Building chimaster-quiz-to-go: A Developer's Journey to SEO Mastery
Project Genesis
Unleashing the Power of Chimaster Quiz to Go: My Journey to a Seamless Web Experience
From Idea to Implementation
1. Initial Research and Planning
- SEO Best Practices: Understanding the importance of sitemaps, metadata, and avoiding common pitfalls like Google redirection issues.
- Responsive Design: Ensuring that the site would be accessible and user-friendly across various devices, including PCs and mobile phones.
- Analytics Integration: The need for tracking user behavior through tools like Google Analytics and Microsoft Clarity to inform future improvements.
- Content Generation: Exploring automated solutions for generating blog content and images to maintain a dynamic and engaging site.
2. Technical Decisions and Their Rationale
- Framework Selection: A static site generator was chosen for its speed and simplicity. This decision was driven by the need for a lightweight solution that could easily integrate with SEO tools and PWA features.
- Sitemap Generation: Implementing an automated sitemap generator based on language subfolders was prioritized to enhance SEO and ensure that all pages were indexed correctly.
- SEO Checks: Integrating tools to automatically check for SEO compliance was essential to avoid issues that could hinder search engine visibility.
- IndexNow Integration: The decision to use IndexNow for submitting URLs to Google was made to streamline the indexing process and improve site visibility.
- Responsive Design: Utilizing CSS frameworks that support responsive design ensured that the site would adapt seamlessly to different screen sizes.
- Analytics and User Tracking: The integration of Google Analytics and Microsoft Clarity was crucial for gathering insights into user behavior and site performance.
3. Alternative Approaches Considered
- Dynamic vs. Static Sites: While a dynamic site could offer more flexibility in content management, the decision to go with a static site was based on performance and simplicity. Static sites are faster and easier to deploy, making them ideal for the project’s goals.
- Custom vs. Pre-built Solutions: There was a consideration of building custom solutions for SEO checks and sitemap generation. However, leveraging existing libraries and tools was deemed more efficient and reliable, allowing the team to focus on other critical aspects of the project.
- Different Analytics Tools: While Google Analytics was the primary choice, other analytics tools were evaluated. Ultimately, the decision was made to stick with Google Analytics due to its widespread use and robust feature set.
4. Key Insights That Shaped the Project
- User-Centric Design: The importance of designing with the user in mind became clear early on. Ensuring that the site was responsive and easy to navigate was paramount to retaining visitors and improving engagement.
- SEO as a Continuous Process: SEO is not a one-time task but an ongoing process. This realization led to the integration of automated checks and tools to continuously monitor and improve the site’s SEO performance.
- Automation for Efficiency: The value of automation in content generation and SEO checks was a significant insight. By automating these processes, the team could focus on higher-level strategic decisions rather than getting bogged down in repetitive tasks.
- Collaboration and Open Source: Engaging with the open-source community and utilizing existing tools and frameworks fostered collaboration and innovation, ultimately leading to a more robust final product.
Under the Hood
Technical Deep-Dive: WebSim Site Building
1. Architecture Decisions
- Frontend: A responsive design that adapts to both PC and mobile devices, ensuring a seamless user experience across platforms.
- Backend: A set of automated scripts and tools that handle tasks such as sitemap generation, SEO checks, and URL submissions.
- Integration Layer: Interfaces with external services like Google Analytics, Microsoft Clarity, and image generation APIs.
2. Key Technologies Used
- HTML/CSS/JavaScript: For building the responsive frontend.
- Node.js: For backend scripting and automation tasks.
- GitHub Actions: For continuous integration and deployment, particularly for static site deployment.
- Google APIs: For SEO checks and URL submissions.
- Cloudflare Workers: For user management and serverless functions.
- PWA (Progressive Web App): To enhance user engagement and performance.
Example: Sitemap Generation
const fs = require('fs');
const path = require('path');
function generateSitemap(langSubfolders) {
let sitemap = '<?xml version="1.0" encoding="UTF-8"?>\n';
sitemap += '<urlset xmlns="http://www.sitemaps.org/schemas/sitemap-image.v1">\n';
langSubfolders.forEach(folder => {
const files = fs.readdirSync(path.join(__dirname, folder));
files.forEach(file => {
if (file.endsWith('.html')) {
sitemap += ` <url>\n <loc>https://example.com/${folder}/${file}</loc>\n </url>\n`;
}
});
});
sitemap += '</urlset>';
fs.writeFileSync('sitemap.xml', sitemap);
}
3. Interesting Implementation Details
SEO Checks
- Missing meta tags
- Proper use of header tags
- Avoiding Google redirection issues
function checkSEORequirements(htmlContent) {
const missingMetaTags = [];
if (!htmlContent.includes('<meta name="description"')) {
missingMetaTags.push('description');
}
if (!htmlContent.includes('<meta name="keywords"')) {
missingMetaTags.push('keywords');
}
return missingMetaTags;
}
Image Generation
async function generateImage(type) {
const response = await fetch(`https://api.imagegen.com/generate?type=${type}`);
const imageBlob = await response.blob();
const imageUrl = URL.createObjectURL(imageBlob);
return imageUrl;
}
4. Technical Challenges Overcome
Handling Multiple Languages
Integration with External APIs
Deployment Automation
name: Deploy to GitHub Pages
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build site
run: npm run build
- name: Deploy to GitHub Pages
uses: peaceiris/actions-gh-pages@v3
with:
github_token: ${{ secrets.GITHUB_TOKEN }}
publish_dir: ./public
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Automation is Key: Automating tasks such as sitemap generation, SEO checks, and URL submissions significantly reduces manual effort and minimizes human error. Implementing tools like Websim for site building can streamline the process.
-
SEO Best Practices: Understanding and implementing SEO requirements early in the project helps avoid common pitfalls like Google redirection issues and indexing problems. Regular checks and updates are essential.
-
Responsive Design: Ensuring that the site is responsive for both PC and mobile users is crucial. This requires thorough testing across different devices and screen sizes.
-
Integration of Analytics: Incorporating tools like Google Analytics and Microsoft Clarity from the start provides valuable insights into user behavior and site performance, which can inform future improvements.
-
Progressive Web App (PWA) Support: Adding PWA support enhances user experience by allowing offline access and faster load times, which can improve engagement and retention.
What Worked Well
-
Sitemap Generation: The automatic generation of sitemaps based on language subfolders and HTML files was effective in improving site navigation and SEO.
-
SEO Checks: The automated checks for SEO requirements helped identify and rectify issues early, ensuring better visibility on search engines.
-
User Engagement Tools: Integrating Google Analytics and Microsoft Clarity provided actionable insights that helped in optimizing user experience.
-
Image Generation: Automating the generation of logos and covers saved time and ensured consistency in branding.
-
Blog Text Generation: Utilizing tools like G4F for blog content generation streamlined content creation, allowing for more frequent updates and engagement.
What You’d Do Differently
-
More Comprehensive Testing: While automation is beneficial, more extensive manual testing could help catch edge cases that automated processes might miss, especially in responsive design.
-
User Feedback Loop: Establishing a more structured feedback loop with users could provide insights into areas for improvement that analytics alone might not reveal.
-
Documentation: Improving documentation throughout the development process would help onboard new contributors more effectively and ensure that knowledge is retained.
-
Scalability Considerations: Planning for scalability from the outset would help accommodate future growth without significant rework.
-
Keyword Research Tools: While tools like SpyFu were mentioned, exploring additional keyword research tools could provide a more comprehensive understanding of market trends and user intent.
Advice for Others
-
Start with a Clear Plan: Define your project goals and requirements clearly before diving into development. This will help keep the project focused and aligned with user needs.
-
Leverage Automation: Use automation tools wherever possible to save time and reduce errors. This includes everything from site generation to SEO checks.
-
Prioritize SEO: Make SEO a priority from the beginning. Regularly audit your site for SEO compliance and stay updated on best practices.
-
Engage Users Early: Involve users in the development process through feedback and testing. Their insights can be invaluable in shaping the final product.
-
Stay Updated: The web development landscape is constantly evolving. Stay informed about new tools, technologies, and best practices to keep your project relevant and effective.
What’s Next?
Conclusion
Current Project Status
Future Development Plans
Call to Action for Contributors
Final Thoughts on the Side Project Journey
Project Development Analytics
timeline gant
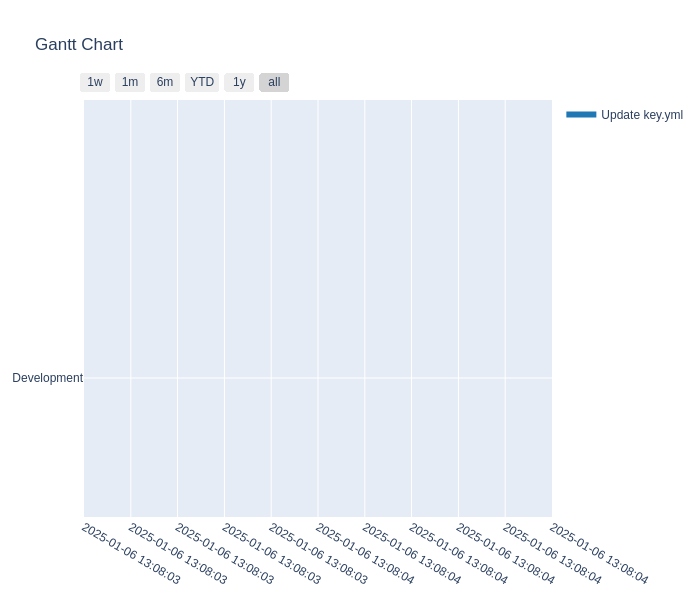
Commit Activity Heatmap
Contributor Network
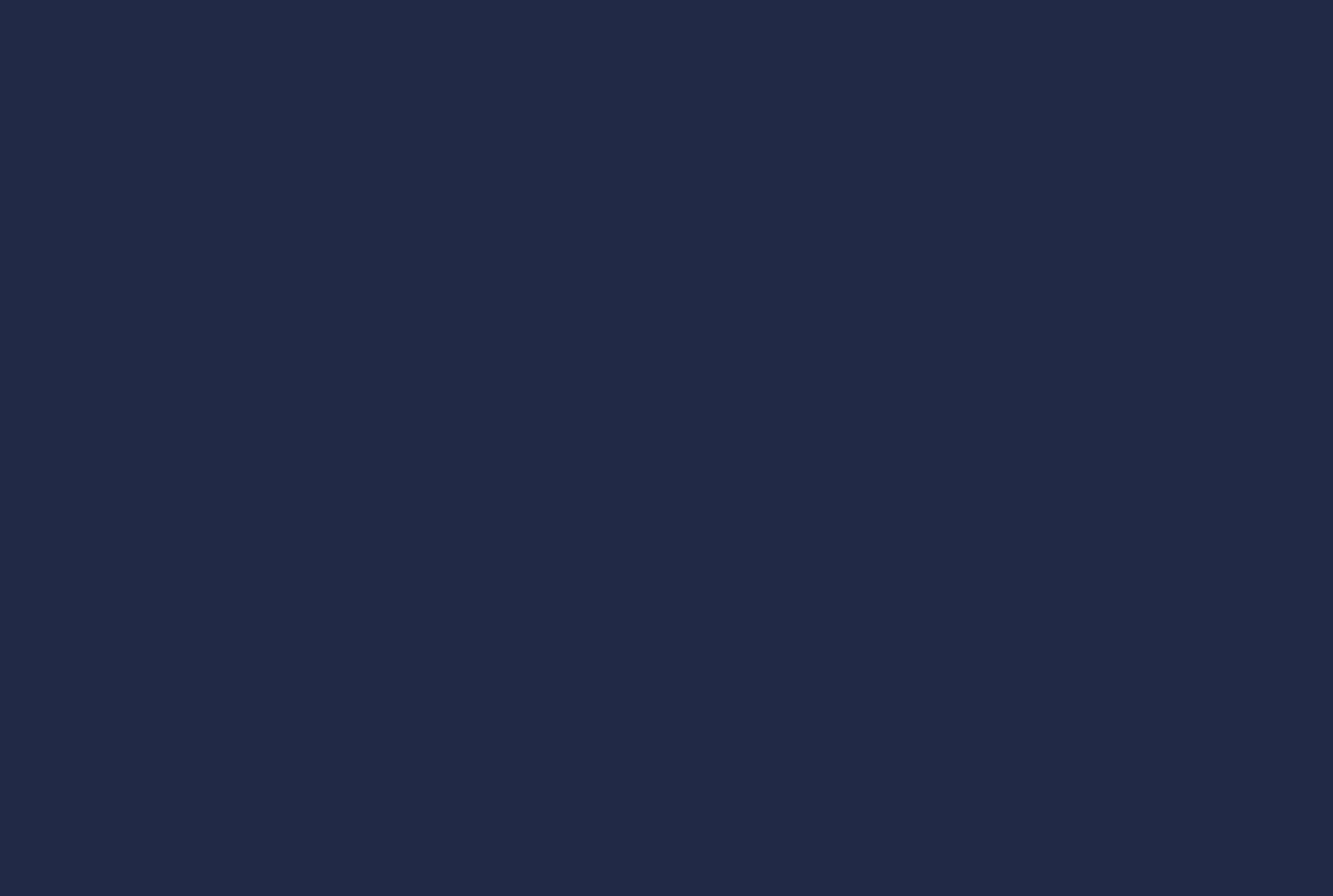
Commit Activity Patterns
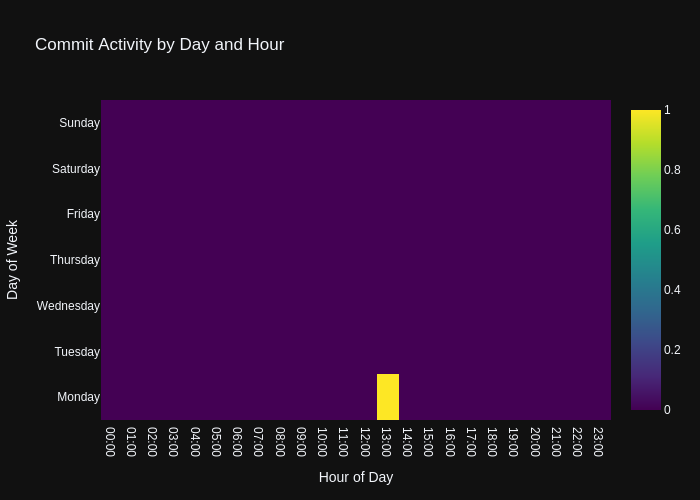
Code Frequency
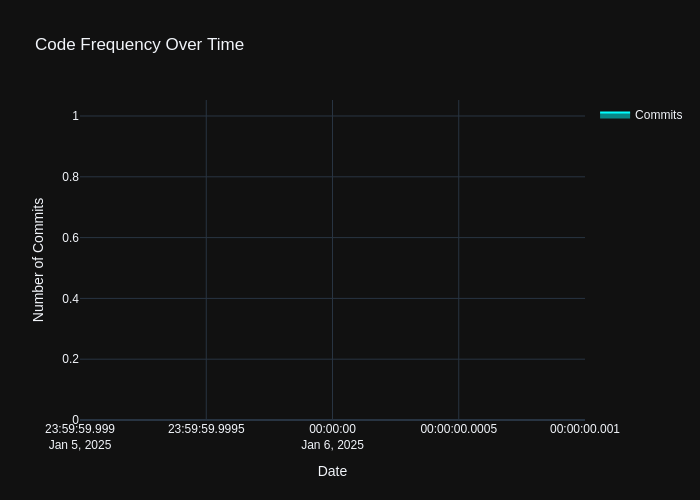
- Repository URL: https://github.com/wanghaisheng/chimaster-quiz-to-go
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 13 日