Building Blackjack Game: Crafting a Chat-Driven Casino Experience
Project Genesis
Unveiling My Blackjack Game: A Journey from Inspiration to Implementation
From Idea to Implementation
Initial Research and Planning
Technical Decisions and Their Rationale
Alternative Approaches Considered
Key Insights That Shaped the Project
-
User Experience is Paramount: The importance of a seamless user experience became evident early on. I focused on creating smooth animations and responsive design to ensure that players could enjoy the game on various devices without any hiccups.
-
Feedback is Essential: Incorporating sound effects for actions like dealing cards and placing bets added an extra layer of immersion. This realization highlighted the importance of sensory feedback in enhancing user engagement.
-
Flexibility and Customization: Allowing players to customize game parameters, such as the dealer’s stand value and maximum bet, was a crucial insight. This feature not only caters to different player preferences but also adds replayability to the game.
-
Iterative Development: The project benefited from an iterative development approach. By continuously testing and refining the game based on user feedback, I was able to identify and address issues early, leading to a more polished final product.
Under the Hood
Technical Deep-Dive: Blackjack Game
1. Architecture Decisions
- HTML Structure: The
index.html
file serves as the main entry point, providing the basic structure of the game interface. - Styling: The
styles.css
file is dedicated to the visual presentation, ensuring a responsive design that adapts to various screen sizes. - Game Logic: The core game mechanics are encapsulated in
blackjack.js
, which manages the game state, rules, and player interactions. - User Interface: The
blackjackui.js
file is responsible for updating the UI based on game events, ensuring a smooth user experience. - Audio Management: The
blackjackaudio.js
file handles sound effects, enhancing the immersive experience. - Card and Deck Management: The
card.js
anddeck.js
files define the data structures and methods for managing cards and decks.
2. Key Technologies Used
- HTML5: Provides the structure for the game interface, utilizing semantic elements for better accessibility.
- CSS3: Used for styling the game, including responsive design techniques such as media queries to ensure compatibility across devices.
- JavaScript (ES6+): The primary programming language for implementing game logic, utilizing modern features like classes, arrow functions, and template literals for cleaner code.
- GSAP (GreenSock Animation Platform): A powerful animation library that enables smooth transitions and animations, enhancing the visual appeal of the game.
Example of Using GSAP for Animations
// Example of card dealing animation using GSAP
gsap.fromTo(cardElement, { x: -100, opacity: 0 }, { x: 0, opacity: 1, duration: 0.5 });
3. Interesting Implementation Details
Game Logic
blackjack.js
file, where the rules of Blackjack are implemented. The game follows standard rules, including hitting, standing, doubling down, and splitting hands.Betting System
Statistics Tracking
// Example of tracking statistics
let stats = {
gamesPlayed: 0,
gamesWon: 0,
winPercentage: 0
};
function updateStats(won) {
stats.gamesPlayed++;
if (won) stats.gamesWon++;
stats.winPercentage = (stats.gamesWon / stats.gamesPlayed) * 100;
}
4. Technical Challenges Overcome
Responsive Design
Animation Performance
Sound Management
blackjackaudio.js
file was created to manage audio playback, ensuring that sounds are triggered at the right moments without causing delays.Example of Sound Management
// Example of playing a sound effect
function playSound(soundFile) {
const audio = new Audio(soundFile);
audio.play().catch(error => console.error("Audio playback failed:", error));
}
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Separation of Concerns: Structuring the project with separate files for different functionalities (e.g., game logic, UI updates, audio management) helped maintain clarity and made debugging easier. This modular approach allowed for easier updates and enhancements.
-
Responsive Design: Implementing responsive design principles was crucial for ensuring the game was playable on various devices. Utilizing CSS media queries and flexible layouts helped achieve a seamless experience across desktops and mobile devices.
-
State Management: Managing the game state effectively was essential for a smooth user experience. Implementing a clear state management strategy in
blackjack.js
allowed for easy tracking of player actions, game outcomes, and statistics. -
Animation Libraries: Using GSAP for animations significantly improved the visual appeal of the game. Learning how to integrate and utilize animation libraries can enhance user engagement and make the gameplay more enjoyable.
What Worked Well
-
User Experience: The combination of smooth animations, sound effects, and a responsive design contributed to an immersive gaming experience. Players appreciated the engaging interface and realistic gameplay.
-
Customization Options: Allowing users to customize game parameters (like dealer rules and betting limits) added flexibility and catered to different player preferences, which was well-received.
-
Statistics Tracking: Implementing a statistics tracking feature provided players with insights into their performance, enhancing the competitive aspect of the game.
-
Community Engagement: Opening the project for contributions encouraged community involvement, leading to valuable feedback and suggestions for improvements.
What You’d Do Differently
-
Testing Framework: Implementing a testing framework from the beginning would have helped catch bugs earlier in the development process. Using tools like Jest or Mocha for unit testing could improve code reliability.
-
Accessibility Features: While the game is visually appealing, I would prioritize accessibility features to ensure that it is playable for users with disabilities. This includes keyboard navigation, screen reader support, and color contrast adjustments.
-
Performance Optimization: As the game grows, performance may become an issue. I would focus on optimizing the code and assets (e.g., image sizes, audio files) to ensure smooth gameplay, especially on lower-end devices.
-
Documentation: While the README provides a good overview, I would create more detailed documentation for developers looking to contribute or modify the game. This could include code comments, a contribution guide, and a more comprehensive explanation of the game mechanics.
Advice for Others
-
Plan Your Architecture: Before diving into coding, take the time to plan the architecture of your project. Define clear responsibilities for each module and how they will interact with each other.
-
Iterate Based on Feedback: Release early and often. Gather feedback from users and iterate on your design and features based on their input. This will help you create a product that better meets user needs.
-
Focus on User Experience: Prioritize user experience in your design decisions. Consider how players will interact with your game and strive to make that experience as intuitive and enjoyable as possible.
-
Stay Updated with Technologies: Keep learning about new technologies and best practices in web development. This will help you improve your skills and apply the latest techniques to your projects.
-
Engage with the Community: Don’t hesitate to reach out to the developer community for support and collaboration. Engaging with others can lead to new ideas, solutions to problems, and potential partnerships.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
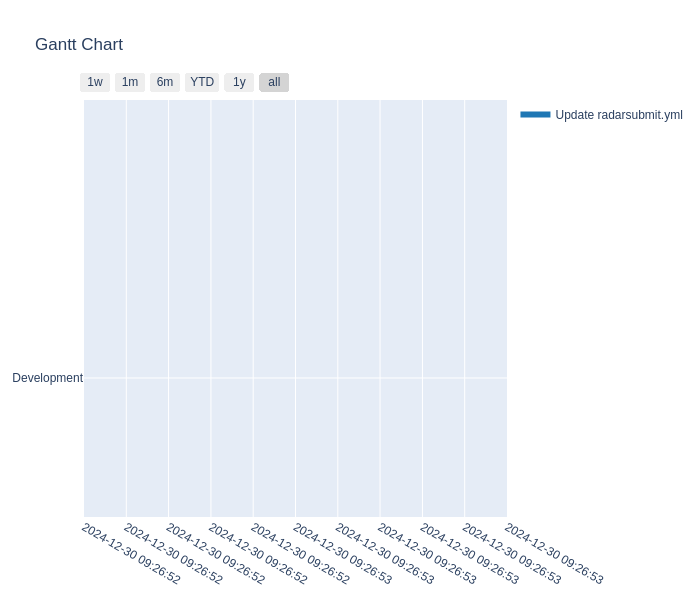
Commit Activity Heatmap
Contributor Network
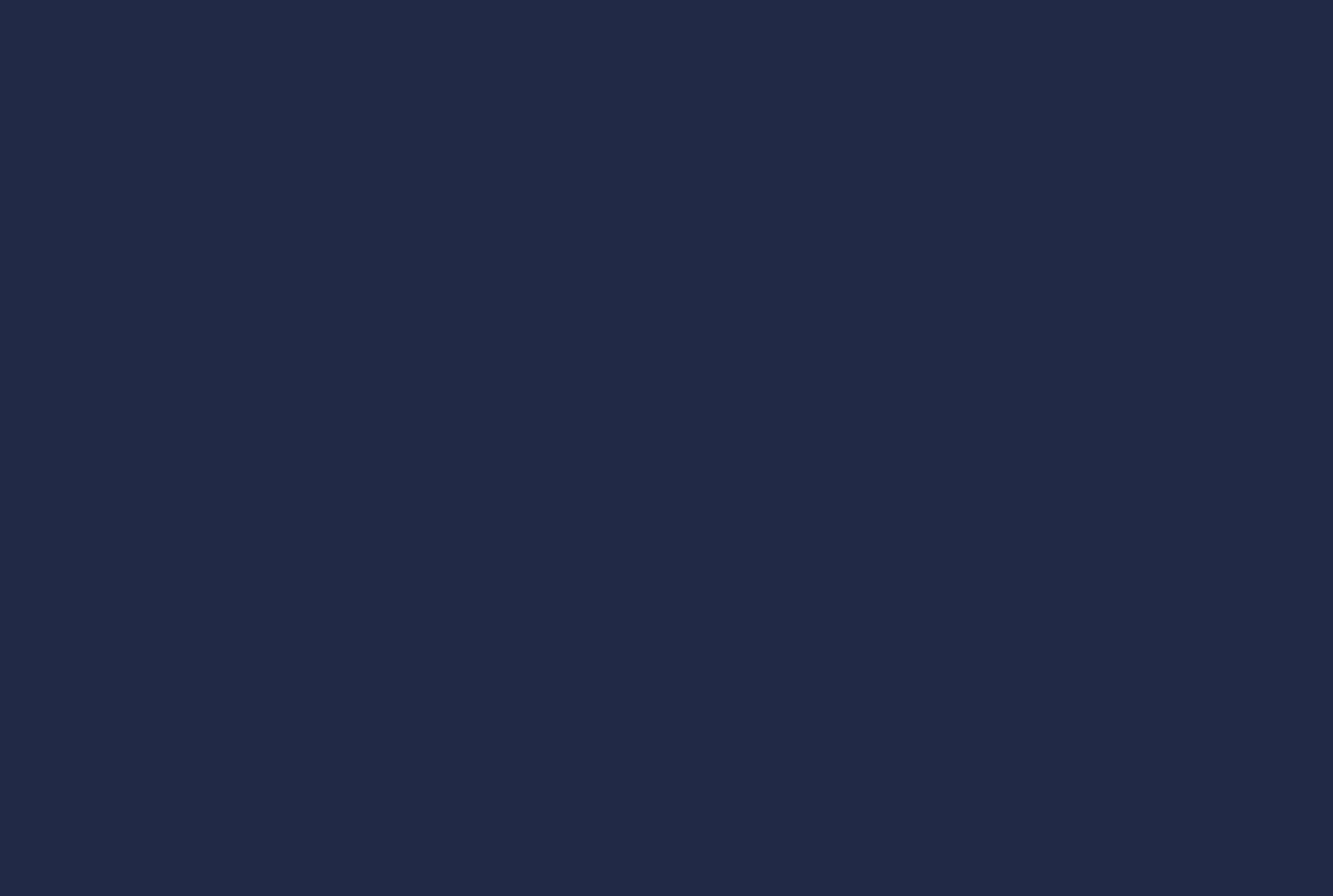
Commit Activity Patterns
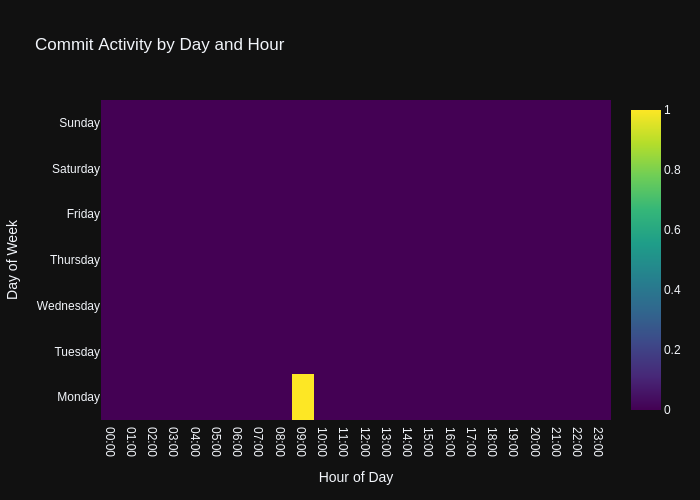
Code Frequency
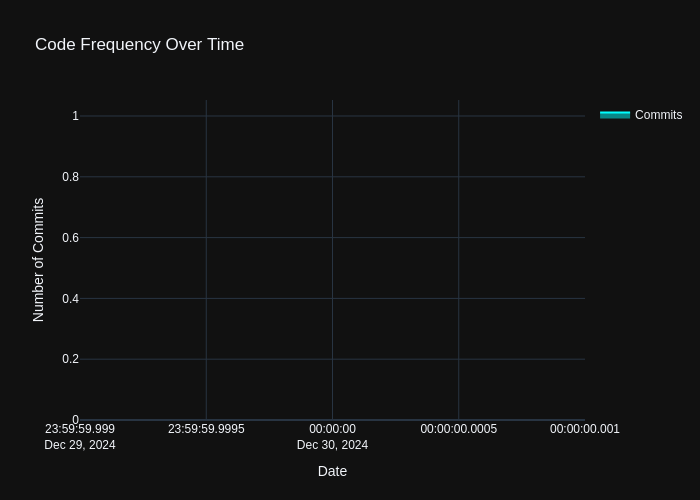
- Repository URL: https://github.com/wanghaisheng/blackjack-game
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日