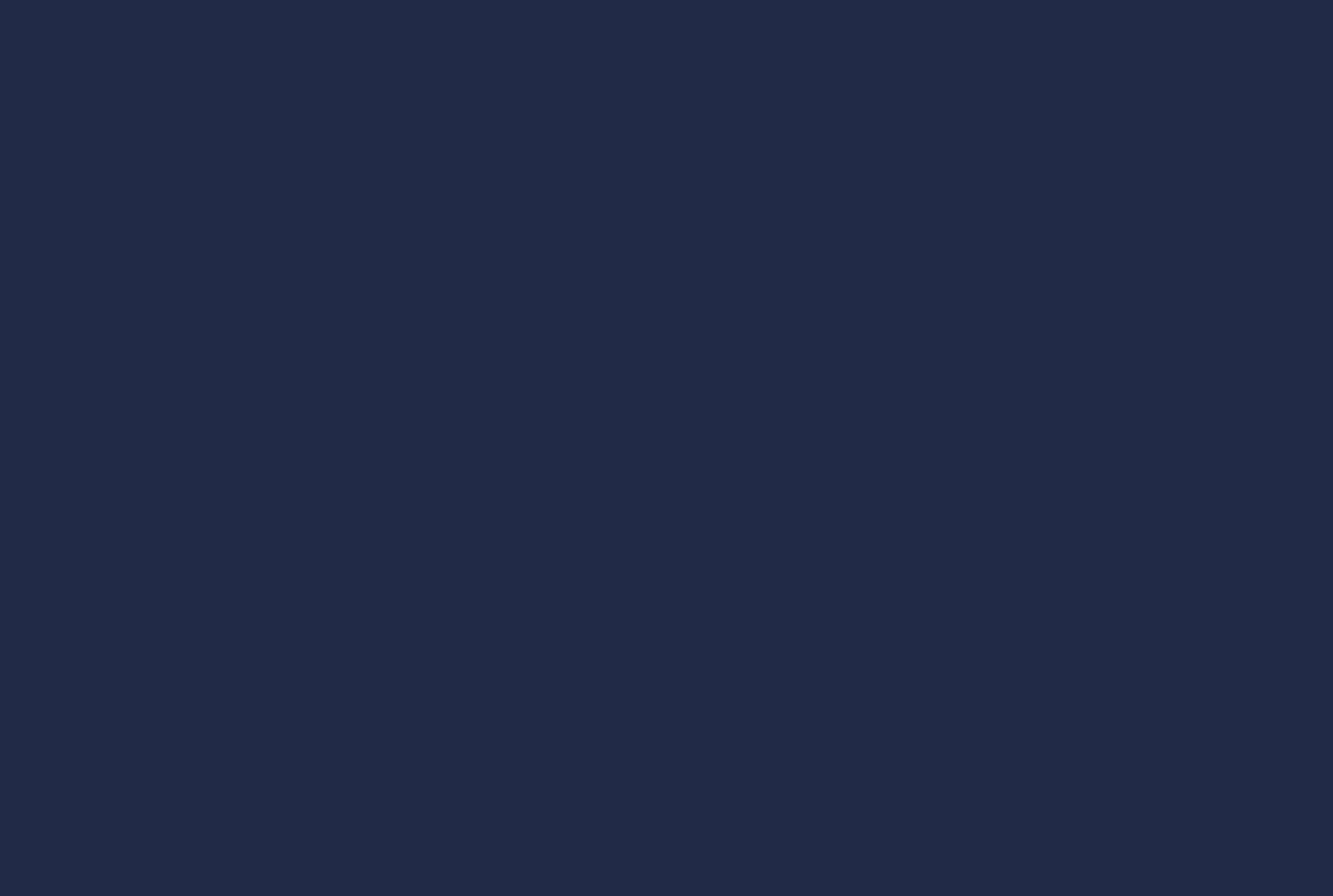
### Commit Activity Patterns
This chart shows when commits typically happen:
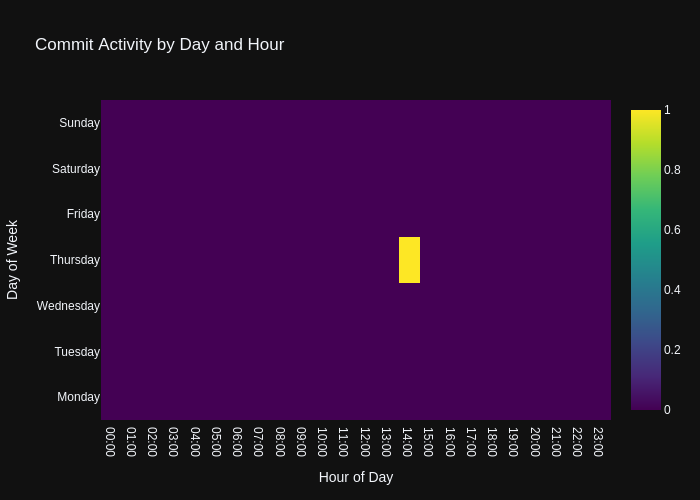
### Code Frequency
This chart shows the frequency of code changes over time:
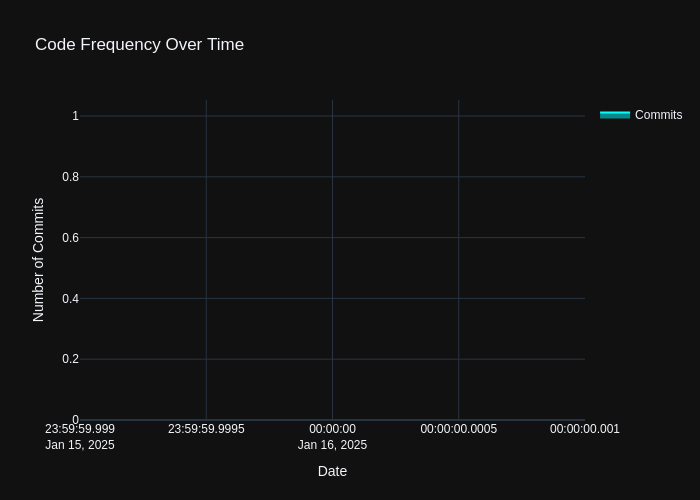
* Repository URL: [https://github.com/wanghaisheng/color-palette-hunter](https://github.com/wanghaisheng/color-palette-hunter)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2025 年 1 月 20 日