Building a-Bube-Boutique-5: Crafting an E-Commerce Experience with Next.js
Project Genesis
Welcome to a-Bube-Boutique-5: My Journey into Next.js Development
From Idea to Implementation
Journey from Concept to Code: A Next.js Project
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Performance Optimization: Next.js offers automatic code splitting and server-side rendering, which significantly improves load times and overall performance. This was crucial for our target audience, who expect fast and responsive applications.
-
File-Based Routing: The built-in routing system simplifies navigation and allows for easy organization of components and pages. This feature streamlined our development process, enabling us to focus on building features rather than managing routing logic.
-
API Routes: Next.js allows us to create API endpoints within the same project, facilitating seamless integration between the frontend and backend. This decision reduced the complexity of managing separate server and client applications.
-
Community and Ecosystem: The strong community support and extensive documentation available for Next.js provided confidence in our choice. We knew we could rely on community resources and plugins to enhance our application.
3. Alternative Approaches Considered
-
Create React App: Initially, we explored Create React App for its simplicity and ease of use. However, we quickly realized that it lacked the server-side rendering capabilities that were essential for our project.
-
Gatsby: Gatsby was another contender due to its static site generation features. However, we found that its build times could be lengthy for larger applications, and the need for dynamic content made Next.js a more suitable option.
-
Nuxt.js: For a brief moment, we considered Nuxt.js, which is similar to Next.js but tailored for Vue.js. However, our team’s expertise in React made Next.js a more logical choice.
4. Key Insights That Shaped the Project
-
User-Centric Design: Early user feedback highlighted the importance of a clean and intuitive interface. This insight led us to prioritize user experience in our design decisions, ensuring that the application was not only functional but also visually appealing.
-
Iterative Development: Embracing an iterative development approach allowed us to continuously refine features based on user feedback. This flexibility was crucial in adapting to changing requirements and ensuring that the final product met user needs.
-
Performance Matters: As we implemented features, we learned that performance optimization should be a continuous focus. Utilizing Next.js’s built-in features, such as image optimization and font loading, became essential in delivering a fast and responsive application.
-
Collaboration and Communication: Regular team meetings and open communication channels fostered collaboration and ensured that everyone was aligned on project goals. This insight reinforced the importance of teamwork in navigating challenges and achieving milestones.
Conclusion
Under the Hood
Technical Deep-Dive: Next.js Project
1. Architecture Decisions
Key Architectural Choices:
-
File-Based Routing: Next.js uses a file-based routing system, where the file structure in the
app
directory directly corresponds to the routes of the application. For example, a file namedapp/about.tsx
would automatically create a route at/about
. -
API Routes: Next.js allows the creation of API endpoints within the same project. This is useful for building full-stack applications without needing a separate backend server. API routes can be defined in the
pages/api
directory. -
Static and Dynamic Rendering: Developers can choose between static generation (using
getStaticProps
) and server-side rendering (usinggetServerSideProps
) for each page, allowing for optimized performance and SEO.
2. Key Technologies Used
-
Next.js: The core framework that provides the structure and features for building React applications.
-
React: The underlying library for building user interfaces, enabling the use of components and hooks.
-
TypeScript: The project uses TypeScript (
.tsx
files) for type safety, enhancing code quality and maintainability. -
Vercel: The deployment platform specifically optimized for Next.js applications, providing features like automatic scaling and serverless functions.
-
next/font: This feature is used for font optimization, allowing for the automatic loading of custom fonts like Inter, which improves performance and user experience.
3. Interesting Implementation Details
Auto-Updating Pages
app/page.tsx
file will automatically reflect in the browser without needing a manual refresh. This is achieved through Webpack’s development server, which watches for file changes.Font Optimization
next/font
simplifies the process of loading custom fonts. For example, to load the Inter font, you can add the following code in your component:import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
export default function Home() {
return (
<main className={inter.className}>
<h1>Hello, Next.js!</h1>
</main>
);
}
4. Technical Challenges Overcome
Managing State Across Pages
import { createContext, useContext, useState } from 'react';
const AuthContext = createContext(null);
export const AuthProvider = ({ children }) => {
const [user, setUser] = useState(null);
return (
<AuthContext.Provider value={{ user, setUser }}>
{children}
</AuthContext.Provider>
);
};
export const useAuth = () => useContext(AuthContext);
Optimizing Performance
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('./components/HeavyComponent'));
export default function Home() {
return (
<div>
<h1>Welcome to My Next.js App</h1>
<DynamicComponent />
</div>
);
}
dynamic
, the HeavyComponent
will only be loaded when it is needed, reducing the initial load time of the application.Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
- File-Based Routing: Next.js uses a file-based routing system, which simplifies the creation of routes. Understanding how to structure the
app
directory effectively is crucial for maintaining a clean and organized codebase. - Automatic Code Splitting: Next.js automatically splits code for each page, which improves performance. Learning how to leverage this feature by creating separate components for different pages helped in optimizing load times.
- API Routes: Utilizing API routes in Next.js allows for building backend functionality directly within the application. This was particularly useful for handling form submissions and fetching data without needing a separate backend service.
- Static Site Generation (SSG) and Server-Side Rendering (SSR): Understanding when to use SSG vs. SSR was key. SSG is great for performance and SEO, while SSR is beneficial for dynamic content. This knowledge helped in making informed decisions about data fetching strategies.
What Worked Well
- Development Experience: The hot-reloading feature in Next.js made the development process smooth and efficient. Changes reflected instantly in the browser, which enhanced productivity.
- Integration with Vercel: Deploying the application on Vercel was seamless. The platform’s integration with GitHub allowed for automatic deployments on push, which streamlined the workflow.
- Font Optimization: Using
next/font
for loading custom fonts like Inter improved the performance and appearance of the application without additional configuration. - Documentation and Community: The extensive documentation and active community around Next.js provided valuable resources and support, making it easier to troubleshoot issues and learn best practices.
What You’d Do Differently
- State Management: Initially, the project relied on local component state for managing data. In hindsight, implementing a state management solution (like Redux or Context API) from the start would have made managing global state more efficient, especially as the application grew.
- Testing: While the project was functional, incorporating testing (unit and integration tests) earlier in the development process would have helped catch bugs sooner and ensured code reliability.
- Performance Monitoring: Setting up performance monitoring tools (like Google Lighthouse or Sentry) from the beginning would have provided insights into performance bottlenecks and error tracking, allowing for proactive optimizations.
Advice for Others
- Start Small: If you’re new to Next.js, start with a small project to familiarize yourself with its features and conventions. Gradually incorporate more complex functionalities as you gain confidence.
- Leverage the Community: Don’t hesitate to seek help from the Next.js community. Forums, GitHub issues, and Discord channels can be invaluable resources for troubleshooting and learning.
- Focus on SEO: Next.js is great for SEO out of the box. Make sure to utilize features like dynamic routing and metadata management to enhance your application’s visibility.
- Stay Updated: Next.js is actively developed, with frequent updates and new features. Keep an eye on the official documentation and changelog to take advantage of the latest improvements and best practices.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
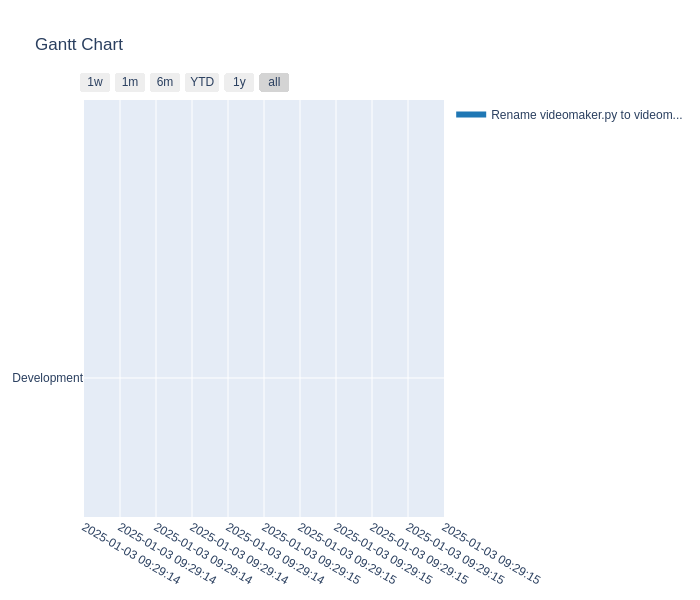
Commit Activity Heatmap
Contributor Network
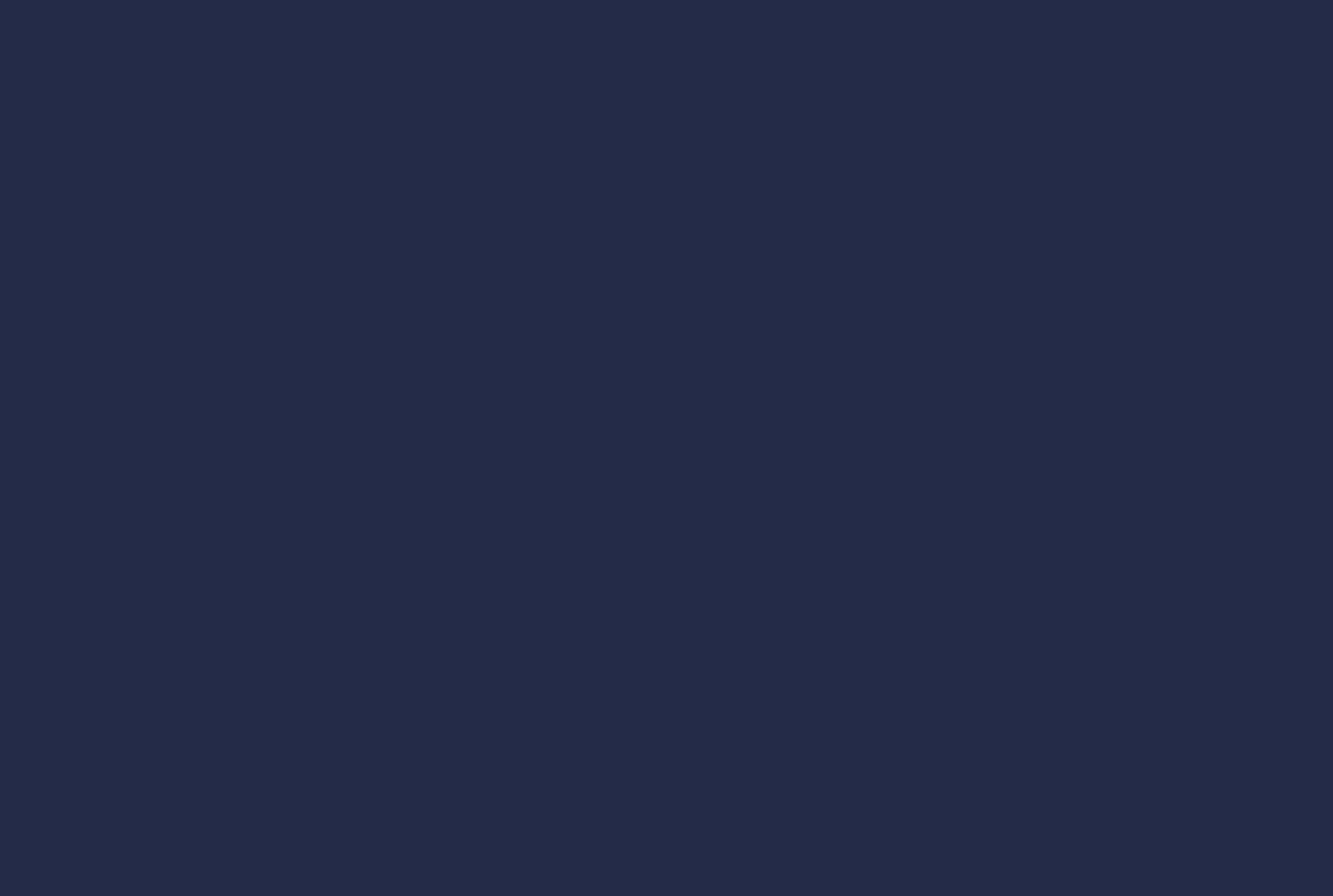
Commit Activity Patterns
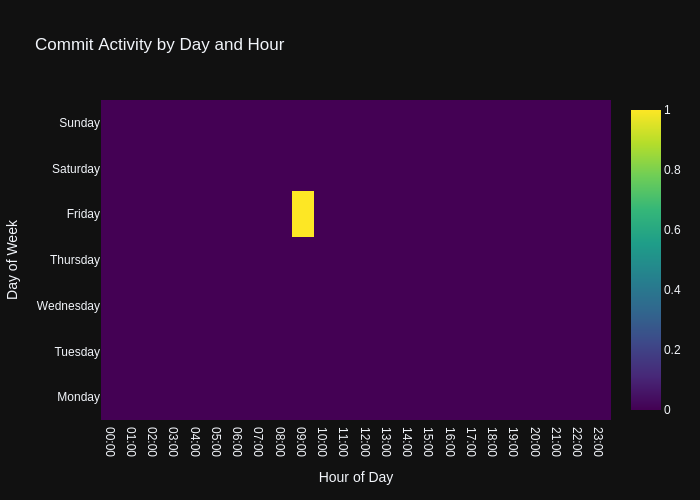
Code Frequency
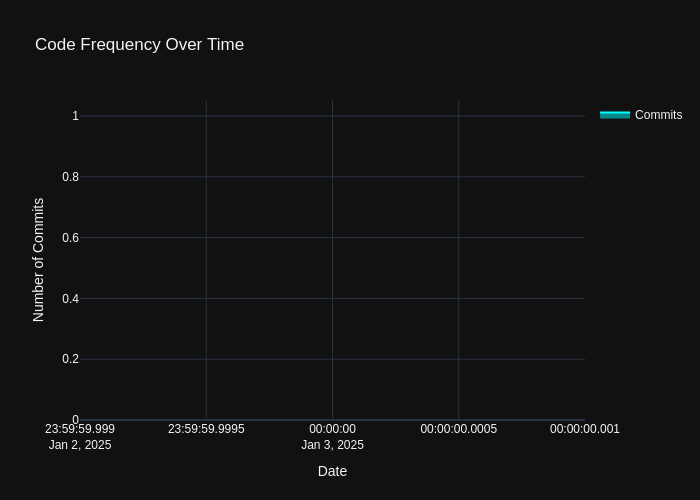
- Repository URL: https://github.com/wanghaisheng/a-Bube-Boutique-5
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 6 日