Building an Arcade Adventure: Crafting 'Can You Pass This Level' with Websim
Project Genesis
Can You Pass This Level? Building a Website with Websim
From Idea to Implementation
1. Initial Research and Planning
- SEO Best Practices: Understanding the importance of sitemaps, metadata, and mobile responsiveness.
- User Experience: Ensuring that the site is accessible and provides a seamless experience across devices.
- Performance Metrics: Identifying tools for tracking user engagement and site performance, such as Google Analytics and Microsoft Clarity.
- Progressive Web App (PWA) Features: Exploring the benefits of PWA support for offline access and improved performance.
2. Technical Decisions and Their Rationale
-
Framework Selection: The decision to use a specific web framework was based on its ability to support responsive design and SEO features. A framework that allows for easy integration of SEO tools was prioritized.
-
Sitemap Generation: Automating the sitemap generation based on language subfolders was chosen to streamline the process of keeping the site indexed correctly by search engines. This decision was rooted in the need for efficient site management, especially as content scales.
-
SEO Checks: Implementing automated checks for SEO requirements was crucial to avoid common pitfalls like Google redirection issues and non-indexing. This proactive approach minimizes the risk of losing visibility in search results.
-
IndexNow Integration: The choice to use IndexNow for URL submission was driven by the need for rapid indexing of new content, ensuring that updates are reflected in search engines as quickly as possible.
-
PWA Support: Incorporating PWA features was a strategic decision to enhance user experience, allowing for offline access and faster load times, which are critical for retaining users.
3. Alternative Approaches Considered
-
Manual vs. Automated Processes: Initially, there was a consideration to handle sitemap generation and SEO checks manually. However, the decision to automate these processes was made to save time and reduce human error.
-
Different Analytics Tools: While Google Analytics was the primary choice for tracking user engagement, other analytics tools were evaluated. Ultimately, the combination of Google Analytics and Microsoft Clarity was selected for their complementary features.
-
Content Generation Tools: Various content generation tools were assessed for blog text generation. The decision to use the G4F tool was based on its ability to produce high-quality, relevant content efficiently.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of designing with the user in mind became clear early on. Features like mobile responsiveness and PWA support were prioritized to enhance user experience.
-
SEO as a Continuous Process: SEO is not a one-time task but an ongoing process. This realization led to the integration of automated checks and tools to ensure that the site remains optimized over time.
-
Collaboration and Community Resources: Leveraging community resources, such as GitHub repositories, provided valuable tools and insights that shaped the development process. Collaborating with existing projects allowed for faster implementation and reduced redundancy.
-
Adaptability: The need for flexibility in the development process was highlighted, as new challenges and opportunities arose. Being open to adjusting the project scope and features based on user feedback and testing results proved essential.
Under the Hood
Technical Deep-Dive: WebSim Site Builder
1. Architecture Decisions
-
Modular Design: Each feature is encapsulated in its own module, making it easier to maintain and extend. For example, the SEO module handles all SEO-related tasks, while the sitemap generator is a separate module.
-
Microservices Approach: Some functionalities, such as image generation and blog text generation, can be implemented as microservices. This allows for independent scaling and deployment of these services.
-
Responsive Design: The site is built with a mobile-first approach, ensuring that it is responsive across devices. This decision is crucial for user experience and SEO.
-
Use of APIs: The architecture leverages external APIs for functionalities like Google Analytics and Microsoft Clarity, allowing for rich analytics without heavy lifting on the server side.
2. Key Technologies Used
-
HTML/CSS/JavaScript: The foundational technologies for building the web interface.
-
Node.js: Used for server-side scripting, enabling the handling of requests and responses efficiently.
-
Express.js: A web application framework for Node.js that simplifies routing and middleware integration.
-
Google Indexing API: Utilized for submitting URLs to Google, enhancing the site’s visibility.
-
Progressive Web App (PWA) Technologies: Service workers and manifest files are used to enable offline capabilities and improve performance.
-
SEO Tools: Libraries and tools for generating sitemaps and checking SEO requirements.
3. Interesting Implementation Details
Auto-Generate Sitemap
.html
files. The implementation can be seen in the following code snippet:const fs = require('fs');
const path = require('path');
function generateSitemap(langFolder) {
const sitemap = [];
const files = fs.readdirSync(langFolder);
files.forEach(file => {
if (file.endsWith('.html')) {
sitemap.push(`<url><loc>${path.join(langFolder, file)}</loc></url>`);
}
});
return `<urlset>${sitemap.join('')}</urlset>`;
}
SEO Requirements Checker
const axios = require('axios');
async function checkSEO(url) {
const response = await axios.get(url);
if (response.status === 200 && !response.headers['x-redirected']) {
console.log('SEO check passed for:', url);
} else {
console.error('SEO issue detected for:', url);
}
}
4. Technical Challenges Overcome
Handling Multiple Languages
Image Generation
async function generateImage(type, data) {
const response = await axios.post('https://image-api.com/generate', { type, data });
return response.data.url;
}
PWA Support
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
});
});
}
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Automation is Key: Automating tasks such as sitemap generation, SEO checks, and URL submissions significantly reduces manual effort and minimizes human error. Implementing tools like Websim for automation can streamline the development process.
-
SEO Best Practices: Understanding and implementing SEO requirements early in the project helps avoid common pitfalls like Google redirection issues and indexing problems. Regularly checking SEO metrics is crucial for maintaining site visibility.
-
Responsive Design: Ensuring that the site is responsive for both PC and mobile users is essential. Utilizing frameworks or libraries that support responsive design can save time and improve user experience.
-
Integration of Analytics: Incorporating tools like Google Analytics and Microsoft Clarity from the start provides valuable insights into user behavior and site performance, allowing for data-driven decisions.
-
Progressive Web App (PWA) Features: Implementing PWA support enhances user engagement and accessibility, making the site more versatile across different devices.
What Worked Well
-
Sitemap Generation: The automatic generation of sitemaps based on language subfolders and HTML files was effective in improving site navigation and SEO.
-
SEO Checks: The automated checks for SEO requirements helped identify and rectify issues early, ensuring better compliance with search engine guidelines.
-
Keyword Research Tools: Utilizing tools like SpyFu for keyword research provided valuable insights into trending keywords and helped optimize content effectively.
-
Image Generation: The ability to generate logos and cover images streamlined the branding process, allowing for a cohesive visual identity.
-
Blog Text Generation: Integrating automated blog text generation tools like G4F facilitated content creation, keeping the site updated with fresh material.
What You’d Do Differently
-
More Comprehensive Testing: While automation is beneficial, incorporating more extensive testing (both manual and automated) could help catch edge cases and improve overall site stability.
-
User Feedback Loop: Establishing a feedback mechanism for users early in the project could provide insights into user experience and areas for improvement.
-
Documentation: Improving documentation throughout the development process would help onboard new contributors and maintain clarity on project goals and features.
-
Performance Optimization: Focusing more on performance optimization from the beginning could enhance load times and overall user experience.
Advice for Others
-
Start with a Clear Plan: Before diving into development, outline clear goals and features. This will help keep the project focused and organized.
-
Leverage Existing Tools: Don’t reinvent the wheel. Utilize existing tools and libraries to save time and effort, especially for common tasks like SEO checks and analytics integration.
-
Iterate Based on Data: Use analytics data to inform decisions and iterate on features. Regularly review performance metrics to identify areas for improvement.
-
Stay Updated on SEO Trends: SEO is constantly evolving. Stay informed about the latest trends and algorithm changes to ensure your site remains competitive.
-
Engage with the Community: Participate in forums and communities related to web development and SEO. Sharing experiences and learning from others can provide valuable insights and support.
What’s Next?
Conclusion: Looking Ahead for Can-You-Pass-This-Level
Project Development Analytics
timeline gant
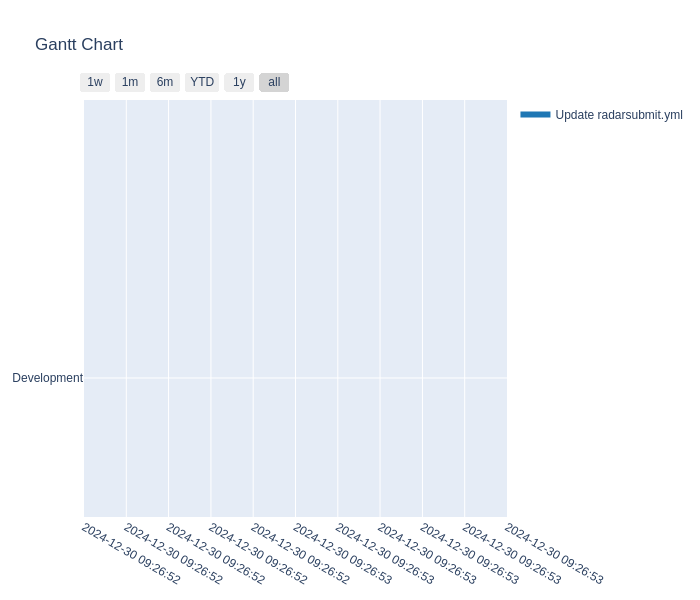
Commit Activity Heatmap
Contributor Network
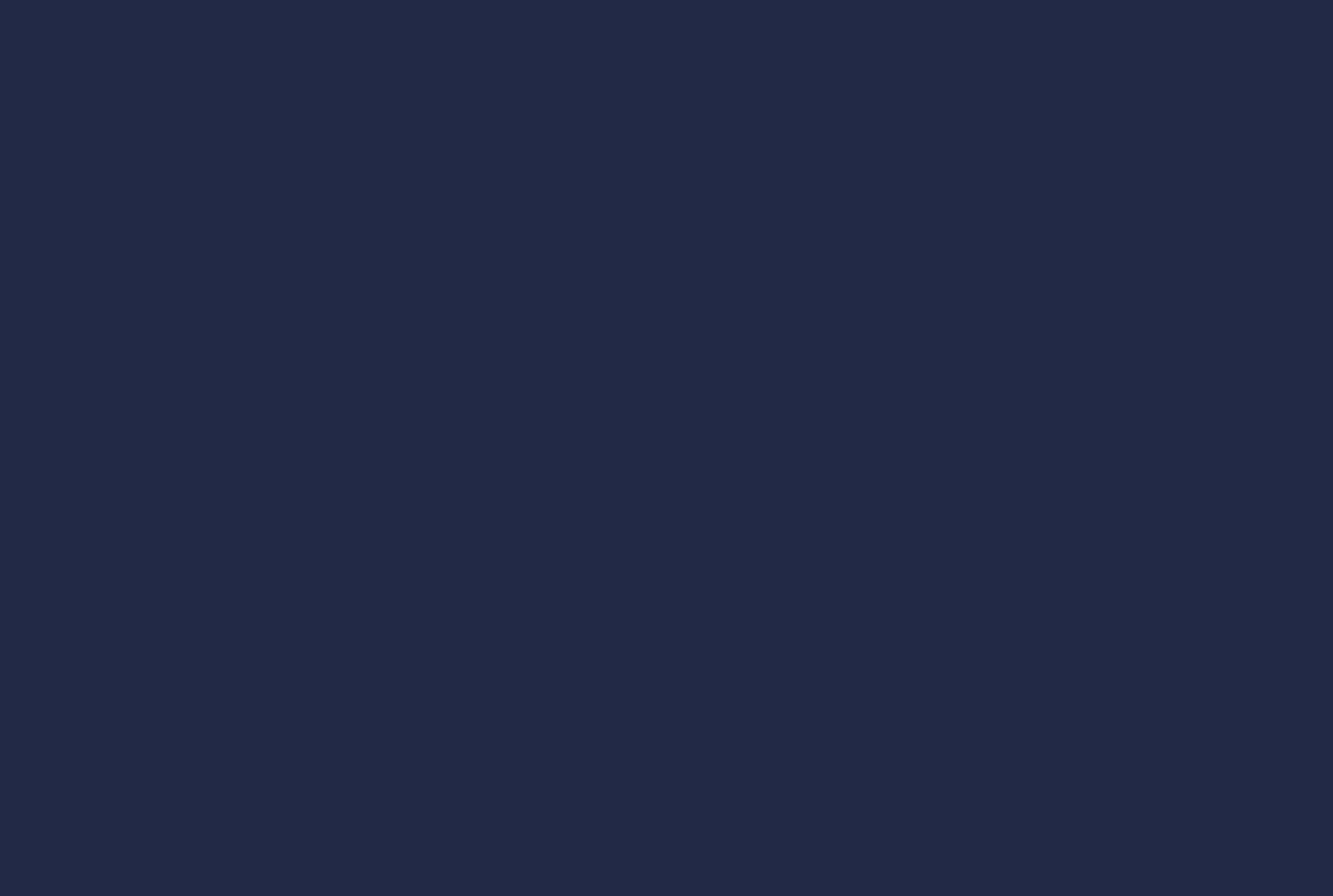
Commit Activity Patterns
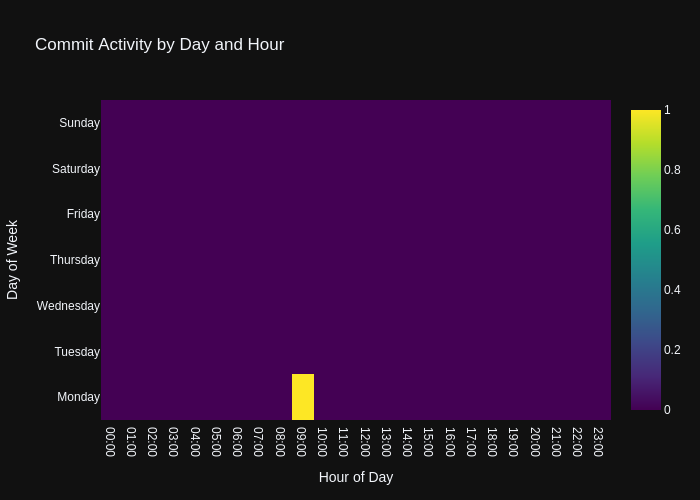
Code Frequency
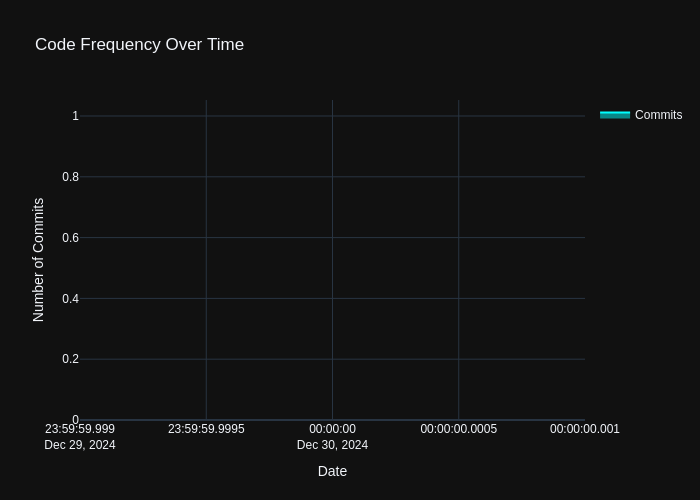
- Repository URL: https://github.com/wanghaisheng/can-you-pass-this-level
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日