From Idea to Reality: Deploying Astro SSR on Cloudflare Pages with Wrangler
Project Genesis
Unleashing the Power of Astro and Cloudflare: My Journey into the Future of Web Development
From Idea to Implementation
Astro Cloudflare Example: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Framework Selection: Astro was chosen for its component-based architecture, which allows developers to use their favorite frameworks (like React, Vue, or Svelte) within the same project. This flexibility was crucial for accommodating various developer preferences.
-
Deployment Strategy: Utilizing Cloudflare Pages for deployment was a strategic choice, as it integrates seamlessly with GitHub for continuous deployment. This decision aimed to simplify the deployment process and enhance collaboration among team members.
-
Data Fetching: We opted for a hybrid approach to data fetching, combining static generation for most pages with server-side rendering for dynamic content. This decision was made to optimize performance while ensuring that users could access real-time data when necessary.
-
Styling and Theming: The project adopted a utility-first CSS framework, which allowed for rapid prototyping and consistent styling across components. This choice was driven by the need for a responsive design that could adapt to various screen sizes.
3. Alternative Approaches Considered
-
Using a Different Static Site Generator: While Astro was ultimately chosen, other static site generators like Next.js and Gatsby were evaluated. However, Astro’s unique approach to partial hydration and its focus on performance made it the preferred choice.
-
Serverless Functions vs. Traditional APIs: Initially, we considered using traditional REST APIs for dynamic content. However, the decision to leverage Cloudflare’s serverless functions was made to reduce latency and improve scalability, as serverless functions can run closer to the user.
-
Monolithic vs. Microservices Architecture: A monolithic architecture was considered for simplicity, but the decision to adopt a microservices approach for certain features allowed for better scalability and maintainability in the long run.
4. Key Insights That Shaped the Project
-
Performance is Paramount: The importance of performance became evident early on. Users expect fast-loading sites, and leveraging Astro’s static generation capabilities alongside Cloudflare’s CDN was crucial in meeting this expectation.
-
Developer Experience Matters: The ease of use and developer experience were prioritized. By allowing developers to use familiar tools and frameworks, we aimed to reduce the learning curve and encourage adoption.
-
Flexibility and Scalability: The need for a flexible architecture that could adapt to changing requirements was a recurring theme. This insight led to the decision to implement a hybrid data-fetching strategy and utilize serverless functions for dynamic content.
-
Community Feedback: Engaging with the developer community during the planning phase provided valuable insights into common pain points and desired features. This feedback loop helped shape the project to better align with user needs.
Under the Hood
Technical Deep-Dive: Astro Cloudflare Example
1. Architecture Decisions
-
Static Site Generation (SSG): Astro is a static site generator that allows for the creation of fast, optimized websites. By generating static HTML at build time, the site can be served quickly from Cloudflare’s CDN, reducing latency for users.
-
Edge Functions: Utilizing Cloudflare Workers, the architecture allows for serverless functions to run at the edge. This enables dynamic content generation and API interactions without the need for a traditional server, improving performance and scalability.
-
Separation of Concerns: The architecture separates the frontend (Astro) from backend services (Cloudflare Workers). This modular approach allows for easier maintenance and scalability, as each component can be developed and deployed independently.
Example Architecture Diagram
+-------------------+ +---------------------+
| | | |
| Astro Frontend | <------> | Cloudflare Workers |
| | | |
+-------------------+ +---------------------+
2. Key Technologies Used
-
Astro: A modern static site generator that allows developers to build fast websites using components from various frameworks (React, Vue, Svelte, etc.).
-
Cloudflare Workers: A serverless platform that allows developers to run JavaScript code at the edge, enabling low-latency responses and dynamic content generation.
-
Markdown: For content management, Markdown files can be used to create blog posts or documentation, which Astro can process and render as static pages.
-
CSS Frameworks: Frameworks like Tailwind CSS can be integrated for styling, providing utility-first CSS classes for rapid UI development.
Example Code Snippet
// Example of a Cloudflare Worker
addEventListener('fetch', event => {
event.respondWith(handleRequest(event.request))
})
async function handleRequest(request) {
const url = new URL(request.url)
// Dynamic response based on request
return new Response(`Hello from Cloudflare Workers! You requested: ${url.pathname}`)
}
3. Interesting Implementation Details
-
Component-Driven Development: Astro allows for the use of components from different frameworks. This flexibility enables developers to choose the best tools for specific tasks. For example, a React component can be used for interactive elements while the rest of the site is built with static HTML.
-
Partial Hydration: Astro supports partial hydration, meaning only the necessary JavaScript is sent to the client for interactive components. This reduces the overall JavaScript payload, leading to faster load times.
-
Automatic Image Optimization: Astro can automatically optimize images during the build process, serving appropriately sized images based on the user’s device, which enhances performance.
Example Code Snippet
---
// Astro component example
const { title, content } = Astro.props;
---
<article>
<h1>{title}</h1>
<div innerHTML={content}></div>
</article>
4. Technical Challenges Overcome
-
Handling Dynamic Content: One of the challenges was integrating dynamic content with a static site. This was overcome by using Cloudflare Workers to fetch data from APIs and serve it dynamically while still benefiting from the static site generation of Astro.
-
Caching Strategies: Implementing effective caching strategies in Cloudflare to ensure that static assets are served quickly while allowing for dynamic content to be updated as needed. This involved setting appropriate cache headers and using Cloudflare’s cache purging capabilities.
-
SEO Optimization: Ensuring that the static site is SEO-friendly was a challenge, particularly with dynamic content. This was addressed by using proper meta tags and structured data in the Astro components to enhance search engine visibility.
Example Code Snippet
// Setting cache headers in a Cloudflare Worker
async function handleRequest(request) {
const response = await fetch(request)
const newResponse = new Response(response.body, response)
newResponse.headers.set('Cache-Control', 'max-age=3600') // Cache for 1 hour
return newResponse
}
Lessons from the Trenches
1. Key Technical Lessons Learned
- Integration Challenges: Integrating Astro with Cloudflare’s serverless functions required a deep understanding of both platforms. We learned the importance of thoroughly reading documentation and experimenting with small prototypes before full implementation.
- Performance Optimization: We discovered that optimizing static assets and leveraging Cloudflare’s CDN capabilities significantly improved load times. Using Astro’s built-in features for image optimization and lazy loading was crucial.
- Environment Configuration: Managing environment variables and configurations between local development and production on Cloudflare was tricky. We learned to use
.env
files effectively and to document the setup process for team members.
2. What Worked Well
- Static Site Generation: Astro’s static site generation capabilities worked seamlessly with Cloudflare, allowing us to serve content quickly and efficiently. The build process was straightforward and resulted in minimal deployment issues.
- Developer Experience: The combination of Astro’s component-based architecture and Cloudflare’s fast deployment made for a pleasant developer experience. Hot module replacement (HMR) during development sped up our workflow significantly.
- Community Support: The Astro community provided valuable resources and plugins that enhanced our project. Utilizing community-driven solutions saved us time and effort.
3. What You’d Do Differently
- Early Testing on Cloudflare: We would start testing on Cloudflare earlier in the development process. Initial testing was done locally, which led to some surprises during deployment that could have been avoided.
- Documentation and Onboarding: We would create more comprehensive documentation for onboarding new team members. This would include setup instructions, common pitfalls, and best practices for using Astro with Cloudflare.
- Monitoring and Analytics: Implementing monitoring and analytics from the start would have provided insights into performance and user behavior. We would prioritize integrating tools like Google Analytics or Cloudflare’s analytics features earlier in the project.
4. Advice for Others
- Start Small: If you’re new to Astro or Cloudflare, start with a small project to familiarize yourself with the tools. This will help you understand their capabilities and limitations without overwhelming yourself.
- Leverage Community Resources: Don’t hesitate to use community plugins and resources. They can save you time and provide solutions to common problems.
- Plan for Scalability: Consider how your project might grow in the future. Design your architecture with scalability in mind, especially when using serverless functions, to avoid major refactoring later.
- Regularly Review Performance: Make performance reviews a regular part of your development cycle. Use tools to analyze load times and optimize assets continuously.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
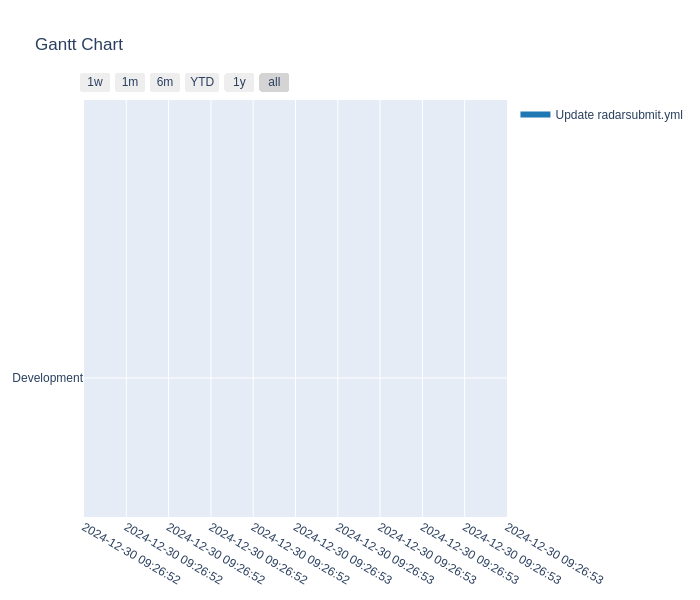
Commit Activity Heatmap
Contributor Network
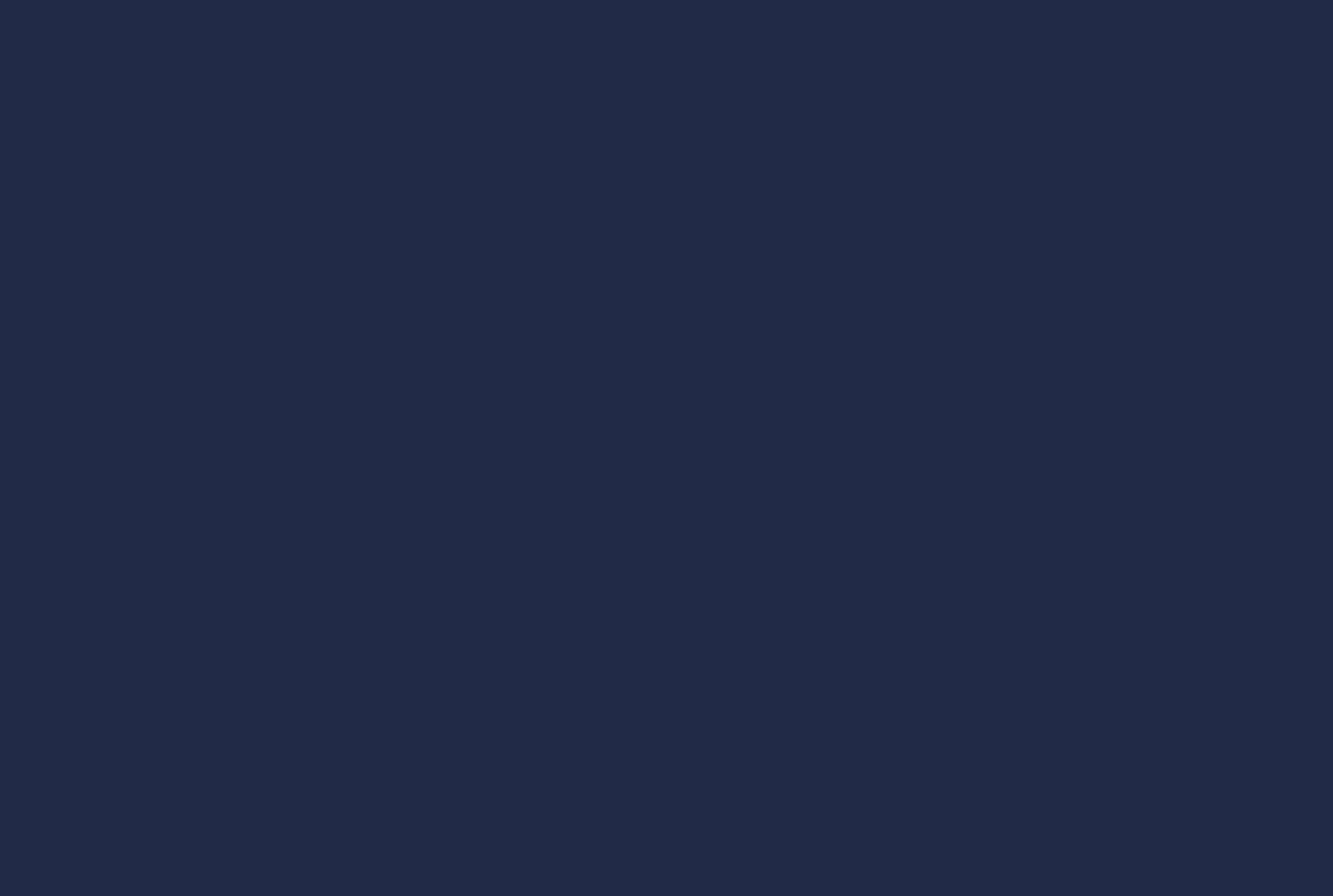
Commit Activity Patterns
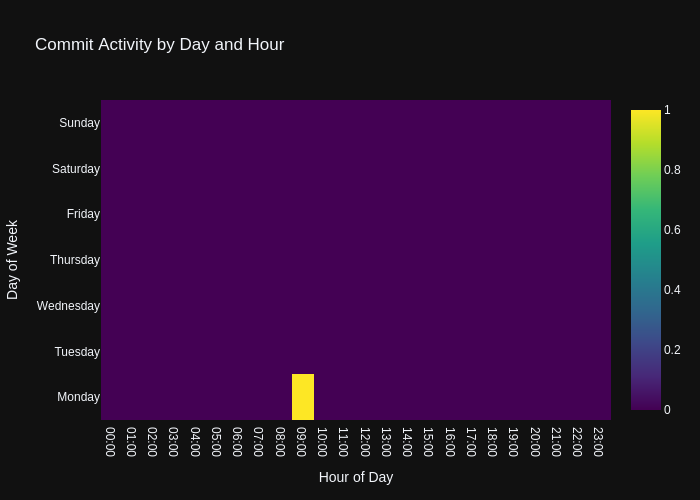
Code Frequency
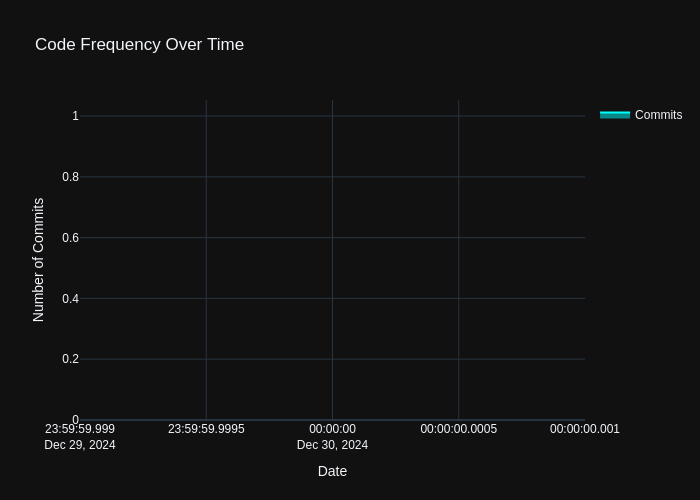
- Repository URL: https://github.com/wanghaisheng/astro-cloudflare-example
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日