Building a Stunning 3D iPhone 15 Pro Landing Page with React & Three.js
Project Genesis
Crafting a 3D Experience: My Journey to Recreate the iPhone 15 Pro Landing Page
From Idea to Implementation
Journey from Concept to Code: iPhone 15 Pro Landing Page Replica
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
React: Chosen as the primary framework due to its component-based architecture, which allows for reusable UI components and efficient state management. This was crucial for maintaining a smooth user experience as the page would have multiple interactive elements.
-
Three.js: Selected for rendering the 3D model of the iPhone 15 Pro. Its powerful capabilities for 3D graphics in the browser made it the ideal choice for creating a lifelike representation of the device.
-
GSAP: The GreenSock Animation Platform was integrated to handle animations. Its performance and ease of use for creating complex animations were significant factors in this decision, as smooth transitions were essential for user engagement.
-
TypeScript: Implemented for its static type-checking features, which helped catch errors early in the development process and improved code maintainability.
-
Vite: Chosen as the build tool for its fast development server and efficient bundling capabilities, which streamlined the development workflow.
-
Tailwind CSS: Adopted for its utility-first approach to styling, allowing for rapid UI development and ensuring that the design remained responsive across different screen sizes.
3. Alternative Approaches Considered
-
Using Vanilla JavaScript: Initially, there was a consideration to build the project using plain JavaScript and CSS. However, this approach was quickly dismissed due to the complexity of managing state and interactions without a framework like React.
-
Other Animation Libraries: Other animation libraries such as Anime.js and Framer Motion were evaluated. However, GSAP was ultimately chosen for its superior performance and flexibility in handling complex animations.
-
Different CSS Frameworks: Frameworks like Bootstrap and Bulma were considered for styling. However, Tailwind CSS was preferred for its customization capabilities and the ability to create a unique design without being constrained by predefined components.
4. Key Insights That Shaped the Project
-
User Experience is Paramount: The importance of a seamless and engaging user experience became clear early on. Every decision, from the choice of technologies to the design elements, was made with the user in mind.
-
Performance Matters: As the project involved 3D rendering and animations, performance optimization became a key focus. This led to careful consideration of how assets were loaded and rendered, ensuring that the page remained responsive and fast.
-
Iterative Development: The project benefited from an iterative development approach. Regular testing and feedback loops allowed for continuous improvement of the user interface and interactions, leading to a more polished final product.
-
Collaboration and Communication: Throughout the project, effective communication among team members was crucial. Sharing insights and challenges helped in making informed decisions and fostering a collaborative environment.
Under the Hood
Technical Deep-Dive: iPhone 15 Pro Landing Page Replica
1. Architecture Decisions
Component Structure
- App Component: The main entry point that renders the entire application.
- Header Component: Contains navigation and branding elements.
- ModelViewer Component: Responsible for rendering the 3D model using Three.js.
- AnimationComponent: Manages animations using GSAP.
- Footer Component: Displays additional information and links.
2. Key Technologies Used
React
Three.js
import * as THREE from 'three';
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// Add a 3D model here
GSAP
import { gsap } from 'gsap';
gsap.to('.element', { duration: 1, x: 100, opacity: 1 });
TypeScript
interface ModelProps {
modelUrl: string;
position: [number, number, number];
}
Vite
Tailwind CSS
<div className="flex justify-center items-center h-screen bg-gray-100">
<h1 className="text-4xl font-bold">iPhone 15 Pro</h1>
</div>
3. Interesting Implementation Details
3D Model Interaction
const handleMouseMove = (event) => {
const mouseX = (event.clientX / window.innerWidth) * 2 - 1;
const mouseY = -(event.clientY / window.innerHeight) * 2 + 1;
// Update the model's rotation based on mouse position
model.rotation.y = mouseX * Math.PI;
model.rotation.x = mouseY * Math.PI;
};
window.addEventListener('mousemove', handleMouseMove);
Responsive Design
4. Technical Challenges Overcome
Performance Optimization
Cross-Browser Compatibility
Animation Synchronization
Lessons from the Trenches
Key Technical Lessons Learned
-
Integration of Multiple Libraries: Combining React with Three.js and GSAP required a deep understanding of how these libraries interact. Managing state and animations in a 3D context was challenging but taught me the importance of structuring components effectively to avoid performance bottlenecks.
-
TypeScript Benefits: Using TypeScript helped catch type-related errors early in the development process. It enforced better coding practices and improved code readability, making it easier to collaborate with others.
-
Responsive Design Principles: Implementing responsive design with Tailwind CSS highlighted the importance of mobile-first design. I learned how utility-first CSS can speed up the styling process while ensuring consistency across different screen sizes.
-
Performance Optimization: Rendering a 3D model in the browser can be resource-intensive. I learned to optimize performance by reducing the polygon count of the 3D model and using techniques like lazy loading for assets.
What Worked Well
-
Smooth Animations: The integration of GSAP for animations was a success. The animations were fluid and added a professional touch to the landing page, enhancing user engagement.
-
3D Model Interaction: The realistic 3D model created with Three.js was a standout feature. Users enjoyed the ability to rotate and zoom in on the iPhone 15 Pro, which made the experience immersive.
-
Development Speed with Vite: Vite’s fast hot module replacement (HMR) significantly improved development speed. The quick feedback loop allowed for rapid iteration and testing of features.
-
Tailwind CSS for Styling: Tailwind CSS made it easy to implement responsive designs quickly. The utility classes allowed for rapid prototyping and adjustments without writing custom CSS.
What You’d Do Differently
-
State Management: For larger projects, I would consider using a state management library like Redux or Zustand. While React’s built-in state management worked for this project, a more complex application might benefit from a dedicated solution.
-
Testing: I would implement more comprehensive testing, including unit tests and integration tests. Using tools like Jest and React Testing Library would help ensure the reliability of components and interactions.
-
Accessibility Considerations: I would focus more on accessibility from the start. Ensuring that the landing page is usable for people with disabilities is crucial, and I would incorporate ARIA roles and keyboard navigation early in the design process.
-
Documentation: While the README is informative, I would create more detailed documentation for future developers. This would include a guide on the project structure, component usage, and how to contribute.
Advice for Others
-
Plan Your Architecture: Before diving into coding, take the time to plan the architecture of your application. Consider how components will interact and how state will be managed. This will save time and reduce complexity later on.
-
Leverage Community Resources: Don’t hesitate to use community resources, such as tutorials and documentation, especially when working with complex libraries like Three.js and GSAP. The community can provide valuable insights and solutions to common problems.
-
Iterate and Test: Build iteratively and test frequently. This approach allows you to catch issues early and make adjustments based on user feedback, leading to a more polished final product.
-
Focus on User Experience: Always keep the user experience in mind. Interactive elements should enhance the experience, not detract from it. Prioritize usability and accessibility to ensure that your application is enjoyable for all users.
What’s Next?
Conclusion
Project Development Analytics
timeline gant
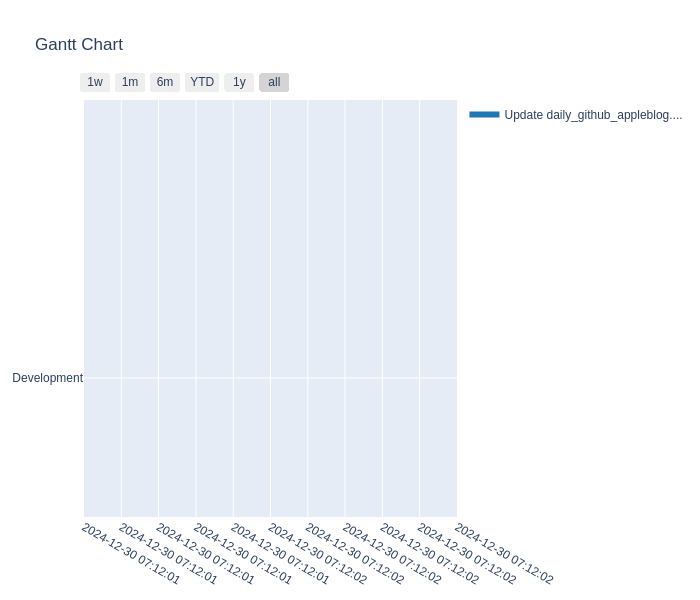
Commit Activity Heatmap
Contributor Network
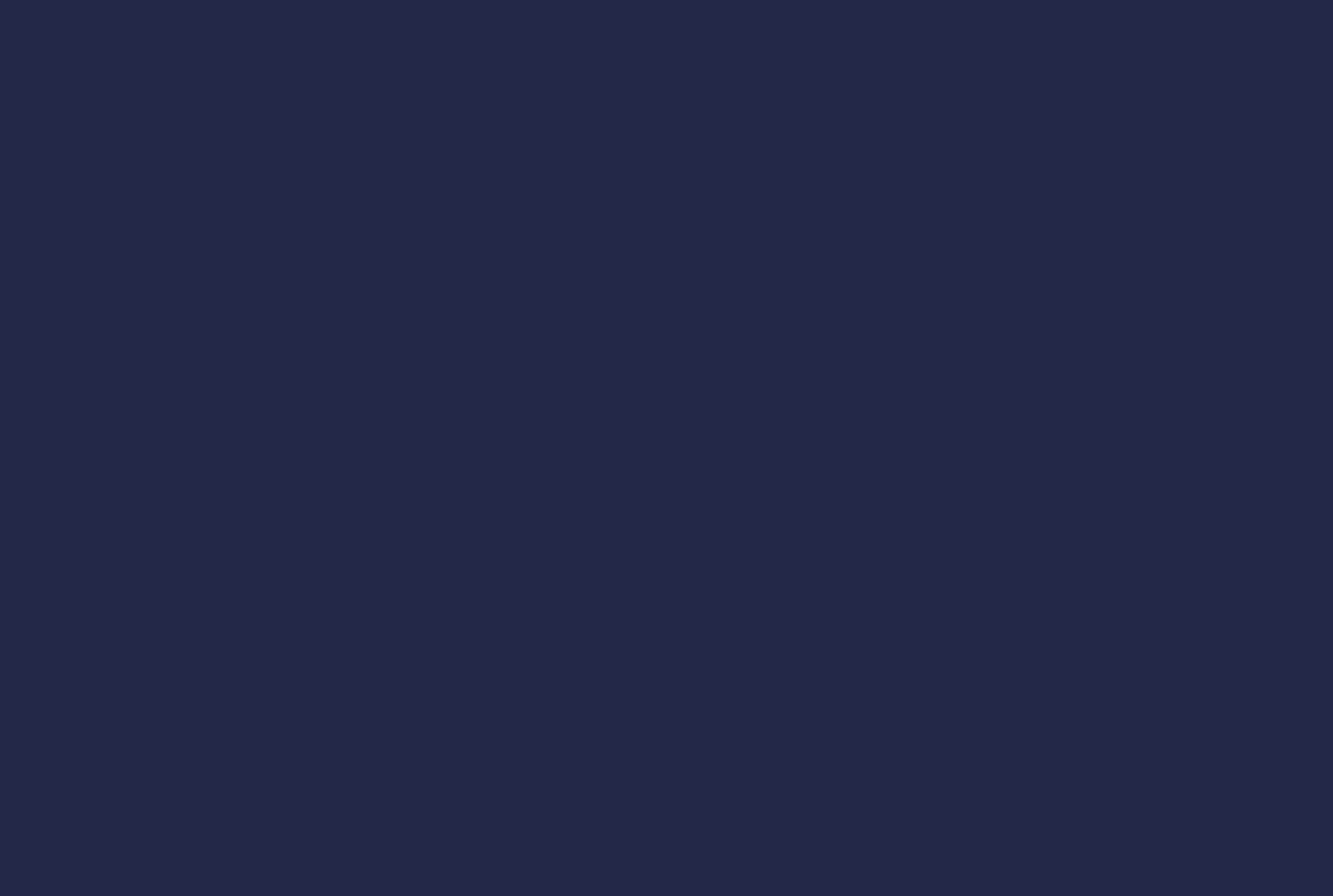
Commit Activity Patterns
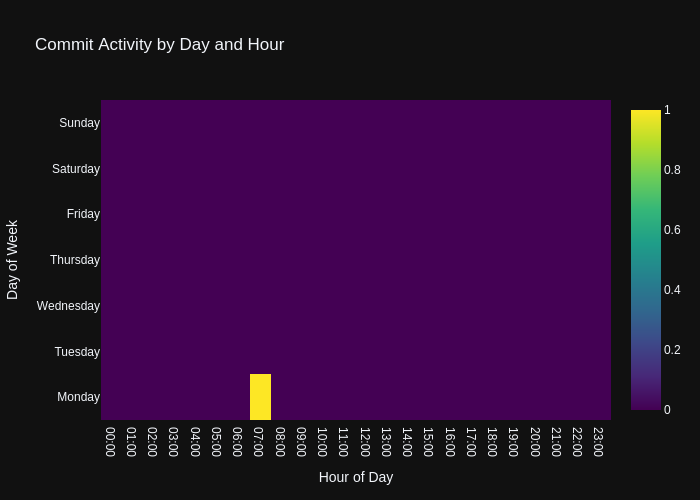
Code Frequency
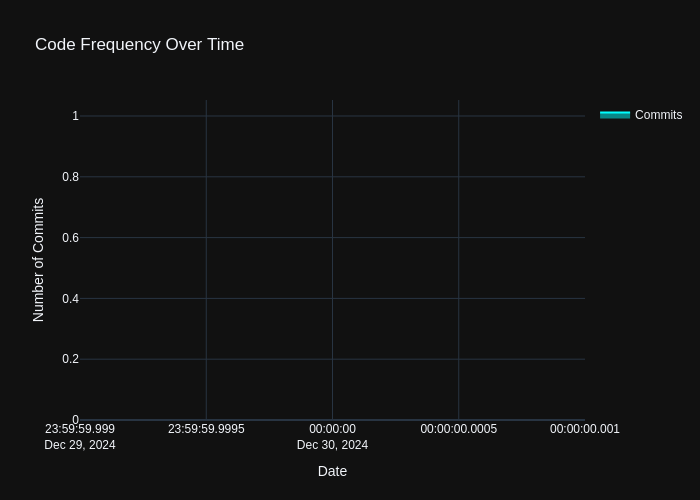
- Repository URL: https://github.com/wanghaisheng/3d-landingpage
- Stars: 1
- Forks: 0
编辑整理: Heisenberg 更新日期:2024 年 12 月 30 日