Building Mood Island: A Color-Mixing Adventure in Emotional Gaming
Project Genesis
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Under the Hood
Technical Deep-Dive: Emotional Adventure - Mood Island Journey
1. Architecture Decisions
Key Architectural Components:
- Client-Side (Frontend): Built using a modern JavaScript framework (e.g., React or Vue.js) to create a dynamic and responsive user interface. The frontend communicates with the backend via RESTful APIs.
- Server-Side (Backend): Developed using Node.js with Express.js to handle API requests, manage game state, and process user data. The server also integrates with a database for persistent storage.
- Database: A NoSQL database (e.g., MongoDB) is used to store user profiles, game progress, and emotional data, allowing for flexible data structures and easy scalability.
2. Key Technologies Used
-
Frontend:
- React: For building the user interface, enabling component-based architecture and efficient state management.
- Redux: For managing application state, particularly useful for handling user sessions and game state across different components.
- CSS Modules: For styling components, ensuring scoped styles and avoiding conflicts.
-
Backend:
- Node.js: For server-side JavaScript execution, allowing for non-blocking I/O operations and real-time capabilities.
- Express.js: For building RESTful APIs, simplifying routing and middleware management.
- MongoDB: For storing user data and game state, providing flexibility in data modeling.
-
Deployment:
- Docker: For containerizing the application, ensuring consistent environments across development and production.
- Heroku/AWS: For hosting the application, providing scalability and reliability.
3. Interesting Implementation Details
Mood Weather Selection
// MoodWeather.js
import React, { useState } from 'react';
const MoodWeather = ({ onSelect }) => {
const [selectedMood, setSelectedMood] = useState('');
const handleMoodChange = (mood) => {
setSelectedMood(mood);
onSelect(mood);
};
return (
<div>
<h2>Select Your Mood Weather</h2>
<button onClick={() => handleMoodChange('Joy')}>Joy</button>
<button onClick={() => handleMoodChange('Sadness')}>Sadness</button>
<button onClick={() => handleMoodChange('Relaxation')}>Relaxation</button>
</div>
);
};
export default MoodWeather;
Golden Eggs and Emotional Gems
// inventoryController.js
const updateInventory = async (userId, item) => {
const user = await User.findById(userId);
user.inventory.push(item);
await user.save();
return user.inventory;
};
4. Technical Challenges Overcome
User Session Management
// authMiddleware.js
const jwt = require('jsonwebtoken');
const authenticateToken = (req, res, next) => {
const token = req.headers['authorization'];
if (!token) return res.sendStatus(401);
jwt.verify(token, process.env.ACCESS_TOKEN_SECRET, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
};
Real-Time Updates
// server.js
const WebSocket = require('ws');
const wss = new WebSocket.Server({ server });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
// Handle incoming messages
});
// Send updates to the client
ws.send(JSON.stringify({ event: 'itemCollected', item: 'Golden Egg' }));
});
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Game Design Principles: Understanding the importance of user experience (UX) in game design was crucial. Balancing engaging gameplay with emotional reflection required careful consideration of mechanics and narrative flow.
-
Emotional Engagement: Incorporating emotional intelligence into game mechanics was a significant learning point. Designing challenges that resonate with players’ feelings helped create a deeper connection to the game.
-
Feedback Loops: Implementing feedback mechanisms (like collecting Emotional Gems) reinforced positive behavior and encouraged players to engage with the content more meaningfully.
-
Iterative Development: The importance of playtesting and gathering user feedback early in the development process was highlighted. Iterating based on player experiences led to improvements in gameplay and emotional impact.
What Worked Well
-
Diverse Activities: The variety of challenges across the Emotion Islands kept players engaged. Activities ranged from puzzles to reflective journaling, catering to different preferences and emotional needs.
-
Visual and Audio Design: The aesthetic choices, including vibrant visuals and calming soundscapes, effectively created an immersive environment that enhanced emotional engagement.
-
Mood Weather Feature: Allowing players to choose their Mood Weather at the start of the game provided a personalized experience, making it easier for them to connect with the content.
-
Community Feedback: Engaging with a community of players during development helped refine the game. Their insights led to valuable adjustments that improved overall enjoyment and emotional resonance.
What You’d Do Differently
-
More Comprehensive Testing: While playtesting was conducted, a more extensive beta testing phase could have provided additional insights into user experience and emotional impact.
-
Accessibility Features: Incorporating more accessibility options from the beginning would have made the game more inclusive. Features like text-to-speech, colorblind modes, and adjustable difficulty levels should be prioritized.
-
Emotional Support Resources: Providing players with additional resources for emotional support outside the game could enhance the experience. This could include links to mental health resources or community forums.
-
Longer Development Timeline: Allowing more time for development would have enabled deeper exploration of emotional themes and more polished gameplay mechanics.
Advice for Others
-
Prioritize Emotional Design: When creating games focused on emotions, prioritize emotional design principles. Understand your audience’s emotional needs and tailor experiences to resonate with them.
-
Engage with Your Audience: Involve potential players early in the development process. Their feedback can provide invaluable insights that shape the game’s direction and improve its impact.
-
Iterate and Adapt: Be open to change. Use feedback to iterate on your design and adapt to what works best for your audience. Flexibility can lead to a more successful final product.
-
Balance Fun and Reflection: Strive to create a balance between engaging gameplay and meaningful emotional reflection. Both elements are essential for a game that aims to inspire and uplift players.
What’s Next?
Conclusion: Looking Ahead in the Mood Island Journey
Project Development Analytics
timeline gant
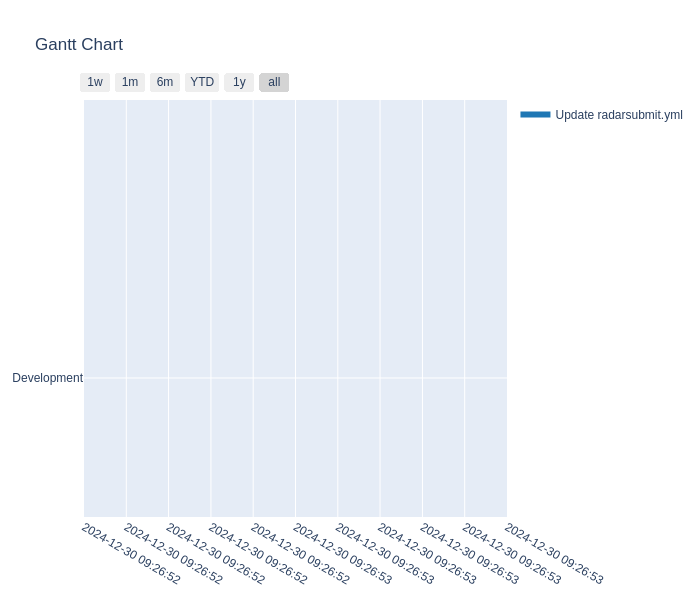
Commit Activity Heatmap
Contributor Network
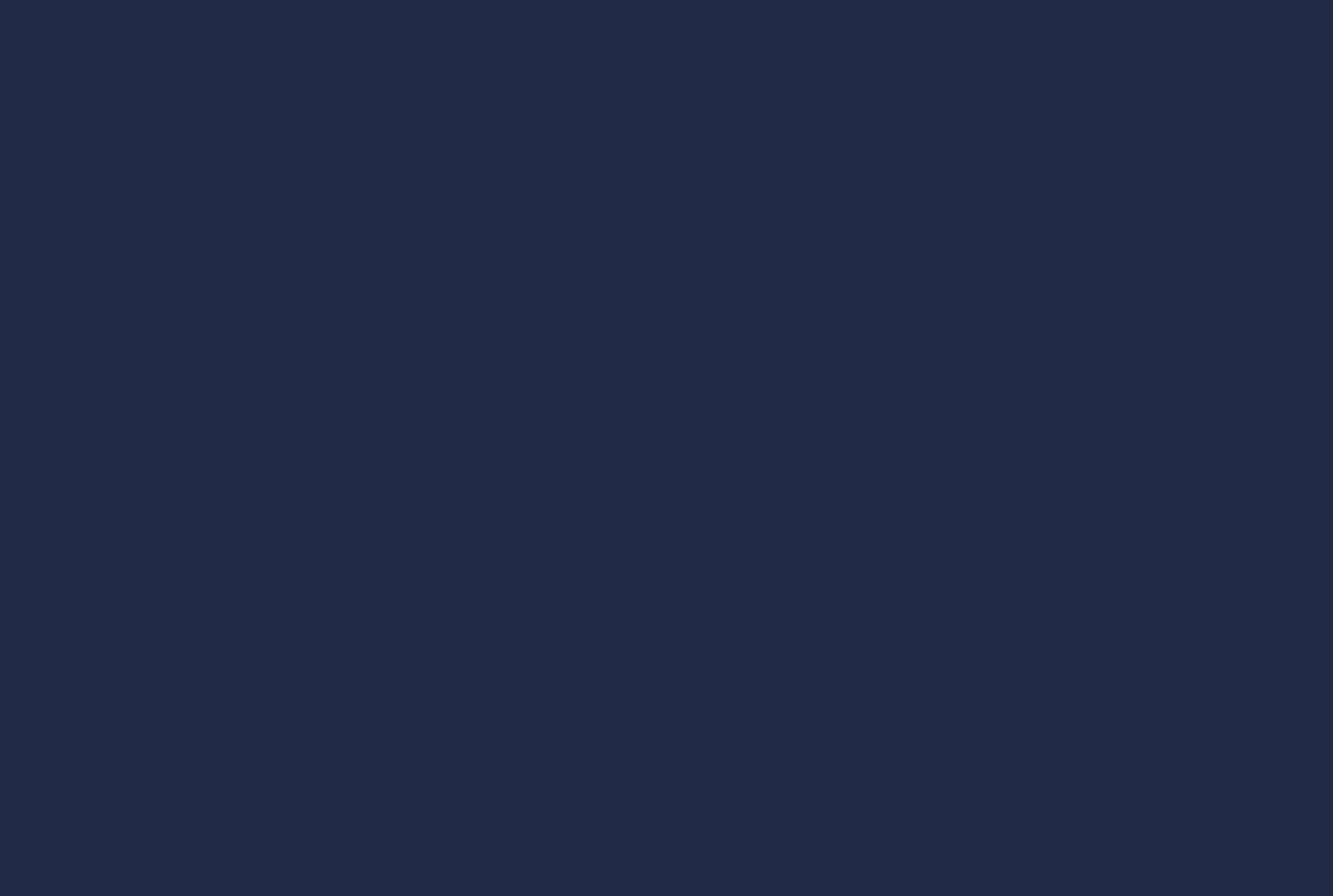
Commit Activity Patterns
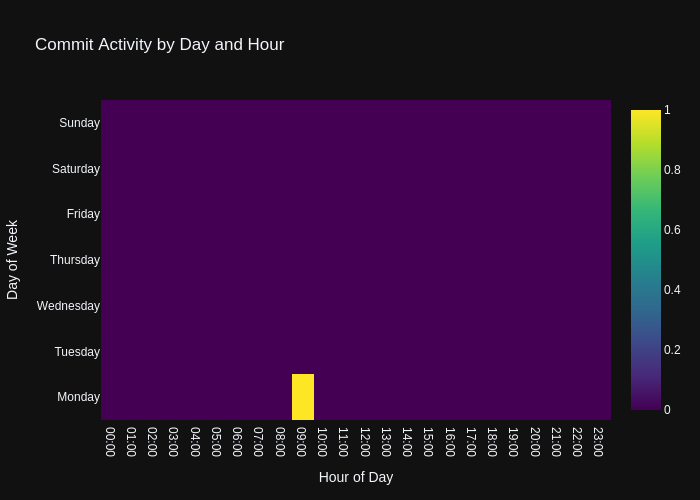
Code Frequency
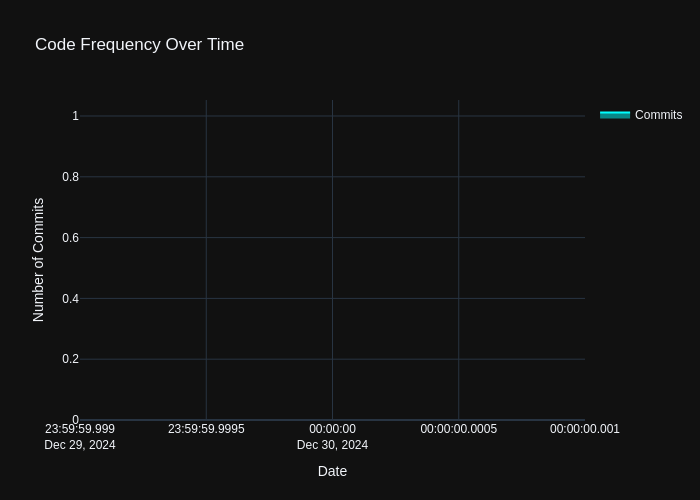
- Repository URL: https://github.com/wanghaisheng/color-mixer
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日