Building COCOS: A Developer's Journey to Connect Pet Owners and Vets
Project Genesis
Unleashing the Power of Community: The Journey Behind COCOS
From Idea to Implementation
1. Initial Research and Planning
2. Technical Decisions and Their Rationale
-
React: Selected for its component-based architecture, which promotes reusability and maintainability. This choice allows the team to build a modular application where components can be easily updated or replaced without affecting the entire system.
-
TypeScript: Adopted to enhance code stability and developer productivity. TypeScript’s static typing helps catch errors early in the development process, making the codebase more robust and easier to maintain.
-
Vanilla Extract: Chosen for styling to provide type-safe and modular CSS. This approach allows for better organization of styles and reduces the risk of style conflicts, which is crucial in a large application.
-
Tanstack Query: Implemented for data fetching and caching. This library simplifies server state management and provides built-in caching mechanisms, which improve the performance and responsiveness of the application.
-
Zustand: Selected for state management due to its simplicity and flexibility. It allows for intuitive state management without the boilerplate code often associated with other state management libraries.
-
Vercel: Chosen for deployment due to its ease of use and ability to provide a fast, global CDN. This ensures that users have a quick and reliable experience when accessing the platform.
3. Alternative Approaches Considered
-
Framework Alternatives: While React was the final choice, the team initially explored Vue.js and Angular. However, React’s large ecosystem and community support ultimately made it the preferred option.
-
State Management Libraries: Alternatives like Redux and MobX were considered for state management. However, the team opted for Zustand due to its minimalistic approach and ease of integration, which aligned better with the project’s goals.
-
Styling Solutions: The team also evaluated CSS-in-JS libraries like Styled Components and Emotion. Ultimately, Vanilla Extract was chosen for its type safety and modularity, which were deemed more beneficial for the project’s long-term maintainability.
4. Key Insights That Shaped the Project
-
User-Centric Design: The importance of involving users in the design process became evident. Regular feedback sessions with pet owners helped refine features and ensure that the platform met their needs effectively.
-
Community Engagement: The research underscored the value of community support for pet owners. The decision to include forums and discussion boards was driven by the understanding that shared experiences can provide comfort and valuable insights.
-
Scalability Considerations: As the project evolved, the team recognized the need for a scalable architecture. This realization led to the adoption of modular design principles and the use of libraries that facilitate easy scaling as the user base grows.
-
Iterative Development: The team embraced an iterative development approach, allowing for continuous improvement based on user feedback and testing. This flexibility enabled the team to adapt to changing requirements and enhance the platform progressively.
Under the Hood
Technical Deep-Dive on COCOS
1. Architecture Decisions
Key Architectural Choices:
- Component-Based Structure: Each feature of the application is encapsulated within its own component, promoting reusability and separation of concerns.
- State Management: Zustand is used for state management, providing a simple and flexible way to manage application state without the boilerplate often associated with Redux.
- API Layer: The application has a dedicated API layer to handle data fetching and caching, ensuring efficient communication with the backend services.
2. Key Technologies Used
- React: For building user interfaces with a component-based architecture.
- TypeScript: Enhances code quality and maintainability by providing static typing.
- Zustand: A minimalistic state management library that allows for easy state management without complex setups.
- Tanstack Query (React Query): For data fetching and caching, simplifying server state management.
- Vercel: For deployment, providing a seamless way to host and serve the application globally.
Example of State Management with Zustand:
import create from 'zustand';
const useStore = create((set) => ({
pets: [],
addPet: (pet) => set((state) => ({ pets: [...state.pets, pet] })),
removePet: (petId) => set((state) => ({ pets: state.pets.filter(pet => pet.id !== petId) })),
}));
3. Interesting Implementation Details
Component Structure
Example of a Component:
import React from 'react';
const PetCard = ({ pet }) => {
return (
<div className="pet-card">
<img src={pet.image} alt={pet.name} />
<h3>{pet.name}</h3>
<p>{pet.symptoms.join(', ')}</p>
</div>
);
};
export default PetCard;
Styling with Vanilla Extract
Example of a Styled Component:
import { style } from '@vanilla-extract/css';
export const petCardStyle = style({
border: '1px solid #ccc',
borderRadius: '8px',
padding: '16px',
textAlign: 'center',
});
4. Technical Challenges Overcome
Challenge: State Management Complexity
Challenge: API Integration
Example of Data Fetching with Tanstack Query:
import { useQuery } from 'react-query';
const useFetchPets = () => {
return useQuery('pets', async () => {
const response = await fetch('/api/pets');
if (!response.ok) throw new Error('Network response was not ok');
return response.json();
});
};
Challenge: Deployment and CI/CD
Conclusion
Lessons from the Trenches
1. Key Technical Lessons Learned
- Component-Based Architecture: Utilizing React for a component-based architecture significantly improved the maintainability and reusability of the code. It allowed for better organization of UI elements and made it easier to manage state and props.
- Type Safety with TypeScript: Implementing TypeScript helped catch errors at compile time rather than runtime, which improved code quality and reduced debugging time. It also facilitated better collaboration among team members by providing clear type definitions.
- State Management: Using Zustand for state management proved to be effective due to its simplicity and flexibility. It allowed for a straightforward implementation of global state without the boilerplate often associated with other state management libraries.
- Efficient Data Fetching: Tanstack Query (React Query) streamlined data fetching and caching, which improved the performance of the application. It simplified the process of synchronizing server state with the UI, reducing the need for manual data management.
- Version Control Best Practices: Adhering to a structured Git branching strategy and commit conventions enhanced collaboration and made it easier to track changes and manage releases.
2. What Worked Well
- Team Collaboration: The clear division of responsibilities among team members (e.g., specific views assigned to each member) fostered a collaborative environment and allowed for parallel development, which accelerated the project timeline.
- Use of Modern Tools: The choice of modern tools and libraries (e.g., Vercel for deployment, pnpm for package management) contributed to a smoother development process and improved performance.
- Documentation and Conventions: Maintaining comprehensive documentation, including coding conventions and commit message guidelines, helped ensure consistency across the codebase and made onboarding new team members easier.
3. What You’d Do Differently
- More Frequent Code Reviews: While the team had a good structure, implementing more frequent code reviews could have further improved code quality and knowledge sharing among team members.
- Automated Testing: Incorporating automated testing earlier in the development process would have helped catch bugs sooner and ensured that new features did not break existing functionality.
- User Feedback Loop: Establishing a more structured user feedback loop during the development phase could have provided valuable insights into user needs and preferences, leading to a more user-centered design.
4. Advice for Others
- Start with a Clear Plan: Before diving into development, take the time to outline the project scope, roles, and responsibilities. A clear plan helps align the team and sets expectations.
- Embrace Modern Technologies: Don’t hesitate to use modern frameworks and libraries that can enhance productivity and code quality. They often come with community support and extensive documentation.
- Prioritize Communication: Regular check-ins and updates among team members can help identify potential roadblocks early and keep everyone aligned on project goals.
- Iterate Based on Feedback: Be open to feedback from users and team members. Iterative development based on real-world usage can lead to a more robust and user-friendly product.
What’s Next?
Conclusion for COCOS Project
Project Development Analytics
timeline gant
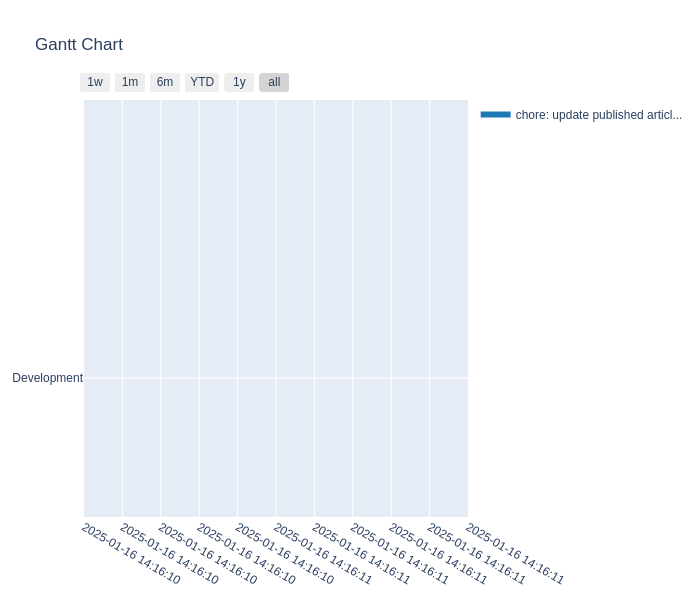
Commit Activity Heatmap
Contributor Network
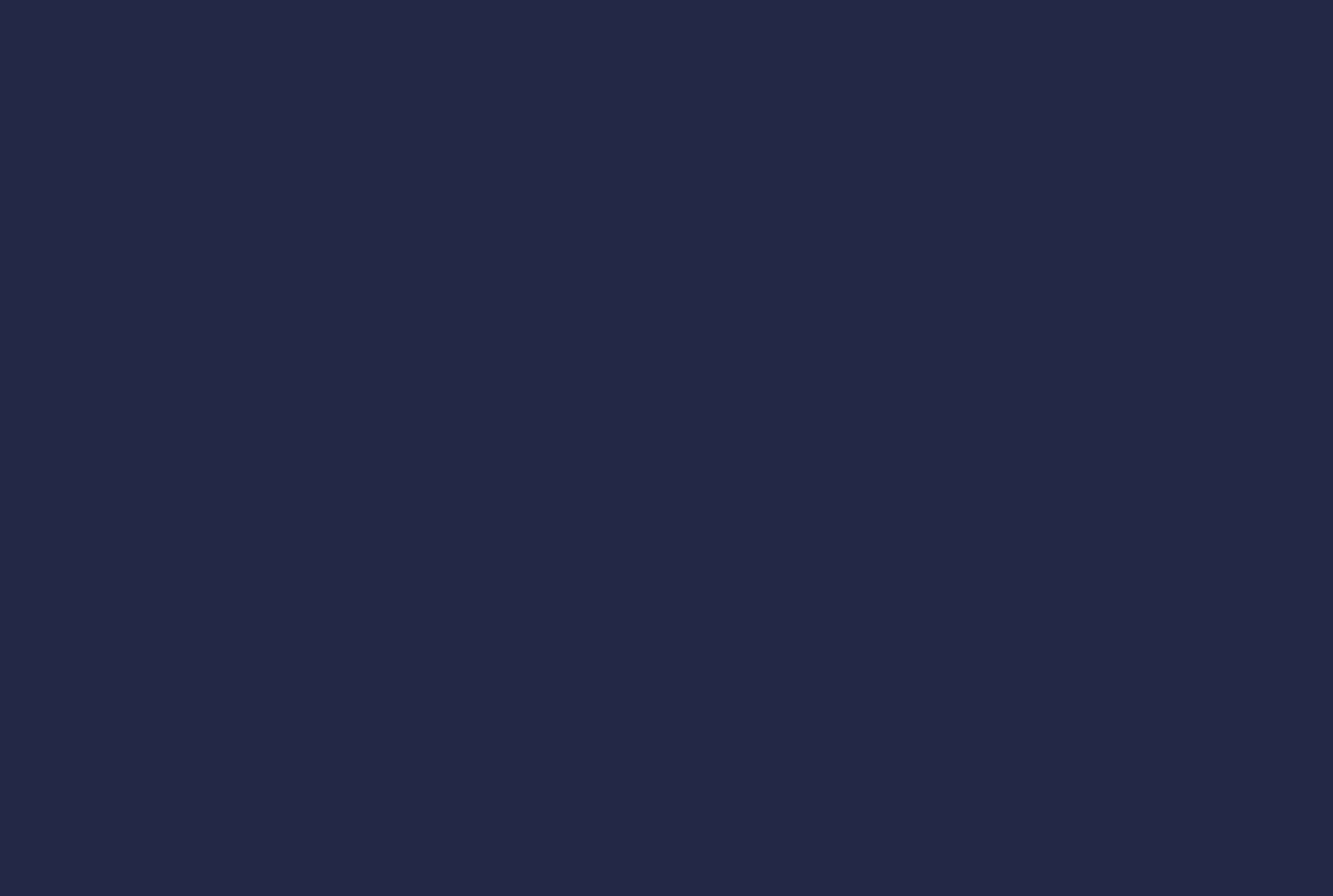
Commit Activity Patterns
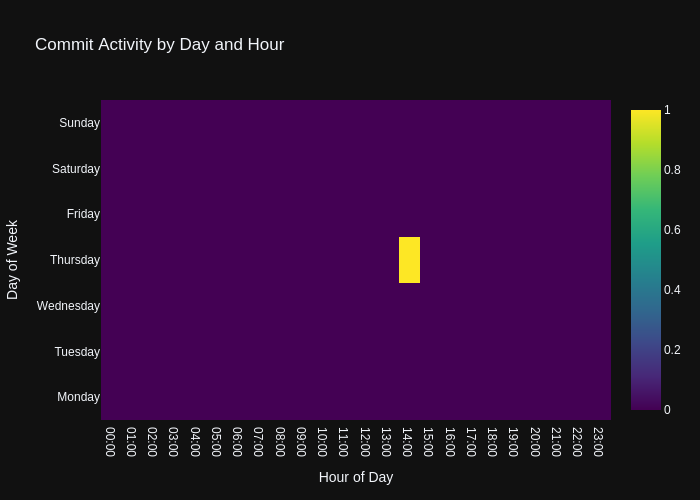
Code Frequency
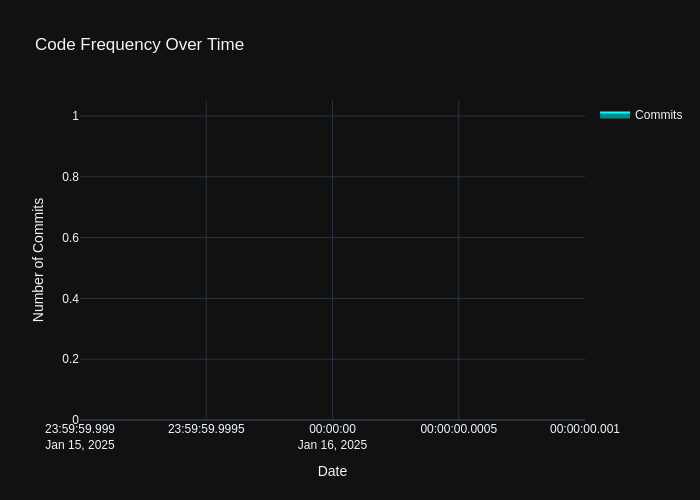
- Repository URL: https://github.com/wanghaisheng/35-APPJAM-WEB-COCOS
- Stars: 0
- Forks: 0
编辑整理: Heisenberg 更新日期:2025 年 1 月 20 日