Building anti-hair-loss: A Developer's Journey to Combat Hair Loss with Websim
Project Genesis
Battling the Baldness: My Journey into Anti-Hair-Loss Solutions
From Idea to Implementation
Journey from Concept to Code: Building a Web Simulation Site
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Auto-generated Sitemap: This was crucial for SEO, as it helps search engines index the site more effectively. The sitemap would be generated based on language subfolders and include all relevant HTML files.
-
SEO Compliance Checks: To avoid common pitfalls like Google redirection issues and non-indexing, we integrated automated checks that would alert us to any potential problems before deployment.
-
URL Submission via IndexNow: This feature was selected to streamline the process of getting our pages indexed by Google, ensuring that new content is quickly recognized.
-
Responsive Design: Given the increasing use of mobile devices, we prioritized a responsive design to enhance user experience across all platforms.
-
PWA Support: Implementing Progressive Web App (PWA) features was a strategic choice to improve user engagement and retention, allowing users to install the site as an app on their devices.
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
Automation is Key: The realization that many SEO tasks could be automated led to the decision to build features that would handle these processes without manual intervention. This not only saved time but also reduced the likelihood of human error.
-
User Experience Matters: The importance of a seamless user experience became increasingly clear. As we developed the site, we focused on ensuring that all features worked harmoniously together, providing users with a smooth and engaging experience.
-
Continuous Improvement: The project underscored the necessity of ongoing monitoring and iteration. By integrating analytics tools, we could gather data on user behavior and site performance, allowing us to make informed decisions for future enhancements.
Under the Hood
Technical Deep-Dive: WebSim Site Builder
1. Architecture Decisions
- Frontend: A responsive web interface that adapts to both PC and mobile devices, ensuring a seamless user experience.
- Backend: A server-side application that handles requests, processes data, and interacts with external APIs (e.g., Google Indexing API).
- Database: A storage solution for managing user data, site configurations, and generated content.
- Static Site Generation: The site is built as a static site, which enhances performance and SEO by serving pre-rendered HTML files.
Key Architectural Decisions:
- Modularity: Each feature (e.g., SEO checks, sitemap generation) is implemented as a separate module, allowing for easier maintenance and updates.
- Static Site Generation: By generating static HTML files, the site benefits from faster load times and improved SEO.
- Responsive Design: Utilizing CSS frameworks (e.g., Bootstrap) to ensure the site is mobile-friendly.
2. Key Technologies Used
- HTML/CSS/JavaScript: Core technologies for building the frontend.
- Node.js: Used for the backend server to handle requests and process data.
- Express.js: A web framework for Node.js that simplifies routing and middleware management.
- Google Indexing API: For submitting URLs to Google for indexing.
- PWA (Progressive Web App): To enhance user experience with offline capabilities and app-like features.
- SEO Tools: Libraries and APIs for checking SEO requirements and generating metadata.
3. Interesting Implementation Details
Auto-Generate Sitemap
.html
files. This is achieved using a simple script that scans the directory structure and compiles a list of URLs.const fs = require('fs');
const path = require('path');
function generateSitemap(langFolder) {
const sitemap = [];
const files = fs.readdirSync(langFolder);
files.forEach(file => {
if (file.endsWith('.html')) {
sitemap.push(`https://example.com/${langFolder}/${file}`);
}
});
fs.writeFileSync('sitemap.xml', `<urlset>${sitemap.map(url => `<url><loc>${url}</loc></url>`).join('')}</urlset>`);
}
SEO Checks
const axios = require('axios');
async function checkSEO(url) {
const response = await axios.get(url);
const isRedirect = response.status >= 300 && response.status < 400;
const isIndexable = !response.headers['x-robots-tag']?.includes('noindex');
return { isRedirect, isIndexable };
}
PWA Support
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
});
});
}
4. Technical Challenges Overcome
Challenge: SEO Compliance
Challenge: Responsive Design
Challenge: Image Generation
Example of Image Generation Code
const { createCanvas } = require('canvas');
function generateLogo(text) {
const canvas = createCanvas(200, 100);
const ctx = canvas.getContext('2d');
ctx.fillStyle = '#000';
ctx.fillRect(0, 0, 200, 100);
ctx.fillStyle = '#fff';
ctx.font = '30px Arial';
ctx.fillText(text, 50, 50);
return canvas.toDataURL();
}
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Automation is Key: Automating tasks such as sitemap generation, SEO checks, and URL submissions significantly reduces manual effort and minimizes human error. Implementing tools like Websim for site building and automation can streamline the development process.
-
SEO Best Practices: Understanding and implementing SEO requirements early in the project helped avoid common pitfalls like Google redirection issues and indexing problems. Regularly checking SEO metrics is crucial for maintaining site visibility.
-
Responsive Design: Ensuring that the site is mobile-responsive from the start is essential. This not only improves user experience but also positively impacts SEO rankings.
-
Integration of Analytics: Incorporating tools like Google Analytics and Microsoft Clarity from the beginning provided valuable insights into user behavior, which informed further development and optimization.
-
Progressive Web App (PWA) Support: Adding PWA support enhanced the user experience by allowing offline access and faster load times, which are critical for user retention.
What Worked Well
-
Sitemap Generation: The automatic generation of sitemaps based on language subfolders and HTML files was effective in improving site navigation and SEO.
-
Keyword Research Tools: Utilizing tools like SpyFu for keyword research helped in identifying valuable keywords and trends, which informed content strategy.
-
Image Generation: Automating the generation of logos and cover images saved time and ensured consistency in branding.
-
Blog Text Generation: Implementing automated blog text generation using the G4F tool provided a steady stream of content, which is vital for SEO and user engagement.
-
Static Framework Deployment: Using GitHub Actions for automatic deployment of static frameworks simplified the deployment process and reduced downtime.
What You’d Do Differently
-
More Comprehensive Testing: While automation is beneficial, more thorough testing of automated processes (like SEO checks and sitemap generation) could prevent issues from arising post-deployment.
-
User Feedback Loop: Establishing a more structured feedback loop with users could provide insights into areas for improvement that may not be captured by analytics alone.
-
Documentation: Improving documentation throughout the development process would help onboard new contributors more effectively and ensure that best practices are followed consistently.
-
Scalability Considerations: Planning for scalability from the outset, especially regarding server resources and content management, could prevent bottlenecks as the site grows.
Advice for Others
-
Start with a Clear Plan: Before diving into development, outline clear goals and requirements. This will guide your decisions and help prioritize features.
-
Leverage Existing Tools: Don’t reinvent the wheel. Use existing tools and libraries to handle common tasks, which can save time and reduce complexity.
-
Focus on SEO Early: Integrate SEO best practices from the beginning rather than as an afterthought. This will save time and effort in the long run.
-
Iterate Based on Data: Use analytics to inform your decisions and iterate on your site based on real user data. This will help you make informed improvements.
-
Engage with the Community: Participate in forums and communities related to your tech stack. Sharing experiences and learning from others can provide valuable insights and support.
What’s Next?
Conclusion: A Forward-Looking Perspective on Our Anti-Hair-Loss Project
Project Development Analytics
timeline gant
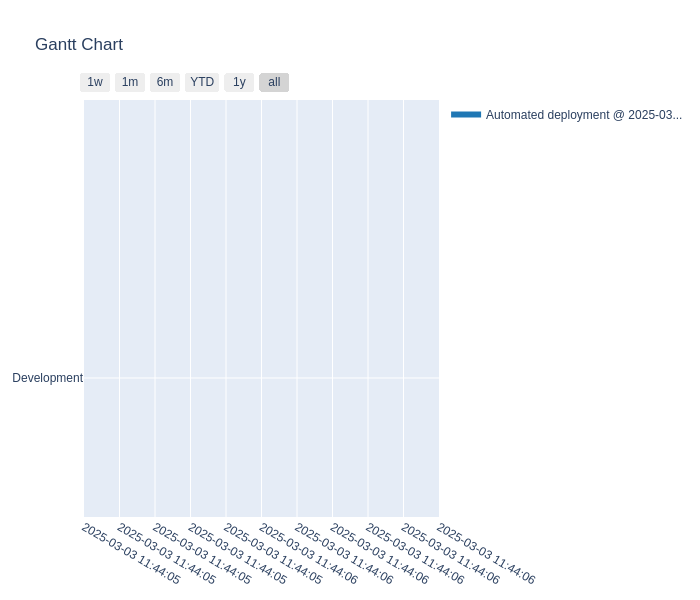
Commit Activity Heatmap
Contributor Network
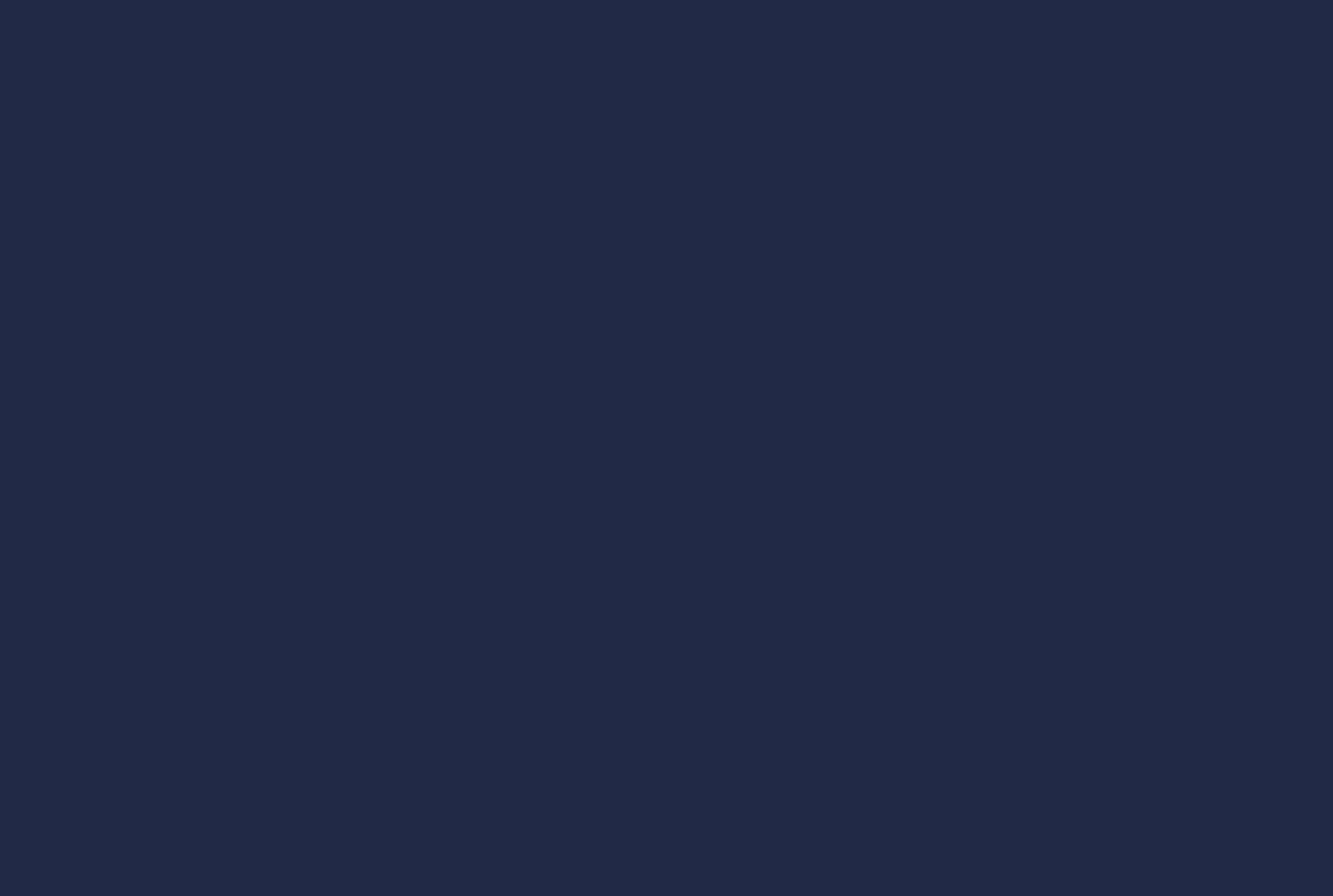
Commit Activity Patterns
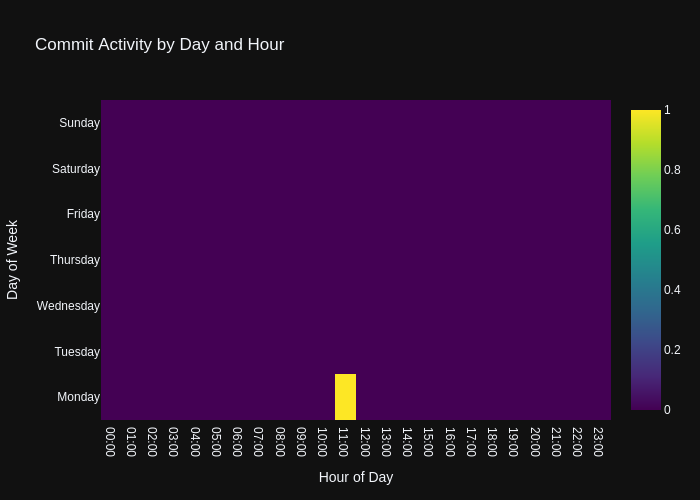
Code Frequency
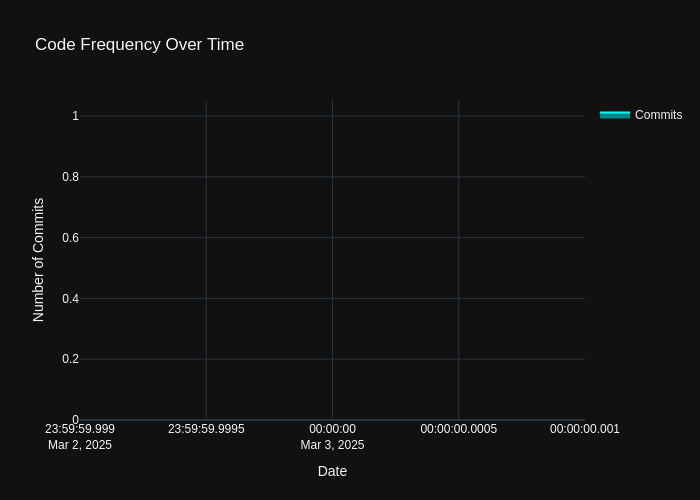
- Repository URL: https://github.com/wanghaisheng/anti-hair-loss
- Stars: 0
- Forks: 1
编辑整理: Heisenberg 更新日期:2025 年 3 月 10 日