Building BitesizeGames: Crafting a Seamless SEO-Friendly Game Hub
Project Genesis
Discovering Bitesize Games: A Journey of Passion and Innovation
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Choice of Programming Language: The team opted for Python due to its rich ecosystem of libraries for web scraping, SEO analysis, and HTTP requests. Libraries like
BeautifulSoup
for HTML parsing andrequests
for making HTTP calls were chosen for their ease of use and community support. -
Sitemap Generation: The decision to auto-generate the sitemap based on language subfolders was driven by the need for a structured approach to indexing. This would ensure that all relevant pages, including
.html
files, were included, improving the site’s discoverability. -
SEO Checks: Implementing automated SEO checks was crucial to avoid common pitfalls such as Google redirection issues and non-indexable pages. The team decided to create a set of rules based on best practices in SEO, which would be integrated into the workflow.
-
IndexNow Protocol: The choice to use the IndexNow protocol for URL submission was influenced by its efficiency and the growing trend of search engines adopting it. This decision aimed to streamline the process of notifying search engines about new or updated content.
3. Alternative Approaches Considered
-
Manual Sitemap Creation: Initially, there was a discussion about manually creating the sitemap. However, this was quickly dismissed due to the potential for human error and the time-consuming nature of the task, especially for larger sites.
-
Using Existing Tools: The team explored existing tools for SEO checks and sitemap generation. While some tools offered comprehensive features, they often came with limitations in customization and integration. Ultimately, the decision was made to build a tailored solution that could be easily adapted to the specific needs of the project.
-
Different Submission Methods: Other methods for submitting URLs to search engines were considered, such as using traditional XML sitemaps. However, the team concluded that IndexNow would provide a more immediate and efficient way to inform search engines of changes.
4. Key Insights That Shaped the Project
-
Importance of Automation: The realization that automation could save time and reduce errors was a driving force behind the project. By automating the sitemap generation and SEO checks, the team could focus on more strategic tasks, such as content creation and marketing.
-
User-Centric Design: Understanding the end-user’s perspective was crucial. The team recognized that a well-structured sitemap and adherence to SEO best practices would not only benefit search engines but also enhance the user experience by making content easier to find.
-
Iterative Development: Embracing an iterative approach allowed the team to test features incrementally and gather feedback. This led to continuous improvements and refinements, ensuring that the final product met the initial goals while adapting to new insights.
-
Collaboration and Communication: Regular communication among team members facilitated the sharing of ideas and challenges. This collaborative environment fostered creativity and problem-solving, ultimately leading to a more robust solution.
Under the Hood
Technical Deep-Dive: WebSim Site Builder
1. Architecture Decisions
- Sitemap Generator: Responsible for creating a sitemap based on the language subfolders and including all relevant
.html
files. - SEO Checker: A module that verifies SEO requirements to prevent issues like Google redirection and indexing problems.
- IndexNow Submission: A component that automates the submission of URLs to Google’s index using the IndexNow protocol.
2. Key Technologies Used
- Node.js: The server-side JavaScript runtime used for building the application. It allows for asynchronous operations, which is beneficial for tasks like checking SEO requirements and submitting URLs.
- Express.js: A web framework for Node.js that simplifies the creation of web applications and APIs.
- Cheerio: A fast, flexible, and lean implementation of core jQuery designed for the server, used for parsing and manipulating HTML.
- Axios: A promise-based HTTP client for the browser and Node.js, used for making requests to Google’s IndexNow API.
- File System (fs): Node.js’s built-in module for interacting with the file system, used for reading and writing sitemap files.
3. Interesting Implementation Details
Sitemap Generation
.html
files. The following code snippet demonstrates how this can be achieved:const fs = require('fs');
const path = require('path');
function generateSitemap(baseDir) {
const sitemap = [];
const languages = fs.readdirSync(baseDir);
languages.forEach(lang => {
const langDir = path.join(baseDir, lang);
if (fs.statSync(langDir).isDirectory()) {
const files = fs.readdirSync(langDir);
files.forEach(file => {
if (file.endsWith('.html')) {
sitemap.push(`https://example.com/${lang}/${file}`);
}
});
}
});
return sitemap;
}
SEO Checking
const cheerio = require('cheerio');
function checkSEO(filePath) {
const content = fs.readFileSync(filePath, 'utf-8');
const $ = cheerio.load(content);
const title = $('title').text();
if (!title) {
console.error(`SEO Error: Missing title tag in ${filePath}`);
}
}
IndexNow Submission
const axios = require('axios');
async function submitToIndexNow(urls) {
const apiKey = 'YOUR_INDEXNOW_API_KEY';
const endpoint = 'https://api.indexnow.org/indexnow';
const requests = urls.map(url => {
return axios.post(endpoint, {
url: url,
key: apiKey
});
});
try {
await Promise.all(requests);
console.log('URLs submitted successfully to IndexNow');
} catch (error) {
console.error('Error submitting URLs:', error);
}
}
4. Technical Challenges Overcome
Handling Asynchronous Operations
async/await
syntax helped streamline the code and improve readability.Error Handling
Scalability
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Understanding Sitemap Generation: Implementing an auto-generated sitemap based on language subfolders highlighted the importance of structuring content for multilingual sites. It reinforced the need for a clear directory structure to facilitate easier navigation and indexing by search engines.
-
SEO Compliance: The process of checking SEO requirements taught the significance of adhering to best practices. It was crucial to understand how Google interprets redirects and indexing, which can significantly impact site visibility.
-
IndexNow Protocol: Integrating the IndexNow API for URL submission was a valuable lesson in leveraging modern tools for SEO. It emphasized the importance of staying updated with the latest technologies that can enhance site performance and visibility.
What Worked Well
-
Automation of Sitemap Generation: The automated sitemap generation was a significant success. It saved time and reduced human error, ensuring that all relevant pages were included and correctly formatted.
-
SEO Checks: The automated checks for SEO compliance were effective in identifying potential issues before deployment. This proactive approach helped in maintaining a healthy site structure and improved overall SEO performance.
-
Integration with IndexNow: The ability to automatically submit URLs to Google’s index through IndexNow streamlined the process of getting new content indexed quickly, which is crucial for maintaining site relevance.
What You’d Do Differently
-
Enhanced Testing: While the initial implementation was successful, more rigorous testing could have been conducted, especially for the SEO checks. Implementing a staging environment to test changes before going live would help catch issues earlier.
-
User Feedback Loop: Establishing a feedback mechanism from users regarding the sitemap and SEO checks could provide insights into real-world performance and areas for improvement.
-
Documentation and Tutorials: Creating more comprehensive documentation and tutorials for future developers or users would facilitate easier onboarding and understanding of the system.
Advice for Others
-
Start with a Clear Plan: Before diving into implementation, outline a clear plan that includes the features you want to build, the technologies you will use, and the expected outcomes. This will help keep the project focused and organized.
-
Prioritize SEO from the Start: Incorporate SEO best practices early in the development process. This will save time and effort later on, as addressing SEO issues post-launch can be more challenging.
-
Leverage Automation: Utilize automation tools wherever possible to streamline repetitive tasks, such as sitemap generation and SEO checks. This not only saves time but also reduces the likelihood of human error.
-
Stay Updated: The web development and SEO landscapes are constantly evolving. Regularly update your knowledge and tools to ensure your site remains competitive and compliant with the latest standards.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
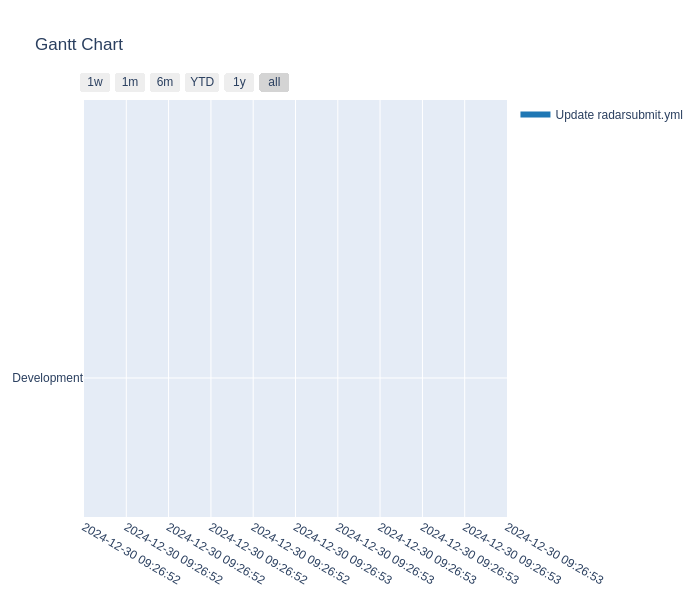
Commit Activity Heatmap
Contributor Network
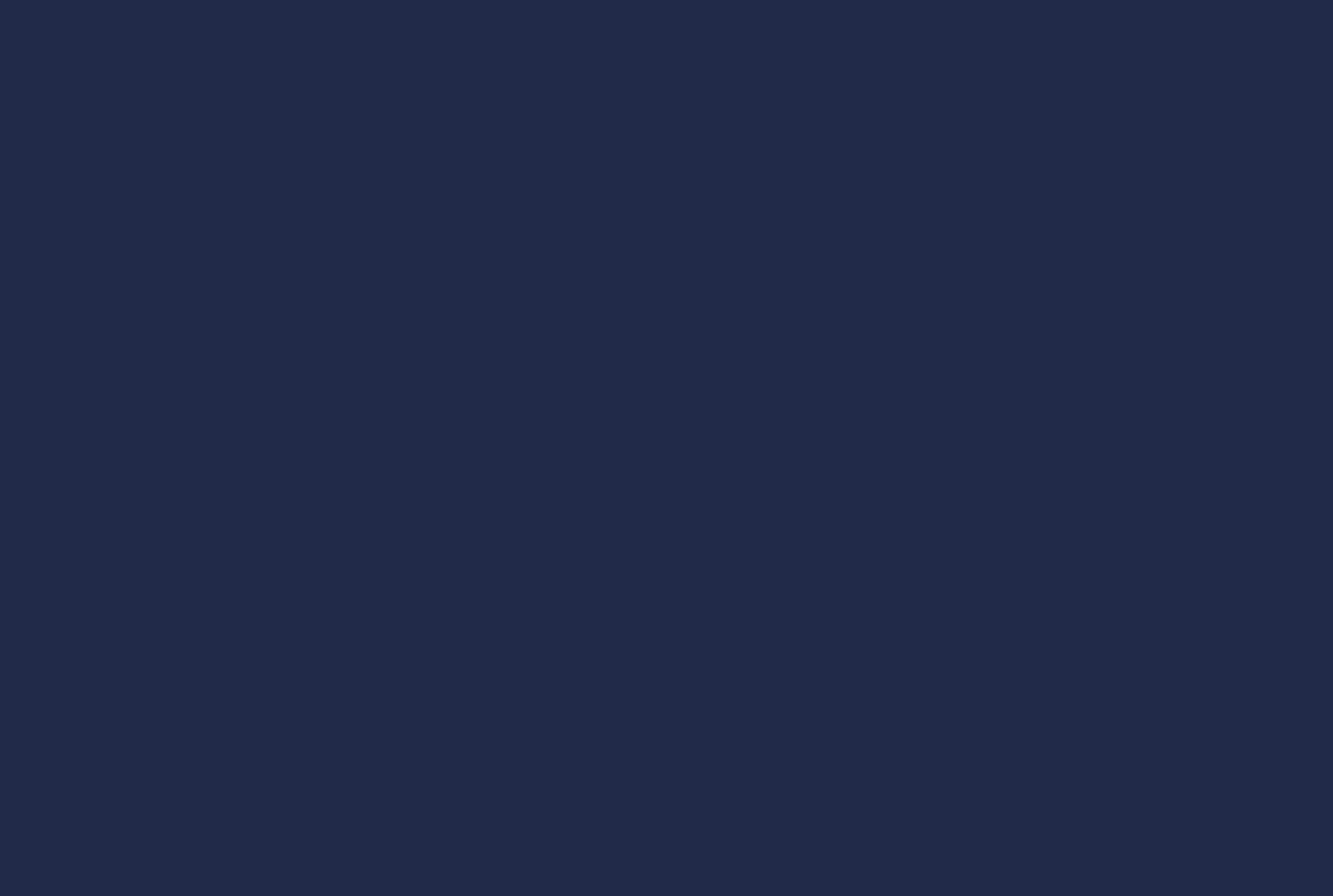
Commit Activity Patterns
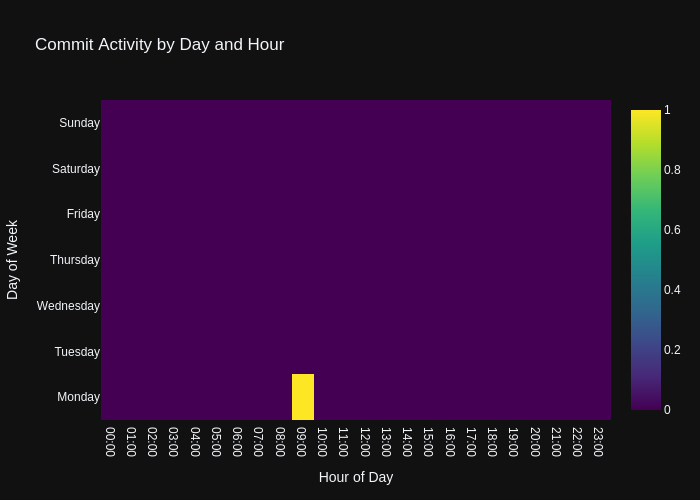
Code Frequency
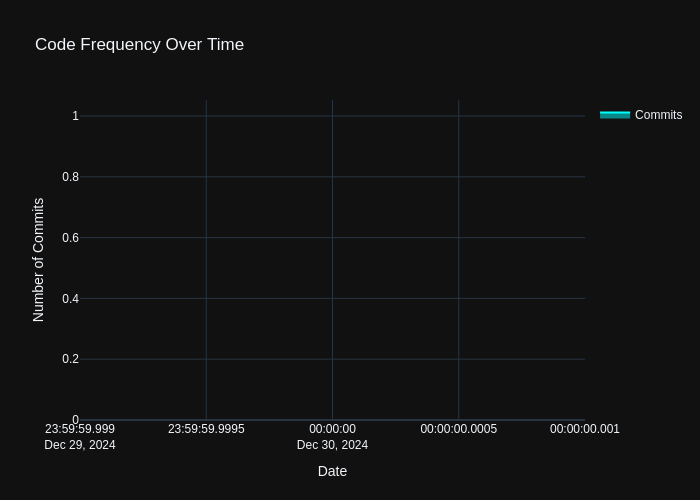
- Repository URL: https://github.com/wanghaisheng/bitesizegames.org
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日