Building Mood Island: A Developer's Journey into Emotional Gaming
Heisenberg
2024 年 12 月 30 日
No description provided.
Built by wanghaisheng | Last updated: 20241230
10 minutes 55 seconds read
Project Genesis
Welcome to Emotional Adventure: Mood Island Journey! I’m thrilled to share this unique, interactive game with you, born from a spark of inspiration during a particularly challenging time in my life. Like many of us, I’ve faced days when the clouds of sadness seemed to linger a little too long, and I found myself yearning for a way to lift my spirits and reconnect with my emotions. That’s when the idea of Mood Island was born—a whimsical escape where we can explore our feelings and find joy, even on the bluest of days.
As I embarked on this creative journey, I was driven by a personal motivation: to create a space where anyone could navigate their emotional landscape with ease and playfulness. I wanted to transform the often daunting task of understanding our moods into an engaging adventure. However, the path wasn’t without its challenges. I grappled with how to represent complex emotions in a way that felt accessible and fun. How could I design experiences that not only entertained but also encouraged reflection and growth?
After countless brainstorming sessions and a few late nights, I found my solution: five distinct Emotion Islands, each embodying a different mood. By allowing players to choose their Mood Weather at the start of their journey, I created a framework that guides them to islands filled with fun, reflective challenges tailored to their emotional state. Whether you’re seeking joy, relaxation, or a little inspiration, Mood Island is here to cheer you up and help you embrace the full spectrum of your feelings. So, let’s set sail on this emotional adventure together!
From Idea to Implementation
1. Initial Research and Planning
The journey of creating Emotional Adventure: Mood Island Journey began with extensive research into emotional well-being and gamification. The goal was to design a game that not only entertains but also serves as a tool for emotional exploration and self-reflection.
We started by reviewing existing literature on emotional intelligence, therapeutic games, and the psychological benefits of play. This research highlighted the importance of creating a safe space for players to engage with their emotions. We also analyzed successful games in the wellness genre, identifying key features that resonated with users, such as interactive storytelling, engaging visuals, and meaningful choices.
Based on our findings, we outlined the core objectives of the game: to provide players with a fun and engaging way to explore their emotions, to offer reflective challenges that promote self-awareness, and to create a supportive environment for emotional growth. This led to the conceptualization of the five Emotion Islands, each representing a distinct mood, and the idea of using Mood Weather as a guiding mechanism for players.
2. Technical Decisions and Their Rationale
With a clear vision in place, we moved on to the technical aspects of development. We chose to build the game using Unity, a versatile game engine that allows for rich graphics and interactive gameplay. Unity’s support for both 2D and 3D environments made it an ideal choice for creating the vibrant, magical world of Mood Island.
For the user interface, we opted for a clean and intuitive design, ensuring that players could easily navigate through the game. We implemented a modular architecture, allowing for easy updates and the addition of new content in the future. This decision was driven by the desire to keep the game fresh and engaging over time.
Additionally, we integrated a simple analytics system to track player choices and emotional responses. This data would help us understand player behavior and refine the game experience based on user feedback.
3. Alternative Approaches Considered
During the planning phase, we considered several alternative approaches. One option was to create a more linear narrative-driven game, where players would follow a set storyline. However, we ultimately decided against this in favor of a more open-ended exploration model. This choice was influenced by our research, which indicated that players benefit from having the freedom to choose their emotional journey.
We also explored the idea of incorporating multiplayer elements, allowing players to share their experiences with friends. While this could enhance social interaction, we concluded that the primary focus should be on individual emotional exploration. We wanted to create a personal space for reflection, which could be compromised in a multiplayer setting.
4. Key Insights That Shaped the Project
Several key insights emerged throughout the development process that significantly shaped the project. One of the most important was the realization that emotional exploration is deeply personal and varies from person to person. This understanding reinforced our decision to allow players to choose their Mood Weather and navigate the islands based on their current emotional state.
Another insight was the importance of balance between fun and reflection. While we wanted to create engaging challenges, we also recognized the need for moments of introspection. This led to the inclusion of thought-provoking questions and activities that encourage players to pause and reflect on their feelings.
Finally, user testing played a crucial role in shaping the final product. Feedback from early testers highlighted the need for clearer instructions and more diverse activities. We iterated on the game design based on this feedback, ensuring that the final version was both enjoyable and effective in promoting emotional well-being.
In conclusion, the journey from concept to code for Emotional Adventure: Mood Island Journey was marked by thoughtful research, strategic technical decisions, and a commitment to creating a meaningful experience for players. The result is a game that not only entertains but also empowers individuals to explore and balance their emotions in a supportive and engaging environment.
Under the Hood
Technical Deep-Dive: Emotional Adventure - Mood Island Journey
1. Architecture Decisions
The architecture of Emotional Adventure: Mood Island Journey is designed to be modular and scalable, allowing for easy updates and the addition of new features. The game is structured around a client-server model, where the client handles user interactions and the server manages game logic, data storage, and user sessions.
Key Components:
- Client-Side (Frontend): Built using a JavaScript framework (e.g., React or Vue.js) to create a responsive and interactive user interface. The client communicates with the server via RESTful APIs.
- Server-Side (Backend): Developed using Node.js with Express.js to handle API requests, manage user sessions, and serve game data.
- Database: A NoSQL database (e.g., MongoDB) is used to store user profiles, game progress, and emotional resources, allowing for flexible data structures.
Example Architecture Diagram:
+-------------------+ +-------------------+
| Client (UI) | <--> | Server (API) |
| (React/Vue.js) | | (Node.js/Express)|
+-------------------+ +-------------------+
|
v
+-------------------+
| Database |
| (MongoDB) |
+-------------------+
2. Key Technologies Used
-
Frontend:
- React: For building the user interface, allowing for component-based architecture and state management.
- Redux: For managing application state, especially useful for handling user mood selections and game progress.
-
Backend:
- Node.js: For server-side JavaScript execution, enabling asynchronous processing and real-time capabilities.
- Express.js: For building RESTful APIs to handle client requests and serve game data.
-
Database:
- MongoDB: For storing user data, game states, and emotional resources in a flexible schema.
-
Deployment:
- Docker: For containerizing the application, ensuring consistent environments across development and production.
- Heroku/AWS: For hosting the application, providing scalability and reliability.
3. Interesting Implementation Details
Mood Weather Selection
The game starts with a mood weather selection, which influences the gameplay experience. This is implemented using a simple form component in React:
import React, { useState } from 'react';
const MoodSelector = ({ onSelectMood }) => {
const [mood, setMood] = useState('');
const handleMoodChange = (event) => {
setMood(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
onSelectMood(mood);
};
return (
<form onSubmit={handleSubmit}>
<label>
Select your Mood Weather:
<select value={mood} onChange={handleMoodChange}>
<option value="happy">Happy</option>
<option value="sad">Sad</option>
<option value="anxious">Anxious</option>
<option value="relaxed">Relaxed</option>
</select>
</label>
<button type="submit">Start Adventure</button>
</form>
);
};
Golden Eggs and Emotional Gems
The game features collectible items like Golden Eggs and Emotional Gems, which are stored in the user’s profile in the database. The following code snippet demonstrates how to update the user’s inventory when they collect an item:
const collectItem = async (userId, itemType) => {
const response = await fetch(`/api/users/${userId}/collect`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ itemType }),
});
if (response.ok) {
const updatedUser = await response.json();
console.log('Updated User Inventory:', updatedUser.inventory);
} else {
console.error('Failed to collect item');
}
};
4. Technical Challenges Overcome
User Session Management
One of the challenges was managing user sessions effectively, especially with multiple concurrent users. This was addressed by implementing JWT (JSON Web Tokens) for authentication, allowing users to securely log in and maintain their session across different devices.
Real-Time Updates
To provide a seamless experience, real-time updates were necessary for user interactions. This was achieved using WebSockets, allowing the server to push updates to the client without requiring the user to refresh the page.
Example of WebSocket Implementation:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
// Broadcast the
## Lessons from the Trenches
### Key Technical Lessons Learned
1. **User Experience Design**: Creating an engaging user interface that resonates with the emotional theme was crucial. We learned the importance of intuitive navigation and visually appealing graphics to enhance user engagement.
2. **Emotion Recognition**: Implementing a system to gauge users' moods effectively was a challenge. We discovered that using simple questionnaires and mood sliders can provide valuable insights without overwhelming users.
3. **Game Mechanics**: Balancing fun and educational elements was key. We learned that incorporating gamification techniques, such as rewards and challenges, can motivate users to engage more deeply with the emotional content.
4. **Feedback Loops**: Establishing a feedback mechanism to gather user input on their experiences helped us iterate and improve the game. We learned that continuous user feedback is essential for refining features and enhancing overall satisfaction.
### What Worked Well
1. **Diverse Activities**: The variety of activities across the Emotion Islands kept users engaged. Users appreciated the mix of reflective questions, mini-games, and creative tasks that catered to different emotional needs.
2. **Visual and Audio Elements**: The use of calming visuals and soothing soundtracks contributed to a positive user experience. Many users reported feeling more relaxed and centered after playing.
3. **Community Engagement**: Building a community around the game through forums and social media allowed users to share their experiences and support each other, enhancing the overall impact of the game.
4. **Progress Tracking**: Implementing a system to track users' emotional journeys and achievements helped them see their growth over time, which was motivating and rewarding.
### What You'd Do Differently
1. **More Personalization**: While we offered some customization options, we would focus on enhancing personalization features, allowing users to tailor their experiences based on their specific emotional needs and preferences.
2. **Expanded Content**: We would invest more time in creating additional content and challenges for each Emotion Island to keep the game fresh and engaging for returning users.
3. **Accessibility Features**: We realized the importance of making the game accessible to a wider audience. In future iterations, we would prioritize features for users with disabilities, such as text-to-speech and adjustable font sizes.
4. **Data Privacy**: We would implement more robust data privacy measures to ensure users feel safe sharing their emotions and experiences within the game.
### Advice for Others
1. **Focus on User-Centric Design**: Always prioritize the user experience. Conduct user testing early and often to gather feedback and make necessary adjustments.
2. **Iterate Based on Feedback**: Be open to change and willing to iterate on your design based on user feedback. Continuous improvement is key to creating a successful product.
3. **Balance Fun and Purpose**: Strive to create a balance between entertainment and educational value. Users should feel engaged while also gaining insights into their emotions.
4. **Build a Community**: Foster a sense of community among users. Encourage sharing and support, as this can enhance the emotional impact of your project and create a loyal user base.
## What's Next?
## Conclusion: The Future of Mood Island
As we stand at the current project status of **Emotional Adventure: Mood Island Journey**, we are thrilled to share that the initial development phase has been successfully completed. The game is now fully functional, featuring five distinct Emotion Islands, each designed to engage players in meaningful ways. Users can select their **Mood Weather** and embark on a journey filled with reflective challenges, emotional tools, and opportunities for personal growth. The feedback we’ve received so far has been overwhelmingly positive, affirming our mission to inspire and uplift those who may be feeling blue.
Looking ahead, we have exciting plans for future development. Our next steps include expanding the game with additional islands, introducing new activities, and enhancing the emotional tools available to players. We aim to incorporate user-generated content, allowing players to share their own experiences and insights, further enriching the community aspect of Mood Island. Additionally, we are exploring partnerships with mental health professionals to ensure that our content remains both engaging and beneficial.
We invite all contributors—whether you’re a developer, artist, writer, or simply someone passionate about mental well-being—to join us on this journey. Your creativity and insights can help shape the future of Mood Island, making it an even more powerful tool for emotional exploration. Together, we can create a vibrant community that supports and uplifts one another through shared experiences and collective growth.
As we reflect on this side project journey, we are filled with gratitude for the support and enthusiasm we’ve encountered. Mood Island is not just a game; it’s a movement towards emotional awareness and balance. We believe that by fostering a space where individuals can explore their feelings, we can contribute to a more compassionate and understanding world. Let’s continue this adventure together, inspiring each other to embrace our emotions and find joy in the journey. The islands await—let’s set sail!
## Project Development Analytics
### timeline gant
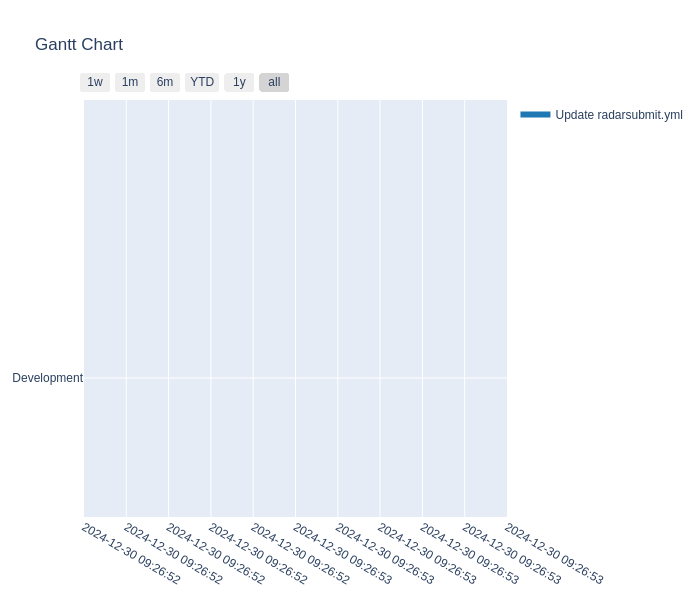
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
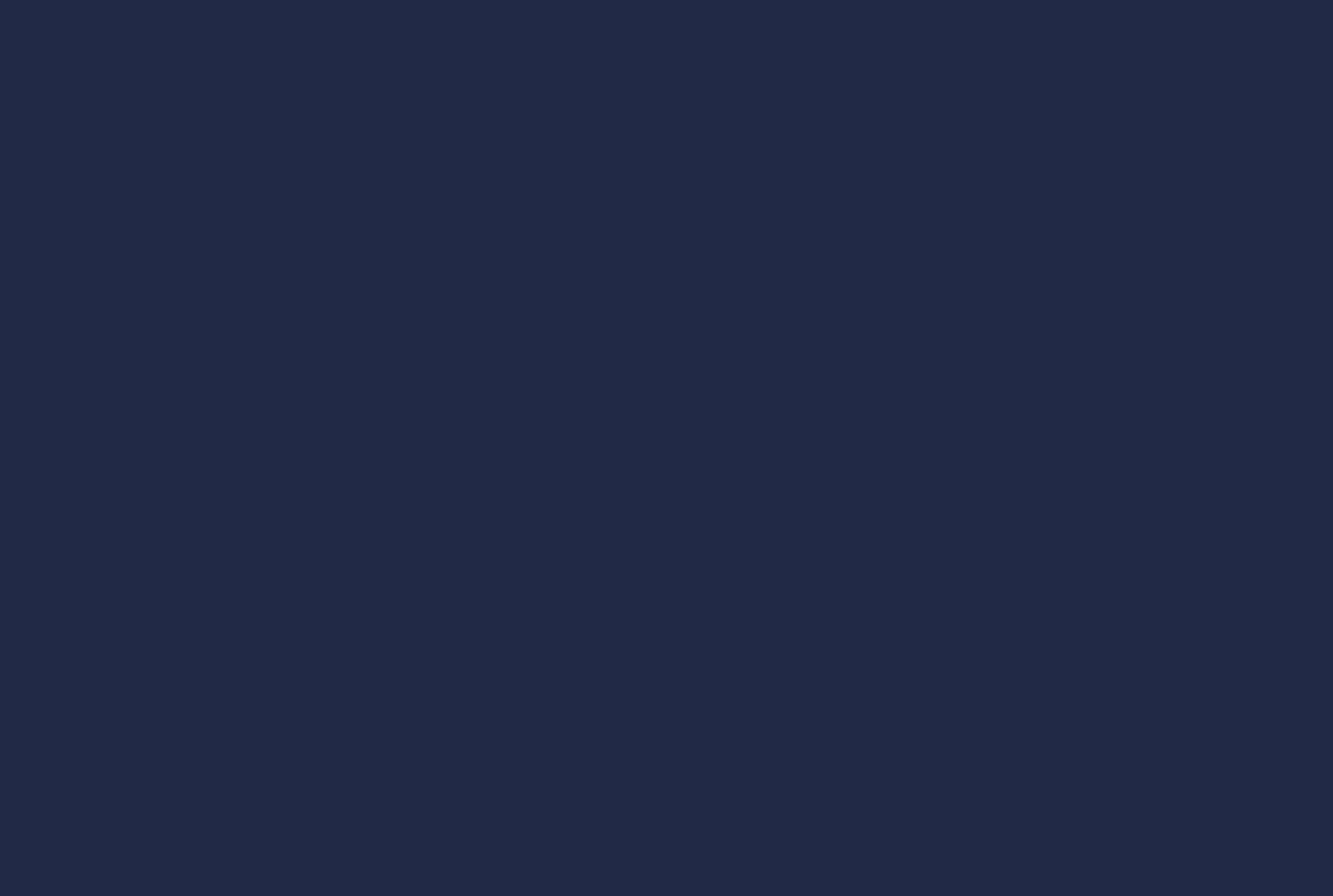
### Commit Activity Patterns
This chart shows when commits typically happen:
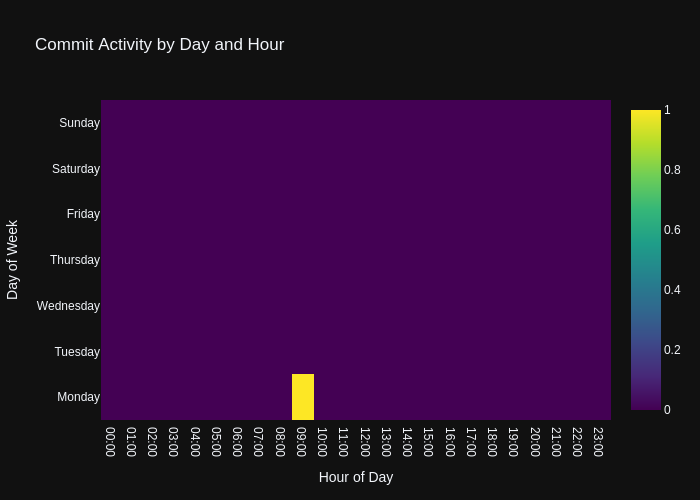
### Code Frequency
This chart shows the frequency of code changes over time:
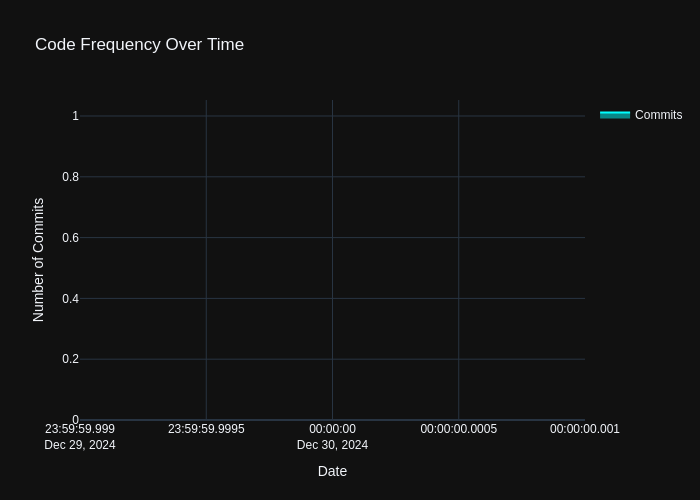
* Repository URL: [https://github.com/wanghaisheng/color-of-the-mood](https://github.com/wanghaisheng/color-of-the-mood)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日