Building Emotional Adventure: Crafting Mood Island with Interactive Gameplay
Project Genesis
From Idea to Implementation
Mood Island: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
Under the Hood
Technical Deep-Dive: Emotional Adventure - Mood Island Journey
1. Architecture Decisions
Key Components:
- Client-Side (Frontend): Built using a modern JavaScript framework (e.g., React or Vue.js) to create a responsive and interactive user interface. The client communicates with the server via RESTful APIs.
- Server-Side (Backend): Developed using Node.js with Express.js to handle API requests, manage user sessions, and serve game data. The server also integrates with a database to store user progress and emotional data.
- Database: A NoSQL database (e.g., MongoDB) is used to store user profiles, game states, and emotional metrics, allowing for flexible data structures and easy scalability.
Example Architecture Diagram:
[Client (React/Vue.js)] <--> [Server (Node.js/Express)] <--> [Database (MongoDB)]
2. Key Technologies Used
-
Frontend:
- React/Vue.js: For building a dynamic user interface that responds to user inputs and displays game content.
- CSS Framework (e.g., Tailwind CSS): For styling and ensuring a visually appealing design.
-
Backend:
- Node.js: For server-side JavaScript execution, enabling asynchronous processing and real-time capabilities.
- Express.js: For creating RESTful APIs to handle client requests and manage routing.
-
Database:
- MongoDB: For storing user data, game states, and emotional metrics in a flexible schema.
-
Deployment:
- Docker: For containerizing the application, ensuring consistency across development and production environments.
- Cloud Provider (e.g., AWS, Heroku): For hosting the application and managing scalability.
3. Interesting Implementation Details
Mood Weather Selection
// Example of mood selection in React
const [mood, setMood] = useState('happy');
const handleMoodChange = (newMood) => {
setMood(newMood);
// Fetch islands based on selected mood
fetchIslands(newMood);
};
Golden Eggs and Emotional Gems
// API endpoint to collect an Emotional Gem
app.post('/api/collect-gem', (req, res) => {
const { userId, gemId } = req.body;
// Logic to update user's collected gems in the database
User.findByIdAndUpdate(userId, { $addToSet: { gems: gemId } }, { new: true })
.then(user => res.json(user))
.catch(err => res.status(500).json({ error: err.message }));
});
4. Technical Challenges Overcome
User Session Management
// Middleware for verifying JWT
const verifyToken = (req, res, next) => {
const token = req.headers['authorization'];
if (!token) return res.sendStatus(403);
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
};
Real-Time Updates
// WebSocket server setup
const wss = new WebSocket.Server({ server });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
// Broadcast message to all connected clients
wss.clients.forEach(client => {
if (client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
});
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
User-Centric Design: Prioritizing user experience is crucial. We learned that incorporating user feedback early in the design process helped us create a more engaging and intuitive interface. Conducting usability tests revealed areas for improvement that we hadn’t considered.
-
Emotional Mapping: Developing a framework for mapping emotions to game mechanics was essential. We discovered that aligning challenges and rewards with specific emotional states enhanced player engagement and provided a more meaningful experience.
-
Data Management: Managing user data securely while allowing for personalized experiences was a challenge. We implemented robust data handling practices to ensure user privacy and compliance with regulations, which ultimately built trust with our players.
-
Iterative Development: Adopting an agile development approach allowed us to iterate quickly based on testing and feedback. This flexibility helped us refine features and fix bugs more efficiently.
What Worked Well
-
Engaging Gameplay: The combination of fun challenges and reflective activities resonated well with players. Many users reported feeling more positive and centered after playing, which validated our game’s purpose.
-
Diverse Emotional Tools: Offering a variety of activities, such as journaling prompts and mindfulness exercises, catered to different player preferences and needs. This diversity kept the gameplay fresh and engaging.
-
Visual and Audio Design: The art style and soundscapes created an immersive experience that enhanced emotional engagement. Players appreciated the calming aesthetics, which contributed to the overall mood of the game.
-
Community Building: Creating a platform for players to share their experiences and insights fostered a sense of community. This social aspect encouraged players to return and engage with the game regularly.
What You’d Do Differently
-
More Extensive Testing: While we conducted usability tests, we realized that more extensive beta testing with a diverse group of users could have provided deeper insights into user behavior and preferences.
-
Enhanced Onboarding: The initial onboarding process could be improved to better guide new players through the game mechanics and emotional tools. A more interactive tutorial could help users feel more comfortable and engaged from the start.
-
Scalability Considerations: We encountered performance issues as the user base grew. Planning for scalability from the beginning, including optimizing code and server infrastructure, would have mitigated these challenges.
-
Feedback Loop: Establishing a more structured feedback loop with players could have helped us identify pain points and areas for improvement more quickly. Regular surveys or feedback sessions could enhance player satisfaction.
Advice for Others
-
Focus on Emotional Impact: When designing games centered around emotions, ensure that every element—from gameplay mechanics to visuals—contributes to the emotional experience you want to create.
-
Incorporate User Feedback: Engage with your audience throughout the development process. Their insights can guide your design choices and help you create a more user-friendly product.
-
Prioritize Accessibility: Make your game accessible to a wide range of players, including those with disabilities. Consider implementing features like text-to-speech, color contrast adjustments, and customizable controls.
-
Build a Supportive Community: Encourage players to share their experiences and support one another. A strong community can enhance player retention and create a positive environment for emotional exploration.
What’s Next?
Conclusion: Looking Ahead for Mood Island Journey
Project Development Analytics
timeline gant
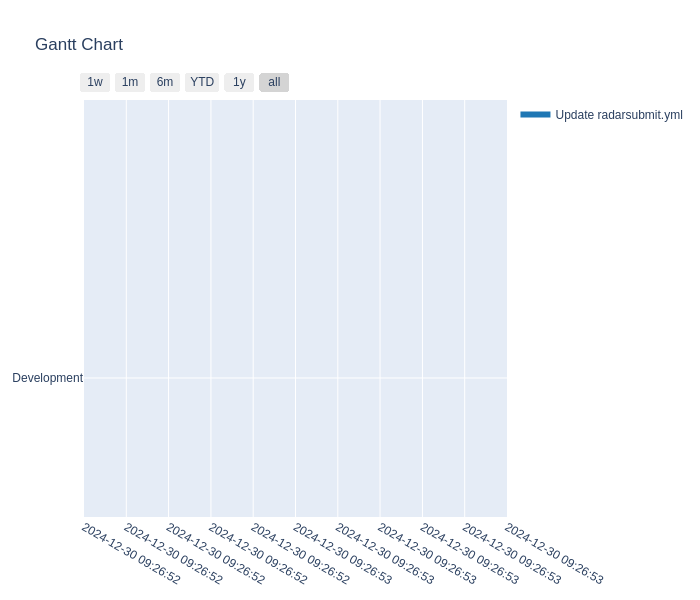
Commit Activity Heatmap
Contributor Network
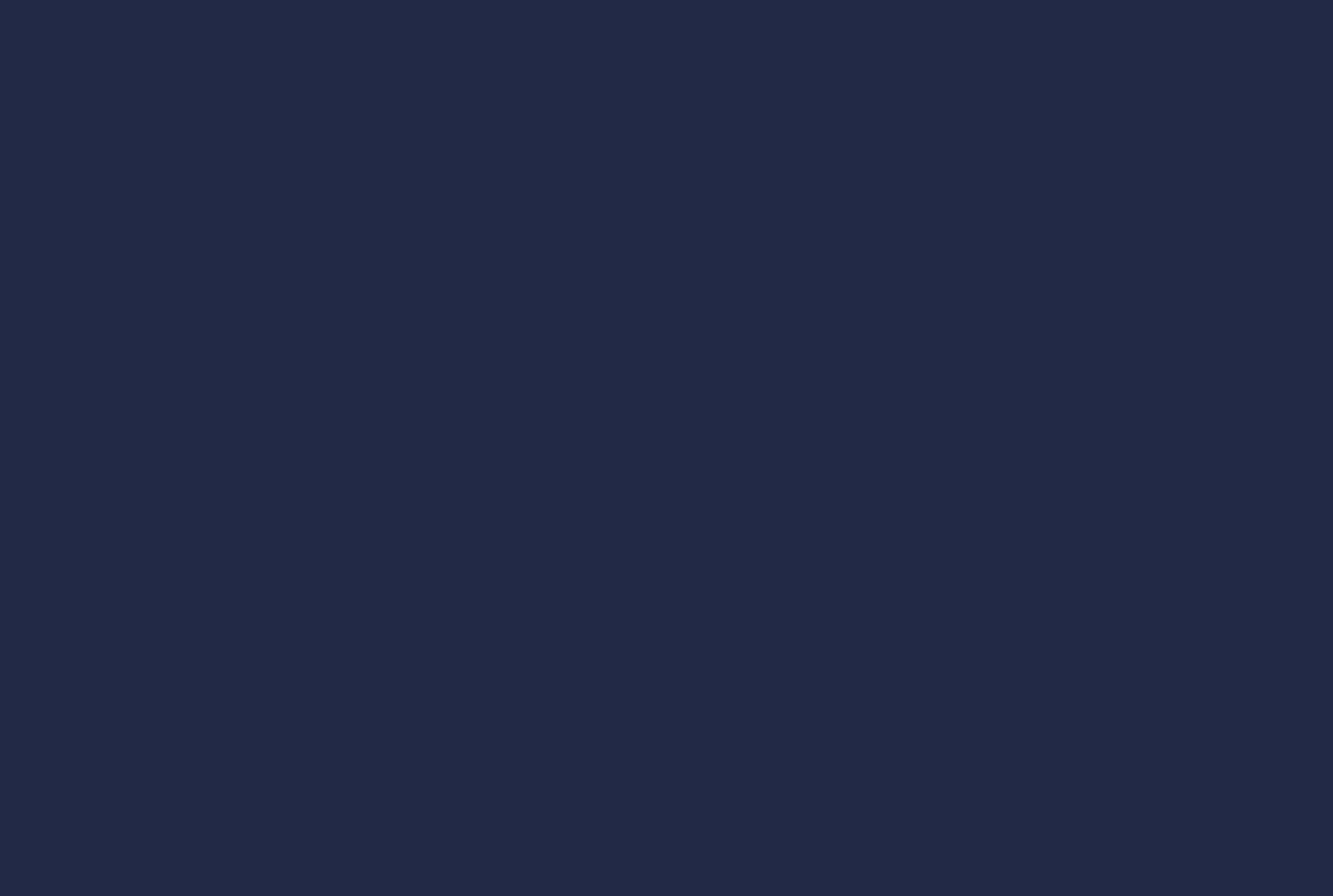
Commit Activity Patterns
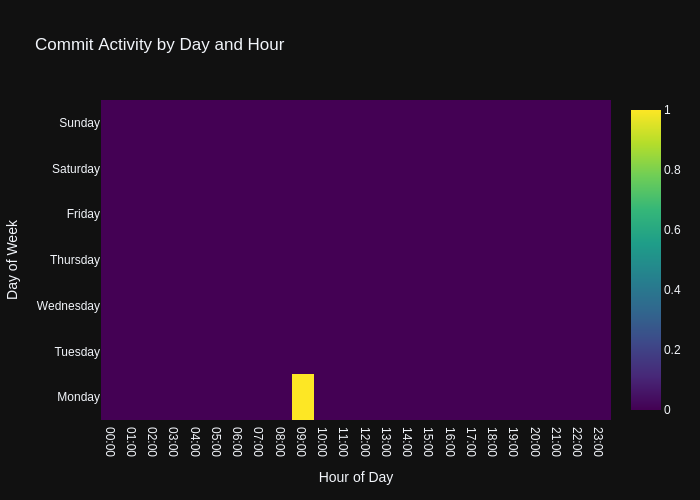
Code Frequency
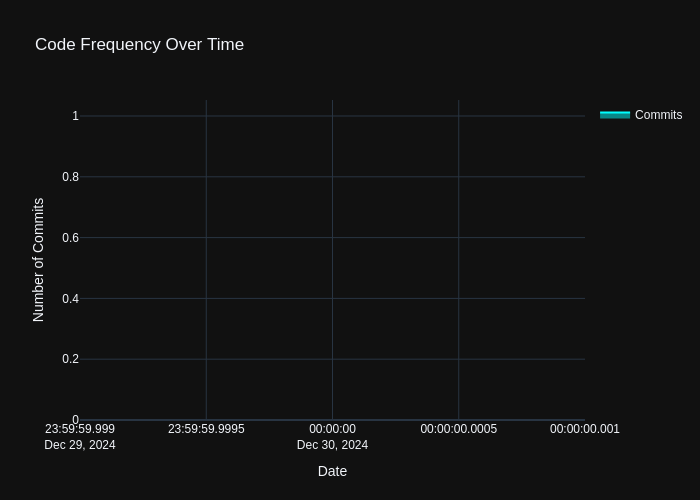
- Repository URL: https://github.com/wanghaisheng/carry-ceolbert-back-to-webserie
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日