Building Bazaar Bridge: Crafting a Scalable E-Commerce Experience
Project Genesis
Unveiling Bazaar Bridge: My Journey into E-Commerce Development
From Idea to Implementation
E-Commerce Application Development Journey
1. Initial Research and Planning
- User Interviews: Engaging potential users, vendors, and administrators to gather insights on their needs and expectations from an e-commerce platform.
- Competitor Analysis: Studying successful e-commerce applications to understand their strengths and weaknesses, which helped in defining unique selling propositions for our application.
- Feature Prioritization: Based on the research findings, a list of essential features was created, focusing on user experience, vendor management, and administrative controls. This led to the identification of key roles (Admin, Vendor, Customer) and their respective functionalities.
2. Technical Decisions and Their Rationale
- Frontend Framework: React was chosen for its component-based architecture, allowing for reusable UI components and efficient rendering. This decision was influenced by the need for a dynamic and responsive user interface.
- State Management: The use of Redux Toolkit was selected for state management due to its simplicity and powerful features, such as built-in middleware and a structured approach to managing application state.
- Styling: Tailwind CSS was adopted for its utility-first approach, enabling rapid styling without the need for extensive custom CSS. This choice facilitated a consistent design language across the application.
- Backend Integration: The decision to use JWT for authentication was made to ensure secure and stateless user sessions, which is crucial for an e-commerce platform handling sensitive user data.
3. Alternative Approaches Considered
- Framework Alternatives: While React was ultimately chosen, other frameworks like Angular and Vue.js were evaluated. However, React’s flexibility and large community support made it the preferred choice.
- State Management Options: Alternatives like MobX and Context API were considered for state management. However, Redux Toolkit’s structured approach and ease of use for complex state scenarios led to its selection.
- Styling Approaches: CSS-in-JS libraries like Styled Components were considered, but Tailwind CSS’s utility-first approach was favored for its speed and ease of use in prototyping.
4. Key Insights That Shaped the Project
- User-Centric Design: The importance of a seamless user experience became evident early on. Features like infinite scrolling, product comparisons, and responsive design were prioritized to enhance user engagement and satisfaction.
- Vendor Empowerment: Enabling vendors to manage their shops effectively was a crucial insight. Features like order history, product management, and customer interaction tools were designed to empower vendors and improve their experience on the platform.
- Scalability and Performance: As the project progressed, the need for a scalable architecture became clear. Implementing paginated APIs and optimizing the frontend for performance were essential to ensure the application could handle increased traffic and data load.
- Security Considerations: The significance of robust security measures, particularly around authentication and payment processing, was underscored. The decision to use JWT for secure access and integrate trusted payment gateways was pivotal in building user trust.
Under the Hood
Technical Deep-Dive: E-Commerce Application
1. Architecture Decisions
Key Architectural Choices:
- Microservices: The application is divided into three main services: Backend, Frontend, and Dashboard. This allows for independent scaling and deployment.
- RESTful API: The backend exposes a RESTful API for communication with the frontend and dashboard, ensuring a clear separation of concerns and enabling easy integration with third-party services.
- JWT Authentication: JSON Web Tokens (JWT) are used for secure authentication and authorization, allowing users to maintain sessions without server-side state management.
2. Key Technologies Used
Frontend:
- React: A JavaScript library for building user interfaces, enabling the creation of reusable UI components.
- Redux: For state management, allowing for a predictable state container that helps manage the application state across components.
- Tailwind CSS: A utility-first CSS framework that enables rapid UI development with a focus on responsiveness and customization.
- Vite: A build tool that provides a fast development environment and optimized production builds.
Backend:
- Node.js: A JavaScript runtime for building scalable network applications, allowing the use of JavaScript on the server side.
- Express.js: A web application framework for Node.js, simplifying the creation of RESTful APIs.
- MongoDB: A NoSQL database used for storing user, product, and transaction data, providing flexibility in data modeling.
Other Technologies:
- Stripe/Aamarpay: For payment processing, integrating secure payment gateways to handle transactions.
- Zod: A TypeScript-first schema declaration and validation library, used for form validation and ensuring data integrity.
3. Interesting Implementation Details
State Management with Redux
import { createSlice } from '@reduxjs/toolkit';
const cartSlice = createSlice({
name: 'cart',
initialState: {
items: [],
totalAmount: 0,
},
reducers: {
addItem(state, action) {
const newItem = action.payload;
state.items.push(newItem);
state.totalAmount += newItem.price;
},
removeItem(state, action) {
const id = action.payload;
const existingItem = state.items.find(item => item.id === id);
if (existingItem) {
state.items = state.items.filter(item => item.id !== id);
state.totalAmount -= existingItem.price;
}
},
},
});
export const { addItem, removeItem } = cartSlice.actions;
export default cartSlice.reducer;
Responsive Design with Tailwind CSS
<div class="max-w-sm rounded overflow-hidden shadow-lg m-4">
<img class="w-full" src="product-image.jpg" alt="Product Image">
<div class="px-6 py-4">
<div class="font-bold text-xl mb-2">Product Name</div>
<p class="text-gray-700 text-base">Product description goes here.</p>
</div>
<div class="px-6 pt-4 pb-2">
<span class="inline-block bg-gray-200 rounded-full px-3 py-1 text-sm font-semibold text-gray-700 mr-2 mb-2">Category</span>
<span class="inline-block bg-gray-200 rounded-full px-3 py-1 text-sm font-semibold text-gray-700">Price: $XX.XX</span>
</div>
</div>
4. Technical Challenges Overcome
Handling Cart Functionality
if (currentVendor !== newProduct.vendor) {
alert('You can only add products from one vendor at a time.');
}
Authentication and Authorization
Lessons from the Trenches
Key Technical Lessons Learned
-
State Management: Utilizing
@reduxjs/toolkit
andreact-redux
significantly simplified state management across the application. The integration ofredux-persist
helped maintain state even after page refreshes, enhancing user experience. -
Form Handling: Implementing
react-hook-form
for form handling streamlined the process of managing form state and validation. Coupling it withzod
for schema validation ensured robust data integrity. -
Responsive Design: The importance of responsive design was reinforced. Using
tailwindcss
allowed for rapid prototyping and ensured that the application was mobile-friendly, which is crucial for e-commerce platforms. -
API Integration: Understanding how to effectively integrate third-party services (like payment gateways) was vital. This experience highlighted the importance of secure API communication and error handling.
-
Component Reusability: Building reusable components with
MUI
andreact-icons
improved development efficiency and consistency across the application.
What Worked Well
-
Modular Architecture: The separation of concerns between the frontend, backend, and dashboard repositories allowed for easier management and deployment. Each component could be developed and tested independently.
-
User Roles and Permissions: Clearly defined roles (Admin, Vendor, Customer) with specific functionalities made the application intuitive and user-friendly. This structure facilitated easier onboarding for new users.
-
Search and Filter Functionality: The implementation of advanced filtering and search capabilities enhanced the user experience, allowing customers to find products quickly and efficiently.
-
Real-time Updates: Features like infinite scrolling and dynamic product updates kept the user engaged and improved the overall shopping experience.
What You’d Do Differently
-
Enhanced Testing: While the application is functional, implementing more comprehensive unit and integration tests would improve reliability. Using tools like Jest and React Testing Library could help catch bugs early in the development process.
-
Error Handling: Improving error handling, especially for API calls, would enhance user experience. Providing users with clear feedback on errors (e.g., network issues, validation errors) is crucial.
-
Documentation: While the README is informative, more detailed documentation on the backend API endpoints and their expected responses would facilitate easier integration and usage for future developers.
-
Performance Optimization: Conducting performance audits and optimizing loading times, especially for images and large datasets, would enhance the overall user experience.
Advice for Others
-
Plan Your Architecture: Before diving into coding, spend time planning the architecture of your application. A well-thought-out structure can save time and effort in the long run.
-
Focus on User Experience: Always prioritize user experience. Features like responsive design, intuitive navigation, and quick load times can significantly impact user satisfaction and retention.
-
Leverage Community Resources: Don’t hesitate to use community resources and libraries. They can save time and provide solutions that you might not have considered.
-
Iterate Based on Feedback: After launching, gather user feedback and be prepared to iterate on your application. Continuous improvement based on real user experiences is key to success.
-
Stay Updated: The tech landscape is always evolving. Stay updated with the latest trends and best practices in web development to keep your skills sharp and your application competitive.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
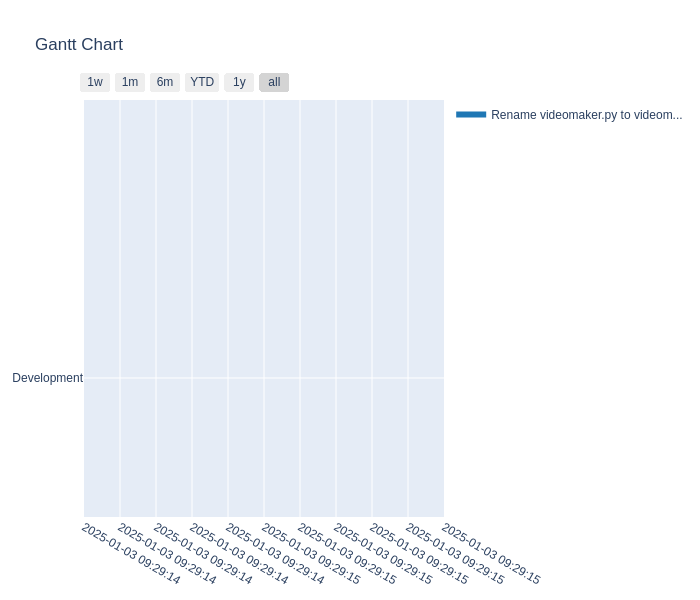
Commit Activity Heatmap
Contributor Network
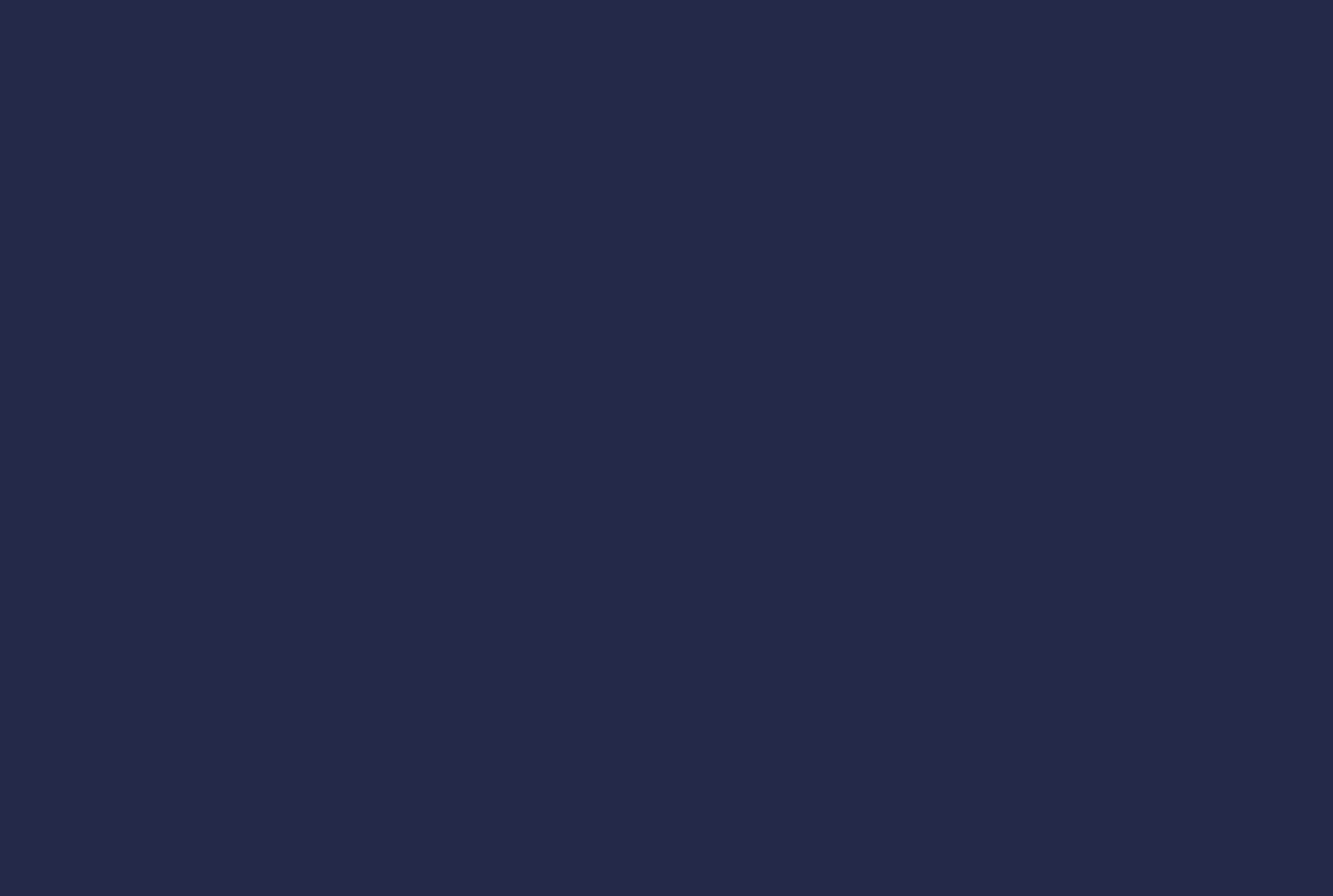
Commit Activity Patterns
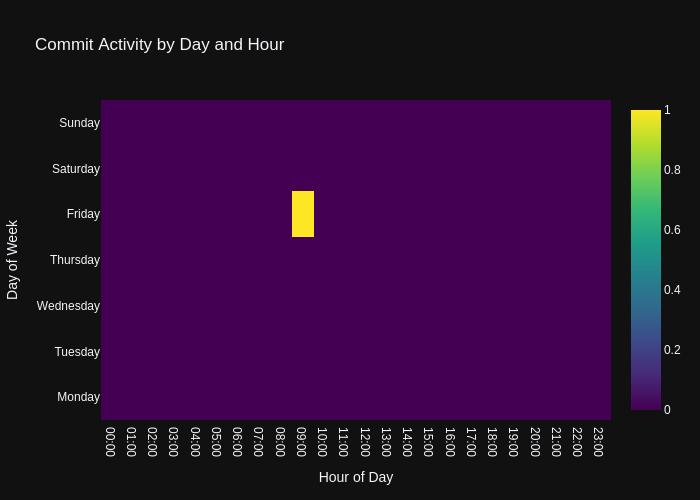
Code Frequency
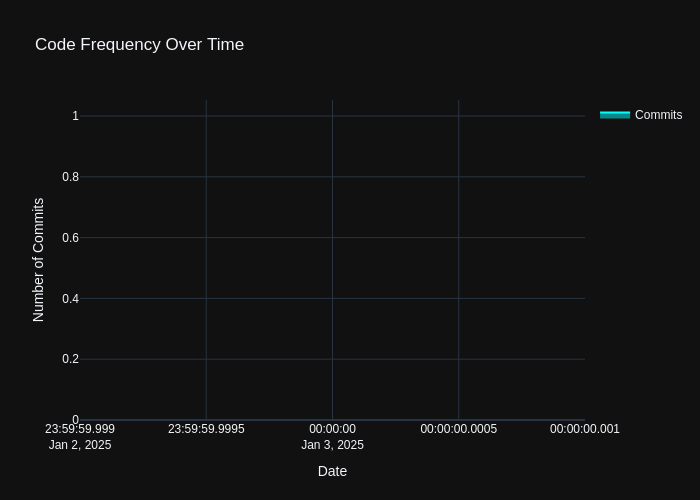
- Repository URL: https://github.com/wanghaisheng/bazaar-bridge-frontend
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日