Weekend Hack: Building Automation Jobs with a Browser Extension
Project Genesis
Unlocking Efficiency: My Journey into Automation with Browser Extensions
From Idea to Implementation
1. Initial Research and Planning
- User Experience: Understanding how users currently automate tasks and what challenges they face.
- YAML Familiarity: Evaluating the level of familiarity users have with YAML and how it can be leveraged for automation.
- Integration Needs: Identifying the systems and tools that users commonly work with and how the new solution could integrate with them.
2. Technical Decisions and Their Rationale
-
Choice of YAML: YAML was selected as the primary scripting language due to its readability and ease of use. It allows users to define complex configurations in a straightforward manner, making it accessible for both technical and non-technical users.
-
Framework Selection: The decision to use a JavaScript-based framework was driven by the need for a robust and flexible environment that could easily integrate with web technologies. This choice also allowed for leveraging existing libraries and tools within the JavaScript ecosystem.
-
Modular Architecture: The project adopted a modular architecture to facilitate scalability and maintainability. This decision enables developers to add new features or modify existing ones without disrupting the overall system.
-
User-Centric Design: Emphasizing a user-centric design approach ensured that the interface was intuitive and aligned with user workflows. This involved iterative prototyping and user testing to refine the user experience.
3. Alternative Approaches Considered
-
Using JSON Instead of YAML: While JSON is widely used for configuration files, it was ultimately deemed less user-friendly for the target audience. The decision to stick with YAML was reinforced by user feedback highlighting its simplicity.
-
Building a Standalone Application: Initially, there was consideration of developing a standalone desktop application. However, this was set aside in favor of a web-based solution to ensure broader accessibility and easier updates.
-
Integration with Existing Automation Tools: The team explored the possibility of integrating with existing automation platforms. However, it was determined that creating a dedicated solution would provide a more tailored experience for users, addressing specific needs that existing tools did not cover.
4. Key Insights That Shaped the Project
-
User Empowerment: The realization that users wanted more control over their automation processes led to the inclusion of features that allow for customization and flexibility in script creation.
-
Community Feedback: Engaging with the community early in the process provided invaluable feedback that shaped the feature set and user interface. This iterative approach ensured that the final product was closely aligned with user expectations.
-
Documentation and Support: The importance of comprehensive documentation became clear as users expressed the need for guidance in using YAML effectively. This insight led to the development of extensive tutorials and examples to support users in their automation journey.
-
Iterative Development: Embracing an agile development methodology allowed the team to adapt to changing requirements and incorporate user feedback continuously, resulting in a more refined and user-friendly product.
Conclusion
Under the Hood
1. Architecture Decisions
- Modularity: The architecture supports the separation of concerns, allowing different components to be developed and maintained independently.
- YAML Scripting: By leveraging YAML, the framework allows users to define automation scripts in a clear and concise manner, making it easier to read and write compared to JSON or XML.
2. Key Technologies Used
- JavaScript: The core language for the framework, enabling dynamic behavior and interaction within web applications.
- YAML: Used for configuration and scripting, allowing for a straightforward way to define automation tasks.
- Node.js: Facilitates server-side execution of scripts, enabling automation tasks to run in a non-blocking manner.
- Express.js: Often used in conjunction with Node.js to create a robust web server for handling requests and serving the automation scripts.
3. Interesting Implementation Details
- YAML Parsing: The framework uses a YAML parser to convert YAML scripts into JavaScript objects. For example:
const yaml = require('js-yaml');
const fs = require('fs');
try {
const doc = yaml.load(fs.readFileSync('script.yaml', 'utf8'));
console.log(doc);
} catch (e) {
console.log(e);
}
- Dynamic Execution: Once the YAML is parsed, the framework dynamically maps the defined tasks to corresponding JavaScript functions, allowing for flexible execution based on user-defined scripts.
4. Technical Challenges Overcome
- Error Handling: Ensuring robust error handling when parsing YAML scripts was crucial. The framework implements try-catch blocks to gracefully handle parsing errors and provide meaningful feedback to users.
try {
const doc = yaml.load(fs.readFileSync('script.yaml', 'utf8'));
} catch (error) {
console.error('Error parsing YAML:', error.message);
}
- Asynchronous Execution: Managing asynchronous operations in Node.js can be challenging. MidScene.js uses Promises and async/await syntax to handle asynchronous tasks defined in YAML scripts, ensuring that tasks are executed in the correct order.
async function executeTask(task) {
try {
await task();
} catch (error) {
console.error('Task execution failed:', error);
}
}
- Extensibility: The framework was designed to be extensible, allowing developers to add custom tasks easily. This was achieved by defining a plugin system where users can register their functions that can be called from YAML scripts.
function registerTask(name, fn) {
tasks[name] = fn;
}
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- YAML for Configuration: Using YAML for scripting and configuration allows for a more human-readable format compared to JSON or XML. This can enhance collaboration among team members who may not be as technically inclined.
- Modular Design: Structuring scripts in a modular way promotes reusability and easier maintenance. Breaking down complex tasks into smaller, manageable scripts can simplify debugging and enhance clarity.
- Error Handling: Implementing robust error handling mechanisms is crucial. This ensures that scripts can fail gracefully and provide meaningful feedback, which is essential for troubleshooting.
- Version Control: Keeping scripts under version control (e.g., using Git) is vital for tracking changes, collaborating with others, and rolling back to previous versions if necessary.
2. What Worked Well
- Community Feedback: Engaging with the community early on helped refine features and identify pain points. This iterative feedback loop led to a more user-friendly product.
- Documentation: Comprehensive documentation, including examples and use cases, facilitated easier onboarding for new users and reduced the learning curve.
- Integration with Existing Tools: The ability to integrate with popular tools and platforms made it easier for users to adopt Midscene.js without overhauling their existing workflows.
3. What You’d Do Differently
- More Extensive Testing: While testing was conducted, a more extensive suite of automated tests could have been implemented to catch edge cases and ensure stability across different environments.
- User Interface Improvements: If applicable, investing more time in creating a user-friendly interface for script management could enhance user experience and accessibility.
- Performance Optimization: Early performance profiling could have identified bottlenecks, allowing for optimizations that would improve the overall efficiency of the scripts.
4. Advice for Others
- Start Small: When developing a new tool or framework, start with a minimal viable product (MVP) that addresses core functionalities. This allows for quicker feedback and iteration.
- Prioritize Documentation: Invest time in creating clear and concise documentation from the beginning. This will save time in the long run and help users adopt the tool more easily.
- Foster a Community: Building a community around your project can provide invaluable support and insights. Encourage contributions and feedback to create a sense of ownership among users.
- Stay Agile: Be prepared to pivot based on user feedback and changing requirements. An agile approach can help you adapt and evolve your project effectively.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
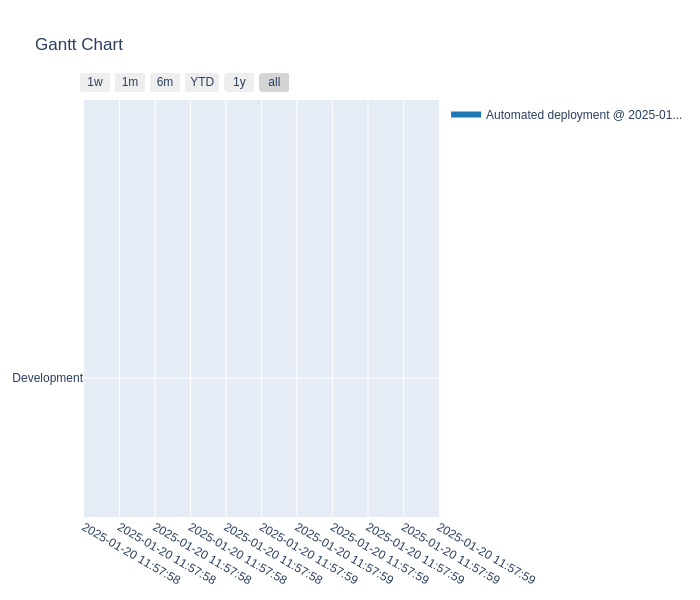
Commit Activity Heatmap
Contributor Network
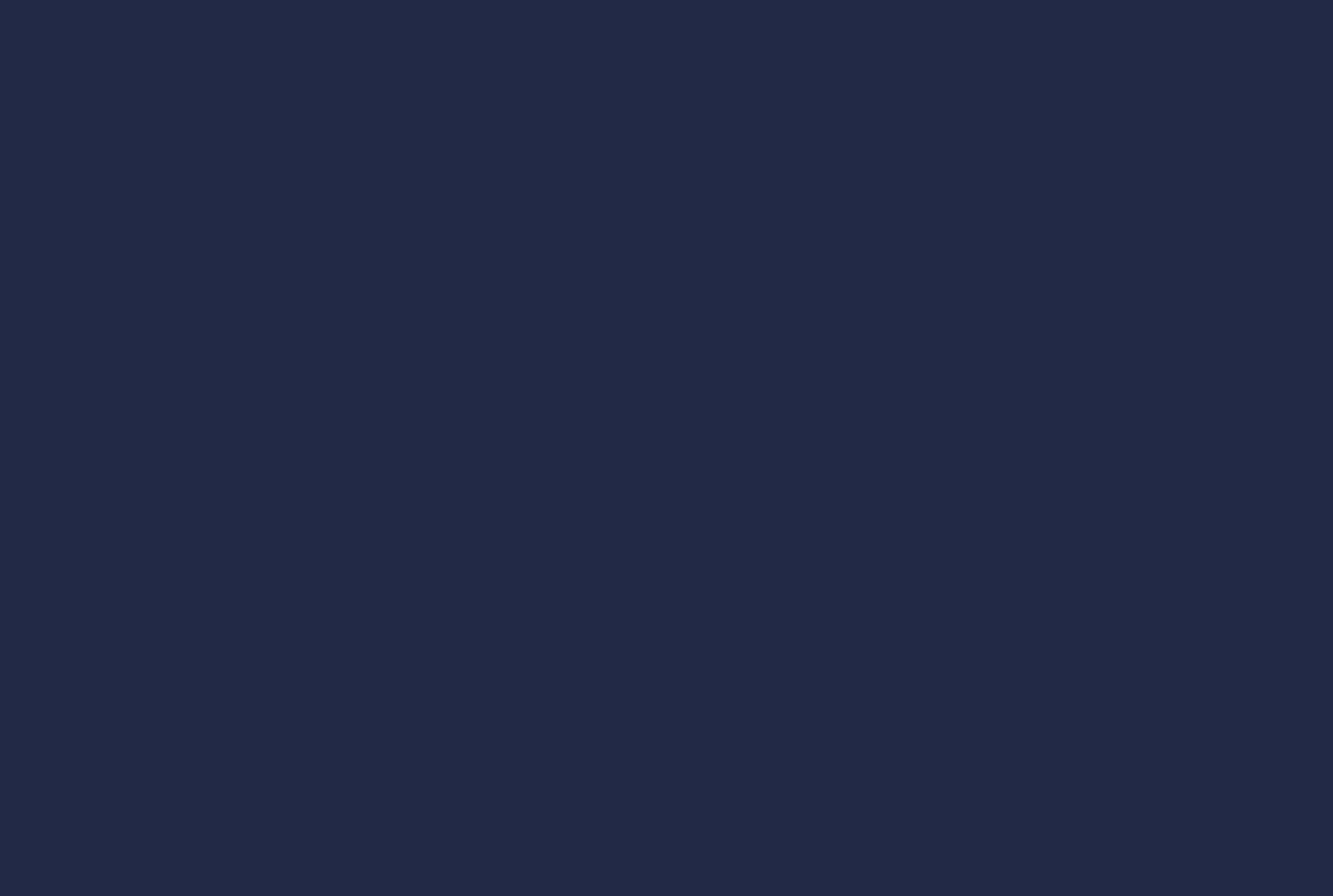
Commit Activity Patterns
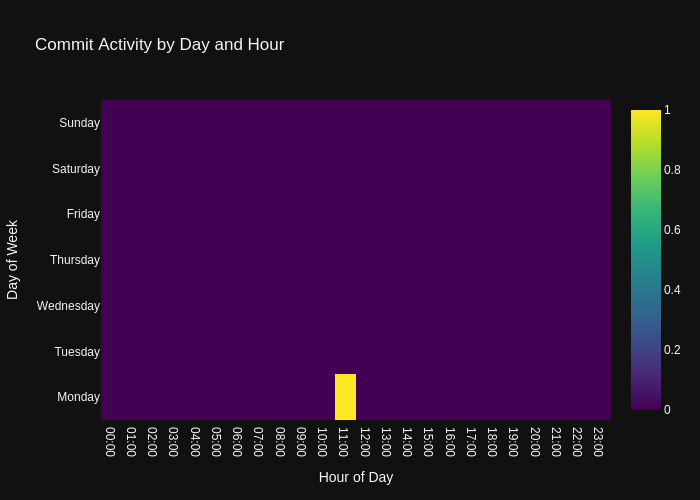
Code Frequency
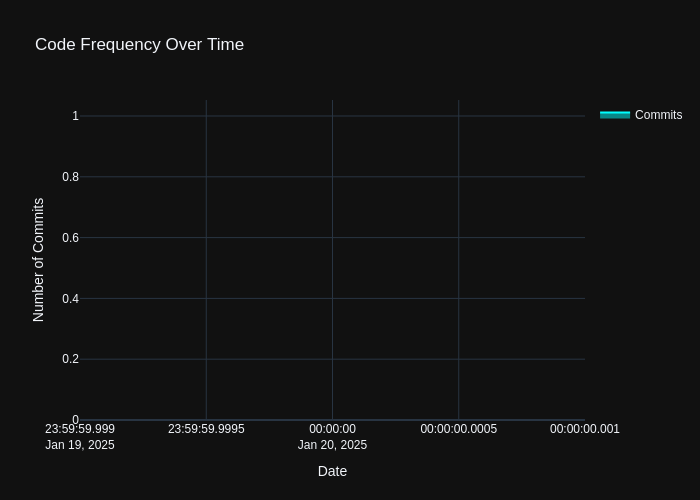
- Repository URL: https://github.com/wanghaisheng/automation-job-with-browser-extension
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日