Building a Modular Sofa Store: My Journey with React and Node.js
Project Genesis
Discovering Comfort: My Journey to Creating a Modular Sectional Sofa Store
From Idea to Implementation
Initial Research and Planning
Technical Decisions and Their Rationale
Alternative Approaches Considered
Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-centric approach became evident early on. Feedback from potential users emphasized the need for intuitive navigation and a visually appealing interface. This insight drove our design decisions and led to iterative testing with real users.
-
Scalability and Modularity: As we built out the architecture, the need for scalability became apparent. By adopting a modular approach with npm workspaces, we ensured that each component could evolve independently, making it easier to add features and maintain the codebase.
-
Community Engagement: The research highlighted the value of community features, such as user reviews and recommendations. This insight led to the integration of social elements within the platform, fostering a sense of belonging among users and encouraging interaction.
-
Continuous Deployment: The decision to implement CI/CD practices through GitHub Actions for deployment was driven by the need for rapid iteration and testing. This approach allowed us to deploy changes frequently and reliably, ensuring that the platform could evolve based on user feedback.
Under the Hood
Technical Deep-Dive: Books About Food
1. Architecture Decisions
Microservices Structure
- Next.js Frontend: Serves the user-facing application.
- Admin Backend: Manages administrative tasks and connects to external services.
- Core Services: Contains shared business logic and APIs.
- Database: Manages database interactions using Prisma.
- End to End Tests: Ensures the application works as expected through automated testing.
- Email: Handles email templates and previews.
- Jobs: Manages asynchronous tasks.
2. Key Technologies Used
- Next.js: A React framework that enables server-side rendering and static site generation, improving performance and SEO.
- React.js: A JavaScript library for building user interfaces, allowing for a component-based architecture.
- Tailwind CSS: A utility-first CSS framework that enables rapid UI development with a focus on responsiveness.
- Prisma.io: An ORM that simplifies database interactions and provides type safety.
- Inngest: A serverless function platform that allows for easy background job management.
- Mailing.run: A service for managing email templates and sending emails.
Example of Next.js Page Component
import React from 'react';
const RecipePage = ({ recipe }) => {
return (
<div>
<h1>{recipe.title}</h1>
<p>{recipe.description}</p>
</div>
);
};
export default RecipePage;
3. Interesting Implementation Details
Environment Variable Management
.env.example
file that serves as a template for required environment variables. This approach ensures that sensitive information is not hard-coded into the application.NPM Workspaces
npm workspaces
to manage multiple packages within a single repository. This allows for easier dependency management and streamlined development processes. For example, running npm run dev
starts all relevant local development servers simultaneously.Email Preview Server
Example of Email Template
<mjml>
<mj-body>
<mj-section>
<mj-column>
<mj-text>Hello, {{name}}!</mj-text>
<mj-button href="{{link}}">View Recipe</mj-button>
</mj-column>
</mj-section>
</mj-body>
</mjml>
4. Technical Challenges Overcome
Database Integration
End-to-End Testing
Deployment Considerations
Example of Deployment Script
#!/bin/bash
# Deploy script for Vercel
vercel --prod --confirm
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Environment Management: Using
.env
files for environment variables is crucial for managing configurations across different packages. It’s important to ensure that all developers are aware of the required environment variables and how to set them up. - NPM Workspaces: Leveraging
npm workspaces
for managing multiple components simultaneously simplifies dependency management and development. However, it’s essential to document this clearly, as it may not be familiar to all developers. - Testing Strategy: Implementing end-to-end tests using Playwright is beneficial for ensuring critical flows work as expected. However, running these tests in a production-equivalent environment can be challenging and requires careful setup.
2. What Worked Well
- Modular Architecture: The separation of concerns through distinct packages (frontend, admin backend, core services, etc.) promotes maintainability and scalability. Each package can evolve independently, which is advantageous for larger teams.
- Use of Modern Technologies: Utilizing Next.js, React.js, and Tailwind CSS for the frontend provides a robust and responsive user experience. The choice of Prisma for database interactions simplifies data management and migrations.
- Deployment Process: Deploying the frontend to Vercel is straightforward and allows for easy scaling. The admin backend’s deployment to platforms like Heroku or Digital Ocean is also effective for managing long-running processes.
3. What You’d Do Differently
- Documentation: While the README provides a good overview, more detailed documentation on setting up the development environment and troubleshooting common issues would be beneficial for new contributors.
- Testing Coverage: Expanding the testing strategy to include unit tests and integration tests for individual packages could help catch issues earlier in the development process.
- CI/CD Pipeline: Enhancing the CI/CD pipeline to include automated testing for all packages before deployment could improve code quality and reduce the risk of introducing bugs.
4. Advice for Others
- Invest in Documentation: Clear and comprehensive documentation is essential for onboarding new developers and ensuring that everyone understands the project structure and setup.
- Embrace Modular Design: Consider adopting a modular architecture from the start. It allows for better organization of code and makes it easier to manage dependencies and updates.
- Prioritize Testing: Implement a robust testing strategy early in the development process. This will save time and effort in the long run by catching bugs before they reach production.
- Stay Updated: Keep dependencies and technologies up to date to leverage improvements and security patches. Regularly review and refactor code to maintain quality and performance.
What’s Next?
Conclusion: Looking Ahead for Books About Food
Project Development Analytics
timeline gant
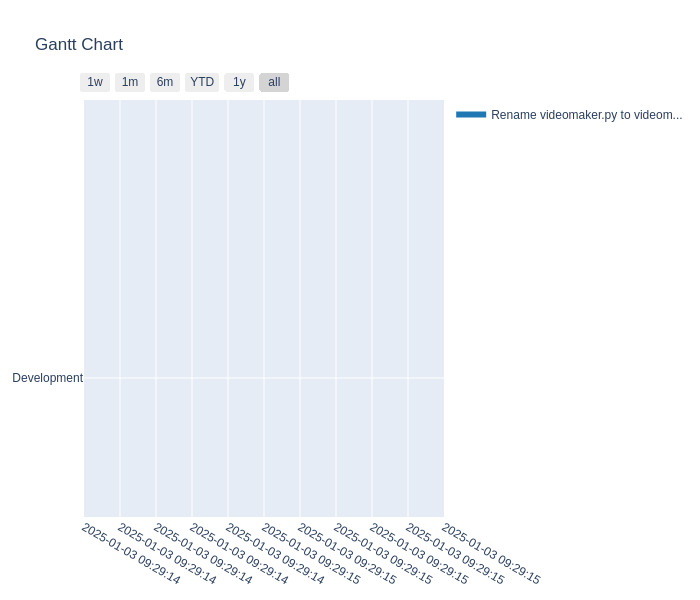
Commit Activity Heatmap
Contributor Network
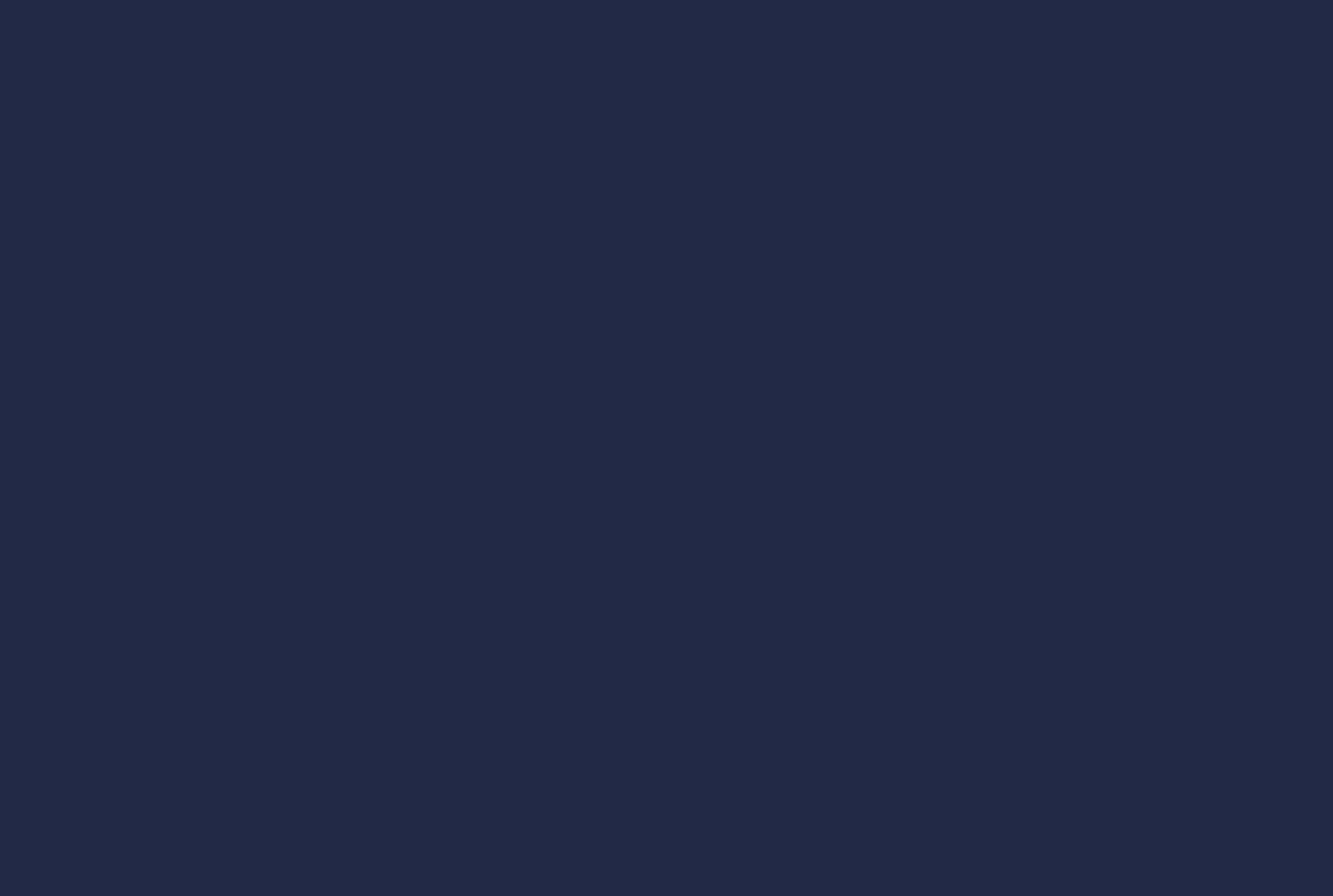
Commit Activity Patterns
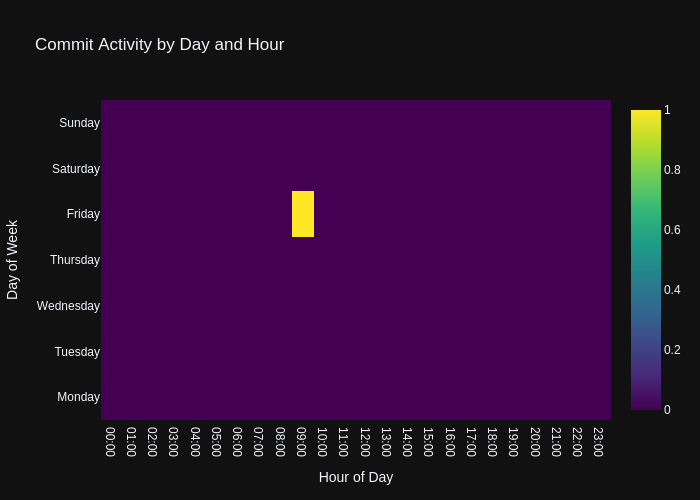
Code Frequency
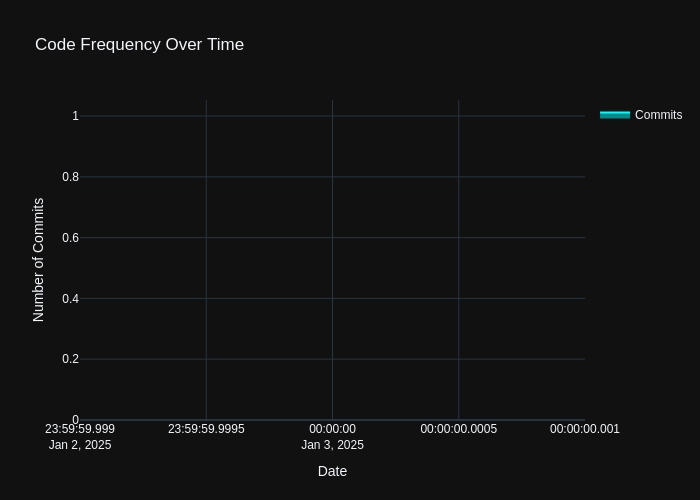
- Repository URL: https://github.com/wanghaisheng/a-modular-sectional-sofa-store
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日