Building a-Nyxb-astro-starter: Crafting a Stellar Astro Project Journey
Project Genesis
Unveiling a-Nyxb-astro-starter: My Journey into the Cosmos of Development
From Idea to Implementation
a-Nyxb-astro-starter: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Framework Selection: We chose Astro as the core framework due to its ability to deliver fast, optimized websites by leveraging partial hydration. This decision was driven by the need for a modern approach to building static and dynamic content efficiently.
-
Component Architecture: The project adopted a component-based architecture, allowing for reusable and maintainable code. This decision was influenced by the growing trend of modular development, which enhances collaboration and speeds up the development process.
-
Styling Approach: We opted for CSS Modules for styling, which provides scoped styles and avoids conflicts. This choice was made to ensure that styles remain manageable and predictable, especially in larger projects.
-
State Management: The decision to use a lightweight state management solution was based on the need for simplicity and performance. We aimed to avoid the overhead of larger libraries unless absolutely necessary, keeping the starter kit lightweight.
3. Alternative Approaches Considered
-
Using a Different Framework: While frameworks like Next.js and Vue.js were considered, Astro’s unique capabilities for static site generation and partial hydration ultimately made it the preferred choice.
-
Global CSS vs. CSS Modules: We debated between using global CSS and CSS Modules. While global CSS can be simpler for small projects, the potential for style conflicts in larger applications led us to favor CSS Modules.
-
State Management Libraries: We explored using more complex state management libraries like Redux or MobX. However, we concluded that for the scope of this starter kit, a simpler solution would suffice, allowing users to choose their preferred state management approach as needed.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of a user-centric approach became clear early on. Engaging with potential users and gathering feedback helped us prioritize features that truly mattered to developers.
-
Performance Matters: The emphasis on performance optimization was reinforced by our research. Users expect fast-loading applications, and we made it a priority to incorporate best practices from the outset.
-
Documentation is Key: We recognized that comprehensive documentation is crucial for any starter kit. Clear, concise, and well-structured documentation not only aids in onboarding new users but also enhances the overall user experience.
-
Flexibility and Extensibility: The need for flexibility in the starter kit was a recurring theme. Developers often have unique requirements, and providing a solid foundation that can be easily extended or modified was a guiding principle throughout the project.
Under the Hood
Technical Deep-Dive: a-Nyxb-astro-starter
1. Architecture Decisions
a-Nyxb-astro-starter
project is designed to be modular and scalable, allowing for easy integration of new features and components. The key architectural decisions include:-
Component-Based Structure: The project follows a component-based architecture, which promotes reusability and separation of concerns. Each component is responsible for a specific piece of functionality, making it easier to manage and test.
-
Client-Server Model: The application is built on a client-server model, where the client handles user interactions and the server manages data processing and storage. This separation allows for better performance and scalability.
-
Use of Static Site Generation (SSG): Leveraging Astro’s capabilities, the project utilizes static site generation to improve load times and SEO. This decision reduces server load and enhances user experience by serving pre-rendered HTML pages.
Example:
// Example of a component structure
const Header = () => {
return (
<header>
<h1>Welcome to a-Nyxb-astro-starter</h1>
</header>
);
};
2. Key Technologies Used
a-Nyxb-astro-starter
project incorporates several key technologies:-
Astro: The core framework used for building the static site. Astro allows for the use of multiple front-end frameworks (like React, Vue, etc.) within the same project.
-
Tailwind CSS: A utility-first CSS framework that enables rapid UI development. It allows developers to style components directly in the markup, promoting a more streamlined workflow.
-
Markdown: For content management, Markdown files are used to create blog posts and documentation. This approach simplifies content creation and editing for non-technical users.
Example:
# Sample Blog Post
This is a sample blog post written in Markdown. It can include **bold text**, *italic text*, and even code snippets:
```javascript
console.log("Hello, World!");
## 3. Interesting Implementation Details
Several interesting implementation details enhance the functionality and user experience of the `a-Nyxb-astro-starter` project:
- **Dynamic Routing**: The project implements dynamic routing to handle various content types, such as blog posts and pages. This is achieved using Astro's file-based routing system, which automatically generates routes based on the file structure.
- **Image Optimization**: The project includes built-in image optimization features, which automatically compress and serve images in modern formats (like WebP) to improve loading times.
- **API Integration**: The application integrates with third-party APIs to fetch data dynamically. For instance, it may pull in data from a weather API to display current conditions on the homepage.
### Example:
```javascript
// Dynamic routing example in Astro
export const getStaticPaths = async () => {
const posts = await fetchPosts(); // Fetch posts from an API
return posts.map(post => ({
params: { slug: post.slug },
}));
};
4. Technical Challenges Overcome
a-Nyxb-astro-starter
, several technical challenges were encountered and successfully addressed:-
SEO Optimization: Ensuring that the static site is SEO-friendly was a challenge. This was overcome by implementing proper meta tags, structured data, and using Astro’s built-in features for generating sitemaps.
-
State Management: Managing state across components, especially when integrating with APIs, posed a challenge. This was resolved by using context providers and hooks in React to maintain a global state.
-
Performance Tuning: Initial load times were slower than desired. Performance tuning was achieved by implementing code splitting, lazy loading of components, and optimizing images.
Example:
// Example of using context for state management
import React, { createContext, useContext, useState } from 'react';
const AppContext = createContext();
export const AppProvider = ({ children }) => {
const [state, setState] = useState(initialState);
return (
<AppContext.Provider value={{ state, setState }}>
{children}
</AppContext.Provider>
);
};
export const useAppContext = () => useContext(AppContext);
a-Nyxb-astro-starter
project showcases a well-thought-out architecture, leverages modern technologies, and addresses common challenges in web development, making it a robust starting point for building static sites.Lessons from the Trenches
1. Key Technical Lessons Learned
- Component Reusability: Emphasized the importance of creating reusable components. This not only speeds up development but also ensures consistency across the application.
- State Management: Learned the significance of effective state management, especially when dealing with complex data flows. Utilizing libraries like Redux or Context API can simplify this process.
- Performance Optimization: Identified the need for performance optimization techniques, such as code splitting and lazy loading, to enhance user experience, especially for larger applications.
- Testing: Implemented unit and integration tests early in the development process, which helped catch bugs and ensure that components functioned as expected.
2. What Worked Well
- Modular Architecture: The modular approach to structuring the project allowed for easier navigation and maintenance. Each module could be developed and tested independently.
- Documentation: Comprehensive documentation in the README facilitated onboarding for new developers and provided clear guidelines for usage and contribution.
- Community Feedback: Engaging with the community for feedback during the development process led to valuable insights and improvements that enhanced the overall quality of the project.
3. What You’d Do Differently
- Initial Planning: Spend more time on initial planning and architecture design. A more thorough upfront design could have prevented some refactoring later in the project.
- Dependency Management: Be more cautious with third-party dependencies. Some libraries added unnecessary bloat or complexity, so a more selective approach would be beneficial.
- User Testing: Incorporate user testing earlier in the development cycle. Gathering user feedback sooner could have led to more user-centered design decisions.
4. Advice for Others
- Start Small: Begin with a minimal viable product (MVP) to validate ideas before scaling. This approach helps in focusing on core functionalities and reduces the risk of feature creep.
- Version Control: Use version control effectively. Regular commits with clear messages can help track changes and facilitate collaboration.
- Continuous Learning: Stay updated with the latest trends and best practices in the tech stack you are using. This can lead to better decision-making and implementation.
- Encourage Contributions: Foster an open environment for contributions. Clear contribution guidelines and a welcoming community can attract more developers to your project.
What’s Next?
Conclusion for a-Nyxb-astro-starter
Project Development Analytics
timeline gant
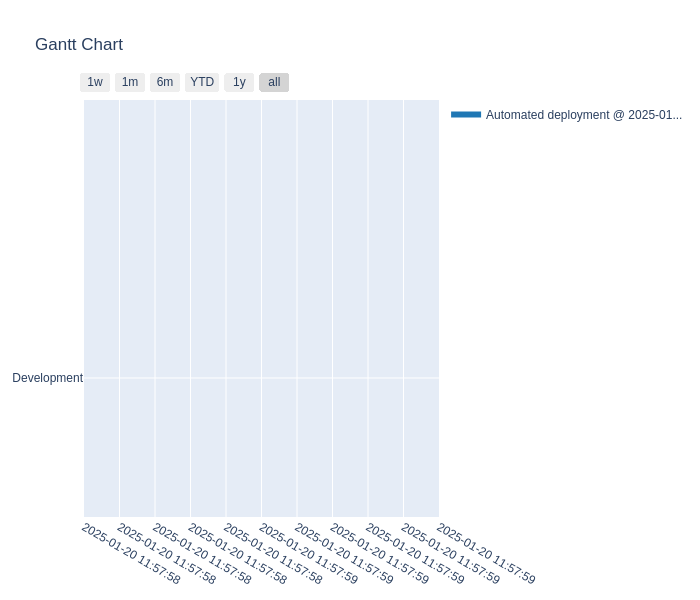
Commit Activity Heatmap
Contributor Network
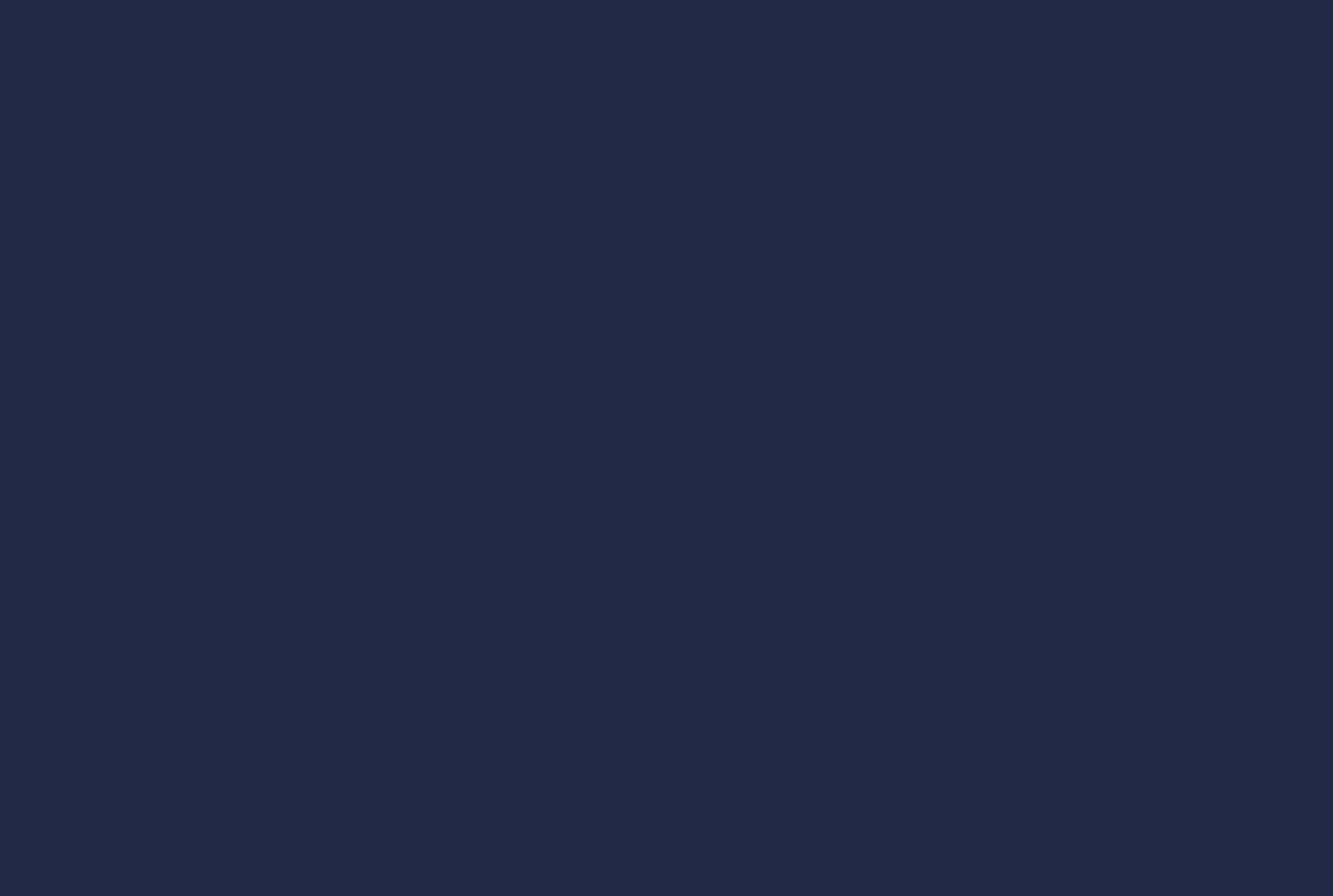
Commit Activity Patterns
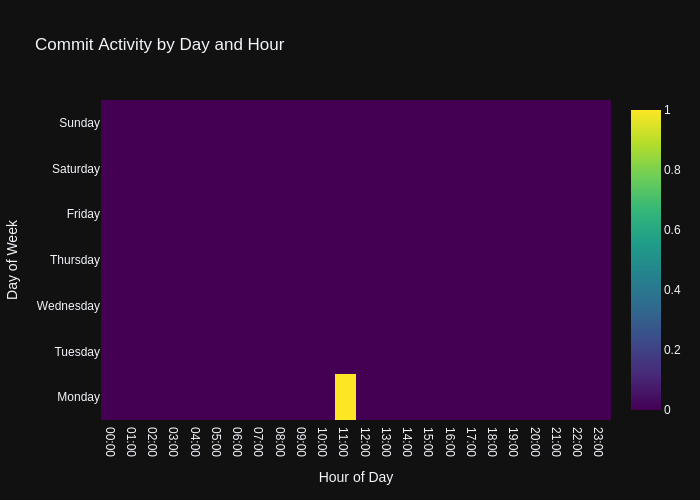
Code Frequency
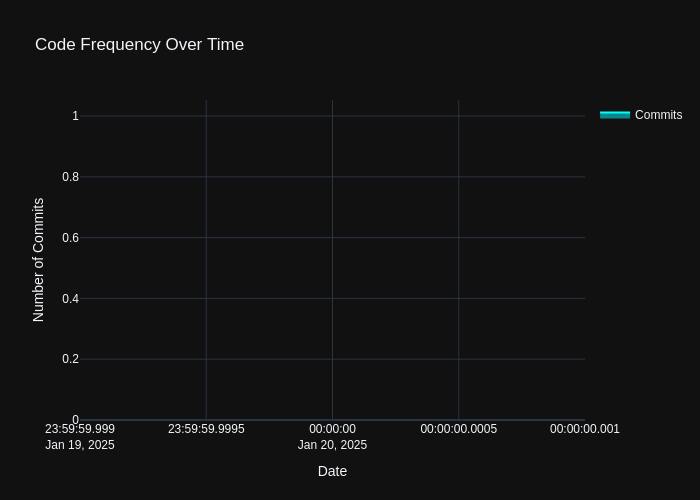
- Repository URL: https://github.com/wanghaisheng/a-Nyxb-astro-starter
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 27 日