From Idea to Reality: Crafting AI-Generated Cards with ai-gacha
Project Genesis
Unleashing Creativity: My Journey into AI Gacha
From Idea to Implementation
AI Gacha Project Journey: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
3. Alternative Approaches Considered
4. Key Insights That Shaped the Project
-
User-Centric Design: Engaging with potential users early in the process highlighted the importance of user experience. We learned that players value customization options, so we incorporated features that allow users to tweak parameters for card generation, such as character traits and backgrounds.
-
Iterative Development: Embracing an iterative development approach allowed us to refine our features based on user feedback continuously. This flexibility enabled us to pivot quickly when certain features did not resonate with users or when new ideas emerged.
-
Community Engagement: Building a community around the project was essential. We created channels for users to share their generated cards and provide feedback, fostering a sense of ownership and encouraging further engagement with the application.
Under the Hood
Technical Deep-Dive: AI Gacha Project
1. Architecture Decisions
- Frontend: A user interface that allows users to interact with the application, input parameters for card generation, and view the generated cards.
- Backend: A server that handles requests from the frontend, processes the input, and interacts with the AI model to generate images.
- AI Model: The Pastel Mix model, which is responsible for generating the anime-style images based on user inputs.
Design Choices
- Microservices Architecture: The project can be structured as a microservice, where the frontend and backend are decoupled. This allows for easier scaling and maintenance.
- RESTful API: The backend exposes a RESTful API for the frontend to communicate with, making it easier to manage requests and responses.
- Asynchronous Processing: Given the potentially long processing time for image generation, the backend can implement asynchronous processing to improve user experience.
2. Key Technologies Used
- Frontend: The frontend can be built using frameworks like React or Vue.js, which provide a responsive and dynamic user interface.
- Backend: The backend can be developed using Node.js with Express.js, which allows for easy handling of HTTP requests and integration with the AI model.
- AI Model: The Pastel Mix model, hosted on Hugging Face, is utilized for generating the anime-style images. This model leverages deep learning techniques to produce high-quality outputs.
- Database: A database (e.g., MongoDB or PostgreSQL) can be used to store user data, generated images, and any other relevant information.
3. Interesting Implementation Details
Image Generation Process
- User Input: The user provides parameters such as character traits, background, and style preferences through the frontend.
- API Request: The frontend sends a request to the backend with the user input.
- Model Invocation: The backend processes the request and invokes the Pastel Mix model with the provided parameters.
- Image Retrieval: Once the model generates the image, the backend retrieves it and sends it back to the frontend for display.
Example Code Snippet
const express = require('express');
const axios = require('axios');
const app = express();
app.use(express.json());
app.post('/generate-card', async (req, res) => {
const { traits } = req.body;
try {
const response = await axios.post('https://api.huggingface.co/pastel-mix/generate', {
inputs: traits,
});
const generatedImage = response.data.image_url;
res.json({ imageUrl: generatedImage });
} catch (error) {
res.status(500).json({ error: 'Image generation failed' });
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
4. Technical Challenges Overcome
Model Integration
Performance Optimization
User Experience
Example of Asynchronous Processing
const Queue = require('bull');
const imageQueue = new Queue('image generation');
imageQueue.process(async (job) => {
const { traits } = job.data;
// Call the AI model to generate the image
const generatedImage = await generateImage(traits);
return generatedImage;
});
// Endpoint to add a job to the queue
app.post('/generate-card', async (req, res) => {
const { traits } = req.body;
const job = await imageQueue.add({ traits });
res.json({ jobId: job.id });
});
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Model Selection: Choosing the right AI model is crucial. The Pastel Mix model was effective for generating anime-style cards, but exploring other models could yield different artistic styles and quality.
- Integration Challenges: Integrating AI-generated content into a mobile game can be complex. Ensuring that the generated cards fit seamlessly into the game’s existing art style and mechanics requires careful planning and testing.
- Performance Optimization: Generating images on-the-fly can be resource-intensive. Implementing caching mechanisms or pre-generating cards can improve performance and user experience.
- User Feedback Loop: Incorporating user feedback on generated cards can help refine the model’s output and improve overall satisfaction with the generated content.
2. What Worked Well
- AI Generation Quality: The use of the Pastel Mix model produced high-quality, visually appealing cards that resonated well with users.
- User Engagement: The novelty of AI-generated cards attracted users, leading to increased engagement and interest in the project.
- Automation of Art Creation: Automating the card creation process saved time and resources, allowing the team to focus on other aspects of game development.
- Continuous Integration: The implementation of CI/CD pipelines (as indicated by the test badge) helped maintain code quality and streamline updates.
3. What You’d Do Differently
- User Customization Options: Allowing users to customize certain aspects of the card generation (e.g., colors, themes) could enhance user satisfaction and engagement.
- Diverse Model Testing: Experimenting with multiple AI models for different styles could provide a broader range of card designs and appeal to a wider audience.
- Scalability Considerations: Planning for scalability from the beginning would help accommodate a growing user base without performance degradation.
- Documentation and Tutorials: Providing more comprehensive documentation and tutorials for users on how to use the AI features could improve user experience and adoption.
4. Advice for Others
- Start Small: Begin with a minimal viable product (MVP) to test the concept before investing heavily in features. Gather user feedback early to guide development.
- Focus on User Experience: Prioritize user experience in the design and functionality of the AI-generated content. Ensure that the generated cards are not only visually appealing but also fit well within the game’s context.
- Iterate Based on Feedback: Be open to iterating on your project based on user feedback. Continuous improvement is key to maintaining user interest and satisfaction.
- Stay Updated on AI Trends: The field of AI is rapidly evolving. Stay informed about new models and techniques that could enhance your project and keep it competitive.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
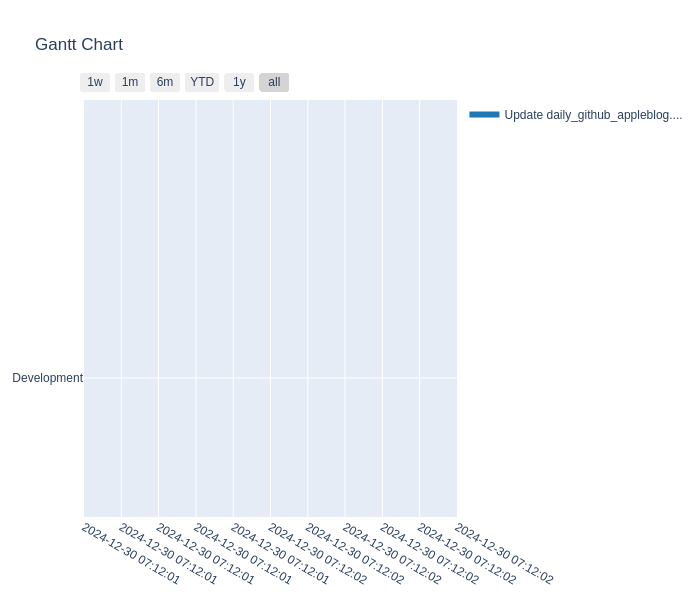
Commit Activity Heatmap
Contributor Network
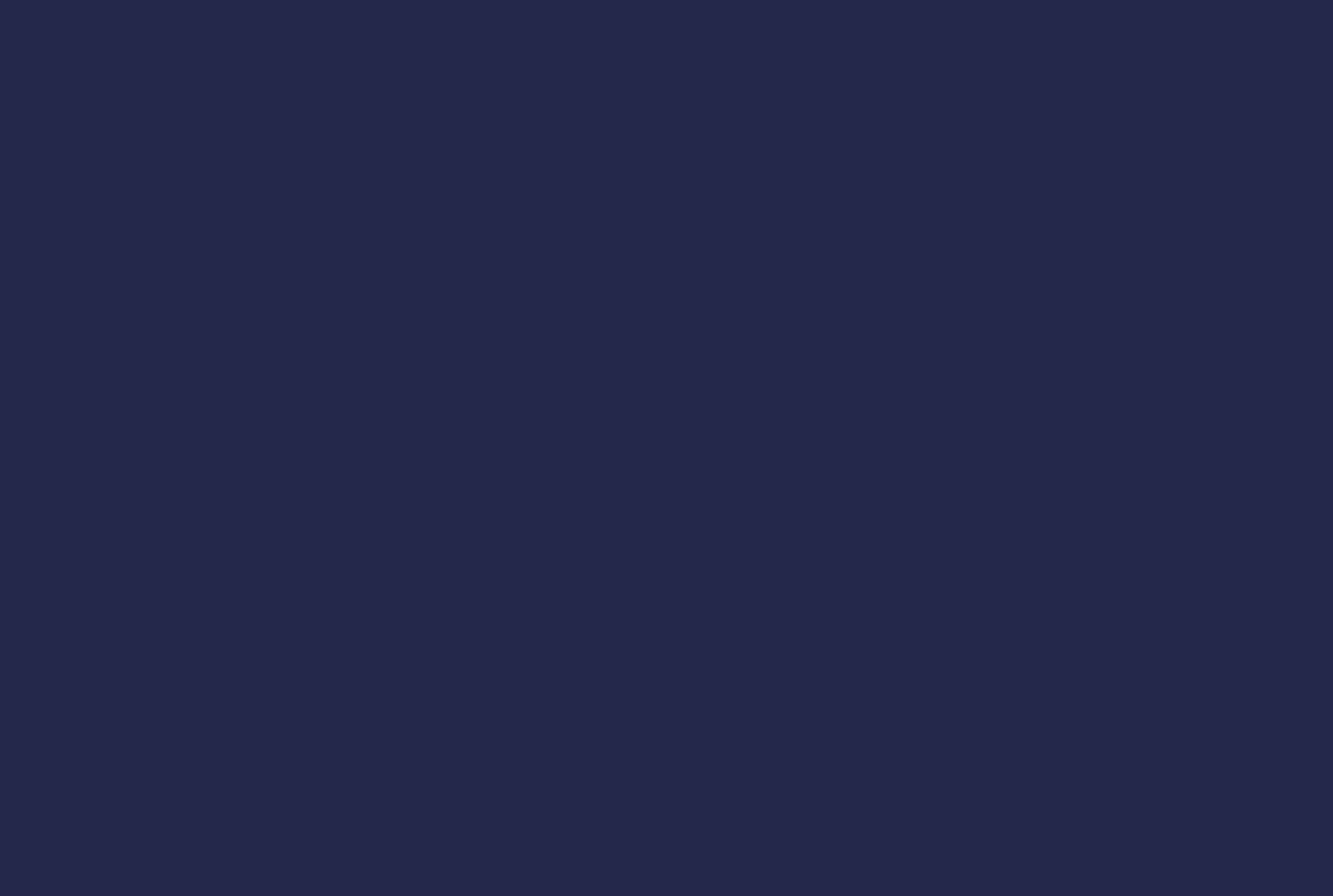
Commit Activity Patterns
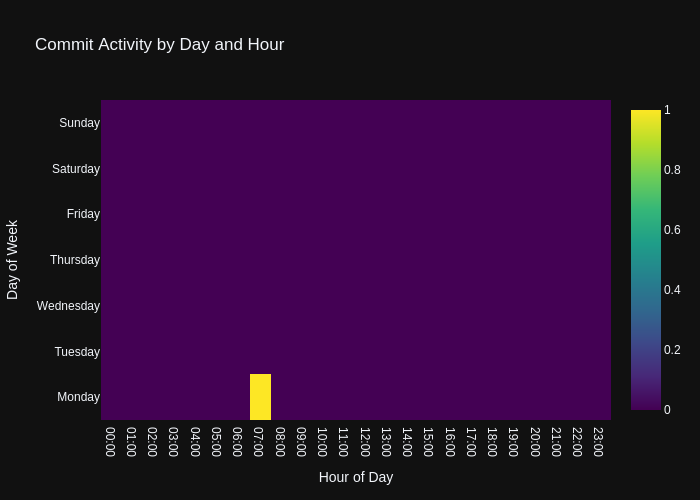
Code Frequency
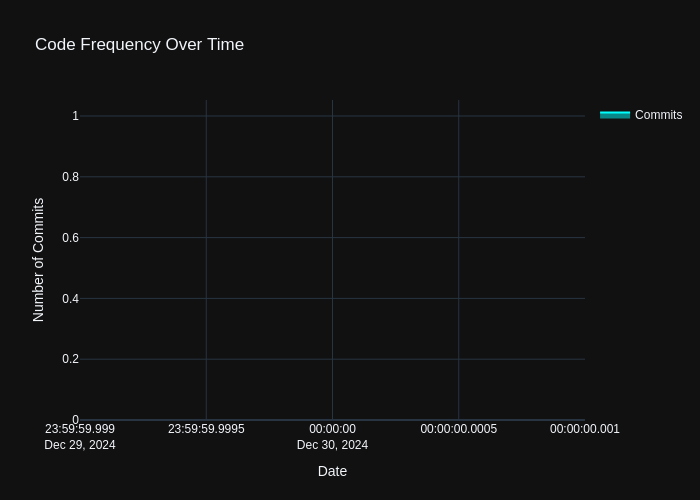
- Repository URL: https://github.com/wanghaisheng/ai-gacha
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日