Building astro-ssr-i18n-params-repro: A StackBlitz JSON API Adventure
Project Genesis
Unraveling the Mysteries of Astro-SSR-I18n-Params-Repro: A Journey of Discovery
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
- Simplicity: JSON is human-readable and easy to manipulate, making it ideal for rapid development and debugging.
- No Setup Overhead: Unlike traditional databases, using JSON files eliminates the need for complex setup and configuration, allowing for faster iteration.
- Flexibility: JSON’s schema-less nature allows for easy modifications to the data structure without requiring migrations or extensive refactoring.
3. Alternative Approaches Considered
- Traditional Relational Database: While robust, the complexity and overhead of setting up a relational database (like MySQL or PostgreSQL) were deemed unnecessary for the project’s scope.
- NoSQL Databases: Options like MongoDB were considered for their flexibility, but the added complexity of managing a database server was not justified for a project focused on simplicity and speed.
- In-memory Data Structures: Using in-memory data structures (like dictionaries in Python) was briefly considered for performance reasons, but this approach would not persist data across server restarts, which was a critical requirement.
4. Key Insights That Shaped the Project
- User-Centric Design: Engaging with potential users early in the process highlighted the importance of usability and clear documentation. This feedback led to a focus on creating intuitive API endpoints and comprehensive usage examples.
- Iterative Development: Emphasizing an iterative development approach allowed for continuous feedback and improvements. This methodology helped identify issues early and adapt the project based on real-world usage.
- Scalability Considerations: While JSON files were suitable for the initial phase, we recognized the potential need for scalability. This insight prompted us to design the API with modularity in mind, allowing for future integration with more robust data storage solutions if necessary.
Under the Hood
Technical Deep-Dive
1. Architecture Decisions
-
Client-Server Architecture: The client (frontend) and server (backend) are separate entities that communicate over HTTP. This separation allows for independent development and scaling.
-
Statelessness: Each API request from the client to the server must contain all the information needed to understand and process the request. This means that the server does not store any client context between requests.
-
Resource-Based: The API is designed around resources, which are represented by URLs. Each resource can be manipulated using standard HTTP methods (GET, POST, PUT, DELETE).
-
JSON as Data Format: JSON (JavaScript Object Notation) is chosen for data interchange due to its lightweight nature and ease of use with JavaScript, making it a popular choice for web applications.
2. Key Technologies Used
-
Node.js: A JavaScript runtime built on Chrome’s V8 engine, ideal for building scalable network applications. It allows for handling multiple requests simultaneously.
-
Express.js: A minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It simplifies the creation of APIs.
-
MongoDB: A NoSQL database that stores data in JSON-like documents. It is well-suited for applications that require high availability and scalability.
-
Postman: A tool for testing APIs, allowing developers to send requests and view responses easily.
3. Interesting Implementation Details
- Routing: Express.js allows for defining routes that correspond to different API endpoints. For example, to create a simple API for managing users, you might have:
const express = require('express');
const app = express();
app.use(express.json()); // Middleware to parse JSON bodies
// Route to get all users
app.get('/api/users', (req, res) => {
// Logic to retrieve users from the database
res.json(users);
});
// Route to create a new user
app.post('/api/users', (req, res) => {
const newUser = req.body; // Assuming the user data is sent in the request body
// Logic to save the new user to the database
res.status(201).json(newUser);
});
- Middleware: Express allows the use of middleware functions to handle requests. For example, you can create a logging middleware to log incoming requests:
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next(); // Pass control to the next middleware
});
- Error Handling: Implementing centralized error handling is crucial for maintaining a clean API. This can be done using middleware:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: 'Internal Server Error' });
});
4. Technical Challenges Overcome
- Handling Asynchronous Operations: Node.js is inherently asynchronous, which can lead to challenges in managing the flow of data. Using Promises or async/await syntax helps in writing cleaner and more manageable asynchronous code.
app.get('/api/users/:id', async (req, res) => {
try {
const user = await User.findById(req.params.id); // Assuming User is a Mongoose model
if (!user) {
return res.status(404).json({ message: 'User not found' });
}
res.json(user);
} catch (error) {
res.status(500).json({ message: 'Error retrieving user' });
}
});
- Data Validation: Ensuring that the data received from clients is valid is crucial. Libraries like Joi or express-validator can be used to validate incoming data before processing it.
const { body, validationResult } = require('express-validator');
app.post('/api/users', [
body('name').isString().notEmpty(),
body('email').isEmail(),
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Proceed with creating the user
});
- CORS Issues: When developing APIs, Cross-Origin Resource Sharing (CORS) can be a challenge. Using the
cors
middleware in Express can help manage these issues effectively.
const cors = require('cors');
app.use(cors()); // Enable CORS for all routes
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Data Structure Design: Properly structuring JSON data is crucial. Nested objects can lead to complex parsing, so keeping the structure flat where possible can simplify data handling.
- Error Handling: Implementing robust error handling is essential. Returning meaningful error messages in JSON format helps clients understand issues quickly.
- Versioning: As the API evolves, versioning the JSON endpoints (e.g.,
/api/v1/resource
) is important to maintain backward compatibility for existing clients. - Security Considerations: Always validate and sanitize incoming JSON data to prevent injection attacks. Implementing authentication and authorization mechanisms is also critical.
- Performance Optimization: Large JSON payloads can slow down performance. Consider pagination for large datasets and use compression (like Gzip) to reduce payload size.
2. What Worked Well
- Simplicity of JSON: JSON’s lightweight nature made it easy to work with across different programming languages and platforms, facilitating quick development and integration.
- Ease of Debugging: Tools like Postman and browser developer tools made it easy to test and debug API endpoints, allowing for rapid iteration.
- Community Support: Leveraging existing libraries and frameworks (like Express.js for Node.js) that handle JSON parsing and response formatting significantly sped up development.
- Clear Documentation: Maintaining clear and concise API documentation helped onboard new developers quickly and reduced the number of support queries.
3. What You’d Do Differently
- More Comprehensive Testing: Implementing a more rigorous testing strategy, including unit tests and integration tests for the API, would have caught issues earlier in the development process.
- Rate Limiting: Introducing rate limiting from the start could have prevented abuse and ensured fair usage of the API resources.
- Monitoring and Analytics: Setting up monitoring tools (like Prometheus or Grafana) earlier would have provided insights into API usage patterns and performance bottlenecks.
- Feedback Loop: Establishing a feedback loop with users to gather insights on API usability and performance could have led to more user-centric improvements.
4. Advice for Others
- Start Simple: Begin with a minimal viable product (MVP) approach. Focus on core functionalities and gradually add features based on user feedback.
- Prioritize Documentation: Invest time in creating comprehensive documentation from the outset. This will save time in the long run and improve collaboration.
- Use Established Frameworks: Don’t reinvent the wheel. Utilize established frameworks and libraries that can handle common tasks, allowing you to focus on unique features.
- Plan for Scalability: Even if your project starts small, design your API with scalability in mind. Consider how you will handle increased load and data growth in the future.
- Engage with the Community: Participate in forums and communities related to your technology stack. Sharing experiences and learning from others can provide valuable insights and support.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
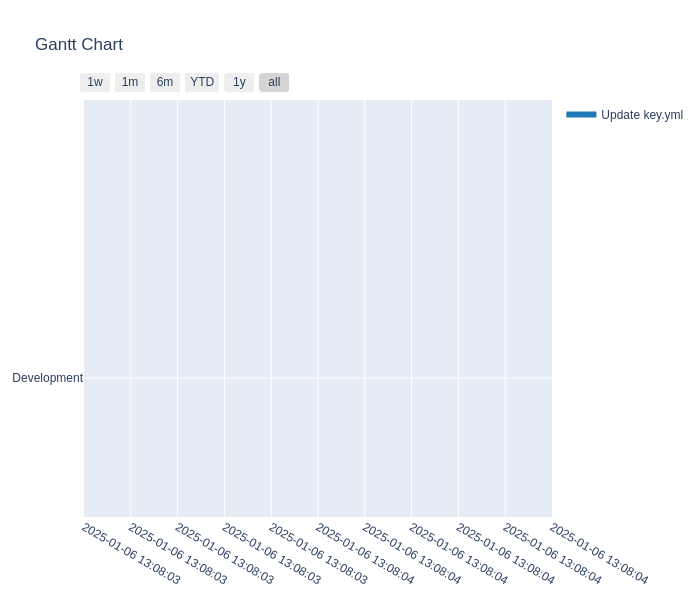
Commit Activity Heatmap
Contributor Network
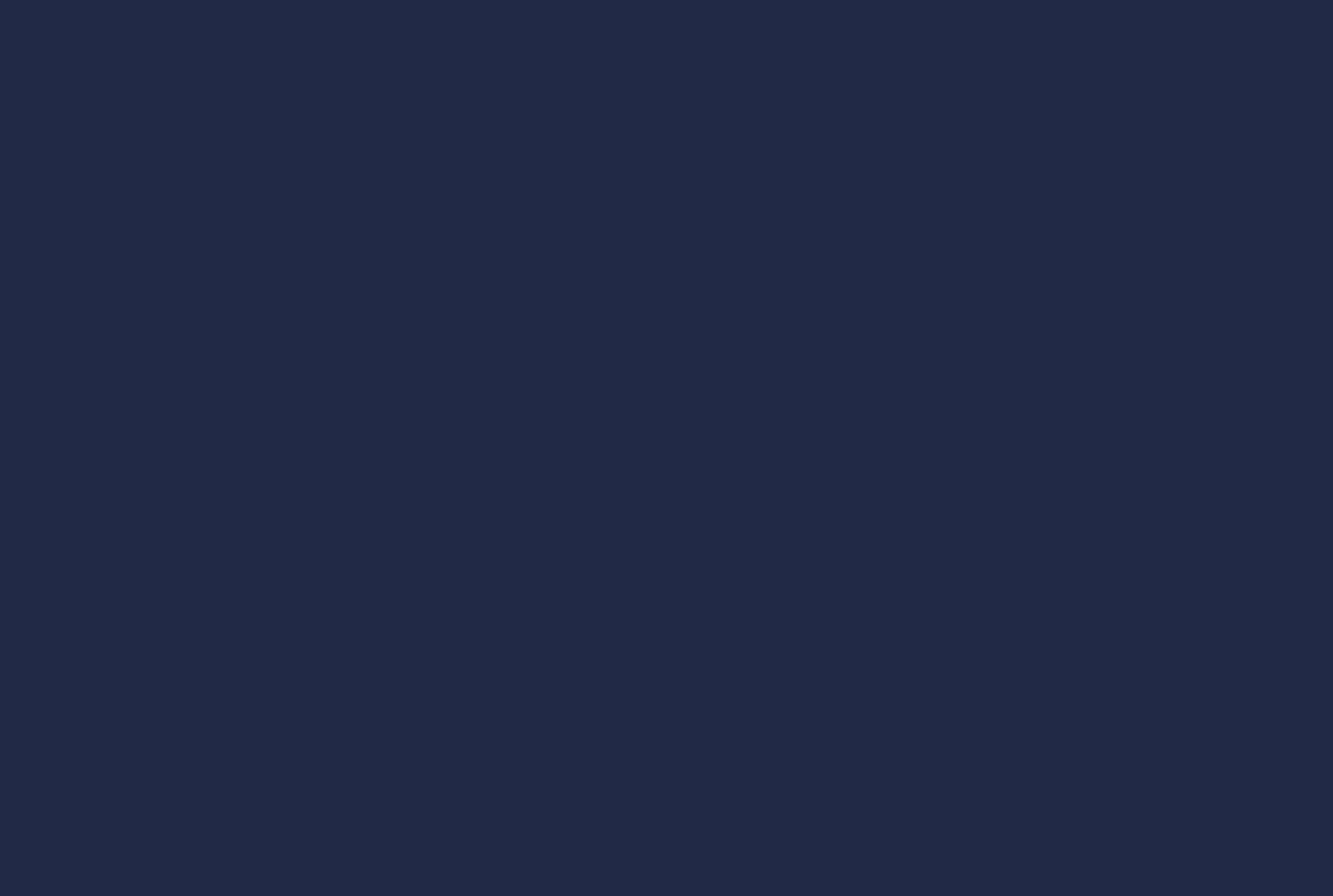
Commit Activity Patterns
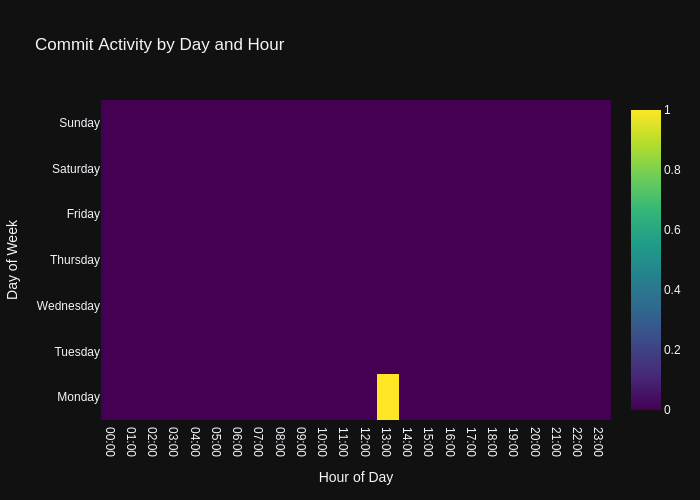
Code Frequency
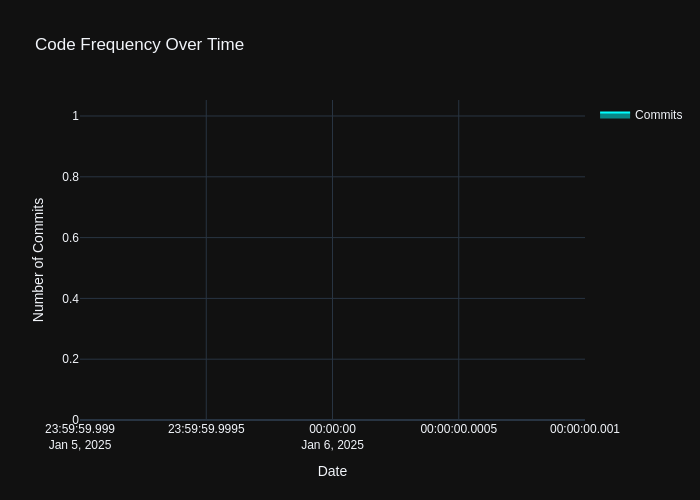
- Repository URL: https://github.com/wanghaisheng/astro-ssr-i18n-params-repro
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 13 日