Building AI-Overflow: Crafting a Modern Forum for Developers with Next.js
Project Genesis
Welcome to AI-Overflow: A New Era for Developer Collaboration
From Idea to Implementation
AI-OVERFLOW Project Journey: From Concept to Code
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
Next.js 14: We opted for Next.js due to its server-side rendering capabilities, which enhance performance and SEO. The framework’s flexibility allows for rapid development and easy integration of various features.
-
Next-Auth: For authentication, Next-Auth was chosen for its simplicity and robust support for various authentication providers. This decision was crucial for ensuring a secure and seamless user experience.
-
Prisma 5 and Postgres @ Neon: We selected Prisma as our ORM for its type safety and ease of use, which accelerates database interactions. Postgres was chosen for its reliability and performance, particularly with complex queries and large datasets.
-
TailwindCSS and ShadCn-UI: For styling, TailwindCSS was preferred for its utility-first approach, allowing for rapid UI development. ShadCn-UI provided pre-built components that align with our design goals, ensuring a consistent and modern look.
-
Lexical: We integrated Lexical for rich text editing capabilities, enabling users to create and format content easily, which is essential for a community-driven platform.
3. Alternative Approaches Considered
-
Monolithic vs. Microservices Architecture: Initially, we debated between a monolithic architecture and a microservices approach. While microservices offer scalability and flexibility, we opted for a monolithic structure for the MVP to simplify development and deployment. This decision allows us to focus on core features before potentially refactoring into microservices as the platform grows.
-
Different Database Solutions: We also explored NoSQL databases like MongoDB for their flexibility. However, we ultimately chose Postgres for its strong relational capabilities, which are essential for managing complex relationships between users, questions, and answers.
-
Alternative Frontend Frameworks: Other frameworks like Vue.js and Angular were considered, but Next.js stood out due to its performance benefits and the growing popularity within the developer community.
4. Key Insights That Shaped the Project
-
Community Engagement: Early engagement with potential users was invaluable. Their feedback not only shaped the feature set but also fostered a sense of ownership and anticipation for the platform.
-
AI Integration: The realization that AI could enhance user experience by providing personalized content and recommendations was a game-changer. This insight led to the incorporation of AI-driven features that set AI-OVERFLOW apart from traditional Q&A platforms.
-
Iterative Development: Emphasizing an iterative development approach allowed us to adapt quickly to feedback and changing requirements. This flexibility was crucial in refining features and improving the overall user experience.
-
Focus on User Experience: Prioritizing a clean, intuitive user interface was essential. We learned that a well-designed UI could significantly impact user engagement and satisfaction, making it a core focus throughout the development process.
Under the Hood
Technical Deep-Dive: AI-OVERFLOW
1. Architecture Decisions
Key Architectural Components:
- Frontend: Built with Next.js 14, which provides server-side rendering and static site generation capabilities, improving performance and SEO.
- Backend: Utilizes a RESTful API structure, allowing for clear separation between the client and server. This architecture facilitates easier updates and maintenance.
- Database: Prisma 5 is used as an ORM to interact with a PostgreSQL database hosted on Neon, providing type safety and a streamlined database management experience.
2. Key Technologies Used
- Next.js 14: A React framework that enables server-side rendering and static site generation, enhancing performance and user experience.
- Next-Auth: A robust authentication solution for Next.js applications, providing support for various authentication providers and session management.
- Prisma 5: An ORM that simplifies database interactions and provides a type-safe API for querying the database.
- PostgreSQL @ Neon: A cloud-native database solution that offers scalability and performance for handling application data.
- TailwindCSS: A utility-first CSS framework that allows for rapid UI development with a focus on responsiveness and customization.
- ShadCn-UI: A component library that provides pre-built UI components, speeding up the development process.
- Lexical: A powerful text editor framework that allows for rich text editing capabilities within the application.
3. Interesting Implementation Details
User Authentication with Next-Auth
pages/api/auth/[...nextauth].js
file:import NextAuth from "next-auth";
import Providers from "next-auth/providers";
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
// Add more providers as needed
],
database: process.env.DATABASE_URL,
});
Database Schema with Prisma
User
model is shown below:model User {
id Int @id @default(autoincrement())
name String?
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
content String
authorId Int
author User @relation(fields: [authorId], references: [id])
}
4. Technical Challenges Overcome
Performance Optimization
- Server-Side Rendering (SSR): Leveraging Next.js’s SSR capabilities to pre-render pages, reducing load times and improving SEO.
- Caching: Implementing caching strategies using Redis to store frequently accessed data, reducing database load and improving response times.
Handling Real-Time Collaboration
const socket = io();
socket.on("newPost", (post) => {
// Update the UI with the new post
});
Conclusion
Lessons from the Trenches
Key Technical Lessons Learned
-
Integration of Modern Technologies: Utilizing Next.js 14 and Prisma 5 allowed for a seamless development experience, especially with server-side rendering and database management. Understanding the nuances of these technologies was crucial for optimizing performance and scalability.
-
Authentication and Security: Implementing Next-Auth for user authentication highlighted the importance of security in web applications. It was essential to understand OAuth flows and session management to protect user data effectively.
-
UI/UX Design Principles: Using TailwindCSS and ShadCn-UI emphasized the significance of responsive design and component-based architecture. Learning how to create reusable components improved the overall maintainability of the codebase.
-
Real-time Collaboration: Integrating Lexical for rich text editing taught us about handling real-time data updates and collaborative features, which are vital for a community-driven platform.
What Worked Well
-
Community Engagement: The focus on community-driven content led to high user engagement and valuable contributions. Features like upvoting and commenting fostered interaction and knowledge sharing.
-
Modular Architecture: The use of a modular architecture with Next.js allowed for easy scaling and feature additions. This made it straightforward to implement new functionalities without disrupting existing ones.
-
Responsive Design: The implementation of TailwindCSS resulted in a highly responsive and visually appealing interface, enhancing user experience across devices.
-
Performance Optimization: Leveraging server-side rendering and static site generation in Next.js significantly improved load times and overall performance, which is critical for user retention.
What You’d Do Differently
-
Early User Feedback: In hindsight, gathering user feedback earlier in the development process would have provided insights that could shape features more effectively. Implementing a beta testing phase could have helped refine the user experience.
-
Documentation: While the README provides a good overview, more detailed documentation on setup and contribution guidelines would have facilitated onboarding for new developers and contributors.
-
Testing Frameworks: Incorporating automated testing frameworks earlier in the development cycle would have helped catch bugs and ensure code quality, especially as the project grew in complexity.
-
Scalability Planning: Planning for scalability from the outset, particularly in database design and API structure, could have mitigated some challenges faced as user traffic increased.
Advice for Others
-
Prioritize User Experience: Always keep the end-user in mind. Conduct user testing and gather feedback regularly to ensure the platform meets their needs and expectations.
-
Embrace Modern Tools: Don’t hesitate to adopt modern frameworks and libraries that can enhance development efficiency and user experience. Stay updated with the latest trends in web development.
-
Foster Community: Build a strong community around your project. Encourage contributions and create a welcoming environment for users to share knowledge and help each other.
-
Iterate and Adapt: Be prepared to iterate on your design and features based on user feedback and changing technology landscapes. Flexibility is key to long-term success.
What’s Next?
Conclusion: Looking Ahead for AI-Overflow
Project Development Analytics
timeline gant
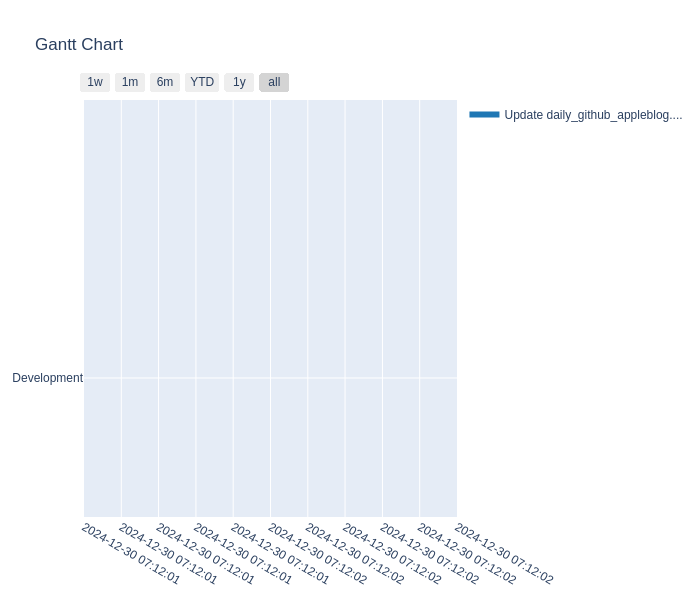
Commit Activity Heatmap
Contributor Network
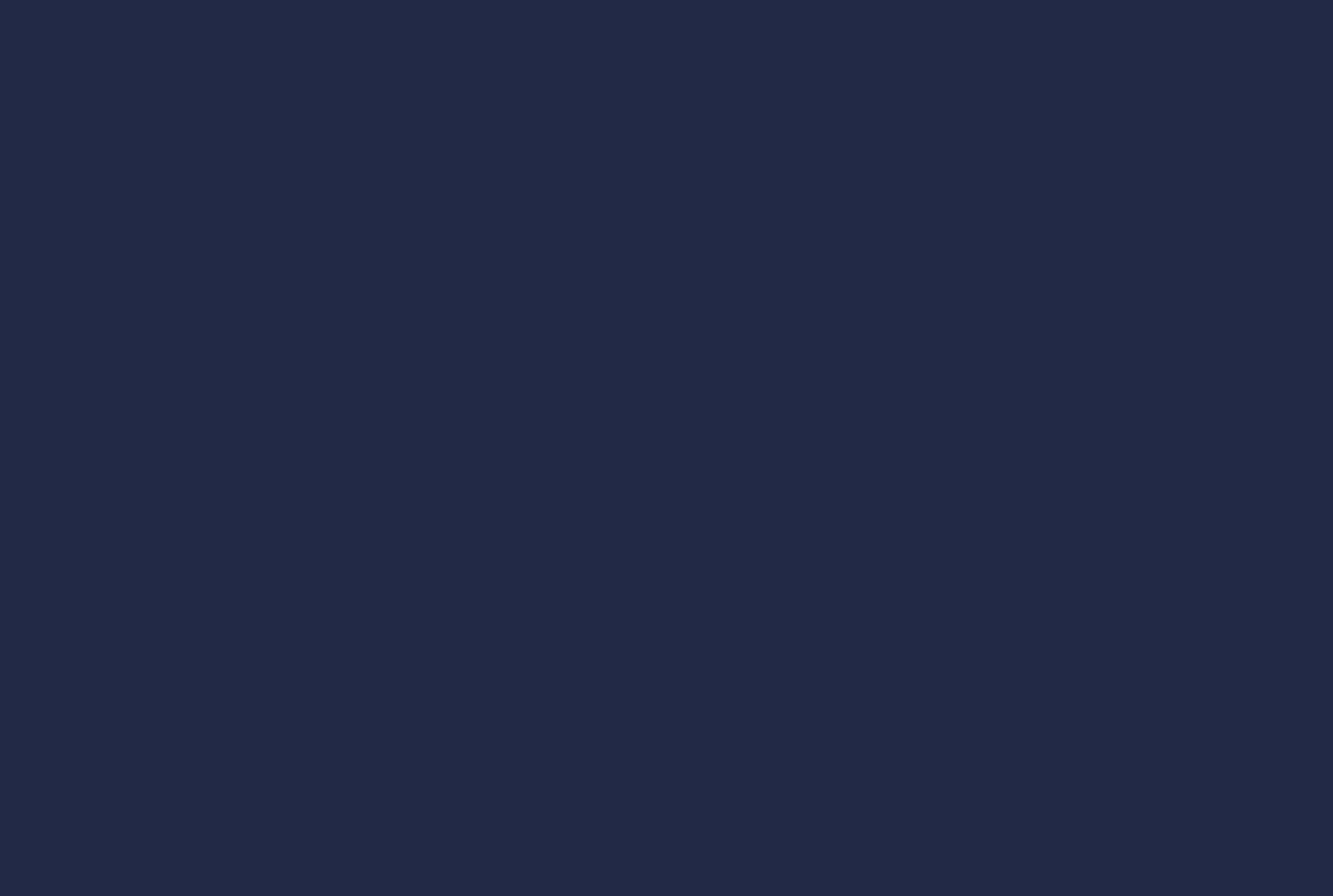
Commit Activity Patterns
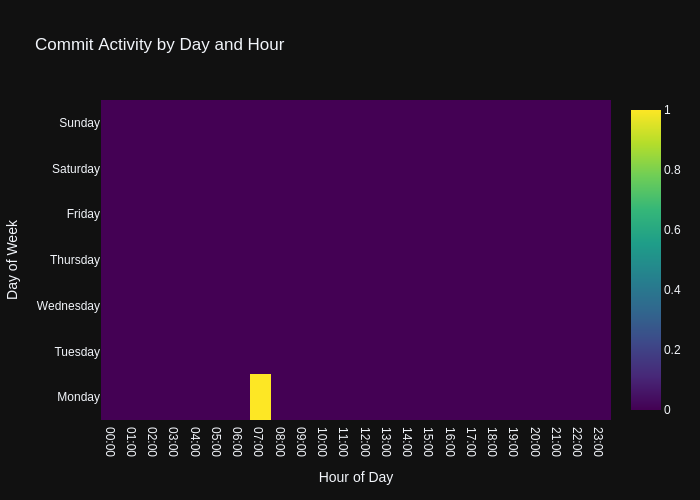
Code Frequency
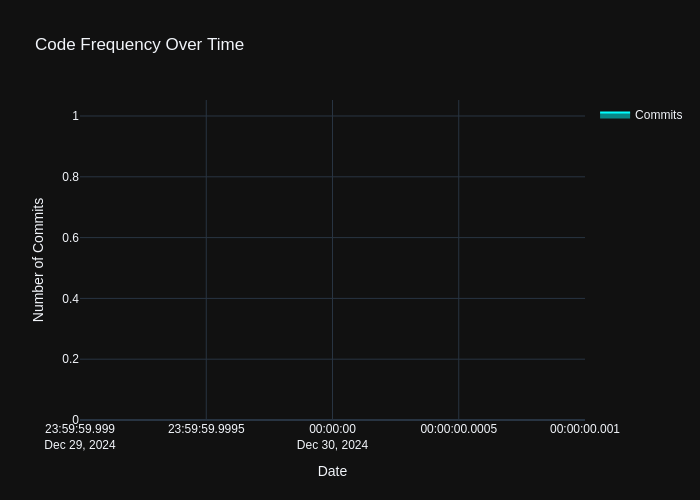
- Repository URL: https://github.com/wanghaisheng/AI-Overflow-Alternative-forum
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日