Building a WebDAV Server with Cloudflare Workers and R2: My Developer Journey
Project Genesis
Unlocking the Power of Cloudflare R2: My Journey with CFr2-webdav
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Use of Cloudflare Workers: We chose Cloudflare Workers as the deployment platform due to its serverless architecture, which eliminates the need for server management. This decision allowed us to focus on the application logic rather than infrastructure concerns, enabling rapid development and deployment.
-
Integration with R2 Storage: Leveraging Cloudflare R2 as the storage backend was a natural choice, given its compatibility with the WebDAV protocol and the cost-effectiveness of its free tier. This integration ensured that users could store and retrieve files without incurring significant costs.
-
WebDAV Protocol Compliance: Ensuring full compliance with the WebDAV protocol was crucial for user experience. This decision meant that users could utilize existing WebDAV clients without any compatibility issues, making the server accessible to a broader audience.
-
Basic Authentication: Implementing basic authentication was a straightforward way to secure the server while keeping the setup simple for users. This decision balanced security needs with ease of use, allowing users to quickly get started without complex configuration.
3. Alternative Approaches Considered
-
Self-Hosted Solutions: One option was to create a self-hosted WebDAV server using traditional server setups (e.g., using Node.js or PHP). However, this approach would require users to manage their own servers, which could deter less technical users.
-
Using Other Cloud Providers: We explored the possibility of integrating with other cloud storage providers, but many of them lacked the same level of free tier support and ease of integration as Cloudflare R2. This led us to focus on Cloudflare as the primary solution.
-
Advanced Authentication Mechanisms: While we initially considered implementing OAuth or other advanced authentication methods, we ultimately decided that basic authentication would suffice for the initial version. This choice allowed us to prioritize rapid deployment and user accessibility.
4. Key Insights That Shaped the Project
-
User-Centric Design: Understanding the target audience was vital. Many potential users were looking for a simple, hassle-free way to manage their files in the cloud. This insight drove the decision to prioritize ease of use and straightforward deployment.
-
Importance of Documentation: Early feedback highlighted the need for comprehensive documentation. We recognized that clear instructions would be essential for users to successfully deploy and utilize the WebDAV server. This led to the creation of detailed README files and deployment guides.
-
Iterative Development: Embracing an iterative development approach allowed us to refine features based on user feedback. By deploying early versions and gathering input, we could make informed decisions about which features to prioritize and how to enhance the user experience.
Under the Hood
Technical Deep-Dive: Cloudflare R2 WebDAV Server
1. Architecture Decisions
-
Serverless Model: By utilizing Cloudflare Workers, the application avoids the overhead of managing traditional server infrastructure. This allows for automatic scaling and reduced operational costs.
-
Decoupled Storage: The use of Cloudflare R2 as a storage backend decouples the file storage from the application logic. This separation allows for easier management of files and directories while taking advantage of R2’s generous free tier.
-
WebDAV Protocol Compliance: The server is built to be fully compliant with the WebDAV protocol, enabling standard file operations (upload, download, delete, etc.) over HTTP. This decision ensures compatibility with a wide range of clients and tools that support WebDAV.
2. Key Technologies Used
-
Cloudflare Workers: A serverless platform that allows developers to run JavaScript code at the edge, close to users, providing low-latency responses.
-
Cloudflare R2: An object storage service that is compatible with S3 APIs, allowing for easy integration and management of files.
-
GitHub Actions: Used for CI/CD, enabling automated deployment of the application whenever changes are pushed to the repository.
-
Node.js: The underlying runtime for the application, allowing for asynchronous operations and efficient handling of I/O tasks.
Example of Cloudflare Worker Script
addEventListener('fetch', event => {
event.respondWith(handleRequest(event.request));
});
async function handleRequest(request) {
const url = new URL(request.url);
if (request.method === 'PUT') {
const bucketName = 'your-bucket-name';
const fileName = url.pathname.slice(1); // Extract file name from URL
const fileData = await request.arrayBuffer(); // Get file data from request
// Upload to R2
await R2_BUCKET.put(fileName, fileData);
return new Response('File uploaded successfully', { status: 201 });
}
return new Response('Method Not Allowed', { status: 405 });
}
3. Interesting Implementation Details
-
Authentication: The server supports basic authentication, which is crucial for securing access to the WebDAV endpoints. The implementation checks for the presence of
Authorization
headers and validates the credentials against stored values. -
GitHub Secrets Management: The deployment process utilizes GitHub Secrets to securely manage sensitive information such as API tokens and passwords. This ensures that sensitive data is not exposed in the codebase.
Example of GitHub Secrets Configuration
CLOUDFLARE_API_TOKEN: <your_api_token>
USERNAME: <your_username>
PASSWORD: <your_password>
BUCKET_NAME: <your_bucket_name>
4. Technical Challenges Overcome
-
Handling CORS: One of the challenges faced was managing Cross-Origin Resource Sharing (CORS) for the WebDAV server. Proper CORS headers were implemented to allow web clients to interact with the server without running into security restrictions.
-
Error Handling: Implementing robust error handling was crucial for providing meaningful feedback to users. The server captures various error scenarios (e.g., file not found, unauthorized access) and returns appropriate HTTP status codes and messages.
Example of Error Handling
async function handleRequest(request) {
try {
// Handle request logic...
} catch (error) {
return new Response('Internal Server Error', { status: 500 });
}
}
- Local Development: Setting up a local development environment that closely mimics the Cloudflare Workers environment was challenging. The use of the Wrangler CLI tool allows developers to test their code locally, but certain features (like R2 interactions) may not be fully replicable.
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
- Understanding WebDAV Protocol: Implementing a WebDAV server required a deep understanding of the WebDAV protocol, including its methods (like GET, PUT, DELETE) and how to handle requests and responses properly.
- Cloudflare Workers Limitations: While Cloudflare Workers provide a serverless environment, there are limitations in terms of execution time and resource usage. Understanding these constraints is crucial for optimizing performance.
- R2 Storage Integration: Integrating with Cloudflare R2 storage taught the importance of handling asynchronous operations and managing API calls efficiently to avoid bottlenecks.
What Worked Well
- Ease of Deployment: The one-click deployment feature to Cloudflare Workers made it easy for users to set up the WebDAV server without extensive configuration, which is a significant advantage for non-technical users.
- Documentation: The README provided clear and concise instructions for both one-click and manual deployment, which helped users navigate the setup process smoothly.
- Basic Authentication: Implementing basic authentication provided a simple yet effective way to secure access to the WebDAV server, ensuring that only authorized users could manage files.
What You’d Do Differently
- Enhanced Error Handling: While the current implementation covers basic operations, adding more robust error handling and logging would improve the user experience by providing clearer feedback on issues.
- Testing and Local Development: The local development setup could be improved by providing more comprehensive testing tools or mock environments that better simulate the Cloudflare Workers environment.
- User Interface: If applicable, developing a simple web interface for file management could enhance usability, allowing users to interact with their files without needing a separate WebDAV client.
Advice for Others
- Thoroughly Document Your Code: Clear documentation within the codebase can help future contributors understand the logic and structure, making it easier to maintain and extend the project.
- Engage with the Community: Encourage users to provide feedback and contribute to the project. This can lead to valuable insights and improvements that may not have been considered initially.
- Stay Updated on Dependencies: Regularly check for updates to dependencies and the Cloudflare platform to ensure that the project remains secure and takes advantage of new features or improvements.
- Consider Scalability: As usage grows, think about how to scale the application effectively. This might involve optimizing code, managing resources, or even considering alternative architectures if necessary.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
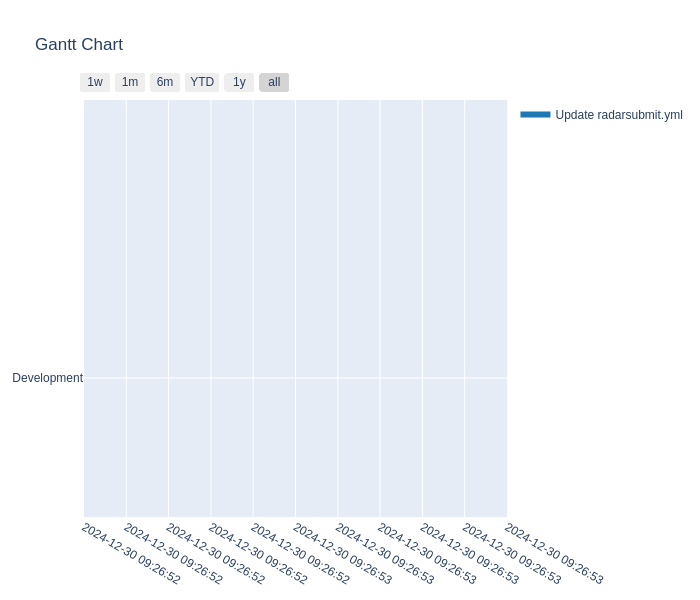
Commit Activity Heatmap
Contributor Network
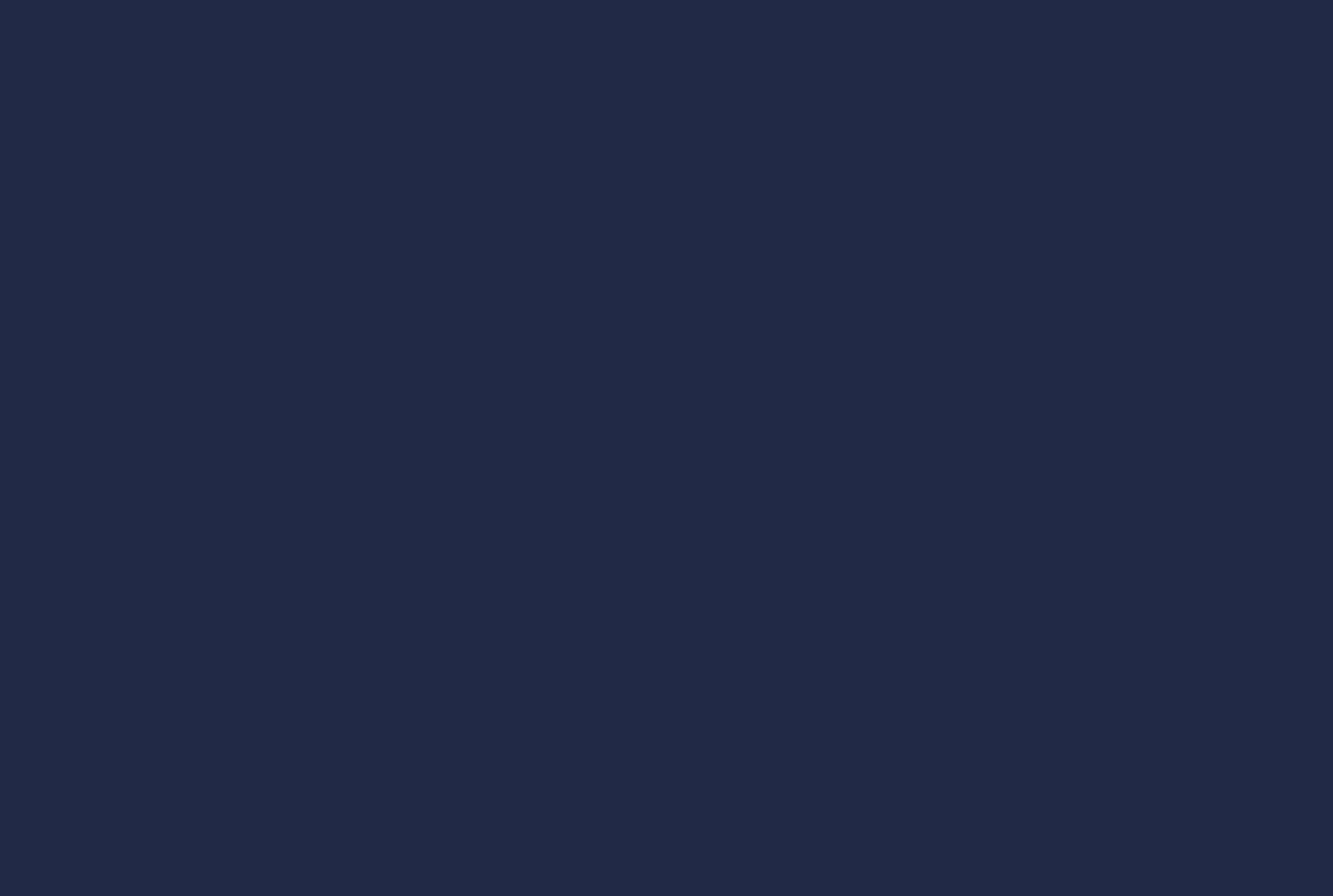
Commit Activity Patterns
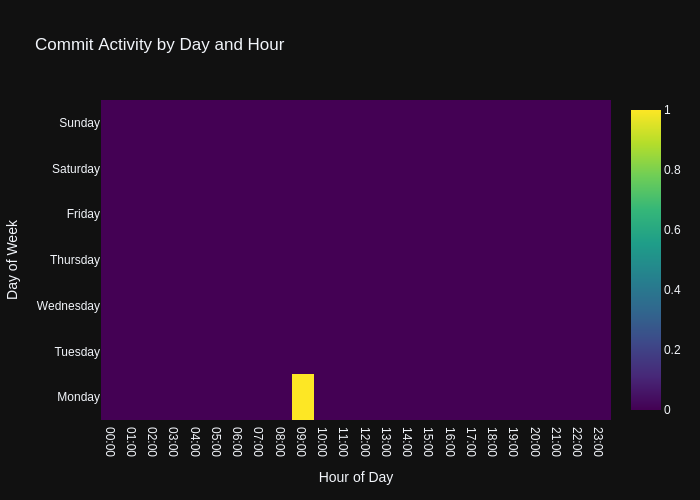
Code Frequency
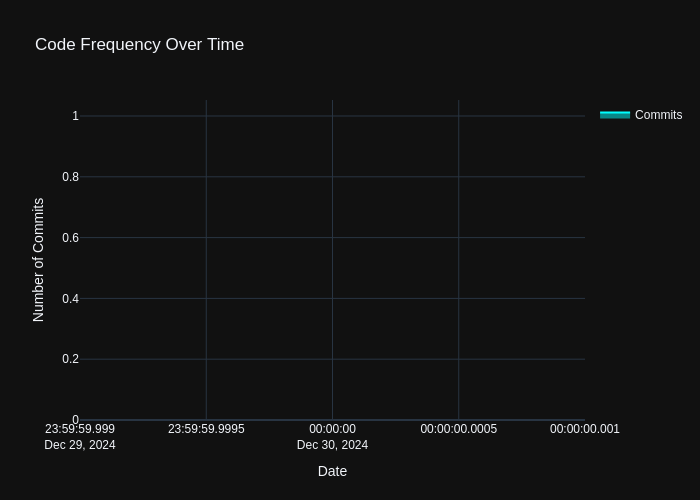
- Repository URL: https://github.com/wanghaisheng/CFr2-webdav
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日