Building Backlink-Building Submit Agent: A Playwright-Powered Side Project
Project Genesis
Unlocking the Power of Backlink Building: My Journey with Backlink-Building-Submit-Agent
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Cross-Browser Support: Playwright supports multiple browsers (Chromium, Firefox, and WebKit), allowing for comprehensive testing across different environments. This was crucial for ensuring that the user guide would be relevant to a wide audience.
-
Ease of Use: Playwright’s API is designed to be user-friendly, which would facilitate quicker development and easier maintenance of the automated tests.
-
Rich Features: Playwright offers advanced features such as auto-waiting, capturing screenshots, and generating PDFs, which are essential for creating a visually appealing and informative user guide.
-
Active Community and Support: The Playwright community is vibrant and active, providing ample resources, documentation, and support, which would be beneficial throughout the development process.
3. Alternative Approaches Considered
-
Selenium: Initially, Selenium was a strong contender due to its long-standing reputation and extensive documentation. However, its complexity and slower execution times compared to Playwright led to its dismissal.
-
Cypress: Cypress was also evaluated for its ease of use and real-time testing capabilities. However, its limitation to only Chromium-based browsers made it less suitable for a project that required cross-browser compatibility.
-
Manual Documentation: Another approach considered was creating the user guide manually without automation. However, this would have been time-consuming and prone to human error, especially when updates to the application occurred.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-centric approach became evident early on. Engaging with potential users and gathering feedback helped shape the content and structure of the user guide, ensuring it addressed real-world needs.
-
Iterative Development: Emphasizing an iterative development process allowed for continuous improvement. Regular testing and feedback loops ensured that the documentation remained relevant and accurate as the application evolved.
-
Documentation as a Living Entity: Recognizing that documentation should be treated as a living entity rather than a one-time project was crucial. This insight led to the implementation of a version control system to manage updates and changes effectively.
-
Collaboration and Communication: The project underscored the value of collaboration among team members. Regular meetings and open communication channels facilitated knowledge sharing and problem-solving, ultimately enhancing the quality of the final product.
Under the Hood
Technical Deep-Dive: Playwright Implementation
1. Architecture Decisions
-
Modularity: The project is structured in a modular way, allowing for easy maintenance and scalability. Each module can handle specific functionalities, such as page interactions, data handling, and reporting.
-
Asynchronous Operations: Playwright is built on top of Node.js, which allows for non-blocking I/O operations. This is crucial for web automation tasks where multiple actions can be performed concurrently.
-
Cross-Browser Support: Playwright supports multiple browsers (Chromium, Firefox, and WebKit). The architecture is designed to abstract browser-specific implementations, allowing for a unified API.
Example Architecture Diagram
+-------------------+
| Test Runner |
+-------------------+
|
v
+-------------------+
| Test Scripts |
+-------------------+
|
v
+-------------------+
| Page Objects |
+-------------------+
|
v
+-------------------+
| Browser Context |
+-------------------+
2. Key Technologies Used
-
Playwright: The core library for browser automation, enabling interaction with web pages, handling events, and managing browser contexts.
-
Node.js: The runtime environment that allows for executing JavaScript code server-side, facilitating asynchronous operations.
-
TypeScript: Often used for type safety and better tooling support, enhancing the development experience.
-
Jest/Mocha: Testing frameworks that can be integrated with Playwright for running tests and assertions.
Example Code Snippet
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
3. Interesting Implementation Details
- Page Object Model (POM): This design pattern is often used to encapsulate page-specific actions and elements. Each page of the application can be represented as a class, making the code more organized and reusable.
Example of Page Object Model
class LoginPage {
constructor(page) {
this.page = page;
this.usernameInput = page.locator('#username');
this.passwordInput = page.locator('#password');
this.submitButton = page.locator('#submit');
}
async login(username, password) {
await this.usernameInput.fill(username);
await this.passwordInput.fill(password);
await this.submitButton.click();
}
}
- Network Interception: Playwright allows for intercepting network requests, which can be useful for mocking API responses during tests.
Example of Network Interception
await page.route('**/api/login', (route) => {
route.fulfill({
status: 200,
contentType: 'application/json',
body: JSON.stringify({ success: true }),
});
});
4. Technical Challenges Overcome
-
Handling Asynchronous Behavior: One of the main challenges in browser automation is managing asynchronous operations. Playwright provides built-in support for promises, which simplifies the handling of async code.
-
Cross-Browser Compatibility: Ensuring that tests run consistently across different browsers can be challenging. Playwright’s unified API helps mitigate this issue, but developers must still account for browser-specific behaviors.
-
Dynamic Content Loading: Many modern web applications load content dynamically. Playwright provides methods like
waitForSelector
to handle such scenarios effectively.
Example of Waiting for Dynamic Content
await page.goto('https://example.com');
await page.waitForSelector('.dynamic-content', { timeout: 5000 });
Lessons from the Trenches
1. Key Technical Lessons Learned
- Cross-Browser Testing: Playwright supports multiple browsers (Chromium, Firefox, and WebKit), which allows for comprehensive cross-browser testing. This capability is crucial for ensuring that web applications function correctly across different environments.
- Headless Mode: Utilizing Playwright’s headless mode can significantly speed up tests and reduce resource consumption. However, it’s important to test in both headless and headed modes to catch any UI-related issues.
- Auto-Waiting Mechanism: Playwright automatically waits for elements to be ready before performing actions, which reduces flakiness in tests. Understanding and leveraging this feature can lead to more stable test scripts.
- Network Interception: The ability to intercept and mock network requests is powerful for testing various scenarios without relying on external services. This can help in testing error handling and edge cases effectively.
2. What Worked Well
- Ease of Setup: The initial setup of Playwright is straightforward, with clear documentation and examples. This made it easy to get started with writing tests quickly.
- Rich API: Playwright’s API is rich and intuitive, allowing for complex interactions with web pages (e.g., handling file uploads, drag-and-drop actions) with minimal code.
- Parallel Execution: The ability to run tests in parallel significantly reduced the overall testing time, which is beneficial for continuous integration/continuous deployment (CI/CD) pipelines.
- Community and Support: The active community and extensive documentation provided ample resources for troubleshooting and learning best practices.
3. What You’d Do Differently
- Test Organization: In hindsight, organizing tests into a more modular structure (e.g., using page object models) could improve maintainability and readability, especially as the test suite grows.
- Error Handling: Implementing more robust error handling and logging mechanisms would help in diagnosing issues when tests fail, making it easier to identify the root cause.
- Performance Testing: While Playwright is excellent for functional testing, incorporating performance testing tools alongside it could provide a more comprehensive view of application health.
- Regular Updates: Keeping dependencies and Playwright itself up to date is crucial. Establishing a routine for updating libraries and reviewing breaking changes would help avoid compatibility issues.
4. Advice for Others
- Start Small: Begin with a small set of critical tests to build confidence in the framework before expanding to cover more features. This approach helps in understanding the tool without becoming overwhelmed.
- Leverage Documentation: Make full use of Playwright’s documentation and examples. They provide valuable insights into best practices and advanced features that can enhance your testing strategy.
- Integrate with CI/CD: Integrate Playwright tests into your CI/CD pipeline early on. This ensures that tests are run consistently and helps catch issues before they reach production.
- Focus on Flaky Tests: Pay attention to flaky tests and invest time in diagnosing and fixing them. Flaky tests can undermine trust in your test suite and slow down development.
- Community Engagement: Engage with the Playwright community through forums, GitHub discussions, or social media. Sharing experiences and learning from others can provide new perspectives and solutions to common challenges.
What’s Next?
Conclusion
Project Development Analytics
timeline gant

Commit Activity Heatmap
Contributor Network
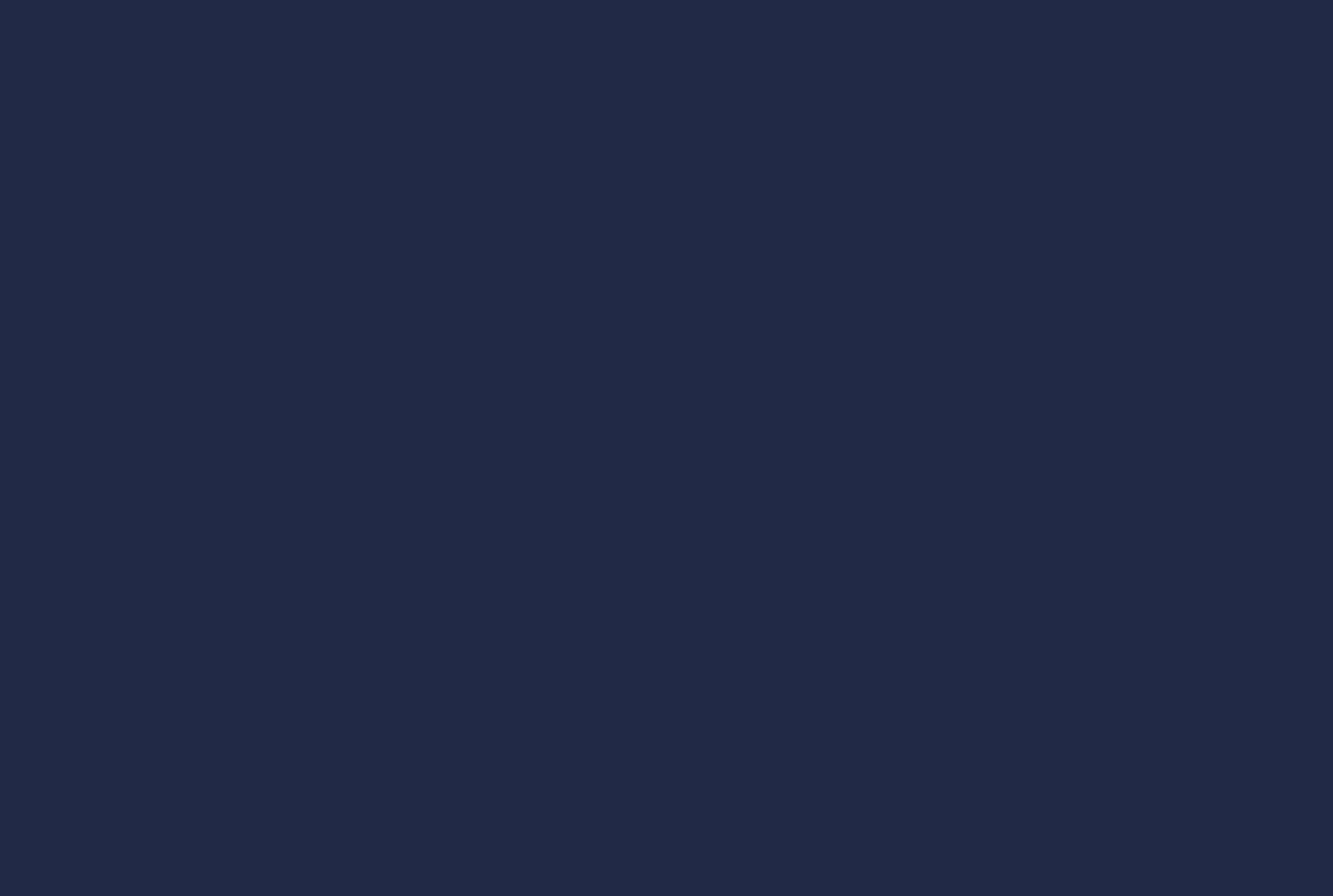
Commit Activity Patterns
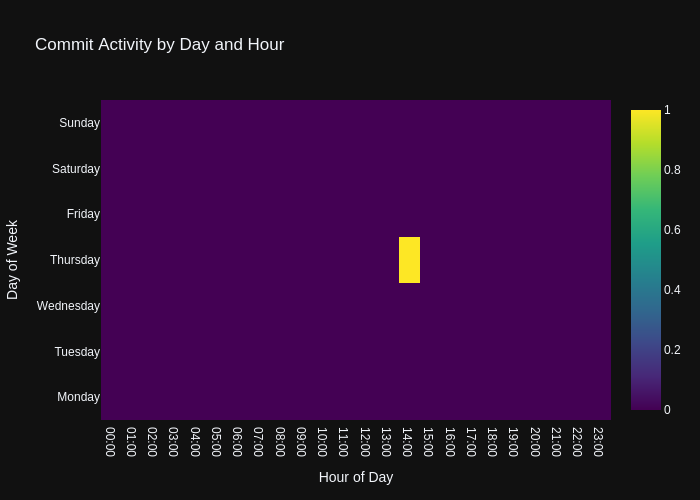
Code Frequency
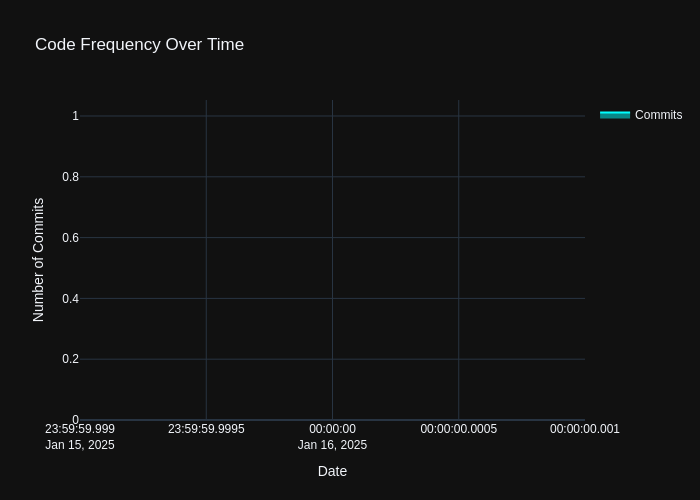
- Repository URL: https://github.com/wanghaisheng/backlink-building-submit-agent
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 20 日