From Idea to Reality: Building CF Flux Remix with Cloudflare Workers
Project Genesis
Unleashing Creativity with CF Flux Remix: My Journey into Image Generation
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Choice of Cloudflare Workers: The decision to use Cloudflare Workers was driven by the need for a scalable, serverless architecture that could handle varying loads without the overhead of managing traditional servers. Cloudflare’s global network also ensures low latency for users around the world.
-
Utilization of Remix Framework: Remix was chosen for its ability to create fast, modern web applications with a focus on user experience. Its data loading and routing capabilities aligned well with our requirements for a responsive interface.
-
API-First Approach: By designing the application with an API-first mindset, we ensured that the backend could be easily integrated with various front-end applications and third-party services. This decision also facilitated the development of a user-friendly interface.
-
Environment Variable Management: To enhance security, we opted to manage sensitive information, such as API keys, through environment variables rather than hardcoding them into the application. This approach minimizes the risk of exposing sensitive data in the codebase.
3. Alternative Approaches Considered
-
Monolithic Architecture: Initially, we contemplated building a monolithic application that combined both the frontend and backend. However, this approach was quickly dismissed due to concerns about scalability and maintainability. The serverless architecture of Cloudflare Workers offered a more flexible solution.
-
Using Other Cloud Providers: While exploring options, we considered using other cloud providers like AWS or Google Cloud. However, the ease of deployment and integration with Cloudflare’s existing services made Cloudflare Workers the more attractive choice.
-
Different Frameworks: Other frameworks such as Next.js and Express were evaluated. Ultimately, Remix’s focus on data loading and its modern approach to routing made it the best fit for our needs.
4. Key Insights That Shaped the Project
-
User-Centric Design: Engaging with potential users early on highlighted the importance of a user-friendly interface. This feedback led to the implementation of features like prompt translation and optimization, which enhanced the overall user experience.
-
Flexibility and Extensibility: The realization that users might want to integrate the API into their applications prompted us to prioritize an extensible API design. This foresight allowed us to accommodate future enhancements and additional features without major overhauls.
-
Importance of Documentation: As the project evolved, it became clear that comprehensive documentation was crucial for user adoption. We invested time in creating clear, concise documentation to guide users through the setup and usage of the API, which ultimately contributed to a smoother onboarding experience.
-
Iterative Development: Embracing an iterative development approach allowed us to continuously refine the application based on user feedback and testing. This flexibility enabled us to adapt to changing requirements and improve the product incrementally.
Conclusion
Under the Hood
Technical Deep-Dive: CF Flux Remix Backend API
1. Architecture Decisions
-
Serverless Architecture: By utilizing Cloudflare Workers, the application benefits from a serverless architecture that allows for automatic scaling and reduced latency. This is particularly advantageous for image generation tasks that can be resource-intensive.
-
Microservices Approach: The API is structured to expose various endpoints for different functionalities, such as image generation and model retrieval. This microservices approach allows for easier maintenance and the ability to scale individual components independently.
-
Decoupled Frontend and Backend: The backend API is designed to be agnostic of the frontend, allowing for easy integration with various client applications. This separation of concerns enhances flexibility and enables the use of different technologies for the frontend.
2. Key Technologies Used
-
Cloudflare Workers: This serverless platform allows developers to run JavaScript code at the edge, providing low-latency responses to user requests. It is used to handle API requests and manage the image generation process.
-
Remix Framework: Remix is a modern web framework that focuses on performance and user experience. It is used to build the backend API, providing routing and data fetching capabilities.
-
PNPM: A fast, disk space-efficient package manager that is used for managing dependencies in the project. It is particularly useful for handling large projects with multiple dependencies.
-
JSON: The API communicates using JSON for request and response payloads, making it easy to integrate with various clients and services.
3. Interesting Implementation Details
API Endpoints
POST /api/image
{
"messages": [{"role": "user", "content": "图像描述"}],
"model": "模型ID",
"stream": false
}
{
"prompt": "原始提示词",
"translatedPrompt": "翻译后的提示词",
"image": "生成的图像数据(Base64编码)"
}
Environment Variables
wrangler.toml
file is used to define these variables:API_KEY = "your_cloudflare_api_key"
CF_ACCOUNT_LIST = '{"accounts": ["account1", "account2"]}'
CF_TRANSLATE_MODEL = "translate_model_id"
4. Technical Challenges Overcome
Handling API Rate Limits
async function fetchWithRetry(url, options, retries = 3) {
for (let i = 0; i < retries; i++) {
const response = await fetch(url, options);
if (response.ok) {
return response.json();
}
if (response.status === 429) { // Too Many Requests
await new Promise(resolve => setTimeout(resolve, 1000)); // Wait 1 second
} else {
throw new Error(`Request failed with status ${response.status}`);
}
}
throw new Error('Max retries reached');
}
Optimizing Image Generation
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Cloudflare Workers Integration: Understanding how to effectively utilize Cloudflare Workers for serverless functions was crucial. The deployment process and the interaction with Cloudflare’s API were key components that required careful attention to detail.
-
API Design: Designing a RESTful API that is intuitive and easy to use was a significant learning experience. Ensuring that the API endpoints were well-defined and that the request/response formats were consistent helped in creating a user-friendly interface.
-
Environment Management: Managing environment variables securely is essential. The importance of keeping sensitive information, such as API keys, out of the codebase and using Cloudflare’s binding feature was a critical lesson.
-
Error Handling: Implementing robust error handling and logging mechanisms in Cloudflare Workers helped in diagnosing issues quickly. Understanding how to read and interpret logs from Cloudflare was invaluable for troubleshooting.
What Worked Well
-
User-Friendly Interface: The design of the user interface was well-received. The responsive design and modern aesthetics made it easy for users to interact with the application.
-
One-Click Deployment: The one-click deployment feature streamlined the setup process for users, making it accessible even for those with limited technical knowledge.
-
Comprehensive Documentation: Providing detailed documentation, including examples and a video tutorial, significantly reduced the learning curve for new users and contributors.
-
Flexibility of Models: Supporting multiple image generation models allowed users to choose the best fit for their needs, enhancing the application’s versatility.
What You’d Do Differently
-
Enhanced Testing: Implementing more comprehensive testing, including unit tests and integration tests, would have helped catch issues earlier in the development process. Automated testing could ensure that new features do not break existing functionality.
-
User Feedback Loop: Establishing a more structured feedback loop with users could provide insights into their experiences and needs, leading to more user-centered improvements.
-
Performance Optimization: Focusing on performance optimization from the beginning could have improved the application’s responsiveness, especially under heavy load.
-
Version Control for API: Implementing version control for the API would allow for smoother transitions when introducing breaking changes, ensuring that existing users are not adversely affected.
Advice for Others
-
Prioritize Documentation: Invest time in creating clear and comprehensive documentation. This will save time for both users and contributors and can significantly improve the adoption of your project.
-
Focus on Security: Always prioritize security, especially when dealing with API keys and sensitive data. Use environment variables and secure storage solutions to protect this information.
-
Iterate Based on User Feedback: Regularly seek feedback from users and be willing to iterate on your design and features based on their input. This will help ensure that your application meets their needs.
-
Leverage Community Contributions: Encourage contributions from the community. Open-source projects thrive on collaboration, and welcoming pull requests can lead to innovative improvements and features.
-
Stay Updated with Technology: Keep abreast of updates and changes in the technologies you are using, such as Cloudflare Workers and Remix. This will help you leverage new features and maintain compatibility with the latest standards.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
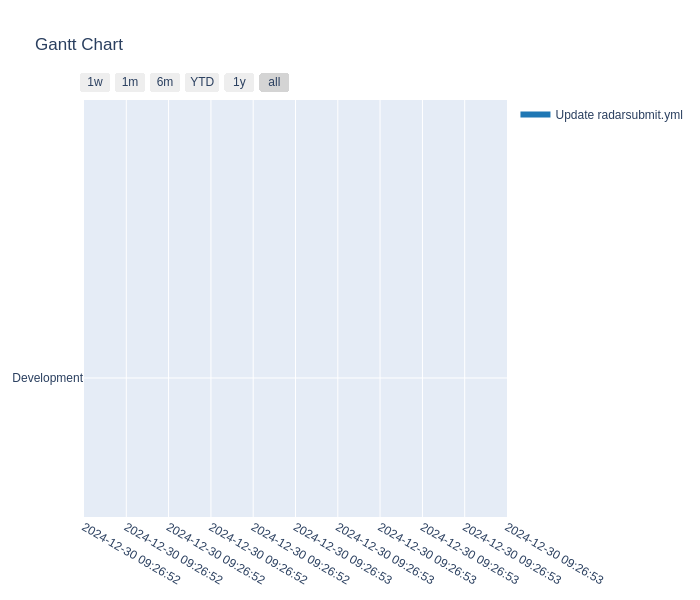
Commit Activity Heatmap
Contributor Network
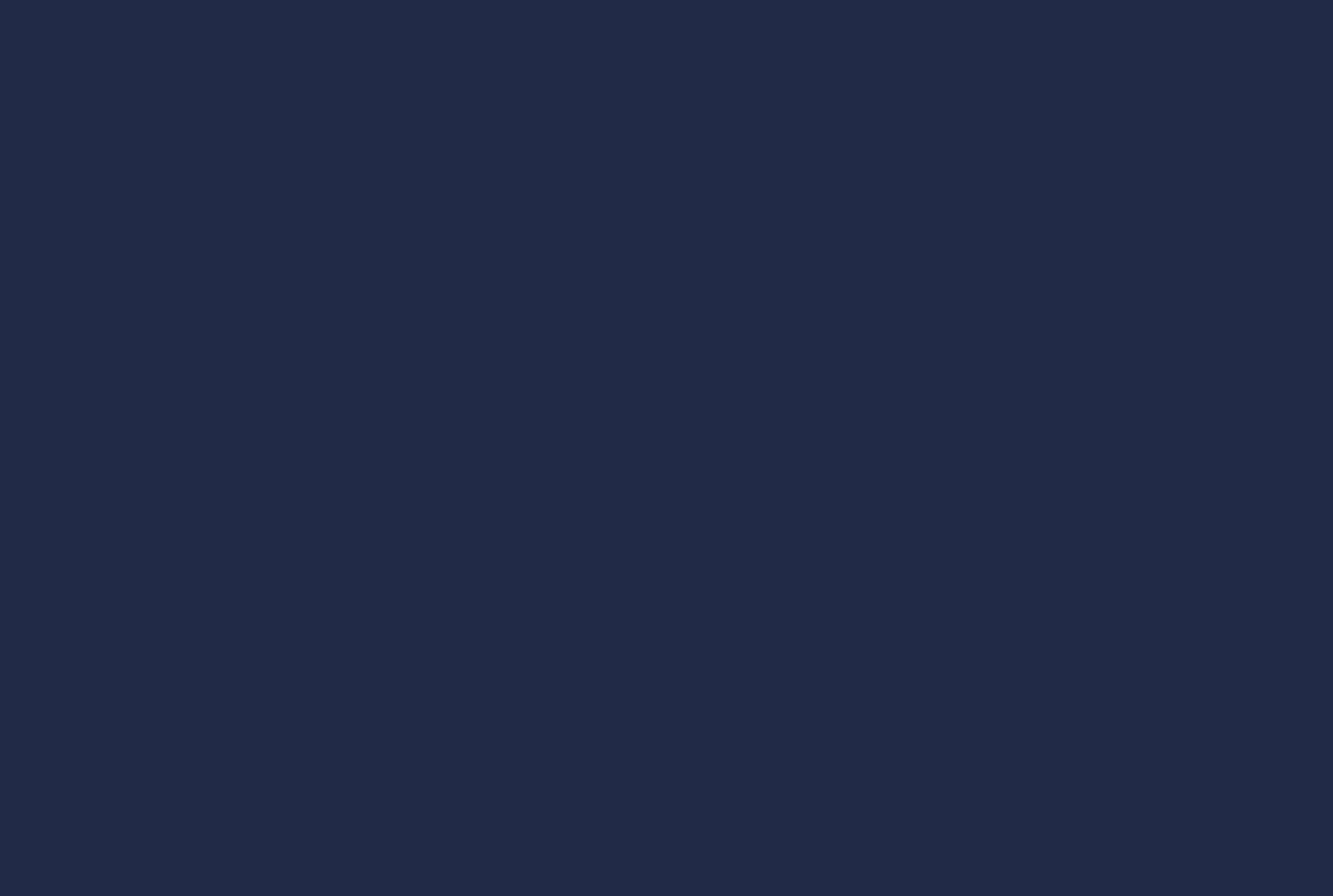
Commit Activity Patterns
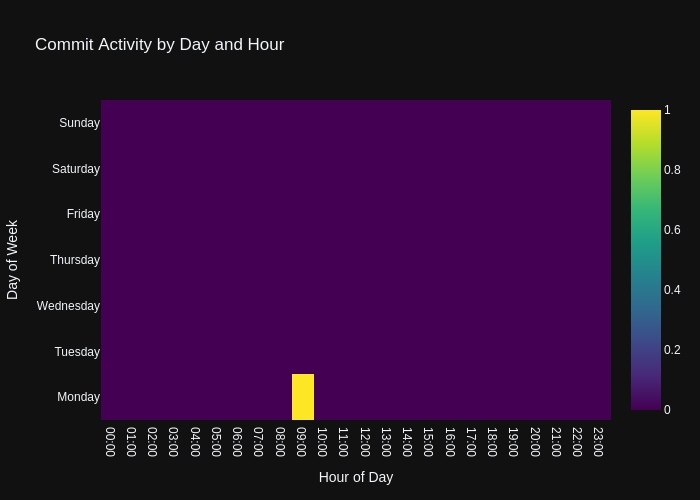
Code Frequency
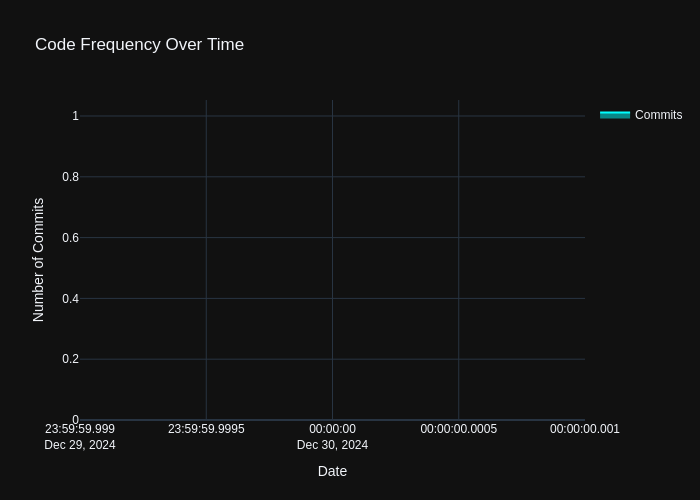
- Repository URL: https://github.com/wanghaisheng/cf-flux-remix
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日