From Idea to Reality: Building background-removal-js for Effortless Image Editing
Project Genesis
Unveiling the Magic of Background Removal in the Browser & Node.js
From Idea to Implementation
1. Initial Research and Planning
@imgly/background-removal
library began with extensive research into the existing solutions for background removal in images. The team analyzed various tools and libraries available in the market, focusing on their capabilities, performance, and user experience. Key considerations included:-
User Needs: Understanding the requirements of developers and end-users was paramount. The team conducted surveys and interviews with potential users, particularly in e-commerce and graphic design sectors, to identify pain points and desired features.
-
Privacy Concerns: With increasing awareness of data privacy, it was crucial to ensure that the solution could operate without sending images to external servers. This led to the decision to develop a library that could run entirely in the browser or Node.js environment.
-
Technical Feasibility: The team evaluated various algorithms for background removal, including traditional image processing techniques and modern machine learning approaches. This research phase helped in identifying the most effective methods that could be implemented efficiently in a JavaScript environment.
2. Technical Decisions and Their Rationale
-
JavaScript and npm Packages: The choice to build the library as an npm package was driven by the popularity of JavaScript in web development. This decision allowed for easy integration into existing projects and a wide reach among developers.
-
In-Browser Processing: To address privacy concerns, the library was designed to perform background removal directly in the browser. This eliminated the need for server-side processing, ensuring that user data remained secure and private.
-
Node.js Compatibility: Recognizing the growing use of Node.js for server-side applications, a separate package (
@imgly/background-removal-node
) was created to allow developers to utilize the same background removal capabilities in their backend services. -
Performance Optimization: The team focused on optimizing the algorithms for speed and efficiency, ensuring that the library could handle real-time background removal without significant lag, which is critical for user experience in applications like e-commerce.
3. Alternative Approaches Considered
-
Server-Side Processing: Initially, the team explored the possibility of a server-side solution where images would be sent to a server for processing. However, this approach was quickly dismissed due to privacy concerns and potential latency issues.
-
Third-Party APIs: The use of third-party APIs for background removal was also considered. While this could have simplified development, it would have introduced dependencies and potential costs, as well as concerns about data privacy.
-
Different Programming Languages: The team evaluated the feasibility of implementing the library in other programming languages, such as Python or C++. However, the decision to focus on JavaScript was made to align with the needs of web developers and the growing popularity of JavaScript frameworks.
4. Key Insights That Shaped the Project
-
User-Centric Design: Engaging with potential users early in the process highlighted the importance of a user-friendly interface and seamless integration. This feedback shaped the design and functionality of the library.
-
Importance of Performance: The need for real-time processing became evident during testing phases. Users expressed frustration with laggy performance, leading to further optimizations in the algorithms.
-
Privacy as a Selling Point: The emphasis on privacy resonated strongly with users, particularly in industries like e-commerce where data security is paramount. This insight reinforced the decision to keep processing client-side.
-
Community Engagement: The team recognized the value of building a community around the library. By encouraging contributions and feedback, they aimed to foster a collaborative environment that would lead to continuous improvement and innovation.
@imgly/background-removal
library was a journey marked by thorough research, thoughtful technical decisions, and a commitment to user needs and privacy. The insights gained throughout the process not only shaped the final product but also laid the groundwork for future enhancements and community engagement.Under the Hood
Technical Deep-Dive: Background Removal in the Browser & Node.js
1. Architecture Decisions
@imgly/background-removal
library is designed to facilitate seamless integration into both browser and Node.js environments. The decision to create two separate npm packages—@imgly/background-removal
for the browser and @imgly/background-removal-node
for Node.js—allows for optimized performance tailored to the specific capabilities and constraints of each environment.Key Architectural Choices:
- Modular Design: By separating the browser and Node.js implementations, the library can leverage the unique features of each environment. For instance, the browser version can utilize Web APIs for image manipulation, while the Node.js version can take advantage of server-side processing capabilities.
- Client-Side Processing: The browser package is designed to perform background removal directly in the client’s browser, minimizing server load and enhancing user privacy by not sending images to a remote server.
- Asynchronous Operations: Both packages utilize asynchronous programming patterns to ensure that image processing does not block the main thread, providing a smooth user experience.
2. Key Technologies Used
- JavaScript: The core language for both packages, enabling cross-platform compatibility.
- HTML5 Canvas: Used in the browser package for rendering images and performing pixel manipulation, which is essential for background removal.
- Node.js: The server-side environment for the Node.js package, allowing for image processing in a scalable manner.
- npm: The package manager used for distributing the library, making it easy for developers to integrate it into their projects.
Example of Using HTML5 Canvas:
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
const image = new Image();
image.src = 'path/to/image.jpg';
image.onload = () => {
canvas.width = image.width;
canvas.height = image.height;
ctx.drawImage(image, 0, 0);
// Further processing for background removal
};
3. Interesting Implementation Details
Background Removal Algorithm
- Color Thresholding: This technique can be used to identify pixels that match the background color and make them transparent.
- Edge Detection: Algorithms like Canny or Sobel can help in identifying the edges of the foreground object, allowing for more precise background removal.
Example of Color Thresholding:
function removeBackground(imageData) {
const data = imageData.data;
for (let i = 0; i < data.length; i += 4) {
// Simple thresholding logic
if (data[i] > 200 && data[i + 1] > 200 && data[i + 2] > 200) {
data[i + 3] = 0; // Set alpha to 0 (transparent)
}
}
return imageData;
}
4. Technical Challenges Overcome
Performance Optimization
- Web Workers: For the browser version, using Web Workers can offload processing to a separate thread, preventing UI blocking.
- Image Data Caching: Caching processed images can reduce the need for repeated calculations, improving performance in applications that require multiple background removals.
Handling Diverse Image Formats
canvas
or sharp
in Node.js to handle different formats effectively.Example of Using Web Workers:
const worker = new Worker('backgroundRemovalWorker.js');
worker.postMessage(imageData);
worker.onmessage = (event) => {
const processedImageData = event.data;
// Use the processed image data
};
Conclusion
@imgly/background-removal
library is a robust solution for developers looking to implement background removal in their applications. By leveraging modern web technologies and optimizing for both browser and Node.js environments, it provides a powerful tool for a variety of use cases, from e-commerce to graphic design. The architectural decisions, key technologies, and implementation details discussed here highlight the library’s capabilities and the challenges it addresses, making it a valuable asset for developers.Lessons from the Trenches
1. Key Technical Lessons Learned
- Performance Optimization: One of the most significant lessons was the importance of optimizing the background removal algorithms for both browser and Node.js environments. Ensuring that the library performs efficiently across different devices and browsers required extensive testing and profiling.
- Cross-Compatibility: Developing a solution that works seamlessly in both browser and Node.js environments highlighted the need for careful consideration of dependencies and APIs. This experience reinforced the importance of maintaining a consistent API while accommodating the unique constraints of each environment.
- User Privacy: Emphasizing privacy in the design of the library was crucial. By processing images locally without sending data to external servers, we learned that user trust can be significantly enhanced, which is a strong selling point for developers and end-users alike.
2. What Worked Well
- Interactive Demo: The inclusion of an interactive demo was a great success. It allowed potential users to experience the library’s capabilities firsthand, leading to increased interest and engagement. This hands-on approach proved effective in demonstrating the library’s value.
- Community Engagement: Actively engaging with the developer community through GitHub issues and discussions helped us gather valuable feedback and foster a sense of community around the project. This engagement led to improvements and new feature requests that aligned with user needs.
- Clear Documentation: Providing comprehensive and clear documentation made it easier for developers to integrate the library into their projects. This included usage examples, API references, and troubleshooting tips, which contributed to a smoother onboarding experience.
3. What You’d Do Differently
- Early User Testing: In hindsight, involving users earlier in the development process could have provided insights that would have shaped the library’s features and usability. Conducting user testing sessions during the beta phase would have helped identify pain points and areas for improvement sooner.
- Modular Architecture: While the library is functional, adopting a more modular architecture from the start could have facilitated easier updates and the addition of new features. This would allow developers to use only the components they need, improving performance and reducing bundle sizes.
- Marketing Strategy: A more proactive marketing strategy could have been implemented to reach a broader audience. Collaborating with influencers in the developer community or creating tutorial content could have increased visibility and adoption rates.
4. Advice for Others
- Focus on User Experience: Always prioritize user experience in your library or tool. Ensure that the integration process is as seamless as possible, and provide clear examples and documentation to help users get started quickly.
- Iterate Based on Feedback: Be open to feedback and willing to iterate on your product. Engaging with your user base can lead to valuable insights that improve your offering and foster loyalty.
- Consider Privacy from the Start: In today’s digital landscape, privacy is paramount. Design your applications with user privacy in mind from the outset, as this can be a significant differentiator in a crowded market.
- Build a Community: Cultivating a community around your project can lead to organic growth and support. Encourage contributions, provide clear guidelines for participation, and recognize the efforts of contributors to foster a collaborative environment.
What’s Next?
Conclusion
@imgly/background-removal
project is currently thriving, providing developers with a robust and efficient solution for background removal in both browser and Node.js environments. Our library has gained traction among users, and we are excited to see the innovative applications being built with it.@imgly/background-removal
library. Whether you have ideas for new features, improvements, or simply want to help with documentation, your contributions can make a significant impact. Join our community, and let’s collaborate to make this project even better!Project Development Analytics
timeline gant
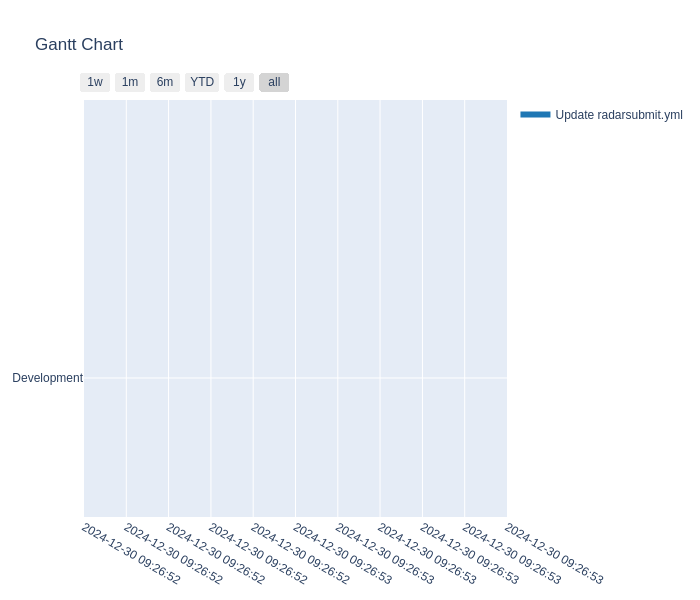
Commit Activity Heatmap
Contributor Network
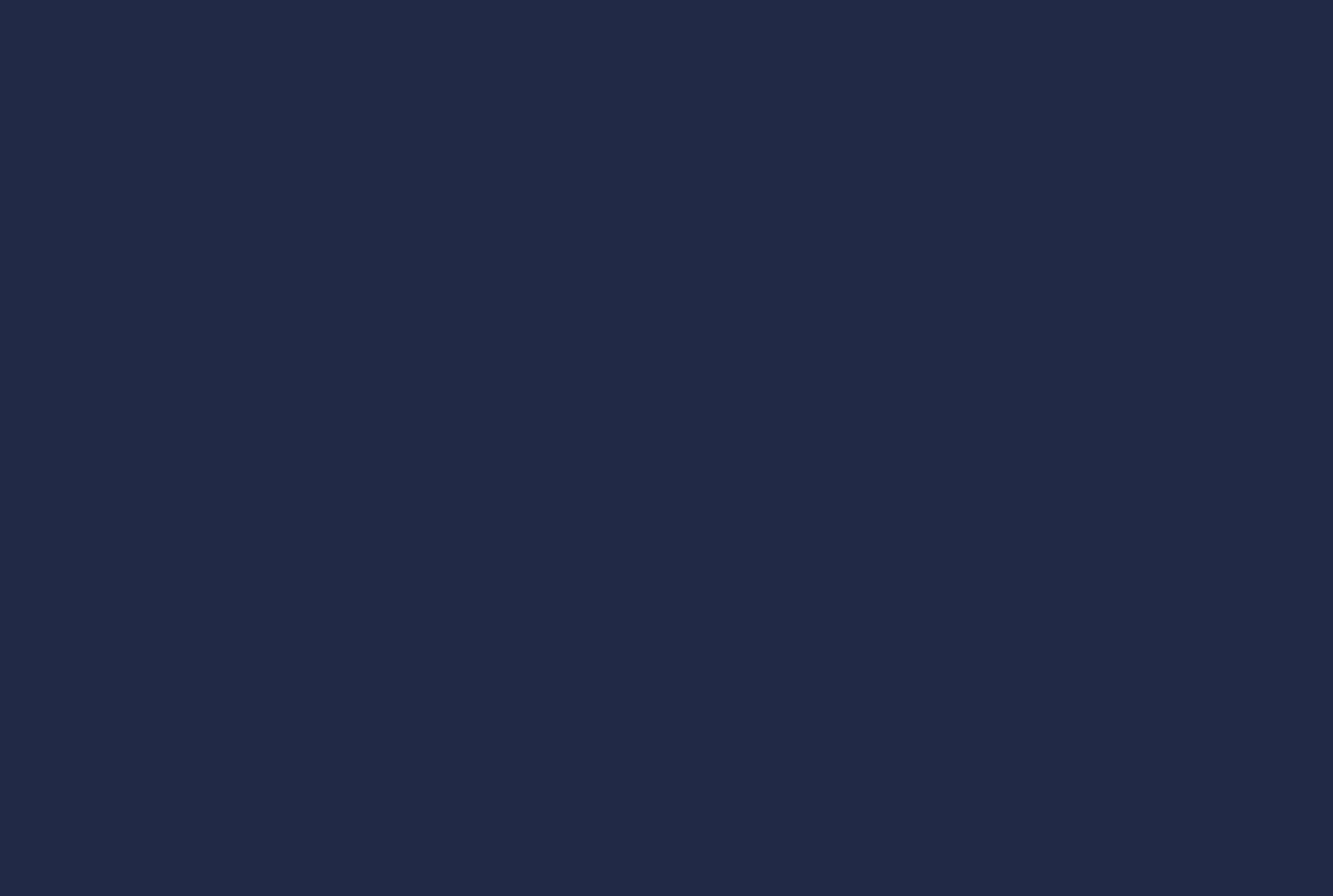
Commit Activity Patterns
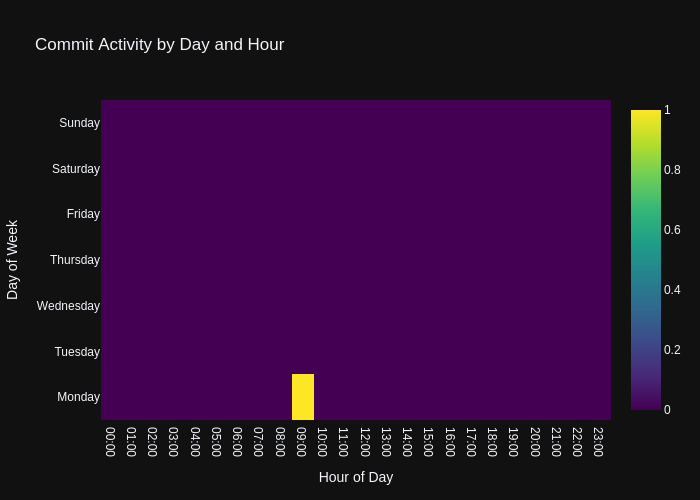
Code Frequency
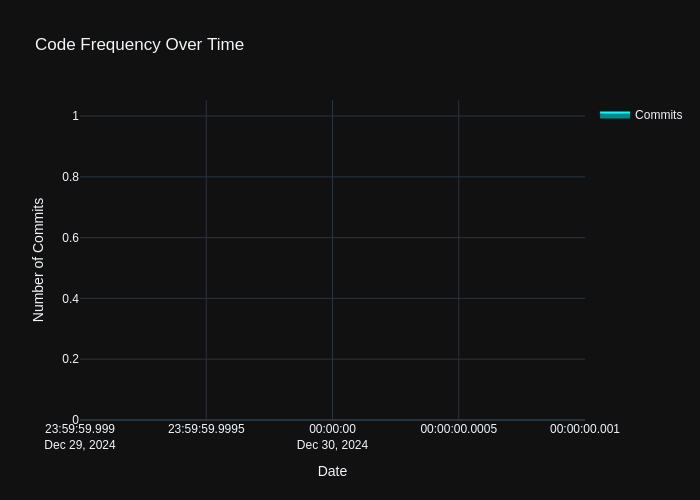
- Repository URL: https://github.com/wanghaisheng/background-removal-js
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日