Transforming Images: Building the Background Change Demo with React & Flask
Project Genesis
Transforming Images with a Click: My Journey into Background Change API Development
From Idea to Implementation
Journey from Concept to Code: Background Change API
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became clear early on. Users expressed a desire for intuitive controls and clear instructions, which led us to prioritize usability in our design.
-
Iterative Development: Emphasizing an iterative development approach allowed us to gather feedback continuously and make adjustments based on user testing. This flexibility was crucial in refining the API and frontend interface.
-
Security Considerations: As we integrated sensitive credentials into the backend, we recognized the need for robust security measures. This realization prompted us to implement environment variables for sensitive data and to educate users on best practices for keeping their credentials secure.
-
Real-Time Processing: The necessity for real-time processing capabilities became apparent as we tested the API. Users expected immediate feedback when changing backgrounds, which led us to optimize our API for performance.
Under the Hood
Technical Deep-Dive: Background Change API
1. Architecture Decisions
Key Architectural Choices:
- Separation of Concerns: The frontend and backend are decoupled, allowing for independent development and deployment. This separation also enhances maintainability and scalability.
- Webhook Integration: Utilizing webhooks allows for real-time communication between the frontend and backend, enabling asynchronous processing of image changes.
- Use of ngrok: Ngrok is employed to expose the local backend server to the internet, facilitating webhook testing and integration without the need for a public server.
2. Key Technologies Used
Frontend:
- React: A JavaScript library for building user interfaces, chosen for its component-based architecture and efficient rendering.
- Node.js: The runtime environment for executing JavaScript on the server side, enabling the use of npm for package management.
Backend:
- Flask: A lightweight Python web framework, selected for its simplicity and flexibility in building RESTful APIs.
- Python: The programming language used for backend development, chosen for its rich ecosystem and libraries for image processing.
Additional Tools:
- ngrok: A tool for creating secure tunnels to localhost, allowing for easy testing of webhooks.
- CORS: Cross-Origin Resource Sharing is configured to allow the frontend to communicate with the backend securely.
3. Interesting Implementation Details
Frontend Configuration
src/App.tsx
:webhookUrl: "https://your-ngrok-url.ngrok-free.app/api/webhook";
Payload Structure
{
"canvas_size": "3840x3840",
"template_url": "https://example.com/template.png",
"origin_img": "https://example.com/origin.png",
"overlay_template_x": 5,
"overlay_template_y": 5
}
Flask API Endpoint
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/api/webhook', methods=['POST'])
def handle_webhook():
data = request.json
# Process the image change based on the received data
return jsonify({"status": "success"})
4. Technical Challenges Overcome
CORS Configuration
from flask_cors import CORS
app = Flask(__name__)
CORS(app, resources={r"/api/*": {"origins": "*"}})
Ngrok Connectivity
Image Processing Performance
Security Considerations
.env
file to store CLIENT_ID
and CLIENT_SECRET
ensures that these values are not hard-coded into the application, reducing the risk of exposure..env
file:CLIENT_ID=your_client_id
CLIENT_SECRET=your_client_secret
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
-
Environment Management: Using
nvm
for Node.js and virtual environments for Python helped maintain clean and isolated environments. This practice prevents dependency conflicts and ensures that the correct versions of libraries are used. -
Webhook Integration: Setting up ngrok for webhook testing was crucial. It allowed for real-time testing of the backend without deploying to a live server. Understanding how to configure and authenticate ngrok was essential for smooth operation.
-
CORS Configuration: Handling Cross-Origin Resource Sharing (CORS) was a significant learning point. Properly configuring CORS on the Flask backend was necessary to allow the frontend to communicate with it without issues.
-
Environment Variables: The importance of using a
.env
file for sensitive information (like API keys) was reinforced. This practice enhances security and keeps sensitive data out of version control.
2. What Worked Well
-
Seamless Integration: The combination of React for the frontend and Flask for the backend worked well. The separation of concerns allowed for a clean architecture where each part could be developed and tested independently.
-
User-Friendly Setup: The step-by-step installation and setup instructions in the README made it easy for new developers to get started quickly. Clear prerequisites and commands helped reduce setup time.
-
Dynamic Webhook URL: The ability to dynamically update the webhook URL in the frontend based on the ngrok URL made testing more flexible and efficient.
3. What You’d Do Differently
-
Persistent ngrok URL: If budget allows, consider using a paid ngrok plan to obtain a persistent URL. This would eliminate the need to update the frontend configuration every time ngrok restarts, streamlining the development process.
-
Enhanced Error Handling: Implement more robust error handling in both the frontend and backend. Providing clear error messages and handling edge cases would improve user experience and make debugging easier.
-
Automated Testing: Introduce automated testing for both the frontend and backend. This would help catch issues early in the development process and ensure that changes do not break existing functionality.
4. Advice for Others
-
Document Everything: Keep documentation up to date, especially when making changes to the setup or configuration. This will save time for both current and future developers.
-
Use Version Control Wisely: Always use
.gitignore
to exclude sensitive files like.env
from version control. This practice helps prevent accidental exposure of sensitive information. -
Stay Updated: Regularly check for updates to dependencies and tools (like Node.js, Flask, and ngrok). Keeping everything up to date can help avoid security vulnerabilities and compatibility issues.
-
Engage with the Community: If you encounter issues, don’t hesitate to seek help from the community. Platforms like Stack Overflow or GitHub discussions can provide valuable insights and solutions.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
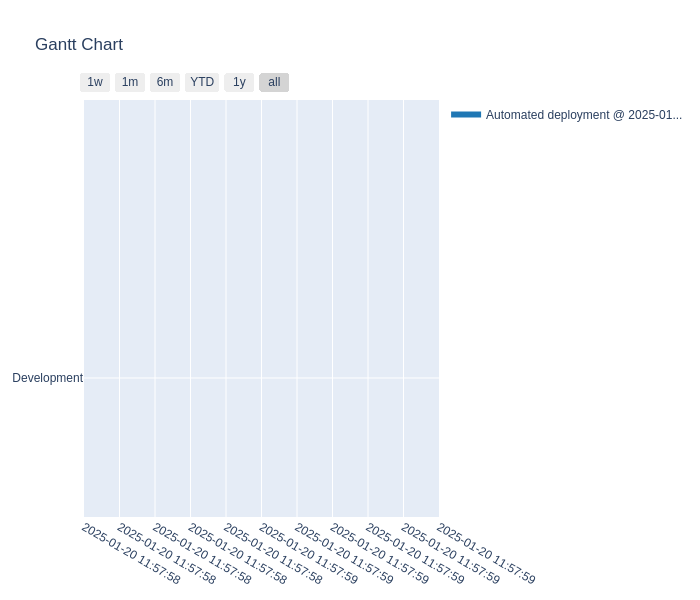
Commit Activity Heatmap
Contributor Network
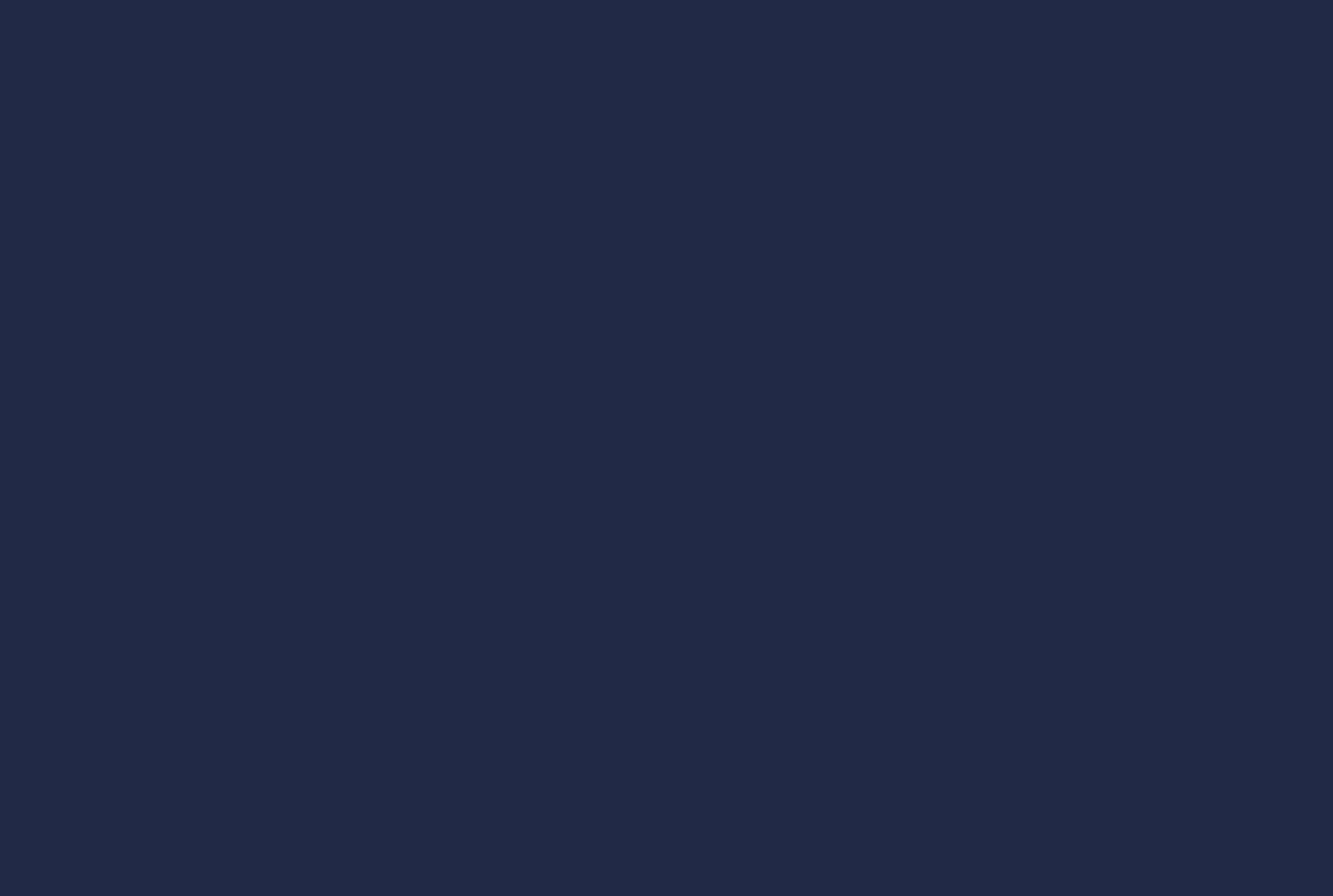
Commit Activity Patterns
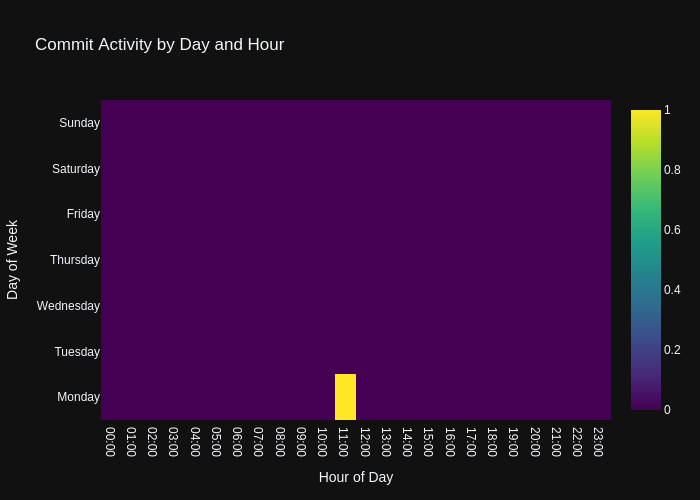
Code Frequency
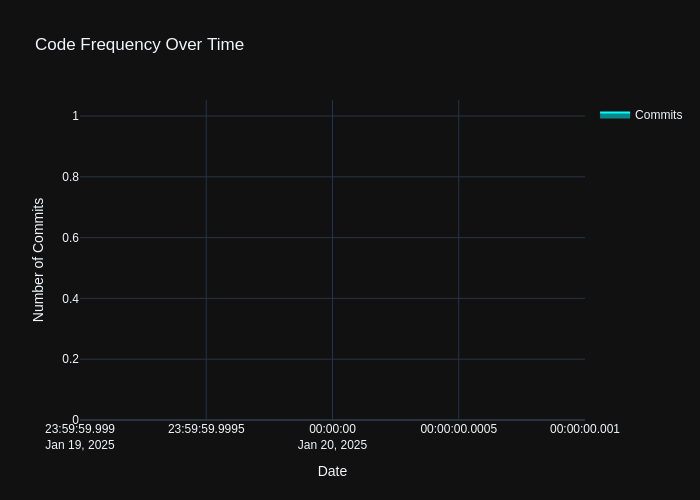
- Repository URL: https://github.com/wanghaisheng/background-change-demo
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日