Building AI-Flashcard-Deepseek: A Next.js Learning Revolution
Heisenberg
2025 年 2 月 3 日
基于Next.js和Deepseek API开发的智能闪卡学习工具
Built by wanghaisheng | Last updated: 20250203
10 minutes 57 seconds read
Project Genesis
Unlocking the Power of Learning: My Journey with AI-Flashcard-Deepseek
As a lifelong learner, I’ve always been fascinated by the art of mastering new concepts and skills. However, I often found myself overwhelmed by the sheer volume of information I wanted to absorb. That’s when the spark of inspiration hit me: what if I could harness the power of artificial intelligence to create a smarter, more efficient way to study? This idea blossomed into the creation of AI-Flashcard-Deepseek, a tool designed to revolutionize the way we learn through intelligent flashcards.
My personal motivation for this project stemmed from my own struggles with traditional study methods. I wanted a solution that not only helped me create flashcards but also made the process engaging and tailored to my learning style. I envisioned a platform where users could easily generate high-quality study materials with just a few clicks, allowing them to focus on what truly matters—understanding and retaining knowledge.
Of course, the journey wasn’t without its challenges. As I dove into the development process using Next.js and the Deepseek API, I encountered hurdles ranging from technical glitches to the intricacies of AI integration. There were moments of frustration, but each obstacle only fueled my determination to create a seamless learning experience. I realized that the key to overcoming these challenges lay in my passion for education and technology, driving me to push through and find innovative solutions.
The result? AI-Flashcard-Deepseek—a user-friendly tool that empowers learners to create, manage, and review flashcards effortlessly. With features like AI-assisted card generation and topic exploration, this platform transforms the way we approach studying. Whether you’re a student preparing for exams or a professional looking to expand your knowledge, AI-Flashcard-Deepseek is here to support your learning journey.
Join me as we explore the exciting world of AI-enhanced learning and discover how this tool can help you unlock your full potential!
From Idea to Implementation
1. Initial Research and Planning
The journey of developing the AI闪卡学习助手 (AI Flashcard Learning Assistant) began with a thorough exploration of the educational technology landscape, particularly focusing on the use of AI in learning tools. The primary goal was to create an intelligent flashcard system that could enhance the learning experience by leveraging AI capabilities.
During the initial research phase, we identified several key features that users typically seek in flashcard applications, such as ease of card creation, efficient management, and effective review mechanisms. We also examined existing solutions to understand their strengths and weaknesses. This analysis highlighted a gap in the market for a tool that not only allows users to create flashcards but also utilizes AI to generate content and suggest related topics, thereby enriching the learning process.
2. Technical Decisions and Their Rationale
With a clear vision in mind, we moved on to the technical planning phase. The decision to use Next.js as the framework was driven by its server-side rendering capabilities, which enhance performance and SEO, making it suitable for a web-based application. Additionally, Next.js provides a robust structure for building React applications, which was essential for creating a dynamic user interface.
For styling, we chose Tailwind CSS due to its utility-first approach, allowing for rapid design iterations and a responsive layout. This choice facilitated a clean and modern aesthetic while maintaining flexibility in design.
The use of SQLite for local data storage was a strategic decision aimed at providing a lightweight and efficient database solution. SQLite’s simplicity and ease of integration with Next.js made it an ideal choice for managing user-generated flashcards without the overhead of a more complex database system.
Finally, integrating the Deepseek API was pivotal for the AI functionalities. This decision was based on Deepseek’s reputation for providing high-quality AI services, which would enable the application to generate flashcards and explore related topics effectively.
3. Alternative Approaches Considered
During the planning phase, we considered several alternative approaches. One option was to build the application using a traditional REST API architecture instead of leveraging Next.js’s capabilities. However, we ultimately decided against this due to the benefits of server-side rendering and static site generation that Next.js offers, which would enhance user experience.
We also explored using other CSS frameworks, such as Bootstrap or Material-UI. While these frameworks are popular, we found that Tailwind CSS provided greater flexibility and customization options, which aligned better with our design goals.
In terms of database solutions, we briefly considered using a more complex relational database like PostgreSQL. However, given the scope of the project and the need for rapid development, SQLite emerged as the more practical choice.
4. Key Insights That Shaped the Project
Several key insights emerged throughout the development process that significantly shaped the project. First, the importance of user feedback became evident early on. Engaging potential users during the design phase helped us refine our features and prioritize functionalities that truly addressed their needs.
Another insight was the realization of the power of AI in education. By integrating AI capabilities, we could not only automate the flashcard creation process but also provide users with personalized learning experiences. This understanding drove the decision to focus heavily on the AI aspects of the application.
Lastly, the iterative nature of development was crucial. We adopted an agile approach, allowing us to continuously test and refine features based on user interactions and feedback. This adaptability ensured that the final product was not only functional but also aligned with user expectations.
Conclusion
The journey from concept to code for the AI闪卡学习助手 was marked by careful research, strategic technical decisions, and a commitment to user-centered design. By leveraging modern technologies and AI capabilities, we aimed to create a powerful learning tool that enhances the educational experience, making studying more efficient and engaging for users.
Under the Hood
Technical Deep-Dive: AI闪卡学习助手
1. Architecture Decisions
The architecture of the AI闪卡学习助手 (AI Flashcard Learning Assistant) is designed to leverage modern web technologies to create an efficient and user-friendly learning tool. The application is built using a client-server model, where the client-side is responsible for user interactions and the server-side handles API requests and data management.
Key Architectural Choices:
- Next.js Framework: The choice of Next.js allows for server-side rendering (SSR) and static site generation (SSG), which enhances performance and SEO. This is particularly beneficial for educational tools where content discoverability is important.
- Deepseek API Integration: By utilizing the Deepseek API, the application can harness AI capabilities for generating flashcards based on user input. This decision significantly reduces the complexity of building AI models from scratch.
- Local Data Storage with SQLite: SQLite is chosen for its lightweight nature and ease of integration. It allows for quick data retrieval and storage without the overhead of a full-fledged database server.
2. Key Technologies Used
The application employs several key technologies that contribute to its functionality and user experience:
- Next.js 14: A React framework that enables server-side rendering and static site generation, improving performance and SEO.
- Tailwind CSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
- SQLite: A self-contained, serverless database engine that provides local data storage capabilities.
- Deepseek API: An external API that provides AI services for generating flashcards based on user-defined topics.
Example of Next.js Usage:
The application structure follows the Next.js conventions, with pages organized in the
pages
directory. For instance, the main page might look like this:// pages/index.js
import Head from 'next/head';
import FlashcardManager from '../components/FlashcardManager';
export default function Home() {
return (
<div>
<Head>
<title>AI闪卡学习助手</title>
</Head>
<FlashcardManager />
</div>
);
}
3. Interesting Implementation Details
AI-Assisted Flashcard Generation
The application allows users to input a topic or custom content, which is then sent to the Deepseek API for flashcard generation. This interaction is handled asynchronously to ensure a smooth user experience.
// utils/api.js
export const generateFlashcards = async (topic) => {
const response = await fetch('https://api.deepseek.com/generate', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.DEEPSKEE_API_KEY}`,
},
body: JSON.stringify({ topic }),
});
return response.json();
};
Card Management
Users can manage their flashcards through a simple interface that allows them to import, view, and navigate through cards using keyboard shortcuts. This enhances usability, especially for users who prefer keyboard navigation.
// components/FlashcardManager.js
const FlashcardManager = () => {
const [cards, setCards] = useState([]);
const handleKeyDown = (event) => {
if (event.key === 'ArrowRight') {
// Logic to show next card
} else if (event.key === 'ArrowLeft') {
// Logic to show previous card
} else if (event.key === ' ') {
// Logic to toggle card visibility
}
};
useEffect(() => {
window.addEventListener('keydown', handleKeyDown);
return () => {
window.removeEventListener('keydown', handleKeyDown);
};
}, [cards]);
return (
<div>
{/* Render flashcards */}
</div>
);
};
4. Technical Challenges Overcome
API Integration
One of the significant challenges was integrating the Deepseek API effectively. Handling API responses and ensuring that the application remains responsive while waiting for data was crucial. This was addressed by implementing asynchronous functions and managing loading states.
State Management
Managing the state of flashcards, especially when dealing with user inputs and API responses, required careful consideration. The use of React’s
useState
and useEffect
hooks allowed for efficient state management and reactivity.User Experience
Ensuring a seamless user experience while navigating through flashcards was another challenge. Implementing keyboard shortcuts and responsive design principles helped create an intuitive interface that caters to various user preferences.
Example of State Management:
const [loading, setLoading] = useState(false);
const fetchFlashcards = async (topic) => {
setLoading(true);
const data = await generateFlashcards(topic);
setCards(data.cards);
setLoading(false);
};
Conclusion
The AI闪卡学习助手 is a well-architect
Lessons from the Trenches
Here’s a breakdown based on the project history and README for the AI闪卡学习助手 (AI Flashcard Learning Assistant):
1. Key Technical Lessons Learned
- Integration of APIs: Successfully integrating the Deepseek API demonstrated the importance of understanding API documentation and handling asynchronous requests effectively. This experience highlighted the need for robust error handling and user feedback mechanisms when dealing with external services.
- State Management: Managing the state of flashcards and user interactions in a React application using Next.js required a solid understanding of React hooks and context. This reinforced the importance of keeping the UI responsive and ensuring that state changes are efficiently managed.
- Responsive Design: Utilizing Tailwind CSS for styling emphasized the benefits of utility-first CSS frameworks in creating responsive and visually appealing interfaces quickly. It also taught the importance of mobile-first design principles.
2. What Worked Well
- User Experience: The AI-assisted flashcard generation feature was well-received, as it significantly reduced the time users spent creating study materials. The ability to explore related topics and generate cards on-the-fly enhanced the learning experience.
- Modular Architecture: The project’s modular structure allowed for easy updates and maintenance. Each component (e.g., card management, API integration) was well-defined, making it easier to debug and extend functionality.
- Community Engagement: Encouraging contributions through issues and pull requests fostered a sense of community around the project. This not only helped in identifying bugs but also in gathering new feature ideas.
3. What You’d Do Differently
- Enhanced Documentation: While the README provided a good starting point, more detailed documentation on the codebase and API usage would have been beneficial for new contributors. Including examples and a more comprehensive guide on extending features could improve onboarding.
- Testing Framework: Implementing a testing framework (e.g., Jest or React Testing Library) from the beginning would have ensured better code quality and reliability. This could help catch bugs early and provide confidence when making changes.
- User Feedback Mechanism: Adding a feedback mechanism within the app to gather user insights on features and usability could guide future development and improvements.
4. Advice for Others
- Start Small and Iterate: When building a project, start with a minimal viable product (MVP) and gradually add features based on user feedback. This approach helps in validating ideas without overcommitting resources.
- Focus on User Experience: Prioritize user experience in your design and functionality. Conduct user testing to understand pain points and areas for improvement.
- Leverage Community Resources: Don’t hesitate to seek help from the community. Platforms like GitHub, Stack Overflow, and relevant forums can provide valuable insights and support.
- Keep Learning: Technology evolves rapidly, so stay updated with the latest trends and best practices in web development. Continuous learning will help you improve your skills and the quality of your projects.
By reflecting on these aspects, you can gain valuable insights that can be applied to future projects and enhance your development practices.
What’s Next?
Conclusion
As we reach the current milestone of the AI闪卡学习助手 project, we are excited to share that the core functionalities are fully operational. Users can now effortlessly create, manage, and review their flashcards with the assistance of AI, thanks to the integration of the Deepseek API. The project has garnered positive feedback, and we are thrilled to see how it enhances the learning experience for users.
Looking ahead, we have ambitious plans for future development. We aim to expand the capabilities of the flashcard tool by introducing features such as collaborative study sessions, enhanced analytics for tracking learning progress, and support for multiple languages. Additionally, we are exploring the possibility of integrating gamification elements to make learning even more engaging. Your input and ideas are invaluable as we shape the future of this project.
We invite all contributors—developers, designers, and educators—to join us on this journey. Whether you have a feature in mind, want to report an issue, or simply wish to improve the documentation, your contributions will help us create a more robust and user-friendly tool. Please feel free to submit issues and pull requests on our GitHub repository.
In closing, the journey of developing AI闪卡学习助手 has been both challenging and rewarding. It has been a testament to the power of collaboration and innovation. We are excited about the road ahead and look forward to building a vibrant community around this project. Together, we can transform the way people learn and engage with information. Thank you for being a part of this adventure!
Project Development Analytics
timeline gant
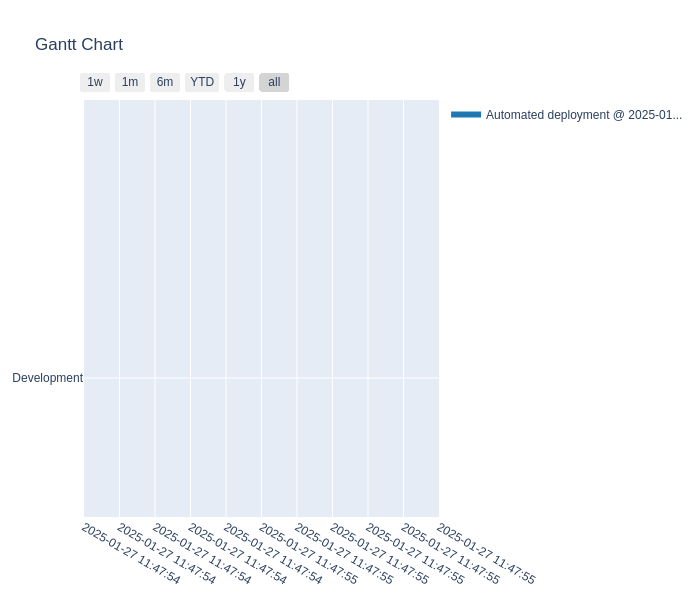
Commit timelinegant
Commit Activity Heatmap
This heatmap shows the distribution of commits over the past year:
Commit Heatmap
Contributor Network
This network diagram shows how different contributors interact:
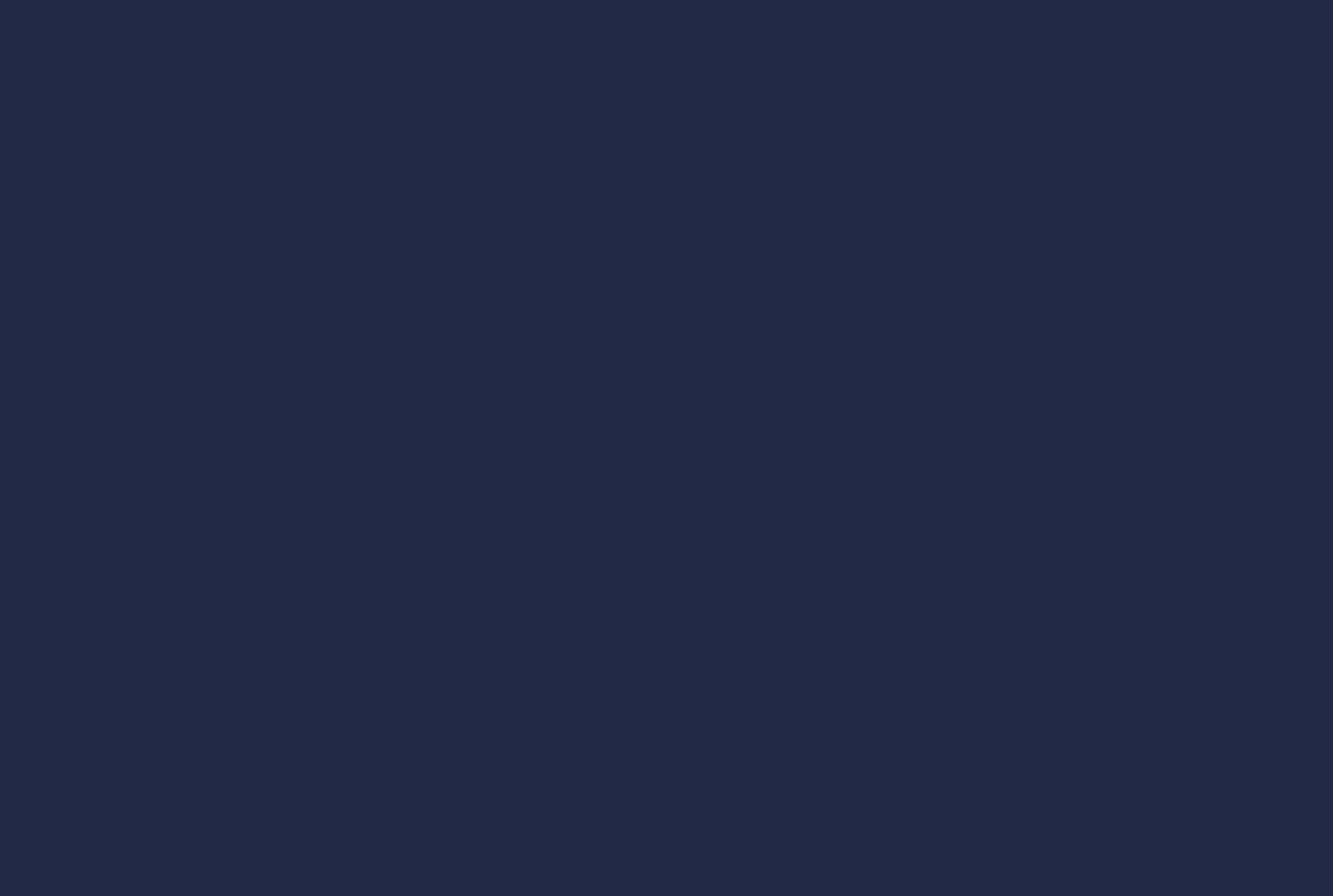
Contributor Network
Commit Activity Patterns
This chart shows when commits typically happen:
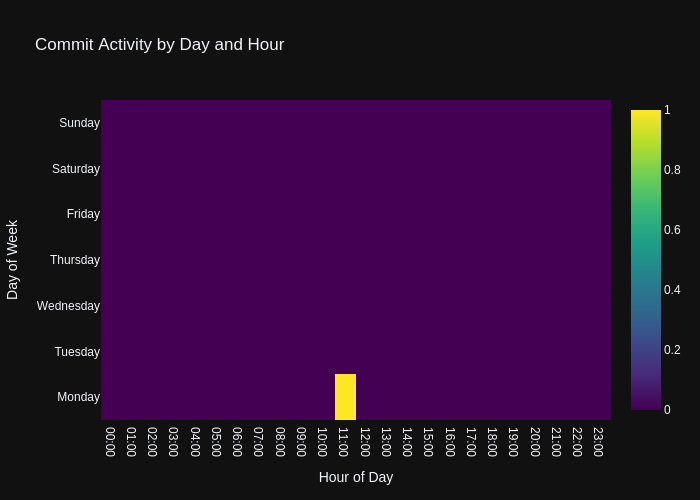
Commit Activity
Code Frequency
This chart shows the frequency of code changes over time:
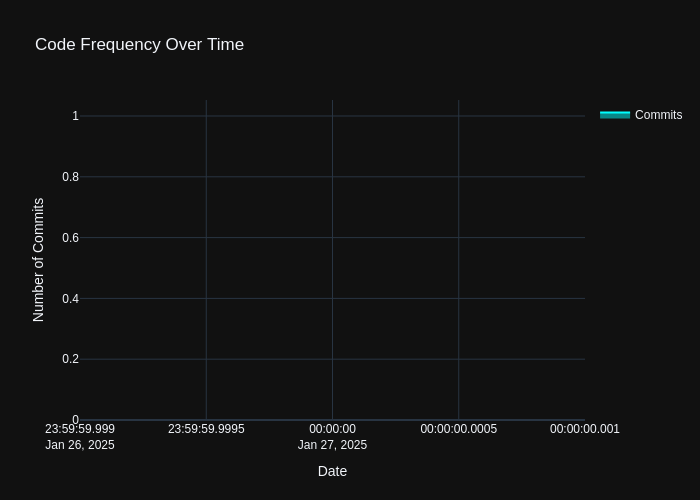
Code Frequency
- Repository URL: https://github.com/wanghaisheng/AI-flashcard-deepseek
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 2 月 3 日