From Idea to Reality: Building an AI Hedge Fund with Intelligent Agents
Project Genesis
Unleashing the Future of Finance: My Journey into AI Hedge Funds
From Idea to Implementation
Journey from Concept to Code: AI Hedge Fund Project
1. Initial Research and Planning
- How can AI effectively analyze market data to make informed trading decisions?
- What investment philosophies can be modeled through AI agents?
- What are the ethical implications of using AI in trading, and how can we ensure that the project remains educational and not for real trading?
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
-
Single-Agent System: Initially, there was a consideration to create a single agent that would encapsulate all investment strategies. However, this approach was quickly dismissed due to the complexity of integrating diverse methodologies into one agent. The multi-agent system was favored for its ability to compartmentalize strategies and allow for specialized analysis.
-
Rule-Based Trading: Another approach considered was a purely rule-based trading system, where predefined rules dictated trading decisions. While this could have simplified the implementation, it lacked the adaptability and learning capabilities that AI could provide. The decision was made to leverage machine learning to allow the agents to learn from historical data and improve their decision-making over time.
-
Real Trading Implementation: There was initial interest in creating a system that could execute real trades. However, this was deemed too risky and outside the project’s educational scope. The focus shifted to simulating trading decisions, allowing users to learn from the system without the financial risks associated with real trading.
4. Key Insights That Shaped the Project
-
Diversity of Strategies: The realization that different investment philosophies could complement each other led to the decision to implement multiple agents. This diversity not only enriched the analysis but also provided a more balanced approach to trading decisions.
-
Importance of Data Quality: The project underscored the critical role of high-quality data in making informed trading decisions. The choice of reliable data sources was pivotal in ensuring that the agents could generate accurate trading signals.
-
User Education: The project reinforced the importance of user education in the realm of AI and finance. By focusing on an educational approach, the project aimed to demystify AI-driven trading and empower users to understand the underlying principles of investment strategies.
-
Ethical Considerations: The journey highlighted the ethical implications of using AI in finance. The commitment to keeping the project educational and non-commercial was a guiding principle that shaped many of the technical and design decisions.
Under the Hood
Technical Deep-Dive: AI Hedge Fund
1. Architecture Decisions
Key Components:
- Agents: Each agent encapsulates a specific investment strategy, such as value investing (Ben Graham Agent) or growth investing (Cathie Wood Agent). This separation of concerns allows for independent development and testing of each agent.
- Risk Management: A dedicated Risk Manager agent ensures that the system adheres to predefined risk metrics and position limits, which is crucial for maintaining a balanced portfolio.
- Portfolio Management: The Portfolio Manager agent acts as the decision-maker, synthesizing inputs from all agents to make final trading decisions.
Workflow:
- Data Input: Financial data is fetched using API keys set in the environment variables.
- Agent Execution: Each agent processes the data according to its strategy and generates trading signals.
- Risk Assessment: The Risk Manager evaluates the signals and sets position limits.
- Decision Making: The Portfolio Manager consolidates the signals and makes the final trading decision.
2. Key Technologies Used
- Python: The primary programming language used for developing the hedge fund system, chosen for its rich ecosystem of libraries and ease of use.
- Poetry: A dependency management tool for Python that simplifies package management and project setup.
- APIs: The system integrates with various APIs for financial data and LLMs (Large Language Models) from OpenAI and Groq, enabling advanced data analysis and decision-making capabilities.
- Environment Variables: Sensitive information such as API keys is managed through environment variables, enhancing security and configurability.
Example of Setting Up Environment Variables:
# Create .env file for your API keys
cp .env.example .env
# Set your API keys
OPENAI_API_KEY=your-openai-api-key
GROQ_API_KEY=your-groq-api-key
FINANCIAL_DATASETS_API_KEY=your-financial-datasets-api-key
3. Interesting Implementation Details
Agent Implementation
valuation.py
agent might look like this:# src/agents/valuation.py
class ValuationAgent:
def __init__(self, data):
self.data = data
def calculate_intrinsic_value(self):
# Implement valuation logic here
intrinsic_value = self.data['earnings'] * 15 # Simplified example
return intrinsic_value
def generate_signal(self):
intrinsic_value = self.calculate_intrinsic_value()
if self.data['current_price'] < intrinsic_value:
return "BUY"
else:
return "SELL"
Backtesting
backtester.py
script is designed to simulate trades based on past data:# src/backtester.py
def backtest_strategy(ticker, start_date, end_date):
# Fetch historical data
historical_data = fetch_historical_data(ticker, start_date, end_date)
results = []
for date, data in historical_data.items():
signal = run_agents(data)
results.append((date, signal))
return results
4. Technical Challenges Overcome
API Integration
# src/tools/api.py
def fetch_data(api_url):
try:
response = requests.get(api_url)
response.raise_for_status()
return response.json()
except requests.exceptions.RequestException as e:
print(f"API request failed: {e}")
return None
Performance Optimization
Example of Asynchronous Data Fetching:
import asyncio
import aiohttp
async def fetch_data_async(session, url):
async with session.get(url) as response:
return await response.json()
async def main(urls):
async with aiohttp.ClientSession() as session:
tasks = [fetch_data_async(session, url) for url in urls]
return await asyncio.gather(*tasks)
# Usage
urls = ["https://api.example.com/data1", "https://api.example.com/data2"]
data = asyncio.run(main(url
## Lessons from the Trenches
### Key Technical Lessons Learned
1. **Modular Design**: The project benefits from a modular architecture where each agent has a specific role (e.g., valuation, sentiment analysis, risk management). This separation of concerns makes it easier to maintain and extend the system. Future enhancements can be made by adding new agents or modifying existing ones without affecting the overall system.
2. **API Integration**: Successfully integrating multiple APIs (OpenAI, Groq, Financial Datasets) highlighted the importance of handling API keys securely and managing dependencies effectively. Using environment variables for sensitive information is a best practice that enhances security.
3. **Backtesting Framework**: Implementing a backtesting framework allowed for the evaluation of trading strategies against historical data. This is crucial for validating the effectiveness of the agents before deploying them in a live environment.
4. **Data Handling**: Managing financial data efficiently is key. The project demonstrated the need for robust data handling and preprocessing techniques to ensure that the agents receive clean and relevant data for analysis.
5. **Risk Management**: The inclusion of a risk manager agent underscored the importance of risk assessment in trading strategies. It is essential to quantify risk and set position limits to protect against significant losses.
### What Worked Well
1. **Agent Collaboration**: The interaction between different agents (e.g., combining insights from sentiment, fundamentals, and technical analysis) provided a comprehensive view of potential trading opportunities. This collaborative approach led to more informed decision-making.
2. **User-Friendly Interface**: The command-line interface (CLI) for running the hedge fund and backtester was intuitive. The ability to specify tickers, date ranges, and reasoning outputs made it easy for users to interact with the system.
3. **Educational Focus**: The project’s emphasis on educational purposes resonated well with users. Providing clear disclaimers and documentation helped set appropriate expectations and encouraged learning.
4. **Community Engagement**: The inclusion of a contributing guide and feature request section fostered community involvement. This can lead to a more vibrant ecosystem around the project, with users contributing improvements and new features.
### What You'd Do Differently
1. **Enhanced Documentation**: While the README is informative, additional documentation, such as a detailed user guide or tutorials, could help new users understand the system better. Including examples of how to interpret agent outputs would also be beneficial.
2. **Testing Framework**: Implementing a comprehensive testing framework (unit tests, integration tests) would improve code reliability and facilitate easier debugging. This is especially important in financial applications where errors can have significant consequences.
3. **Performance Optimization**: As the project scales, performance may become an issue, especially with multiple agents processing data simultaneously. Profiling the code and optimizing bottlenecks would be a priority in future iterations.
4. **User Interface**: While the CLI is functional, developing a graphical user interface (GUI) could enhance user experience, making it more accessible to those who may not be comfortable with command-line tools.
### Advice for Others
1. **Start Small**: If you're new to building complex systems, start with a small, focused project. Gradually add features and complexity as you become more comfortable with the technologies involved.
2. **Focus on Modularity**: Design your system with modularity in mind. This will make it easier to test, maintain, and extend your project over time.
3. **Prioritize Security**: Always prioritize security when handling sensitive information, such as API keys. Use environment variables and avoid hardcoding sensitive data in your codebase.
4. **Engage with the Community**: Don’t hesitate to seek feedback and engage with the community. Open-source projects thrive on collaboration, and community input can lead to valuable improvements.
5. **Document Everything**: Good documentation is crucial for any project. It not only helps others understand your work but also serves as a reference for you in the future.
## What's Next?
## Conclusion
As we reach the current milestone of our AI Hedge Fund project, we are excited to share that our proof of concept has successfully integrated multiple AI agents, each embodying distinct investment philosophies. From the value-driven insights of the Ben Graham Agent to the innovative strategies of the Cathie Wood Agent, our system is designed to simulate trading decisions and provide a comprehensive educational experience. The initial testing phase has yielded promising results, showcasing the potential of AI in analyzing market sentiment, fundamentals, and technical indicators.
Looking ahead, our development plans are ambitious. We aim to enhance the capabilities of our agents by incorporating more sophisticated machine learning algorithms and expanding our dataset to include a broader range of financial instruments. Additionally, we plan to implement a user-friendly interface that will allow contributors to interact with the system more intuitively. We invite you to join us on this journey by contributing your ideas, code, or feedback. Your involvement can help shape the future of this project and push the boundaries of what AI can achieve in the realm of finance.
As we reflect on this side project journey, we are reminded of the power of collaboration and innovation. This endeavor has not only deepened our understanding of AI and finance but has also fostered a vibrant community of learners and contributors. We encourage you to explore the project, experiment with the code, and share your insights. Together, we can unlock new possibilities and pave the way for the next generation of AI-driven investment strategies.
Thank you for being a part of the AI Hedge Fund project. Let’s continue to learn, innovate, and grow together!
## Project Development Analytics
### timeline gant
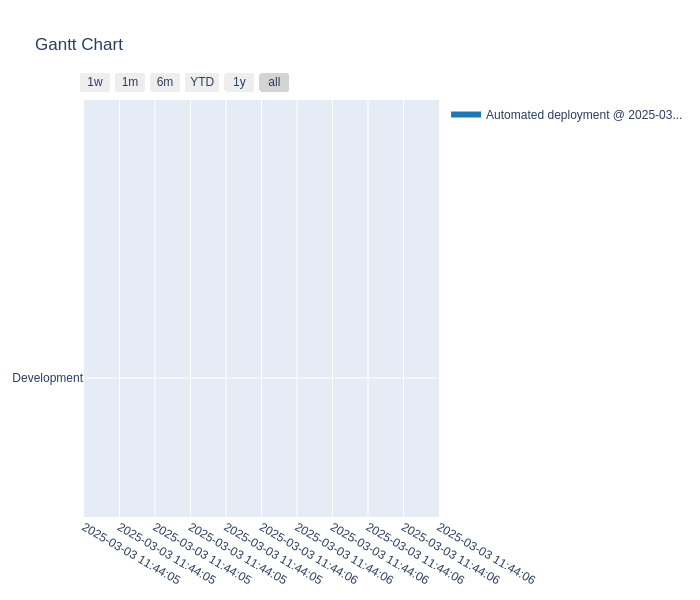
### Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
![Commit Heatmap]()
### Contributor Network
This network diagram shows how different contributors interact:
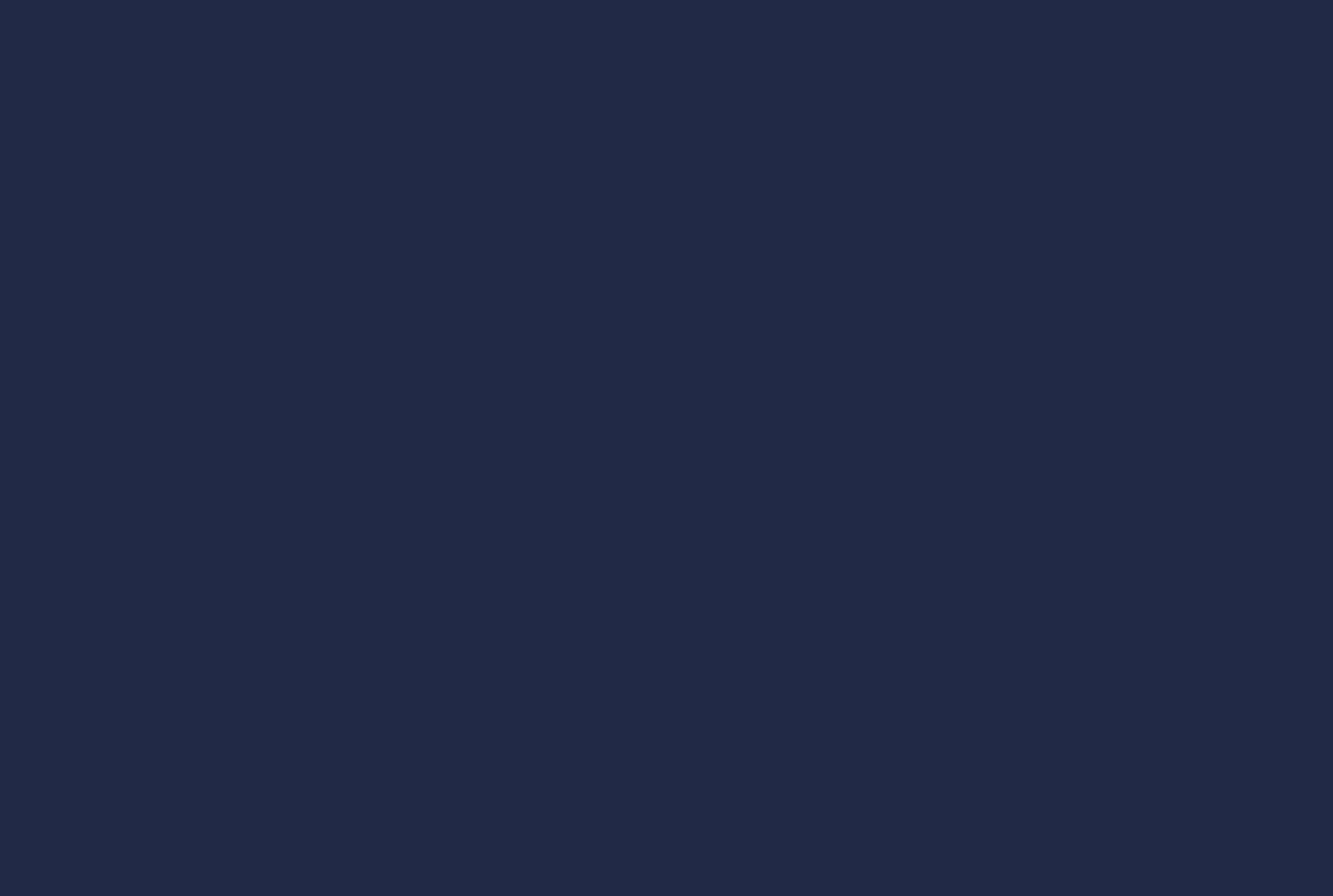
### Commit Activity Patterns
This chart shows when commits typically happen:
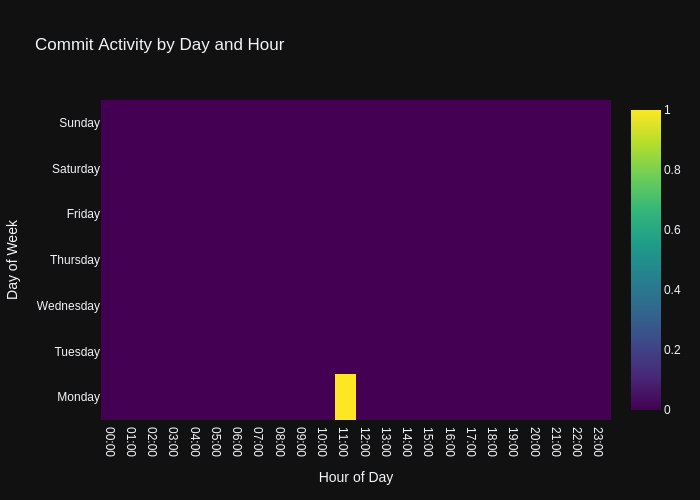
### Code Frequency
This chart shows the frequency of code changes over time:
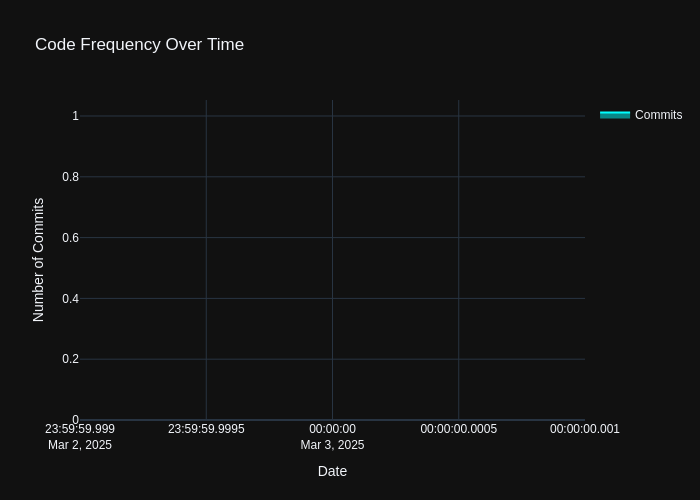
* Repository URL: [https://github.com/wanghaisheng/ai-hedge-fund](https://github.com/wanghaisheng/ai-hedge-fund)
* Stars: **0**
* Forks: **0**
编辑整理: Heisenberg 更新日期:2025 年 3 月 10 日