From Idea to Reality: Building PopShop with React, TypeScript, and Supabase
Project Genesis
🌟 PopShop: A Journey of Creativity and Collaboration 🌟
From Idea to Implementation
Journey from Concept to Code: PopShop
1. Initial Research and Planning
- User Experience (UX): Research indicated that a seamless and intuitive user interface was crucial for retaining customers. This led to the decision to use Daisy UI, which integrates well with Tailwind CSS for rapid UI development.
- Community Engagement: The goal was to create a space where users could showcase their projects and connect with like-minded individuals. This influenced the decision to make the project open-source, encouraging contributions and collaboration.
2. Technical Decisions and Their Rationale
- React: Chosen for its component-based architecture, which allows for reusable UI components and efficient rendering.
- TypeScript: Implemented to enhance code quality and maintainability through static typing, reducing runtime errors.
- Daisy UI and Tailwind CSS: These frameworks were selected for their utility-first approach, enabling rapid styling and customization of components.
- Supabase: Opted for as the backend solution due to its ease of integration with front-end technologies and its powerful features like real-time data and user authentication.
3. Alternative Approaches Considered
- Framework Alternatives: While React was the final choice, other frameworks like Vue.js and Angular were evaluated. React’s large community and ecosystem made it a more appealing option for future scalability and support.
- Backend Solutions: Initially, Firebase was considered for backend services. However, Supabase was chosen for its SQL-based database, which provided more flexibility and control over data management.
- Design Systems: The team explored various design systems but ultimately settled on Daisy UI due to its compatibility with Tailwind CSS and its focus on accessibility and responsiveness.
4. Key Insights That Shaped the Project
- Community-Centric Development: The importance of fostering a collaborative environment became evident. By making PopShop open-source, the team aimed to create a platform where contributions from diverse individuals could enhance the project.
- User-Centric Design: Continuous feedback from potential users highlighted the need for a user-friendly interface. This led to iterative design processes, ensuring that the final product met user expectations and provided a seamless shopping experience.
- Documentation and Contribution Guidelines: The necessity for clear documentation and contribution guidelines was recognized early on. This not only facilitated onboarding for new contributors but also ensured that the project maintained high standards of quality and consistency.
Conclusion
Under the Hood
Technical Deep-Dive: PopShop eCommerce Website
1. Architecture Decisions
-
Microservices Approach: The application is structured to separate concerns between the front-end and back-end. The front-end is built using React with TypeScript, while Supabase serves as the back-end, providing both database and authentication services.
-
Component-Based Architecture: React’s component-based architecture allows for reusable UI components, which enhances maintainability and scalability. Each component is designed to encapsulate its own logic and presentation.
-
State Management: The application utilizes React’s built-in state management along with context API for global state management, ensuring that the application remains responsive and efficient.
-
Responsive Design: The use of Daisy UI and Tailwind CSS ensures that the application is responsive and visually appealing across different devices.
2. Key Technologies Used
-
React: A JavaScript library for building user interfaces, allowing for the creation of dynamic and interactive web applications.
-
TypeScript: A superset of JavaScript that adds static typing, which helps in catching errors during development and improving code quality.
-
Daisy UI: A component library that provides pre-designed UI components, speeding up the development process and ensuring a consistent design.
-
Supabase: An open-source Firebase alternative that provides a backend-as-a-service, including authentication, real-time databases, and storage.
-
Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on customization and responsiveness.
3. Interesting Implementation Details
User Authentication
import { createClient } from '@supabase/supabase-js';
const supabase = createClient('https://your-supabase-url', 'your-anon-key');
// Sign up a new user
const { user, error } = await supabase.auth.signUp({
email: '[email protected]',
password: 'password123',
});
// Sign in an existing user
const { user, error } = await supabase.auth.signIn({
email: '[email protected]',
password: 'password123',
});
Component Structure
ProductCard
component is designed to display individual products:const ProductCard = ({ product }) => {
return (
<div className="border rounded-lg p-4">
<img src={product.image} alt={product.name} className="w-full h-48 object-cover" />
<h2 className="text-lg font-bold">{product.name}</h2>
<p className="text-gray-600">${product.price}</p>
<button className="bg-blue-500 text-white rounded px-4 py-2">Add to Cart</button>
</div>
);
};
API Integration
const fetchProducts = async () => {
const { data, error } = await supabase.from('products').select('*');
if (error) console.error('Error fetching products:', error);
return data;
};
4. Technical Challenges Overcome
Handling Real-Time Updates
const subscription = supabase
.from('products')
.on('INSERT', payload => {
console.log('New product added:', payload.new);
// Update state to reflect new product
})
.subscribe();
Ensuring Security
Performance Optimization
const LazyImage = ({ src, alt }) => {
const [isVisible, setIsVisible] = useState(false);
const ref = useRef();
const observer = useRef(
new IntersectionObserver(([entry]) => {
if (entry.isIntersecting) {
setIsVisible(true);
observer.current.disconnect();
}
})
);
useEffect(() => {
observer.current.observe(ref.current);
return () => observer.current.disconnect();
}, []);
return (
<div ref={ref
## Lessons from the Trenches
### Key Technical Lessons Learned
1. **Integration of Technologies**: Successfully integrating React with TypeScript and Supabase taught me the importance of choosing the right tech stack for scalability and maintainability. Understanding how to leverage Supabase for backend services simplified user authentication and data management.
2. **Component-Based Architecture**: Building the application using a component-based architecture in React emphasized the importance of reusability and separation of concerns. This approach made it easier to manage the codebase and implement new features.
3. **Responsive Design**: Utilizing Daisy UI and TailwindCSS for styling reinforced the significance of responsive design. I learned how to create a seamless user interface that adapts to different screen sizes, enhancing user experience.
4. **Version Control and Collaboration**: Managing contributions through GitHub highlighted the importance of version control in collaborative projects. It taught me how to effectively handle pull requests, code reviews, and maintain a clean commit history.
### What Worked Well
1. **Community Engagement**: The open-source nature of the project attracted contributors who were eager to collaborate. This fostered a sense of community and led to diverse ideas and improvements.
2. **Documentation**: Providing clear documentation, including a comprehensive README, contribution guidelines, and a code of conduct, facilitated onboarding for new contributors and helped maintain project standards.
3. **User Authentication**: Implementing user authentication with Supabase was straightforward and effective. It allowed for secure user management without the overhead of building a custom authentication system.
4. **Deployment Process**: Using Vercel for deployment streamlined the process, allowing for quick iterations and easy access to the latest version of the application for testing and feedback.
### What I'd Do Differently
1. **More Comprehensive Testing**: While the project has a solid foundation, I would implement more extensive testing (unit and integration tests) to ensure the reliability of features and reduce the likelihood of bugs in future updates.
2. **Enhanced User Feedback Mechanisms**: Incorporating user feedback mechanisms, such as surveys or feedback forms, could provide valuable insights into user experience and areas for improvement.
3. **Performance Optimization**: I would focus on performance optimization earlier in the development process, particularly in terms of loading times and responsiveness, to enhance user satisfaction.
4. **Feature Prioritization**: Establishing a clearer roadmap for feature development and prioritization would help manage contributions more effectively and align the community's efforts with the project's goals.
### Advice for Others
1. **Start with a Clear Vision**: Before diving into development, outline a clear vision and goals for your project. This will guide your decisions and help attract like-minded contributors.
2. **Emphasize Documentation**: Invest time in creating thorough documentation. It not only helps new contributors but also serves as a reference for existing team members.
3. **Encourage Collaboration**: Foster a welcoming environment for contributions. Encourage discussions, provide constructive feedback, and recognize the efforts of contributors to build a positive community.
4. **Iterate and Adapt**: Be open to feedback and willing to adapt your project based on user needs and contributor suggestions. Continuous improvement is key to a successful open-source project.
By reflecting on these lessons and experiences, I hope to contribute to the growth of the PopShop project and inspire others in their open-source journeys.
## What's Next?
## Conclusion: The Future of PopShop
As we wrap up this phase of the PopShop project, we are excited to share our current status and future development plans. The PopShop eCommerce platform, built with React TypeScript and Daisy UI, is already live and operational, showcasing a seamless user interface and robust user authentication features. Our integration with Supabase for backend and database management has laid a solid foundation for further enhancements.
Looking ahead, we have ambitious plans to expand PopShop's capabilities. Future development will focus on enhancing user experience through additional features such as advanced product filtering, personalized recommendations, and improved payment options. We also aim to foster a vibrant community of contributors, encouraging collaboration and innovation. Your ideas and skills can help us shape the future of PopShop!
We invite developers, designers, and anyone passionate about eCommerce to join us on this journey. Whether you want to contribute code, design elements, or simply share your ideas, your involvement is crucial to our success. Check out our [Contributing Guidelines](CONTRIBUTING.md) to get started, and let’s build something amazing together!
In closing, the journey of creating PopShop has been a rewarding experience filled with learning and growth. We are grateful for the contributions made so far and are excited about the potential that lies ahead. Together, we can cultivate a collaborative community where creativity thrives and innovation flourishes. Thank you for being a part of this adventure, and we look forward to seeing what we can achieve together!
## Project Development Analytics
### timeline gant
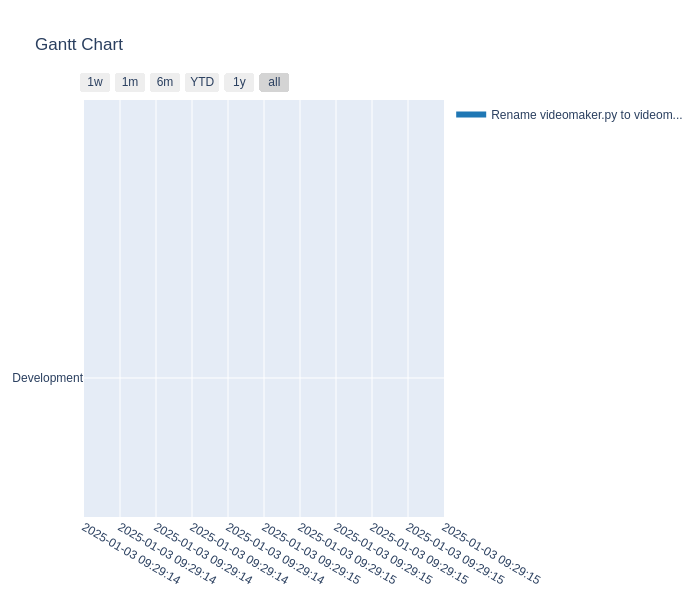
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
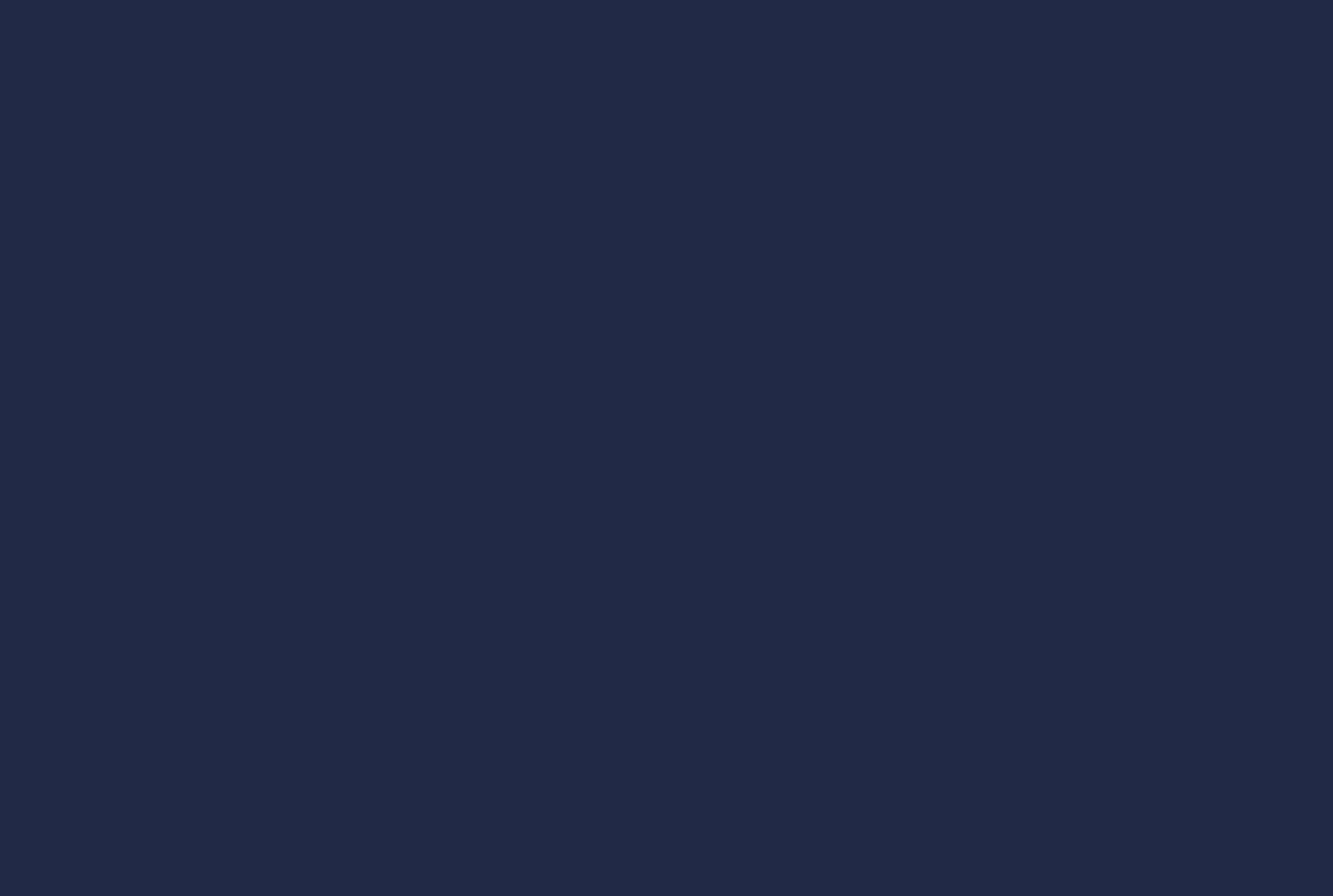
### Commit Activity Patterns
This chart shows when commits typically happen:
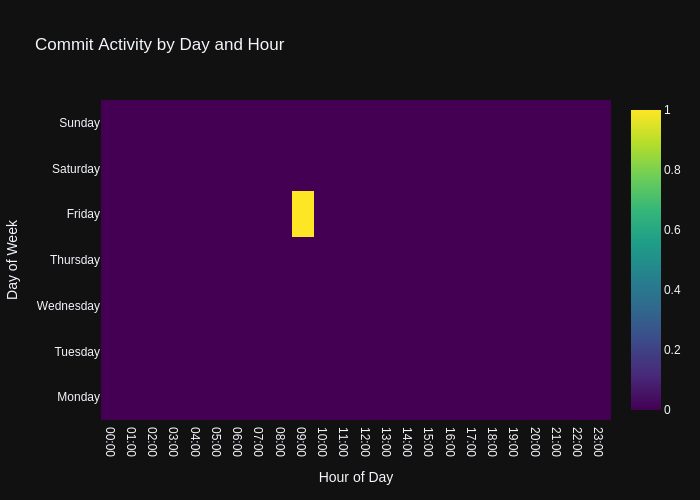
### Code Frequency
This chart shows the frequency of code changes over time:
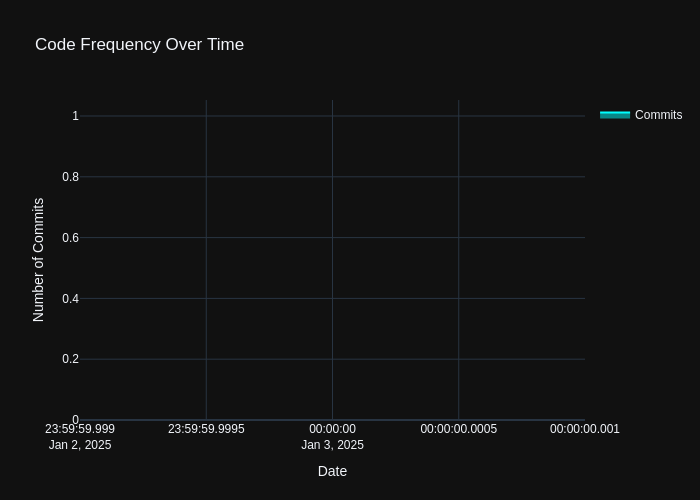
* Repository URL: [https://github.com/wanghaisheng/allthingssilk.com](https://github.com/wanghaisheng/allthingssilk.com)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日