Building a Simple Calculator PWA: My Journey with Astro and Svelte
Project Genesis
Unleashing the Power of Simplicity: My Journey with Calculator PWA
From Idea to Implementation
Journey from Concept to Code: Calculator PWA
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: Prioritizing user experience was paramount. We conducted user testing sessions to gather feedback on the interface and functionality, which led to iterative improvements. This reinforced the importance of designing with the end-user in mind.
-
Performance Matters: The decision to focus on performance was validated during testing. Users appreciated the quick load times and smooth interactions, which are essential for a tool that is often used in quick, on-the-go scenarios.
-
Simplicity is Key: The initial feature set was kept minimal, focusing on essential functionalities. This allowed us to launch the app quickly and gather user feedback before adding more complex features like memory functions. This iterative approach ensured that we were building features that users genuinely wanted.
Under the Hood
Technical Deep-Dive: Calculator PWA
1. Architecture Decisions
Key Architectural Choices:
- Component-Based Structure: Svelte’s component-based architecture allows for reusable UI components, making it easier to manage the calculator’s UI and logic.
- Separation of Concerns: The application is structured to separate the UI components from the business logic, enhancing maintainability and scalability.
- Routing and State Management: Although this simple calculator may not require complex routing, Astro provides a straightforward way to manage routes, while Svelte’s built-in stores can be used for state management.
2. Key Technologies Used
- Astro: A static site generator that allows for building fast websites with a focus on performance. It enables the use of various frameworks (like Svelte) within the same project.
- Svelte: A modern JavaScript framework that compiles components into highly efficient vanilla JavaScript, resulting in faster load times and better performance.
- Service Workers: To enable PWA features such as offline access and caching, service workers are implemented to manage network requests and cache assets.
Example of Service Worker Registration:
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(error => {
console.error('Service Worker registration failed:', error);
});
});
}
3. Interesting Implementation Details
History Feature
Example of History Store:
import { writable } from 'svelte/store';
export const historyStore = writable([]);
export function addToHistory(calculation) {
historyStore.update(history => [...history, calculation]);
}
Memory Feature (Planned)
UI Components
Example of a Button Component:
4. Technical Challenges Overcome
Performance Optimization
Offline Functionality
Example of Cache Management in Service Worker:
self.addEventListener('install', event => {
event.waitUntil(
caches.open('calculator-cache').then(cache => {
return cache.addAll([
'/',
'/index.html',
'/global.css',
'/build/bundle.js',
]);
})
);
});
User Experience
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Integration of Frameworks: Combining Astro and Svelte was a valuable experience. Astro’s static site generation capabilities complemented Svelte’s reactive components well, allowing for a smooth user experience.
- Service Workers: Implementing service workers for offline functionality was crucial. Understanding how to cache assets and manage network requests improved the app’s performance and usability.
- State Management: Managing state in a reactive framework like Svelte taught the importance of keeping the UI in sync with the underlying data model, especially for features like history and memory.
2. What Worked Well
- User Interface: The design of the calculator was intuitive and user-friendly. The layout and button responsiveness contributed to a positive user experience.
- Performance: The app performed well across different devices, thanks to the lightweight nature of both Astro and Svelte. The loading times were minimal, enhancing user engagement.
- Progressive Enhancement: The PWA features, such as installability and offline access, worked seamlessly, providing users with a native app-like experience.
3. What You’d Do Differently
- Memory Feature Implementation: The memory feature was left incomplete. If revisiting the project, I would prioritize this feature earlier in the development process to enhance functionality and user experience.
- Testing: Implementing a more robust testing strategy, including unit tests and end-to-end tests, would have helped catch bugs earlier and ensured the app’s reliability.
- Documentation: While the README provided a good overview, more detailed documentation on setup, usage, and contribution guidelines would benefit future developers and users.
4. Advice for Others
- Start Small: When building a PWA, start with core functionalities and gradually add features. This approach helps maintain focus and allows for iterative improvements.
- Leverage Community Resources: Utilize community forums and documentation for both Astro and Svelte. Engaging with the community can provide insights and solutions to common challenges.
- Focus on User Experience: Prioritize user experience from the beginning. Conduct user testing to gather feedback and iterate on the design based on real user interactions.
- Plan for Offline Use: Consider offline capabilities early in the design process. Implementing service workers and caching strategies can significantly enhance the app’s usability.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
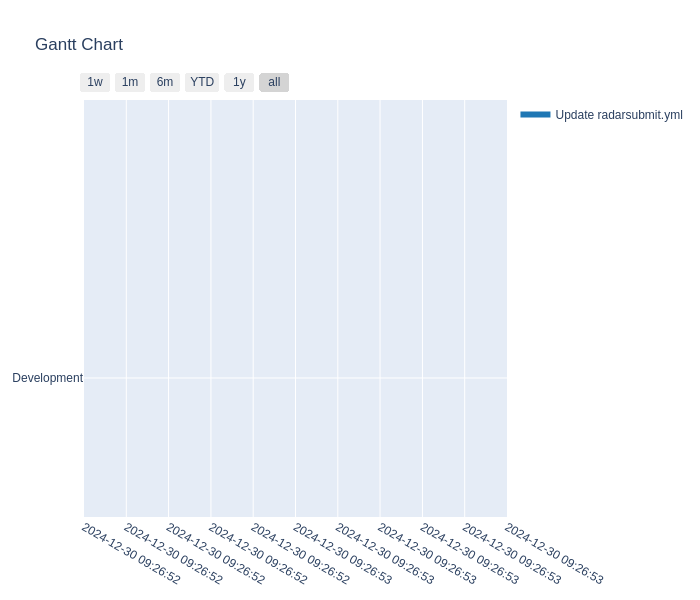
Commit Activity Heatmap
Contributor Network
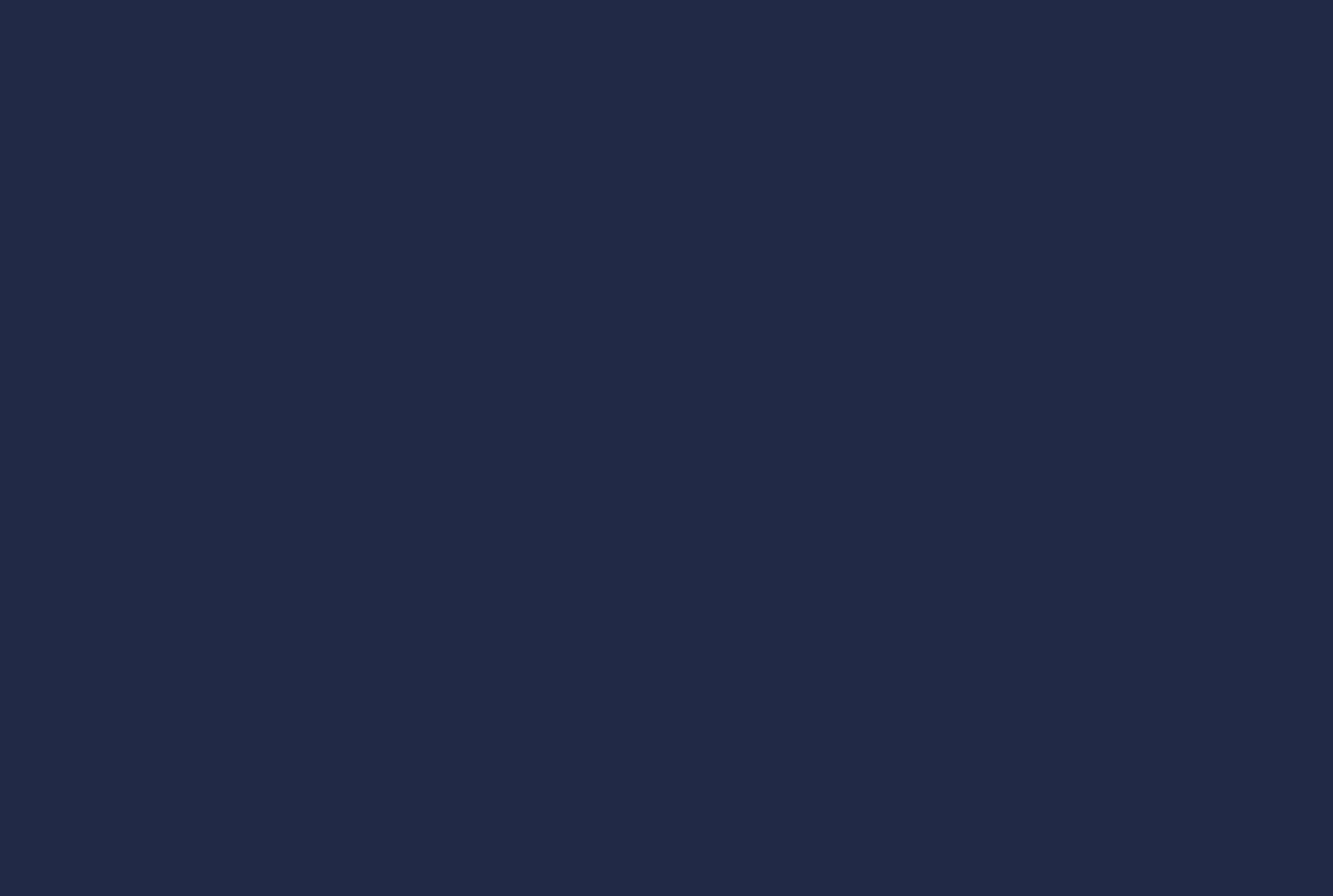
Commit Activity Patterns
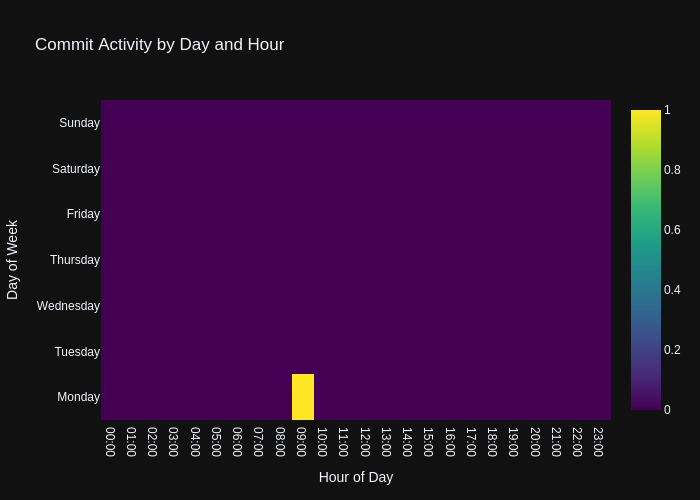
Code Frequency
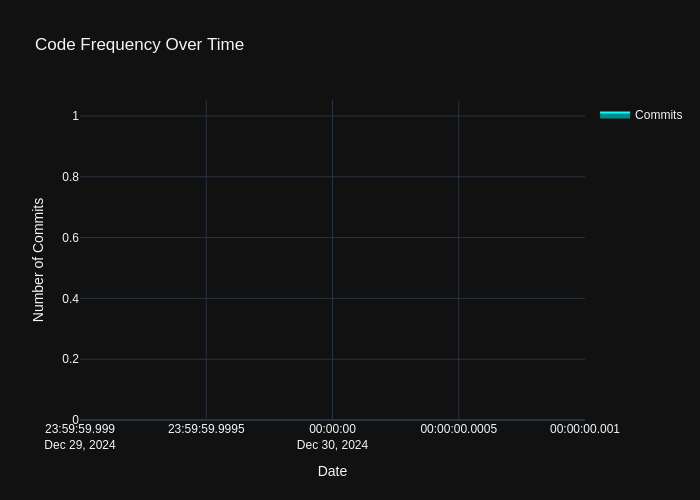
- Repository URL: https://github.com/wanghaisheng/calculator_pwa
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日