From Idea to Reality: Building a_websim-website-starter with GPT Magic
Project Genesis
Building a Better Web Experience: My Journey with a_websim Website Starter
From Idea to Implementation
1. Initial Research and Planning
- SEO Best Practices: Understanding the importance of sitemaps, metadata, and mobile responsiveness.
- User Experience: Ensuring the site is accessible and engaging across devices.
- Performance Metrics: The need for analytics tools to track user behavior and site performance.
- Progressive Web App (PWA) Features: Exploring how PWAs can enhance user experience through offline capabilities and faster load times.
2. Technical Decisions and Their Rationale
-
Sitemap Generation: Implementing an automated sitemap generator based on language subfolders and HTML files was crucial for SEO. This decision was driven by the need to ensure that search engines could easily crawl and index the site.
-
SEO Checks: Integrating automated checks for SEO requirements helped avoid common pitfalls like Google redirection issues and non-indexable pages. This proactive approach aimed to enhance the site’s visibility from the outset.
-
IndexNow Submission: The choice to use IndexNow for URL submission to Google was based on its efficiency in notifying search engines about content updates, thereby improving indexing speed.
-
Responsive Design: Prioritizing a mobile-responsive design was essential, given the increasing number of users accessing websites via mobile devices. This decision was informed by user behavior trends and Google’s mobile-first indexing approach.
-
Analytics Integration: Incorporating Google Analytics and Microsoft Clarity provided valuable insights into user interactions, allowing for data-driven decisions to improve the site over time.
3. Alternative Approaches Considered
-
Manual Sitemap Management: Initially, there was a consideration to manage sitemaps manually. However, this approach was quickly dismissed due to the potential for human error and the inefficiency of keeping sitemaps updated as content changes.
-
Third-Party SEO Tools: While there are numerous third-party SEO tools available, the decision was made to build in-house solutions for SEO checks and keyword research. This allowed for greater customization and integration with the site’s specific needs.
-
Static vs. Dynamic Content: The team debated between using static content versus a dynamic content management system. Ultimately, a hybrid approach was chosen to leverage the benefits of both, allowing for flexibility in content updates while maintaining performance.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of designing with the user in mind became clear early on. Features like mobile responsiveness and intuitive navigation were prioritized to enhance user experience.
-
SEO as a Continuous Process: The realization that SEO is not a one-time task but an ongoing process shaped the decision to include automated checks and analytics. This ensures that the site can adapt to changing SEO standards and user behavior.
-
Integration of Tools: The value of integrating various tools (like analytics and keyword research) into a cohesive system was recognized. This integration allows for a more streamlined workflow and better data utilization.
-
Emphasis on Automation: The need for automation in tasks like sitemap generation and URL submission was highlighted as a way to reduce manual workload and minimize errors, ultimately leading to a more efficient development process.
Under the Hood
Technical Deep-Dive: WebSim Site Builder
1. Architecture Decisions
-
Modular Design: Each feature is encapsulated in its own module, making it easier to maintain and extend. For example, the SEO module handles all SEO-related tasks, while the sitemap generator is a separate module.
-
Microservices Approach: Some functionalities, such as image generation and blog text generation, can be implemented as microservices. This allows for independent scaling and deployment of these services.
-
Responsive Design: The site is built with a mobile-first approach, ensuring that it is responsive across devices. This decision is crucial for user experience and SEO.
-
Use of APIs: The architecture leverages external APIs for functionalities like Google Analytics and Microsoft Clarity, allowing for rich analytics without heavy lifting on the server side.
2. Key Technologies Used
-
HTML/CSS/JavaScript: The foundational technologies for building the web interface.
-
Node.js: Used for server-side scripting, enabling the handling of requests and responses efficiently.
-
Express.js: A web application framework for Node.js that simplifies routing and middleware integration.
-
Google Indexing API: Utilized for submitting URLs to Google, enhancing the site’s visibility.
-
Progressive Web App (PWA) Technologies: Service workers and manifest files are used to enable offline capabilities and improve performance.
-
SEO Libraries: Libraries for generating sitemaps and checking SEO requirements, such as
sitemap-generator
andseo-checker
.
3. Interesting Implementation Details
Auto-Generating Sitemap
.html
files.const fs = require('fs');
const path = require('path');
function generateSitemap(dir, lang) {
let sitemap = [];
fs.readdirSync(dir).forEach(file => {
const fullPath = path.join(dir, file);
if (fs.statSync(fullPath).isDirectory()) {
sitemap = sitemap.concat(generateSitemap(fullPath, lang));
} else if (file.endsWith('.html')) {
sitemap.push(`/${lang}/${file}`);
}
});
return sitemap;
}
SEO Requirements Checker
const checkSEO = (htmlContent) => {
const missingMetaTags = [];
if (!/<meta name="description"/.test(htmlContent)) {
missingMetaTags.push('Missing description meta tag');
}
// Additional checks...
return missingMetaTags;
};
4. Technical Challenges Overcome
Handling Google Indexing
const { google } = require('googleapis');
const indexing = google.indexing('v3');
async function submitUrl(url) {
const auth = await authenticate(); // OAuth 2.0 authentication
await indexing.urlNotifications.publish({
auth,
requestBody: {
url: url,
type: 'URL_UPDATED',
},
});
}
Responsive Design Implementation
/* Mobile-first styles */
body {
font-size: 16px;
}
/* Tablet styles */
@media (min-width: 768px) {
body {
font-size: 18px;
}
}
/* Desktop styles */
@media (min-width: 1024px) {
body {
font-size: 20px;
}
}
Image Generation
const generateImage = async (type) => {
const response = await fetch(`https://api.imagegen.com/generate?type=${type}`);
const imageBlob = await response.blob();
return URL.createObjectURL(imageBlob);
};
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Automation is Key: Automating tasks such as sitemap generation, SEO checks, and URL submissions significantly reduces manual effort and minimizes human error. Implementing tools like Websim for automation can streamline the development process.
-
SEO Best Practices: Understanding and implementing SEO requirements early in the project helped avoid common pitfalls like Google redirection issues and indexing problems. Regularly checking SEO metrics is crucial for maintaining site visibility.
-
Responsive Design: Ensuring that the site is responsive for both PC and mobile users is essential. This requires thorough testing across different devices and screen sizes to ensure a consistent user experience.
-
Integration of Analytics: Incorporating tools like Google Analytics and Microsoft Clarity from the start provided valuable insights into user behavior, which informed further development and optimization.
-
PWA Implementation: Adding Progressive Web App (PWA) support enhanced user engagement and performance, allowing users to access the site offline and receive push notifications.
What Worked Well
-
Sitemap Generation: The automatic generation of sitemaps based on language subfolders and HTML files was effective in improving site navigation and SEO.
-
Keyword Research Tools: Utilizing tools like SpyFu for keyword research helped identify valuable keywords and trends, which informed content creation and optimization strategies.
-
Image Generation: Automating the generation of logos and cover images saved time and ensured consistency in branding.
-
Blog Text Generation: Integrating the auto-blog feature using G4F streamlined content creation, allowing for regular updates and engagement with users.
What You’d Do Differently
-
More Comprehensive Testing: While the site was responsive, more extensive testing across various devices and browsers could have identified additional issues earlier in the development process.
-
User Feedback Loop: Establishing a more structured feedback loop with users could have provided insights into usability and areas for improvement, leading to a more user-centered design.
-
Documentation: Improving documentation throughout the development process would facilitate easier onboarding for new developers and provide clearer guidance on project features and functionalities.
-
SEO Monitoring Tools: Implementing ongoing SEO monitoring tools earlier in the project could have helped in continuously optimizing the site based on real-time data.
Advice for Others
-
Start with a Clear Plan: Before diving into development, outline a clear plan that includes features, timelines, and responsibilities. This will help keep the project organized and on track.
-
Leverage Automation: Use automation tools wherever possible to save time and reduce errors. This includes everything from sitemap generation to SEO checks and content creation.
-
Prioritize SEO from the Start: Integrate SEO best practices into the development process from the beginning to avoid costly rework later on.
-
Engage with Users: Regularly seek feedback from users to understand their needs and preferences. This will help create a more user-friendly and effective site.
-
Stay Updated on Trends: The web development and SEO landscapes are constantly evolving. Stay informed about the latest trends and technologies to keep your project relevant and competitive.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
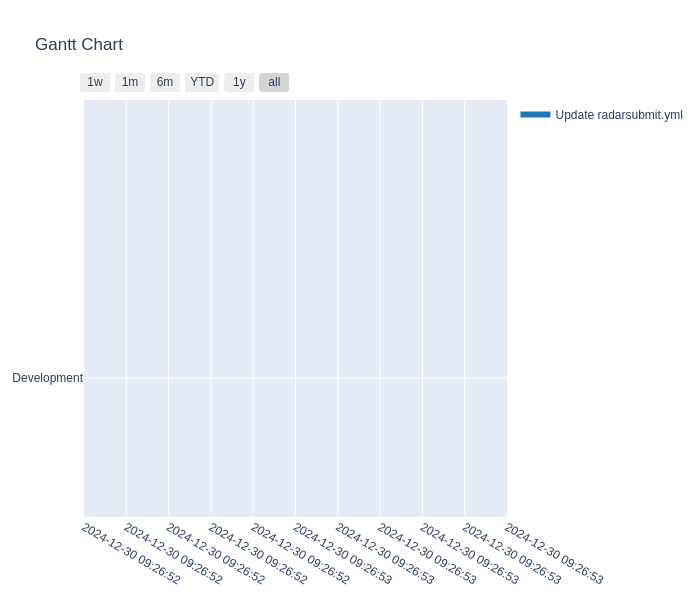
Commit Activity Heatmap
Contributor Network
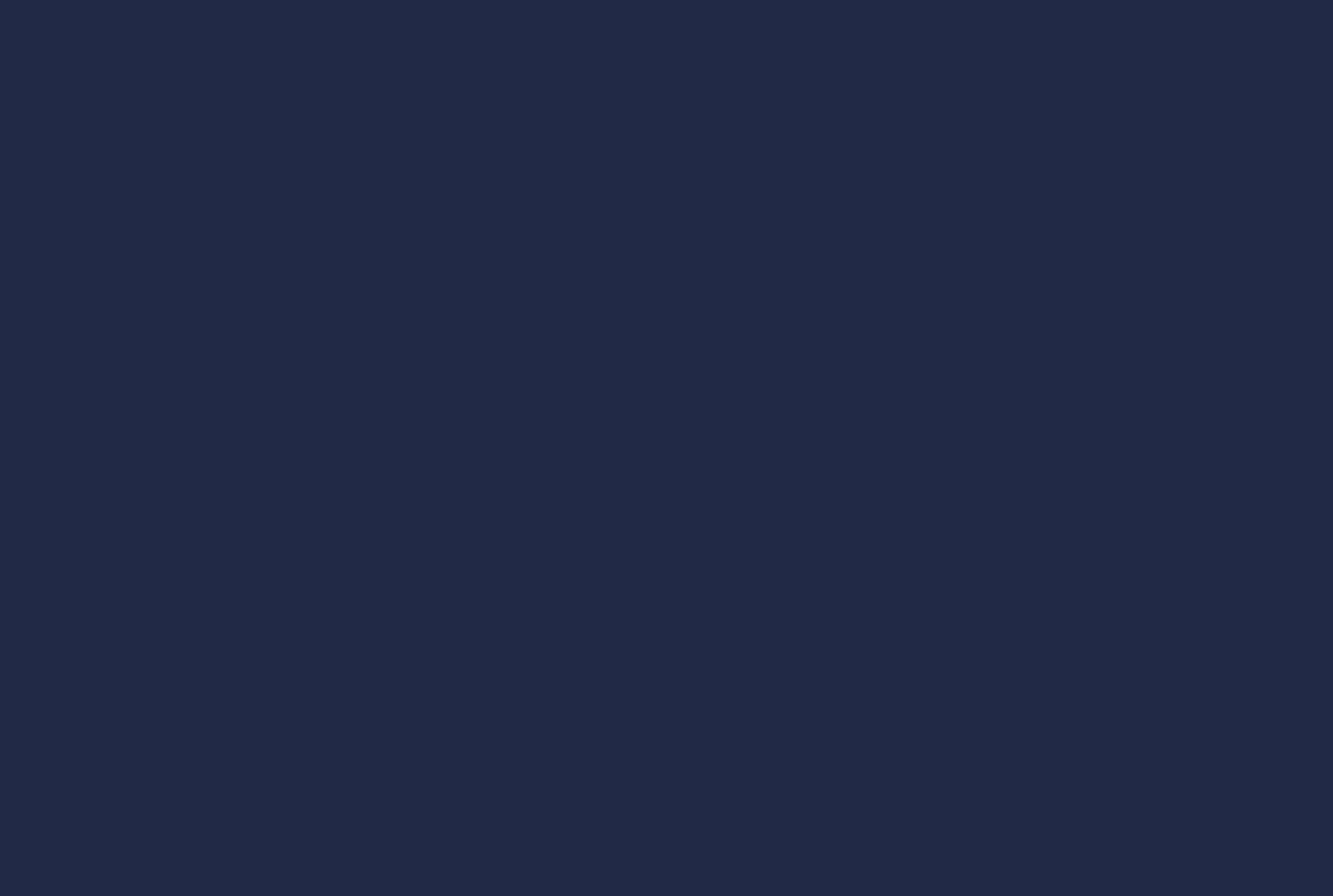
Commit Activity Patterns
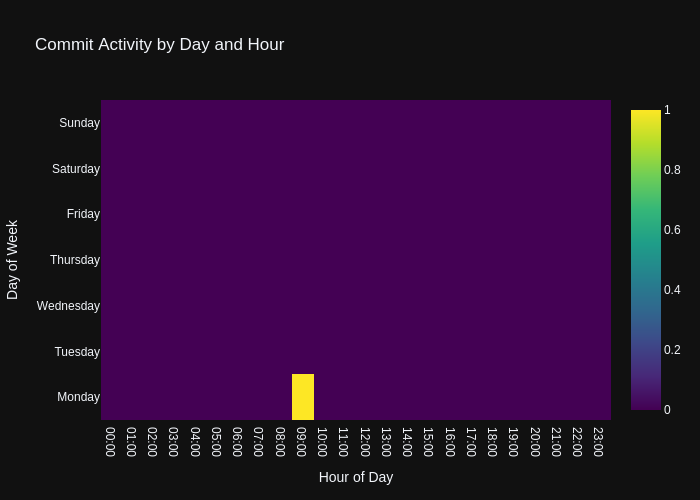
Code Frequency
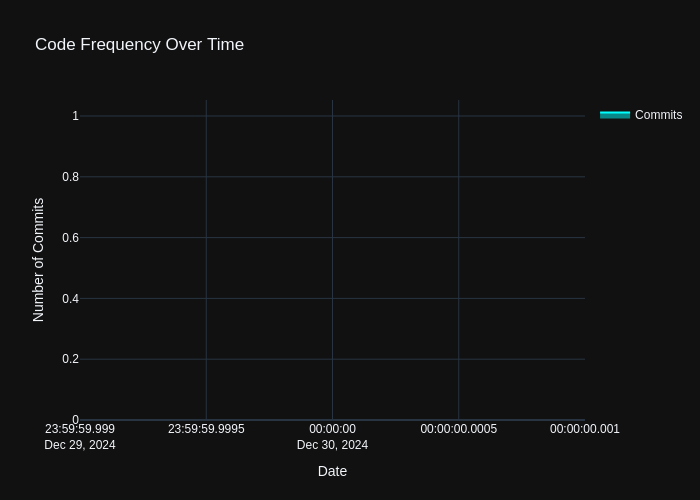
- Repository URL: https://github.com/wanghaisheng/a_websim-website-starter
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日