Building AniProject: Crafting an Ad-Free Anime Hub with Next.js & Firebase
Project Genesis
Welcome to AniProject: Your Ultimate Anime and Manga Hub!
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-friendly interface became evident early on. We prioritized features that enhance user engagement, such as personalized watchlists, notifications for new episodes, and the ability to mark favorite content. This focus on user experience guided our design and development decisions.
-
Community Engagement: Understanding the community aspect of anime and manga consumption was crucial. We recognized that users often seek recommendations and discussions about their favorite shows. This insight led to the inclusion of features like a news feed and the ability to track and share progress on watched episodes.
-
Scalability and Flexibility: As we built the application, we realized the need for a scalable architecture that could accommodate future features and user growth. This understanding influenced our choice of technologies, ensuring that the application could evolve without significant overhauls.
-
Legal Considerations: The non-commercial nature of the project necessitated a careful approach to content usage. We ensured that our implementation adhered to legal guidelines, which shaped our decisions regarding API usage and data handling.
Under the Hood
Technical Deep-Dive: AniProject
1. Architecture Decisions
Client-Side Architecture
- Framework: The front-end is built using Next.js, a React framework that enables server-side rendering and static site generation. This choice enhances performance and SEO, making the application more accessible.
- State Management: Redux is employed for state management, allowing for a centralized store to manage the application’s state, which is particularly useful for handling user authentication and media data.
- API Integration: The application integrates with multiple APIs (AniList, Consumet, and Aniwatch) to fetch data about anime and manga. This modular approach allows for easy updates and maintenance of API connections.
Server-Side Architecture
- Back-End: The back-end is powered by Firebase, which provides a Firestore database for data storage and Firebase Authentication for user management. This eliminates the need for a custom server, simplifying deployment and scaling.
- Environment Configuration: The application relies on environment variables to manage sensitive information and API keys, ensuring that configurations can be easily adjusted for different environments (development, staging, production).
2. Key Technologies Used
- Next.js: For building the front-end, enabling server-side rendering and static site generation.
- TypeScript: Provides type safety, improving code quality and maintainability.
- Firebase: Used for authentication and as a NoSQL database, allowing for real-time data synchronization.
- Axios: For making HTTP requests to external APIs.
- GraphQL: Utilized for querying data from APIs, providing a flexible and efficient way to retrieve only the data needed.
- Framer Motion: For animations, enhancing the user experience with smooth transitions and interactions.
3. Interesting Implementation Details
User Authentication
import firebase from 'firebase/app';
import 'firebase/auth';
const signInWithGoogle = async () => {
const provider = new firebase.auth.GoogleAuthProvider();
const result = await firebase.auth().signInWithPopup(provider);
// Handle result
};
Data Fetching
import axios from 'axios';
const fetchAnimeData = async (animeId) => {
const response = await axios.get(`https://api.example.com/anime/${animeId}`);
return response.data;
};
Firestore Database Structure
const userRef = firebase.firestore().collection('users').doc(userId);
const userData = await userRef.get();
4. Technical Challenges Overcome
API Rate Limiting
Cold Start Issues
Handling User Preferences
const updateUserPreferences = async (userId, preferences) => {
await firebase.firestore().collection('users').doc(userId).update({
preferences: preferences,
});
};
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
API Integration: Successfully integrating multiple APIs (AniList, Consumet, and Aniwatch) taught me the importance of understanding each API’s documentation and capabilities. I learned how to handle asynchronous requests and manage data flow between the front-end and back-end effectively.
-
State Management: Utilizing Redux for state management helped me understand the importance of maintaining a predictable state in a complex application. It allowed for easier debugging and improved performance by minimizing unnecessary re-renders.
-
Firebase Authentication: Implementing Firebase for user authentication provided insights into secure user management. I learned how to configure authentication methods and manage user sessions effectively.
-
Environment Variables: Managing environment variables for different deployment environments (development, staging, production) was crucial. It reinforced the need for security and flexibility in handling sensitive information.
-
Responsive Design: Building a responsive UI using CSS frameworks and custom styles taught me the importance of user experience across different devices. I learned to use media queries and flexible layouts to ensure accessibility.
What Worked Well
-
Modular Architecture: The project’s modular structure allowed for easy maintenance and scalability. Each component was designed to be reusable, which simplified the development process.
-
User Experience Features: Features like “Keep Watching” and notifications for new episodes significantly enhanced user engagement. These features were well-received during testing and contributed to a positive user experience.
-
Deployment Process: The deployment process on platforms like Vercel and Render was straightforward. The integration of CI/CD practices allowed for quick updates and rollbacks, which streamlined the development workflow.
-
Community Feedback: Engaging with the anime community for feedback on features and design helped refine the application. User input was invaluable in prioritizing development tasks and improving overall functionality.
What You’d Do Differently
-
Documentation: While the README provided a good overview, I would invest more time in creating comprehensive documentation for developers. This would include detailed API usage examples, component structure, and troubleshooting tips.
-
Testing: Implementing a more robust testing strategy (unit tests, integration tests) would have been beneficial. Automated testing could help catch bugs early and ensure that new features do not break existing functionality.
-
Performance Optimization: I would focus more on performance optimization techniques, such as code splitting and lazy loading, to improve the initial load time of the application.
-
User Analytics: Integrating user analytics from the start would provide insights into user behavior and help prioritize features based on actual usage patterns.
Advice for Others
-
Plan Before You Code: Spend time planning the architecture and features of your project before diving into coding. A clear roadmap can save time and effort in the long run.
-
Leverage Open Source: Utilize existing open-source libraries and frameworks to speed up development. Don’t reinvent the wheel; instead, build upon the work of others.
-
Engage with Users Early: Involve potential users in the development process early on. Their feedback can guide feature development and help create a product that meets their needs.
-
Stay Updated: Technology evolves rapidly, so keep learning and stay updated with the latest trends and best practices in web development. This will help you make informed decisions and improve your skills continuously.
-
Focus on Security: Always prioritize security, especially when handling user data. Implement best practices for authentication, data storage, and API usage to protect your application and its users.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
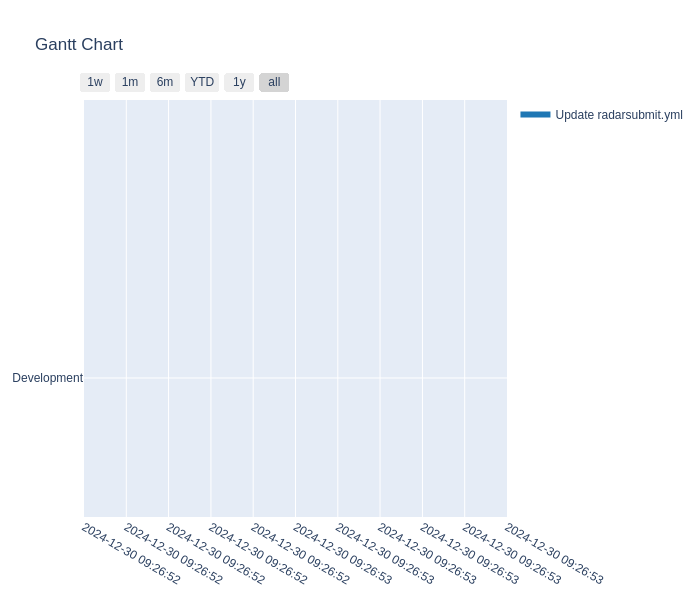
Commit Activity Heatmap
Contributor Network
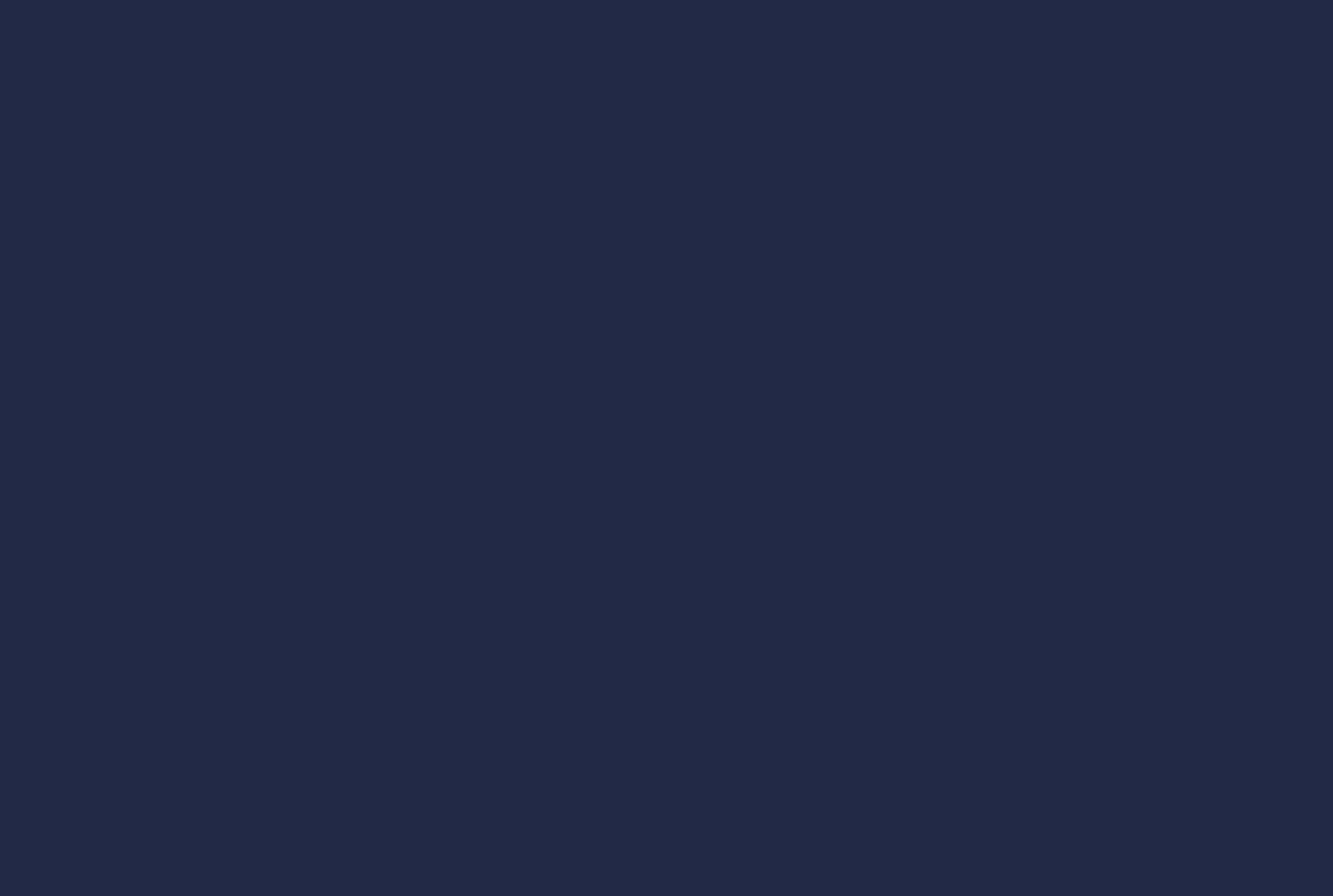
Commit Activity Patterns
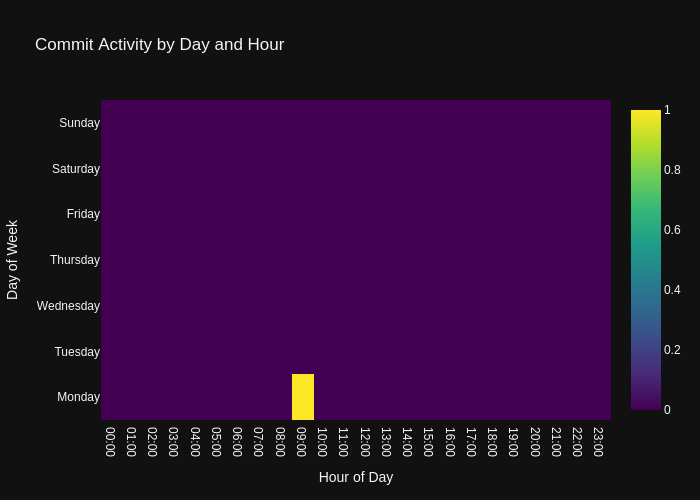
Code Frequency
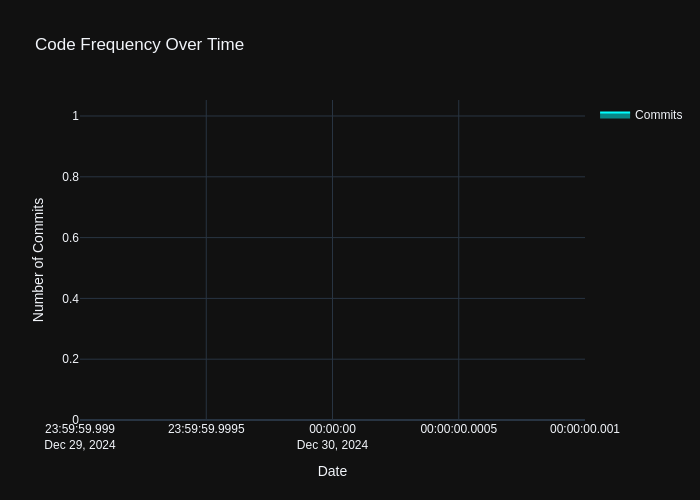
- Repository URL: https://github.com/wanghaisheng/anime-website-hub
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日