Building Aipan-Netdisk-Search: Crafting a Vue-Powered Cloud Resource Hub
Project Genesis
Unleashing the Power of Aipan: My Journey into Netdisk Resource Search
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Frontend: Nuxt.js 3 and Vue 3 were chosen for their flexibility and performance. Nuxt.js provides server-side rendering capabilities, which enhances SEO and improves load times. TailwindCSS was selected for its utility-first approach, allowing for rapid UI development and customization.
-
Backend: Node.js v20.18.0 was chosen for its non-blocking architecture, which is ideal for handling multiple requests simultaneously. PostgreSQL was selected as the database due to its robustness and support for complex queries. Prisma ORM was integrated for its ease of use in database management and its ability to optimize connection pooling.
-
Authentication: JWT (JSON Web Token) was implemented for secure user authentication, providing a stateless and scalable solution.
3. Alternative Approaches Considered
-
Frontend Frameworks: While React was a strong contender, we ultimately chose Vue.js due to its simplicity and ease of integration with Nuxt.js. This combination allowed for rapid development without sacrificing performance.
-
Database Options: We evaluated NoSQL databases like MongoDB for their flexibility. However, we decided on PostgreSQL for its strong relational capabilities and support for complex data structures, which were essential for our resource management features.
-
Deployment Strategies: Initially, we considered traditional server deployments but opted for Vercel and Docker for their ease of use and scalability. Vercel’s seamless integration with frontend frameworks and Docker’s containerization capabilities provided a more efficient deployment process.
4. Key Insights That Shaped the Project
-
User-Centric Design: Early user feedback highlighted the importance of intuitive navigation and a clean interface. This led to iterative design improvements, ensuring that the user experience remained a top priority.
-
Performance Optimization: As we integrated multiple data sources, we realized the need for efficient data handling and caching strategies. This insight prompted the implementation of connection pooling and optimized API calls, resulting in faster response times.
-
Community Engagement: Engaging with potential users and contributors through discussions and feedback loops proved invaluable. This approach not only helped refine features but also fostered a sense of community around the project, encouraging contributions and support.
Under the Hood
Technical Deep-Dive: 爱盘-网盘资源搜索 Web
1. Architecture Decisions
Key Architectural Choices:
- Microservices Approach: The backend is structured to handle different functionalities as separate services, which can be independently developed and deployed. This is particularly useful for scaling specific features like resource management or user authentication.
- API-Driven Development: The application relies heavily on RESTful APIs for communication between the frontend and backend. This allows for a clear separation of concerns and enables the frontend to be developed independently of the backend.
- Database Management: PostgreSQL is chosen for its robustness and support for complex queries, which is essential for managing the diverse data types associated with cloud storage resources.
2. Key Technologies Used
Frontend:
- Nuxt.js 3: A powerful framework for Vue.js that enables server-side rendering and static site generation, improving performance and SEO.
- Vue 3: The progressive JavaScript framework for building user interfaces, allowing for reactive data binding and component-based architecture.
- TailwindCSS: A utility-first CSS framework that enables rapid UI development with a focus on customization and responsiveness.
- Element Plus: A Vue 3 UI library that provides a set of high-quality components for building user interfaces.
Backend:
- Node.js v20.18.0: A JavaScript runtime built on Chrome’s V8 engine, allowing for asynchronous event-driven programming, which is ideal for I/O-heavy applications.
- PostgreSQL: A powerful, open-source relational database system that supports advanced data types and performance optimization.
- Prisma ORM: An ORM that simplifies database interactions and provides type safety, making it easier to manage database migrations and queries.
- JWT Authentication: A stateless authentication mechanism that allows secure communication between the client and server.
3. Interesting Implementation Details
Resource Management
model Resource {
id Int @id @default(autoincrement())
name String
type String
url String
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
Batch Import/Export
const csv = require('csv-parser');
const fs = require('fs');
fs.createReadStream('path/to/file.csv')
.pipe(csv())
.on('data', (row) => {
// Process each row and save to the database
saveResource(row);
})
.on('end', () => {
console.log('CSV file successfully processed');
});
Admin Dashboard
Resource List
-
{{ resource.name }} - {{ resource.type }}
4. Technical Challenges Overcome
API Rate Limiting
Database Migration Management
User Authentication
Lessons from the Trenches
Key Technical Lessons Learned
-
Choosing the Right Tech Stack: The combination of Nuxt.js, Vue 3, and TailwindCSS for the frontend, along with Node.js and PostgreSQL for the backend, provided a robust and scalable architecture. Understanding the strengths of each technology helped in building a responsive and efficient application.
-
Database Management: Using Prisma ORM for database interactions simplified the process of managing database migrations and queries. The connection pool optimization and shared client instances improved performance and resource management.
-
Authentication and Security: Implementing JWT for authentication ensured secure access to the backend APIs. It’s crucial to understand the importance of secure authentication mechanisms in web applications.
-
Deployment Strategies: Offering multiple deployment options (Vercel, Docker, traditional) allowed flexibility for users with different preferences and environments. This approach can significantly enhance user adoption.
What Worked Well
-
User Interface and Experience: The use of TailwindCSS and Element Plus contributed to a clean and modern UI, making it user-friendly. The design choices facilitated easy navigation and resource management.
-
Feature Set: The inclusion of features like multi-source aggregation, online video playback, and a blog system provided comprehensive functionality that met user needs. The batch import/export feature was particularly well-received.
-
Documentation: The README file is well-structured, providing clear instructions for setup, deployment, and contribution. This clarity helps new developers onboard quickly and reduces the learning curve.
-
Community Engagement: Encouraging contributions and feedback through GitHub discussions and issues fostered a sense of community and collaboration, which is vital for open-source projects.
What You’d Do Differently
-
Enhanced Error Handling: While the project has a solid foundation, implementing more robust error handling and logging mechanisms could improve debugging and user experience, especially in production environments.
-
Automated Testing: Incorporating automated testing (unit and integration tests) from the beginning would help ensure code quality and reduce the likelihood of bugs during development and deployment.
-
Performance Optimization: While the project is functional, continuous performance monitoring and optimization should be a priority, especially as user traffic increases. This could include optimizing database queries and frontend performance.
-
User Feedback Loop: Establishing a more formalized process for gathering user feedback could help prioritize features and improvements based on actual user needs and experiences.
Advice for Others
-
Start with a Clear Plan: Before diving into development, outline the project scope, features, and technology stack. This helps in maintaining focus and aligning the team’s efforts.
-
Prioritize Documentation: Invest time in creating comprehensive documentation. This not only aids in onboarding new contributors but also serves as a reference for existing team members.
-
Embrace Community Contributions: Encourage contributions from the community. Open-source projects thrive on collaboration, and diverse input can lead to innovative solutions and improvements.
-
Iterate Based on Feedback: Be open to feedback and willing to iterate on features and design. User-centric development can lead to a more successful and widely adopted project.
-
Stay Updated: Technology evolves rapidly. Keep abreast of updates in the tech stack and best practices to ensure the project remains relevant and secure.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
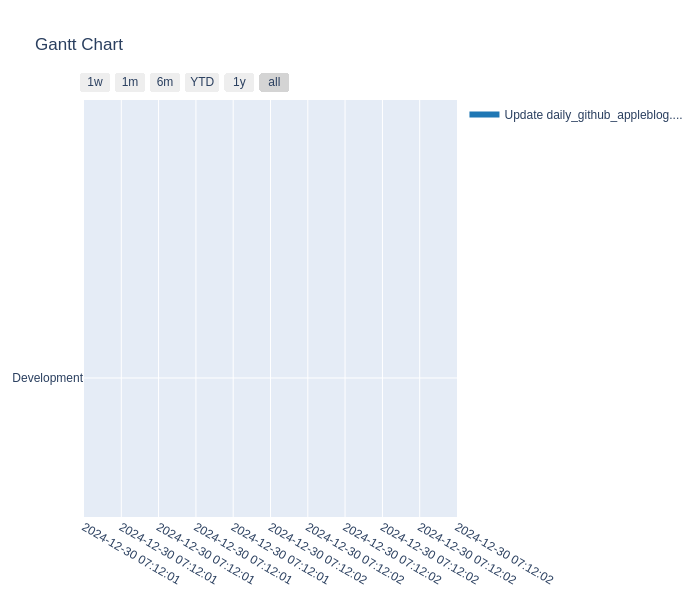
Commit Activity Heatmap
Contributor Network
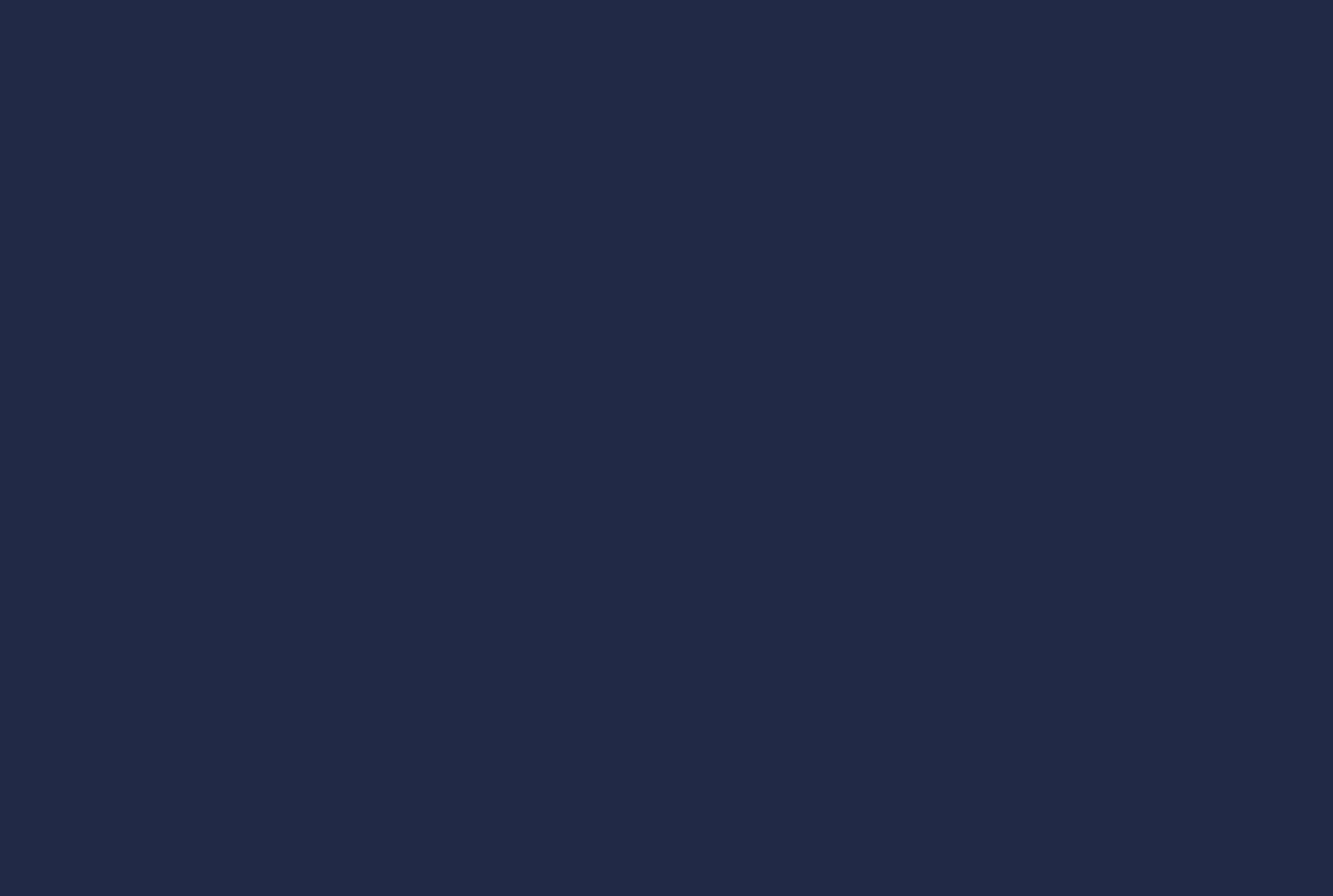
Commit Activity Patterns
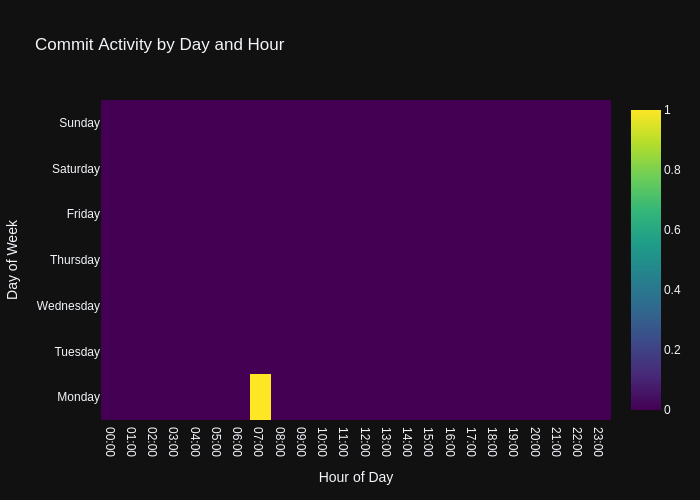
Code Frequency
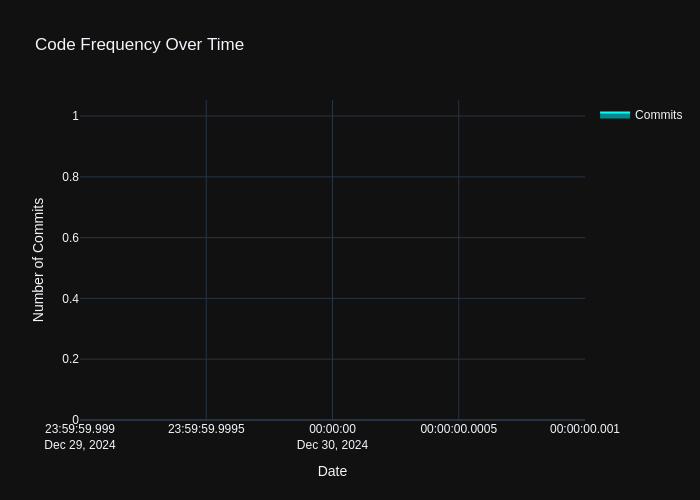
- Repository URL: https://github.com/wanghaisheng/aipan-netdisk-search
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日