Building Apple Accessories Hub: A React & Next.js Side Project Journey
Project Genesis
Unleashing My Creativity: The Journey Behind the Apple Accessories Hub
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
React and Next.js: React was chosen for its component-based architecture, which promotes reusability and maintainability. Next.js was selected to leverage its server-side rendering capabilities, improving performance and SEO, which are crucial for e-commerce sites.
-
TypeScript: The decision to use TypeScript was made to enhance code quality and maintainability. TypeScript’s static typing helps catch errors early in the development process, making the codebase more robust.
-
Tailwind CSS: For styling, Tailwind CSS was chosen due to its utility-first approach, allowing for rapid prototyping and a consistent design system. This framework enabled the creation of a responsive layout that adapts seamlessly to different screen sizes.
-
Redux: State management was handled with Redux to manage the application’s global state effectively. This was particularly important for features like the shopping cart, where the state needs to be accessible across multiple components.
-
Stripe for Payments: Integrating Stripe for payment processing was a strategic choice to ensure secure and reliable transactions. The use of a test card for simulating payments provided a safe environment for users to experience the checkout process without real financial transactions.
3. Alternative Approaches Considered
-
Framework Alternatives: While React and Next.js were ultimately chosen, other frameworks like Vue.js and Angular were considered. However, React’s popularity and the extensive ecosystem of libraries made it a more appealing choice.
-
CSS Frameworks: Other CSS frameworks like Bootstrap and Bulma were evaluated. However, Tailwind CSS’s flexibility and customization options aligned better with the project’s design goals.
-
State Management Alternatives: While Redux was selected for state management, alternatives like Context API or MobX were also considered. Redux was favored for its scalability and community support, especially for larger applications.
4. Key Insights That Shaped the Project
-
User Experience is Paramount: The importance of a seamless user experience became evident during testing. Features like the image carousel and intuitive navigation were crucial in keeping users engaged and encouraging them to explore products.
-
Performance Matters: The need for fast load times and smooth interactions was highlighted during the initial testing phases. Utilizing Next.js for server-side rendering and optimizing images helped improve performance, which is vital for retaining users.
-
Iterative Development: Embracing an iterative development approach allowed for continuous feedback and improvements. Regular testing and user feedback sessions helped identify pain points and areas for enhancement, leading to a more polished final product.
-
Security in Transactions: The significance of secure payment processing was underscored during the integration of Stripe. Ensuring that users felt safe while making transactions was a top priority, influencing the decision to use a well-established payment processor.
Under the Hood
Technical Deep-Dive: Apple Accessories Clone
1. Architecture Decisions
Key Architectural Choices:
- Single Page Application (SPA): Utilizing Next.js allows for server-side rendering (SSR) and static site generation (SSG), improving performance and SEO.
- State Management: Redux is employed for state management, enabling a centralized store for application state, which is particularly useful for managing the shopping cart and user authentication.
- Responsive Design: Tailwind CSS is used for styling, which promotes a mobile-first approach and ensures that the application is responsive across various devices.
2. Key Technologies Used
- React: A JavaScript library for building user interfaces, allowing for the creation of reusable UI components.
- Next.js: A React framework that enables server-side rendering and static site generation, enhancing performance and SEO.
- TypeScript: A superset of JavaScript that adds static typing, improving code quality and maintainability.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness.
- Redux: A predictable state container for JavaScript apps, facilitating state management across the application.
- React Slick: A carousel component for React, used to create the image carousel on the homepage.
- Vercel: A cloud platform for static sites and serverless functions, used for deploying the application.
- Stripe: A payment processing platform that allows for secure payment transactions.
3. Interesting Implementation Details
Image Carousel
import Slider from "react-slick";
const ImageCarousel = () => {
const settings = {
dots: true,
infinite: true,
speed: 500,
slidesToShow: 1,
slidesToScroll: 1,
};
return (
<Slider {...settings}>
<div>
<img src="image1.jpg" alt="Product 1" />
</div>
<div>
<img src="image2.jpg" alt="Product 2" />
</div>
{/* Additional slides */}
</Slider>
);
};
Shopping Cart Functionality
// actions/cartActions.js
export const addToCart = (product) => ({
type: 'ADD_TO_CART',
payload: product,
});
// reducers/cartReducer.js
const cartReducer = (state = { cartItems: [] }, action) => {
switch (action.type) {
case 'ADD_TO_CART':
return { ...state, cartItems: [...state.cartItems, action.payload] };
default:
return state;
}
};
4. Technical Challenges Overcome
Payment Integration
import { useStripe, useElements, CardElement } from '@stripe/react-stripe-js';
const CheckoutForm = () => {
const stripe = useStripe();
const elements = useElements();
const handleSubmit = async (event) => {
event.preventDefault();
const cardElement = elements.getElement(CardElement);
const { error, paymentMethod } = await stripe.createPaymentMethod({
type: 'card',
card: cardElement,
});
if (error) {
console.error(error);
} else {
console.log('Payment Method:', paymentMethod);
// Handle successful payment
}
};
return (
<form onSubmit={handleSubmit}>
<CardElement />
<button type="submit" disabled={!stripe}>Pay</button>
</form>
);
};
Responsive Design
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
State Management with Redux: Implementing Redux for state management was a significant learning experience. Understanding the flow of data and how to manage global state effectively helped in maintaining a clean architecture. I learned the importance of structuring actions and reducers properly to avoid unnecessary complexity.
-
TypeScript Integration: Using TypeScript with React and Next.js improved code quality and reduced runtime errors. I learned how to define interfaces for props and state, which made the components more predictable and easier to debug.
-
Responsive Design with Tailwind CSS: Tailwind CSS allowed for rapid prototyping and responsive design. I learned how utility-first CSS can streamline the styling process and make it easier to maintain consistency across the application.
-
Payment Integration with Stripe: Implementing Stripe for payment processing taught me about handling sensitive data securely and the importance of following best practices for payment integrations. I learned how to use test cards effectively and the significance of error handling during payment processes.
What Worked Well
-
Component Reusability: The use of functional components and hooks in React allowed for high reusability of components. This made it easier to manage and update the UI without duplicating code.
-
Image Carousel: The integration of React Slick for the image carousel was seamless and provided a smooth user experience. Users appreciated the visual appeal and interactivity of the homepage.
-
User Experience: The overall user experience was enhanced by the clean design and intuitive navigation. Users could easily find products and complete their purchases, which was reflected in positive feedback during testing.
-
Deployment on Vercel: Deploying the application on Vercel was straightforward, and the platform’s features, such as automatic scaling and easy integration with GitHub, made the deployment process efficient.
What You’d Do Differently
-
Improved Error Handling: While the application had basic error handling, I would implement more comprehensive error handling for API calls and payment processing. This would enhance user experience by providing clearer feedback in case of issues.
-
Performance Optimization: I would focus more on performance optimization techniques, such as code splitting and lazy loading of components, to improve the initial load time of the application.
-
Testing: I would incorporate more unit and integration tests using tools like Jest and React Testing Library. This would ensure that components behave as expected and help catch bugs early in the development process.
-
Accessibility: I would prioritize accessibility features from the beginning, ensuring that the application is usable for all users, including those with disabilities. This includes proper ARIA roles and keyboard navigation support.
Advice for Others
-
Plan Your Architecture: Before diving into coding, take the time to plan your application’s architecture. Define how components will interact, how state will be managed, and how data will flow through the application. This will save time and reduce complexity later on.
-
Leverage Documentation: Make use of the documentation for the libraries and frameworks you are using. Understanding the capabilities and best practices of tools like Redux, TypeScript, and Tailwind CSS can significantly enhance your development process.
-
Iterate Based on Feedback: After launching your project, gather feedback from users and iterate on your design and functionality. User insights can provide valuable information on what works and what needs improvement.
-
Stay Updated: The tech landscape is constantly evolving. Stay updated with the latest trends and best practices in web development to ensure your skills and projects remain relevant.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
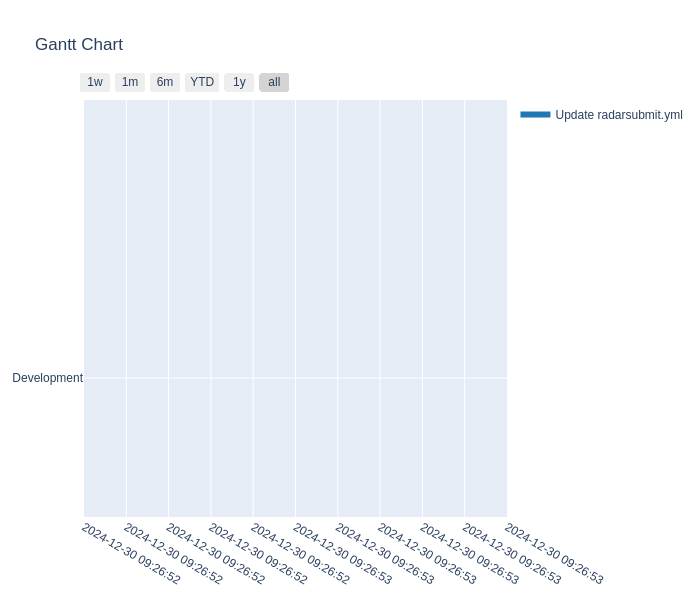
Commit Activity Heatmap
Contributor Network
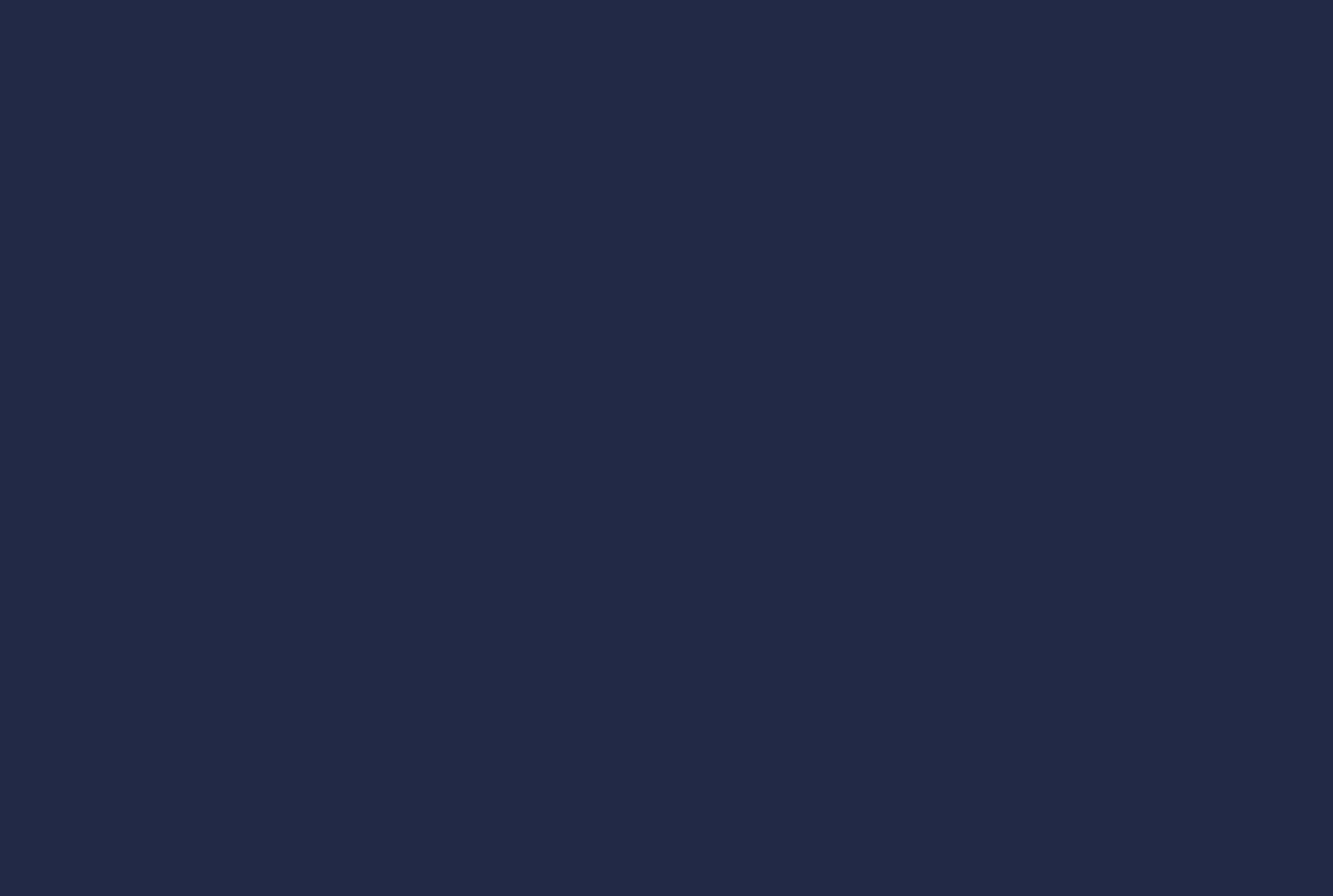
Commit Activity Patterns
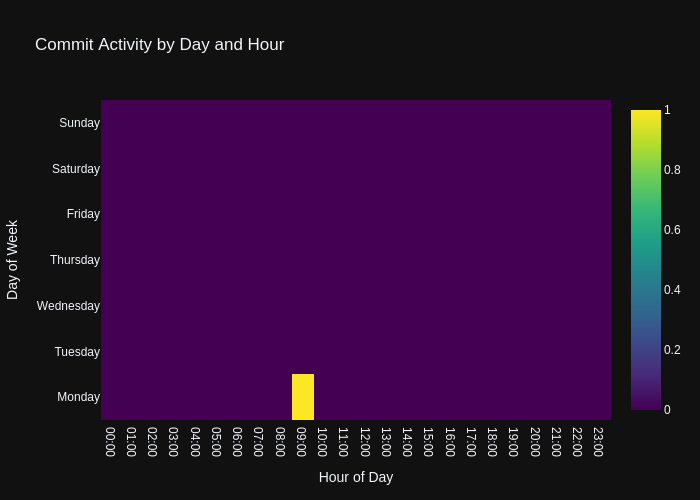
Code Frequency
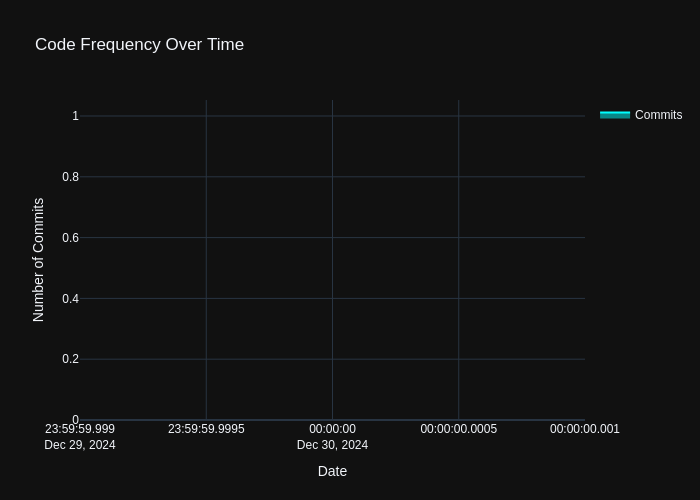
- Repository URL: https://github.com/wanghaisheng/apple-accessories-hub
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日