Creating Buzz: Building BetaBuzz to Rate the Hottest New Products
Project Genesis
Welcome to BetaBuzz: Where Innovation Meets Community
From Idea to Implementation
Initial Research and Planning
Technical Decisions and Their Rationale
Alternative Approaches Considered
Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became evident early on. Feedback from potential users highlighted the need for intuitive navigation and a clean layout, which guided design decisions.
-
Community Engagement: The realization that community interaction is vital for the success of a product discovery platform led to the incorporation of features like comments and voting. This insight emphasized the need for a robust moderation system to maintain a healthy community.
-
Iterative Development: Embracing an agile development approach allowed the team to iterate quickly based on user feedback. This flexibility was crucial in refining features and addressing user needs effectively.
-
Scalability Considerations: The foresight to build a scalable architecture from the outset was a significant insight. Anticipating future growth and user demand shaped many technical decisions, ensuring that the platform could evolve without major overhauls.
Under the Hood
Technical Deep-Dive: BetaBuzz
1. Architecture Decisions
Key Architectural Choices:
- Microservices Approach: The application is divided into two main components: the API (backend) and the app (frontend). This separation allows for independent development and deployment.
- RESTful API: The backend is built using Express.js, providing a RESTful interface for the frontend to interact with. This design choice simplifies the communication between the client and server.
- JWT Authentication: For user authentication, JSON Web Tokens (JWT) are used. This stateless authentication method enhances security and scalability.
- Cloud Storage: User-generated content is stored in Amazon S3, allowing for scalable and reliable storage solutions.
2. Key Technologies Used
-
Frontend:
- Next.js: A React framework that enables server-side rendering and static site generation, improving performance and SEO.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
-
Backend:
- Express.js: A minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications.
- MongoDB: A NoSQL database used for storing user data and product information, allowing for flexible data models.
-
Payment Processing:
- Stripe: Integrated for handling subscriptions and premium features, providing a secure and reliable payment processing solution.
3. Interesting Implementation Details
Folder Structure
.
├── api
│ ├── src
│ │ ├── config
│ │ ├── controllers
│ │ ├── models
│ │ ├── routes
│ │ └── services
└── app
├── components
├── pages
├── services
└── styles
JWT Authentication
// Example of generating a JWT token
const jwt = require('jsonwebtoken');
function generateToken(user) {
return jwt.sign({ id: user._id }, process.env.JWT_SECRET, { expiresIn: '1h' });
}
Recursive Comments
const commentSchema = new mongoose.Schema({
content: String,
userId: { type: mongoose.Schema.Types.ObjectId, ref: 'User' },
parentId: { type: mongoose.Schema.Types.ObjectId, ref: 'Comment', default: null },
createdAt: { type: Date, default: Date.now }
});
4. Technical Challenges Overcome
Handling User-Generated Content
const AWS = require('aws-sdk');
const s3 = new AWS.S3();
function uploadFile(file) {
const params = {
Bucket: process.env.S3_BUCKET,
Key: file.name,
Body: file.data,
};
return s3.upload(params).promise();
}
Subscription Management
const stripe = require('stripe')(process.env.STRIPE_SECRET_KEY);
app.post('/webhook', express.json(), (req, res) => {
const event = req.body;
switch (event.type) {
case 'customer.subscription.updated':
// Handle subscription update
break;
// Handle other event types
}
res.json({ received: true });
});
Scalability
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Authentication and Security: Implementing JWT-based authentication was crucial for securing user data and managing sessions. Understanding the intricacies of token expiration and refresh mechanisms was essential to ensure a smooth user experience.
-
File Storage Management: Using S3 for storing user-generated content taught me about handling file uploads, managing permissions, and optimizing storage costs. It also highlighted the importance of considering scalability when choosing a storage solution.
-
Real-time Features: Implementing a comments system with recursive threads required careful consideration of data structures and state management. This experience reinforced the importance of designing APIs that can efficiently handle nested data.
-
Payment Integration: Integrating Stripe for subscription services provided insights into handling sensitive user data and compliance with payment regulations. It emphasized the need for thorough testing in payment workflows to avoid potential issues.
-
Responsive Design: Utilizing Tailwind CSS for styling allowed for rapid prototyping and ensured a responsive design. This experience underscored the importance of mobile-first design principles in modern web applications.
What Worked Well
-
Modular Architecture: The separation of the API and frontend into distinct folders (
api
andapp
) facilitated easier development and maintenance. This modular approach allowed different teams to work on the backend and frontend simultaneously without conflicts. -
Community Engagement: The voting and commenting features fostered community interaction, which was a key aspect of the platform’s success. Encouraging user feedback helped in iterating on features and improving user experience.
-
Documentation: Maintaining clear and concise documentation throughout the project made onboarding new contributors easier and helped in keeping the development process organized.
-
Testing Strategy: Implementing a robust testing strategy with unit and integration tests ensured that new features could be added with confidence, reducing the likelihood of introducing bugs.
What You’d Do Differently
-
Early User Feedback: In hindsight, involving users earlier in the development process could have provided valuable insights and helped prioritize features that truly matter to the community.
-
Performance Optimization: While the application performs well, I would focus more on performance optimization from the start, particularly in areas like database queries and API response times, to enhance user experience.
-
CI/CD Pipeline: Setting up a continuous integration and deployment (CI/CD) pipeline earlier in the project would have streamlined the deployment process and reduced manual errors.
-
Scalability Considerations: Planning for scalability from the beginning, especially in terms of database architecture and API design, would have made it easier to handle increased traffic as the user base grows.
Advice for Others
-
Start with a Clear Vision: Define the core purpose of your project and the problems it aims to solve. This clarity will guide your development process and help you stay focused.
-
Prioritize User Experience: Always keep the end-user in mind. Regularly gather feedback and iterate on your design and features based on user needs.
-
Invest in Testing: Don’t underestimate the importance of testing. A well-tested application is more reliable and easier to maintain, ultimately saving time and resources in the long run.
-
Leverage Open Source: Utilize existing libraries and frameworks to speed up development. Don’t reinvent the wheel; instead, build on the shoulders of giants.
-
Engage with the Community: Foster a community around your project. Engaging with users and contributors can provide valuable insights and help create a loyal user base.
What’s Next?
Conclusion: The Future of BetaBuzz
Project Development Analytics
timeline gant
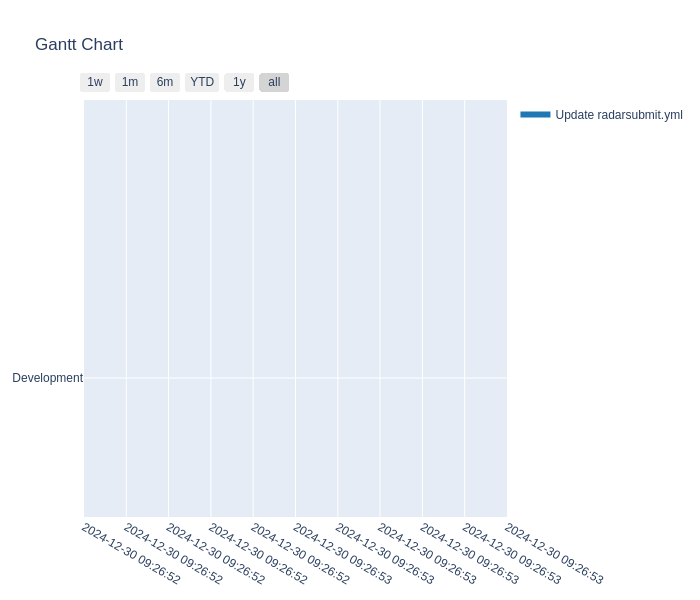
Commit Activity Heatmap
Contributor Network
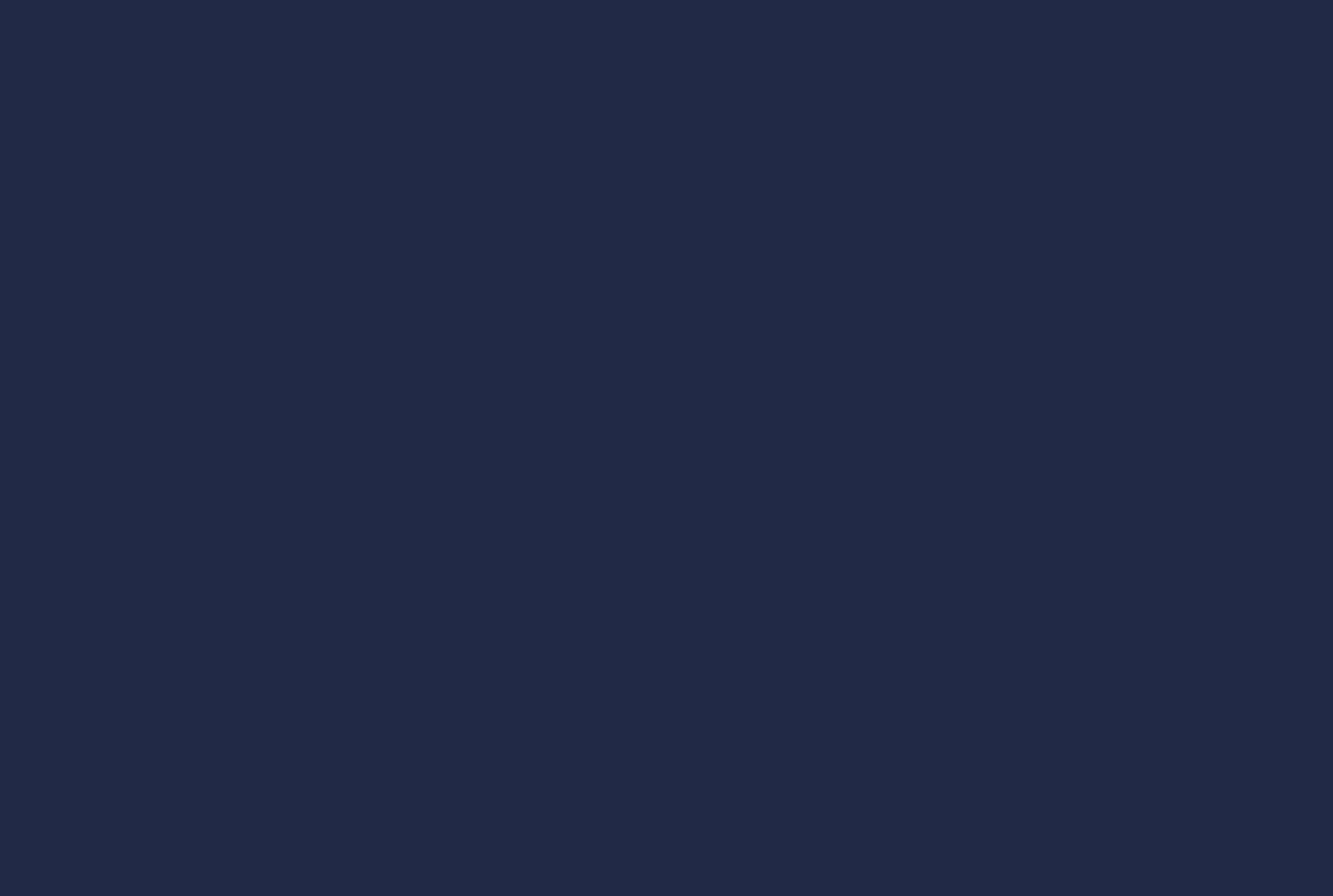
Commit Activity Patterns
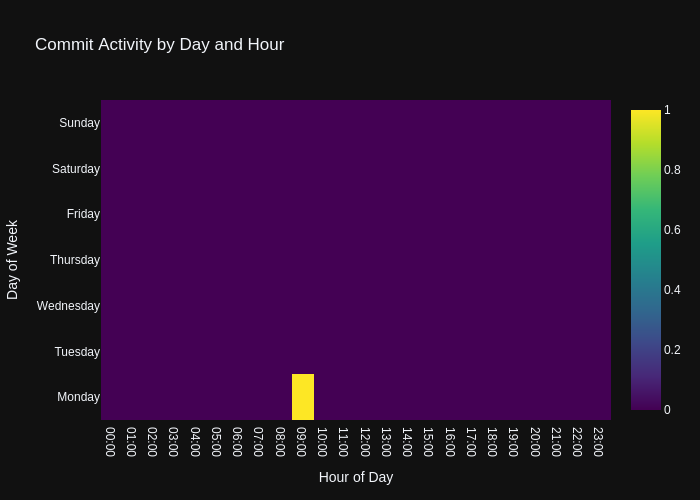
Code Frequency
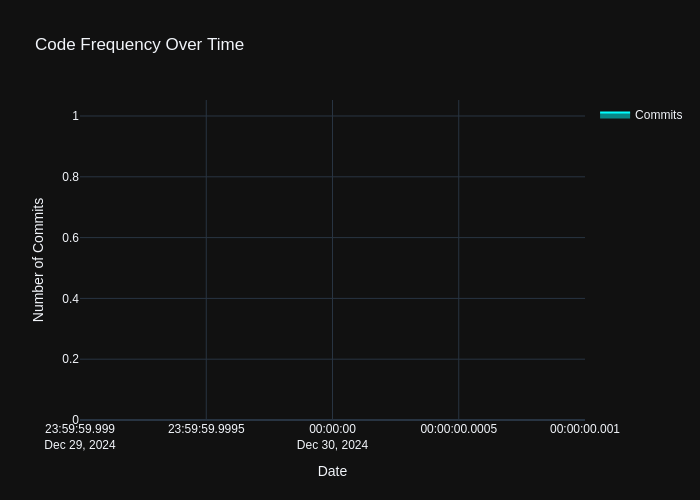
- Repository URL: https://github.com/wanghaisheng/betabuzz
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日